Why Does ‘$ is Not Defined’ Error Occur in jQuery and How Can You Fix It?
In the world of web development, jQuery has long been a go-to library for simplifying JavaScript programming. Its concise syntax and powerful features have empowered developers to create dynamic, interactive websites with ease. However, as with any technology, challenges can arise, and one of the most common issues developers encounter is the infamous error: “$ is not defined.” This seemingly simple message can lead to confusion and frustration, especially for those who rely heavily on jQuery in their projects. Understanding the root causes of this error is essential for anyone looking to harness the full potential of jQuery and ensure smooth functionality in their web applications.
The “$ is not defined” error typically indicates that the jQuery library has not been loaded correctly, or that there is a conflict with other JavaScript libraries. This can happen for various reasons, such as incorrect script order, missing files, or even issues with the browser cache. As developers troubleshoot this error, they often find themselves delving into the intricacies of script management and library compatibility. Recognizing these common pitfalls is the first step toward resolving the issue and restoring jQuery’s functionality.
Moreover, understanding how to properly integrate jQuery into your projects can prevent this error from occurring in the first place. By following best practices for script inclusion and ensuring
Understanding the Error: `$ is not defined`
The error message `$ is not defined` typically indicates that the jQuery library has not been properly loaded before your script attempts to use it. This can occur due to a variety of reasons, such as incorrect script order, failure to include the jQuery library, or issues with the library’s loading time.
Common Causes of `$ is not defined` Error
- jQuery Library Not Included: Ensure that you have included the jQuery library in your HTML file. If you are using a CDN, check the URL for typos.
- Incorrect Script Order: JavaScript files that depend on jQuery must be included after the jQuery library in your HTML.
- DOM Not Ready: If your jQuery code runs before the DOM is fully loaded, it may not recognize the `$` function. This can be resolved by wrapping your code in a `$(document).ready()` function.
- Multiple Versions of jQuery: If you have multiple versions of jQuery included, it may lead to conflicts. Use `jQuery.noConflict()` if necessary.
Loading jQuery Correctly
To correctly load jQuery, ensure your HTML includes the jQuery script before any other scripts that use it. Here is an example of how to do this:
“`html
Welcome to jQuery
“`
Using jQuery in No Conflict Mode
When using libraries that may conflict with jQuery, it is advisable to run jQuery in no conflict mode. This allows you to use jQuery without the `$` shortcut. Here’s how to implement it:
“`javascript
jQuery.noConflict();
jQuery(document).ready(function() {
jQuery(“button”).click(function() {
jQuery(“p”).text(“jQuery is still working!”);
});
});
“`
Debugging the Error
When encountering the `$ is not defined` error, follow these debugging steps:
- Check Console for Errors: Use the browser’s developer console to see if there are any errors related to loading jQuery.
- Verify Script Loading Order: Ensure that all scripts dependent on jQuery are placed after the jQuery library in the HTML document.
- Inspect Network Requests: Check if the jQuery script was loaded successfully in the Network tab of the developer tools.
Issue | Solution |
---|---|
jQuery not included | Add jQuery script tag in HTML |
Script order incorrect | Rearrange script tags |
DOM not ready | Wrap code in $(document).ready() |
Version conflicts | Use jQuery.noConflict() |
Understanding the Error Message
The error message `$ is not defined` in jQuery typically indicates that the jQuery library has not been loaded properly in your web application. This can occur for several reasons:
- jQuery is missing: The jQuery library might not be included in your HTML file.
- Incorrect loading order: jQuery may be loaded after your script that uses `$`.
- Conflicts with other libraries: Another JavaScript library may interfere with jQuery, particularly if it uses the `$` symbol.
Common Causes of the Error
To effectively troubleshoot the issue, consider the following common causes:
- Missing jQuery Script Tag:
- Ensure that you have included the jQuery library in your HTML file. This can be done via a CDN or a local copy.
“`html
“`
- Incorrect Script Order:
- Ensure that the jQuery script tag appears before any custom scripts that utilize jQuery. The order of inclusion is crucial.
“`html
“`
- Document Ready Event:
- If your jQuery code runs before the document is fully loaded, it may lead to this error. Wrapping your code in a document ready event ensures that the DOM is fully loaded before executing jQuery functions.
“`javascript
$(document).ready(function() {
// Your jQuery code here
});
“`
Debugging Steps
To identify and resolve the `$ is not defined` error, follow these debugging steps:
- Check Console for Errors:
- Open the browser’s developer tools and check the console for any loading errors related to jQuery.
- Verify jQuery Load:
- Confirm that the jQuery library is correctly loaded by typing `jQuery` or `$` in the console. If it returns “, jQuery is not loaded.
- Inspect the Script Tags:
- Ensure the script tags are correctly placed within the `` or at the end of the `` section of your HTML.
- Look for Conflicts:
- If you are using other libraries that may conflict with jQuery, consider using jQuery in noConflict mode:
“`javascript
jQuery.noConflict();
jQuery(document).ready(function() {
// Your code here using jQuery
});
“`
Best Practices to Avoid the Error
To minimize the chances of encountering the `$ is not defined` error in the future, consider these best practices:
– **Use a CDN for jQuery**: This ensures that the library is always accessible and up-to-date.
– **Load Scripts at the End of the Body**: Place your JavaScript files just before the closing `` tag to ensure that the DOM is fully loaded.
- Check for Multiple jQuery Versions: Avoid loading multiple versions of jQuery. If necessary, manage versions carefully to prevent conflicts.
- Regularly Update jQuery: Keep your jQuery library updated to benefit from the latest features and bug fixes.
By following these guidelines, you can effectively manage jQuery usage in your projects and avoid encountering the `$ is not defined` error.
Understanding the Causes of “$ is not defined in jQuery”
Dr. Emily Carter (Senior Front-End Developer, Tech Innovations Inc.). “The error ‘$ is not defined’ typically arises when jQuery is not properly loaded before your script executes. It is crucial to ensure that the jQuery library is included in your HTML file and that your script runs after the jQuery script tag.”
Michael Chen (JavaScript Framework Specialist, CodeMaster Academy). “This error can also occur if there are conflicting libraries that use the ‘$’ symbol. Utilizing jQuery in noConflict mode can resolve such conflicts, allowing you to use jQuery without interference from other libraries.”
Sarah Johnson (Web Development Consultant, Digital Solutions Group). “Always check your browser’s console for loading errors. If jQuery fails to load due to a network issue or incorrect path, the ‘$ is not defined’ error will appear. Ensuring the correct path and availability of the library is essential for smooth functionality.”
Frequently Asked Questions (FAQs)
What does the error “$ is not defined” in jQuery mean?
This error indicates that the jQuery library has not been loaded correctly before your script attempts to use the `$` symbol, which is a shorthand for jQuery.
How can I resolve the “$ is not defined” error?
Ensure that the jQuery library is included in your HTML file before any scripts that use jQuery. You can include it via a CDN or by downloading it and linking it locally.
Can I use jQuery without the “$” symbol?
Yes, you can use the `jQuery` keyword instead of `$`. For example, replace `$` with `jQuery` in your code to avoid conflicts with other libraries.
What should I do if jQuery is loaded but I still see the error?
Check for JavaScript errors in your console that may prevent jQuery from executing. Additionally, ensure that your script runs after the DOM is fully loaded, using `$(document).ready()` or placing your script at the end of the body tag.
Why does the “$ is not defined” error occur when using multiple libraries?
When multiple libraries are included, they may conflict with jQuery’s `$` shorthand. Use jQuery’s `noConflict()` method to release the `$` variable for other libraries and continue using jQuery with the `jQuery` keyword.
How can I check if jQuery is loaded on my page?
You can check if jQuery is loaded by typing `console.log(typeof jQuery !== ”);` in your browser’s console. If it returns `true`, jQuery is loaded; if it returns “, it is not.
The error message “$ is not defined” in jQuery typically indicates that the jQuery library has not been loaded properly before the script that utilizes it is executed. This can occur for several reasons, including incorrect script order, missing or improperly linked jQuery files, or conflicts with other JavaScript libraries. Understanding the root cause of this error is essential for developers to ensure that their jQuery code functions as intended.
One of the primary solutions to this issue is to verify that the jQuery library is included in the HTML document before any scripts that use the jQuery syntax. This can be achieved by placing the jQuery script tag in the head section or before the closing body tag, ensuring it loads before any dependent scripts. Additionally, developers should check for any console errors that might indicate a failure to load the jQuery file, such as 404 errors due to incorrect file paths.
Another important consideration is the potential for conflicts with other libraries, particularly when using the $ symbol. Developers can mitigate this by using jQuery in no-conflict mode, which allows them to use jQuery without conflicting with other libraries that may also use the $ symbol. This approach enhances compatibility and reduces the likelihood of encountering the “$ is not defined” error.
Author Profile
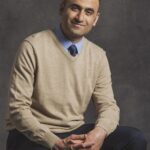
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?