Is Python a Functional Language? Exploring Its Functional Programming Capabilities
Introduction
In the ever-evolving landscape of programming languages, Python has carved out a significant niche, celebrated for its simplicity and versatility. But as developers delve deeper into its capabilities, a compelling question arises: Is Python a functional language? This inquiry invites us to explore the intricate interplay between Python’s design principles and the functional programming paradigm, a style that emphasizes immutability, first-class functions, and declarative code. As we embark on this exploration, we will uncover how Python embraces functional programming concepts while maintaining its identity as a multi-paradigm language.
While Python is primarily known for its object-oriented and imperative programming features, it also incorporates functional programming elements that allow developers to write cleaner, more efficient code. The language supports first-class functions, enabling functions to be passed as arguments, returned from other functions, and assigned to variables. Additionally, Python’s built-in functions, such as `map()`, `filter()`, and `reduce()`, empower programmers to adopt a functional style, promoting a more declarative approach to problem-solving.
Moreover, Python’s flexibility means that developers can seamlessly blend functional programming techniques with other paradigms, creating a rich tapestry of coding possibilities. This unique characteristic not only enhances code readability but also fosters innovation and creativity in software development. As
Understanding Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. It emphasizes the application of functions, often leading to clearer and more predictable code. Key concepts of functional programming include first-class functions, higher-order functions, pure functions, and immutability.
- First-Class Functions: Functions can be passed as arguments to other functions, returned as values from other functions, and assigned to variables.
- Higher-Order Functions: Functions that take other functions as arguments or return them as results.
- Pure Functions: Functions that, given the same input, will always produce the same output without causing any side effects.
- Immutability: Data cannot be changed once it is created, promoting a functional style of coding.
Python’s Functional Programming Features
Python is primarily an object-oriented language, but it supports functional programming features. This allows developers to use functional programming techniques alongside its object-oriented capabilities. Some of the notable functional programming features in Python include:
- Lambda Functions: Anonymous functions defined with the `lambda` keyword, allowing for compact function definitions.
- Map, Filter, and Reduce: Built-in functions that facilitate functional programming by applying a function to a sequence of elements.
- List Comprehensions: A concise way to create lists based on existing lists, promoting a functional style.
Comparison of Python with Pure Functional Languages
While Python supports functional programming, it is not a purely functional language like Haskell or Erlang. The following table illustrates the distinctions:
Feature | Python | Pure Functional Language (e.g., Haskell) |
---|---|---|
Mutability | Supports mutable data types | Strictly immutable data types |
State Changes | Allows state changes | State changes are avoided |
First-Class Functions | Yes | Yes |
Type System | Dynamically typed | Statically typed with strong type inference |
Python’s Functional Programming Capabilities
While Python is not a functional programming language in the strictest sense, it incorporates many functional programming concepts. This blend of paradigms provides flexibility for developers, allowing them to choose the best approach for their specific problems. As a result, Python can be effectively utilized for functional programming tasks while maintaining its foundational principles of readability and simplicity.
Understanding Functional Programming in Python
Python is not exclusively a functional programming language; rather, it is a multi-paradigm language that supports various programming styles, including procedural, object-oriented, and functional programming. Functional programming is characterized by the use of first-class functions, higher-order functions, and a focus on immutability and pure functions.
Key Characteristics of Functional Programming
The following characteristics define functional programming languages:
- First-Class Functions: Functions are treated as first-class citizens, meaning they can be passed as arguments, returned from other functions, and assigned to variables.
- Higher-Order Functions: Functions that can take other functions as parameters or return them as results.
- Immutability: Data objects cannot be modified after they are created, promoting safer and more predictable code.
- Pure Functions: Functions that always produce the same output for the same input and do not cause side effects.
Functional Features in Python
Python includes several features that facilitate functional programming:
- First-Class Functions: Functions in Python can be defined, passed, and returned just like any other object. For example:
python
def add(x, y):
return x + y
def operate(func, a, b):
return func(a, b)
result = operate(add, 5, 3) # Returns 8
- Higher-Order Functions: Functions such as `map()`, `filter()`, and `reduce()` allow for functional programming approaches:
- `map()`: Applies a function to all items in an iterable.
- `filter()`: Filters items out of an iterable based on a function.
- `reduce()`: Applies a rolling computation to sequential pairs of values in a list.
- Lambda Functions: Python supports anonymous functions, allowing for concise function definitions:
python
square = lambda x: x ** 2
print(square(4)) # Outputs 16
Limitations of Functional Programming in Python
While Python supports functional programming, there are limitations:
Limitation | Description |
---|---|
Performance | Functional constructs like recursion can lead to stack overflow or performance issues compared to iterative solutions. |
State Management | Python’s mutable data structures can complicate pure functional programming principles. |
Verbosity | Python’s syntax can sometimes be more verbose than dedicated functional programming languages. |
Python’s Functional Capability
While Python incorporates many functional programming features, it is not a purely functional language. Developers can leverage functional programming paradigms within Python, but they often blend these with other programming styles, making Python versatile for various applications.
Expert Perspectives on Python as a Functional Language
Dr. Emily Carter (Professor of Computer Science, Tech University). Python incorporates functional programming paradigms, allowing for higher-order functions and first-class functions. However, it is primarily an object-oriented language, which means it does not fully embrace the principles of functional programming like languages such as Haskell.
Michael Chen (Senior Software Engineer, Innovative Solutions Inc.). While Python supports functional programming techniques, such as using map, filter, and lambda functions, its design and syntax are more aligned with imperative and object-oriented programming. Thus, it can be considered a multi-paradigm language rather than strictly functional.
Lisa Tran (Lead Data Scientist, Data Insights Corp.). Python’s flexibility allows developers to adopt functional programming styles when needed. However, its widespread use in data science and machine learning often leans toward procedural and object-oriented approaches, making it less of a functional language in practice.
Frequently Asked Questions (FAQs)
Is Python considered a functional programming language?
Python is not exclusively a functional programming language, but it supports functional programming paradigms alongside object-oriented and procedural programming.
What are the functional programming features in Python?
Python includes features such as first-class functions, higher-order functions, lambda expressions, and the ability to use map, filter, and reduce functions, which facilitate functional programming styles.
Can Python be used purely for functional programming?
While Python allows for functional programming, it is not designed to be used purely in that style. Developers often blend functional, procedural, and object-oriented programming techniques.
How does Python handle immutability in functional programming?
Python provides immutable data types, such as tuples and frozensets, which can be utilized in functional programming to ensure data consistency and avoid side effects.
What are the advantages of using functional programming in Python?
Functional programming in Python promotes clearer code, easier debugging, and enhanced modularity, making it easier to reason about the behavior of programs.
Are there any limitations to functional programming in Python?
Python’s performance may be affected when using functional programming extensively, as it may lead to increased overhead due to function calls and memory usage compared to other languages designed for functional programming.
Python is not classified strictly as a functional programming language; rather, it is a multi-paradigm language that supports various programming styles, including procedural, object-oriented, and functional programming. While Python incorporates several functional programming features, such as first-class functions, higher-order functions, and list comprehensions, it does not enforce a functional programming approach. This flexibility allows developers to choose the paradigm that best suits their needs.
One of the key characteristics of functional programming is the emphasis on immutability and the avoidance of side effects. Python does allow for immutable data types, such as tuples and frozensets, but it also permits mutable data types like lists and dictionaries. This duality enables developers to utilize functional programming concepts when desired, while also leveraging Python’s procedural and object-oriented capabilities for more complex applications.
In summary, while Python embraces functional programming principles, it is not exclusively a functional language. Its design encourages a blend of programming paradigms, making it versatile and accessible to a wide range of developers. This adaptability is one of the reasons Python remains a popular choice for various applications, from web development to data analysis and artificial intelligence.
Author Profile
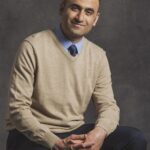
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?