Is ‘None’ in Python Really None? Understanding the Concept of ‘is not None’
In the world of Python programming, understanding how to handle variables and their values is crucial for writing effective and error-free code. Among the many concepts that developers encounter, the notion of “None” stands out as a fundamental aspect of the language. The phrase “is not None” often surfaces in discussions about conditionals, data validation, and function returns, making it an essential topic for both novice and experienced programmers alike. This article delves into the significance of checking for None in Python, exploring its implications and best practices to ensure your code runs smoothly.
At its core, None is a special constant in Python that signifies the absence of a value or a null value. It serves as a placeholder, indicating that a variable has been defined but does not yet hold any meaningful data. Understanding how to effectively check if a variable is not None can help developers avoid common pitfalls, such as attempting to perform operations on uninitialized variables or misinterpreting the flow of data within their applications. By leveraging the “is not None” condition, programmers can create robust and reliable code that gracefully handles various scenarios.
As we navigate through the intricacies of this concept, we’ll uncover the practical applications of checking for None, including its role in function returns, conditional statements, and data integrity. Whether you’re
Understanding `is not None` in Python
In Python, the expression `is not None` is a crucial aspect of condition checking, particularly when dealing with variables that might not hold a value. This expression evaluates whether a variable is not equivalent to the special value `None`, which signifies the absence of a value or a null value in Python.
When you want to ascertain that a variable holds a valid or meaningful value, using `is not None` is the preferred approach due to its clarity and specificity. It ensures that your code behaves as expected without ambiguity.
Use Cases for `is not None`
The `is not None` check is commonly used in several scenarios:
- Function Arguments: To verify that an argument passed to a function is not `None`, ensuring that the function can operate correctly.
- Conditional Logic: In if-statements, to control the flow based on the presence of a value.
- Data Validation: To validate if data received from user input, APIs, or databases is present and usable.
Here is a basic example:
python
def process_data(data):
if data is not None:
# Process the data
print(“Processing:”, data)
else:
print(“No data provided.”)
Comparison with Other Methods
Using `is not None` provides a more explicit check compared to other methods such as using equality operators (`!=`). Below is a comparison of different approaches:
Method | Example | Notes |
---|---|---|
Using `is not None` | `if variable is not None:` | Preferred for clarity and intent. |
Using `!=` | `if variable != None:` | Less explicit; can lead to unexpected behavior with overloaded operators. |
Using truthiness | `if variable:` | Checks for any falsy value (0, [], ”, None, etc.), which may not be desired. |
Performance Considerations
In terms of performance, using `is not None` is efficient as it directly checks the identity of the object. This method is faster than using equality checks, especially in critical performance-sensitive applications. While the performance difference is often negligible for most use cases, it can accumulate in scenarios involving large data processing or frequent checks.
Common Pitfalls
While `is not None` is straightforward, developers should be aware of a few common pitfalls:
- Overlooking Other Falsy Values: A variable may be “, `0`, or an empty collection, which can lead to confusion if only `if variable:` is used.
- Misinterpretation of `None`: In certain contexts, `None` may be a valid state (e.g., to indicate the end of data). Ensure that using `is not None` aligns with the logic of your application.
By employing `is not None` judiciously in your Python code, you can create more robust and clear applications.
Understanding `is not None` in Python
In Python, `None` is a special constant that represents the absence of a value or a null value. The expression `is not None` is commonly used to check if a variable has been assigned a value other than `None`. This is an important concept in Python programming, particularly when dealing with default parameters, optional values, or when you need to validate inputs.
Usage of `is not None`
The expression `is not None` is generally used in conditional statements. Here are some key points regarding its usage:
- Type Checking: Ensures that a variable is not `None` before performing operations.
- Control Flow: Often used to direct the flow of logic in functions, such as when validating user input or configuration settings.
- Avoiding Errors: Prevents `AttributeError` by ensuring that operations are performed only on objects that are not `None`.
Examples of `is not None` in Action
Consider the following scenarios where `is not None` is utilized:
python
def process_data(data):
if data is not None:
# Proceed with processing
print(“Processing data…”)
else:
print(“No data provided.”)
In this example, the function `process_data` checks if the `data` argument is not `None` before attempting to process it.
Another common use case is filtering data:
python
values = [1, 2, None, 4, None, 5]
filtered_values = [value for value in values if value is not None]
print(filtered_values) # Output: [1, 2, 4, 5]
This list comprehension filters out `None` values from the original list.
Comparative Analysis: `is not None` vs. Other Comparisons
In Python, there are multiple ways to check for the presence of a value. Here is a comparison between `is not None`, `!= None`, and other methods:
Method | Description | Best Practices |
---|---|---|
`is not None` | Checks identity with `None`, recommended for clarity and performance. | Preferred for checking against `None`. |
`!= None` | Checks equality, may cause issues with objects that override equality. | Less preferred; use `is not None` instead. |
`if variable:` | Evaluates truthiness; fails for variables that can be (0, “”, ). | Use when checking for existence in broader contexts. |
Common Pitfalls
When using `is not None`, developers may encounter some common pitfalls:
- Unintended Comparisons: Confusing `None` with other falsy values (e.g., `0`, “, `””`). Ensure clarity in logic.
- Immutable Types: Using `is not None` on immutable types without understanding their behavior can lead to unexpected results.
- Function Defaults: Be cautious when using `None` as a default parameter in function definitions; it may lead to mutable default issues.
Best Practices
To effectively use `is not None`, consider the following best practices:
- Clear Intent: Use `is not None` for clarity in your code, especially when checking for the absence of a value.
- Consistent Checks: Apply the same pattern of checks throughout your codebase to maintain readability.
- Documentation: Document your functions clearly to indicate that `None` is an expected input and how it should be handled.
By adhering to these practices, you can ensure that your code remains robust and maintainable, effectively managing the presence or absence of values in Python.
Understanding the Importance of `is not None` in Python Programming
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “In Python, checking if a variable is not None is crucial for ensuring that your code behaves as expected. It prevents runtime errors and helps maintain the integrity of data throughout the application lifecycle.”
Michael Chen (Lead Data Scientist, Tech Innovations Inc.). “Using `is not None` is a best practice in Python programming. It allows developers to explicitly verify that a variable holds a meaningful value before proceeding with operations that depend on that variable, thus enhancing code reliability.”
Sarah Johnson (Python Instructor, Code Academy). “For beginners, understanding the difference between None and other data types is vital. The `is not None` check serves as a foundational concept that reinforces the importance of type checking in Python, ultimately leading to better coding habits.”
Frequently Asked Questions (FAQs)
What does “is not None” mean in Python?
“Is not None” is a conditional expression used in Python to check if a variable does not hold the value of None, which signifies the absence of a value or a null reference.
How do you use “is not None” in a Python if statement?
You can use “is not None” in an if statement to execute a block of code only when the variable is not None. For example: `if variable is not None: # execute code`.
Why is “is not None” preferred over “!=” for checking None?
Using “is not None” is preferred because it checks for identity, ensuring that the variable is not the singleton None, while “!=” checks for equality, which may lead to unexpected results with custom objects.
Can “is not None” be used with other data types in Python?
Yes, “is not None” can be used with any data type in Python to verify that a variable is not assigned the value None, regardless of whether it is a string, list, dictionary, or custom object.
What happens if you use “is not None” on a variable that has not been defined?
Using “is not None” on an undefined variable will raise a NameError in Python, as the variable must be defined before it can be checked against None.
Is “is not None” a common practice in Python programming?
Yes, using “is not None” is a common and recommended practice in Python programming for clarity and to avoid ambiguity when checking for the absence of a value.
In Python, the expression “is not None” is a crucial part of the language’s approach to handling null values. The keyword “None” represents the absence of a value or a null value in Python, and using “is not None” allows developers to check if a variable contains a meaningful value. This is particularly important in scenarios where the presence or absence of a value significantly impacts the flow of a program. Understanding how to use this expression effectively can help prevent errors and improve the robustness of code.
One key takeaway is that using “is not None” is preferred over equality checks (e.g., “!=”) when determining if a variable is not null. This is because “is” and “is not” check for identity, which is more reliable for singleton objects like None. Additionally, this practice aligns with Python’s philosophy of explicitness, making the code easier to read and understand. Developers should consistently apply this pattern to ensure clarity in their codebase.
Moreover, the use of “is not None” is not just a syntactical choice; it reflects a broader understanding of how Python manages memory and object identity. By leveraging this expression, developers can write cleaner, more efficient code that adheres to best practices.
Author Profile
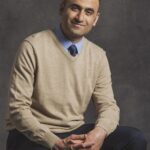
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?