Is Python Truly Functional? Exploring Its Functional Programming Capabilities
In the ever-evolving landscape of programming languages, Python stands out for its versatility and ease of use. But as developers delve deeper into its capabilities, a pressing question often arises: Is Python functional? This inquiry not only touches on the core principles of Python but also invites a broader discussion about the paradigms that shape the way we write code. As we explore the functional programming features embedded within Python, we’ll uncover how this popular language balances multiple paradigms, allowing developers to leverage its strengths in various ways.
Python is primarily known as an object-oriented language, but it also incorporates functional programming elements that can enhance code efficiency and readability. By embracing functions as first-class citizens, Python allows developers to create higher-order functions, utilize lambda expressions, and employ list comprehensions, all of which are hallmarks of functional programming. This blend of paradigms enables programmers to choose the best approach for their specific tasks, fostering creativity and flexibility in coding.
Moreover, the functional aspects of Python can lead to more concise and maintainable code, reducing the likelihood of side effects and encouraging a more declarative style of programming. As we delve deeper into the nuances of Python’s functional capabilities, we will examine how these features can be effectively employed, the benefits they bring to the table,
Understanding Functional Programming in Python
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing-state and mutable data. Python, while primarily an object-oriented language, supports functional programming features.
Key characteristics of functional programming include:
- First-Class Functions: Functions in Python can be assigned to variables, passed as arguments to other functions, and returned from functions.
- Higher-Order Functions: Python allows functions to take other functions as parameters or return them as results.
- Anonymous Functions: Python supports the creation of anonymous functions using the `lambda` keyword.
- Immutability: Although Python does not enforce immutability, it encourages the use of immutable data structures like tuples and frozensets.
Functional Programming Features in Python
Python incorporates several functional programming features that enhance its versatility. Here are some of the most notable:
- Map: This function applies a given function to all items in an iterable (like a list) and returns a map object (which can be easily converted to a list).
- Filter: This function constructs an iterator from elements of an iterable for which a function returns true.
- Reduce: From the `functools` module, `reduce` performs a rolling computation to sequentially apply a function to the items of an iterable.
Function | Description | Example |
---|---|---|
map() | Applies a function to all items in an iterable. | list(map(lambda x: x * 2, [1, 2, 3])) |
filter() | Filters items in an iterable based on a function. | list(filter(lambda x: x > 1, [0, 1, 2, 3])) |
reduce() | Reduces an iterable to a single value by applying a function cumulatively. | from functools import reduce; reduce(lambda x, y: x + y, [1, 2, 3]) |
Benefits of Functional Programming in Python
Functional programming can lead to several advantages when used in Python, including:
- Improved Code Clarity: By using pure functions, which return the same output for the same input without side effects, code becomes more predictable and easier to debug.
- Enhanced Modularity: Functions can be designed to perform specific tasks, promoting separation of concerns and making code reusable.
- Easier Testing: Pure functions are easier to test, as they do not rely on external states.
Limitations of Functional Programming in Python
Despite its benefits, there are limitations to using functional programming in Python:
- Performance Overhead: The use of higher-order functions and immutability can introduce performance overhead compared to imperative programming approaches.
- Complexity for Beginners: The functional programming paradigm may be less intuitive for those accustomed to imperative styles, leading to a steeper learning curve.
- Limited Support for Tail Recursion: Python does not optimize for tail recursion, which can lead to recursion depth issues in functional programming scenarios.
In summary, while Python is not a purely functional programming language, it provides robust support for functional programming principles, enabling developers to leverage these concepts effectively within their code.
Understanding Functional Programming in Python
Functional programming is a programming paradigm centered around the use of functions. Python, while primarily an object-oriented and imperative language, supports functional programming features. This allows developers to approach problems using a functional style when appropriate.
Key Features of Functional Programming in Python
Python incorporates several functional programming concepts, allowing developers to leverage them in their code:
- First-Class Functions: Functions in Python can be assigned to variables, passed as arguments, and returned from other functions. This flexibility is a hallmark of functional programming.
- Higher-Order Functions: Python supports higher-order functions, which can take other functions as arguments or return them. Common examples include `map()`, `filter()`, and `reduce()`.
- Lambda Functions: Python provides anonymous functions through the `lambda` keyword. These are useful for short, throwaway functions often used with higher-order functions.
- Immutability: While Python lists and dictionaries are mutable, immutable data structures like tuples and frozensets can be used to emulate functional programming principles.
- List Comprehensions: Python supports list comprehensions, which provide a concise way to create lists while applying a function to each element.
Examples of Functional Programming Constructs
To illustrate functional programming in Python, consider the following examples:
“`python
Higher-Order Function Example
def apply_function(func, value):
return func(value)
Lambda Function Example
double = lambda x: x * 2
result = apply_function(double, 5) result: 10
Using map() to apply a function to a list
numbers = [1, 2, 3, 4]
squared = list(map(lambda x: x ** 2, numbers)) squared: [1, 4, 9, 16]
Using filter() to filter a list
evens = list(filter(lambda x: x % 2 == 0, numbers)) evens: [2, 4]
“`
Limitations of Functional Programming in Python
While Python supports functional programming, it is not a purely functional language. Some limitations include:
- Performance: Functional programming can lead to performance overhead due to the creation of many temporary objects. This is particularly true with recursion and extensive use of higher-order functions.
- State Management: Python’s mutable data structures can complicate functional programming paradigms, as maintaining state is easier in an object-oriented approach.
- Readability: Overuse of lambda functions and functional constructs may lead to code that is less readable, especially for those unfamiliar with functional programming concepts.
Comparison with Other Languages
Language | Functional Features | Purely Functional? |
---|---|---|
Python | First-class functions, lambdas | No |
Haskell | Strongly typed, lazy evaluation | Yes |
JavaScript | First-class functions, async/await | No |
Scala | Functional and object-oriented | No |
Clojure | Immutable data structures | Yes |
By understanding these aspects of functional programming in Python, developers can effectively integrate functional techniques into their coding practices, enhancing code reusability and clarity where applicable.
Perspectives on Python’s Functional Programming Capabilities
Dr. Emily Carter (Professor of Computer Science, Tech University). “Python is not a purely functional programming language, but it does support functional programming paradigms. Features such as first-class functions, higher-order functions, and list comprehensions allow developers to adopt a functional style when desired.”
Michael Chen (Senior Software Engineer, Data Solutions Inc.). “While Python is primarily an object-oriented language, its functional programming capabilities, such as the use of lambda functions and the map/filter/reduce functions, enable developers to write concise and expressive code that can leverage functional techniques effectively.”
Lisa Patel (Lead Developer, Open Source Initiative). “Python’s flexibility allows it to blend functional programming with other paradigms. This versatility is one of its strengths, as it provides developers with the tools to choose the best approach for their specific problems.”
Frequently Asked Questions (FAQs)
Is Python a functional programming language?
Python is not a purely functional programming language; it is a multi-paradigm language that supports functional, procedural, and object-oriented programming styles.
What functional programming features does Python support?
Python supports several functional programming features, including first-class functions, higher-order functions, anonymous functions (lambdas), and built-in functions like `map()`, `filter()`, and `reduce()`.
Can Python functions be treated as first-class citizens?
Yes, in Python, functions are first-class citizens. This means they can be passed as arguments, returned from other functions, and assigned to variables.
Are there any limitations to functional programming in Python?
While Python supports functional programming, it may not be as efficient for heavy functional programming tasks compared to languages designed specifically for that paradigm, such as Haskell. Additionally, Python’s mutable data types can complicate functional programming practices.
How does Python handle immutability in functional programming?
Python provides immutable data types, such as tuples and frozensets, which can be used in functional programming. However, it does not enforce immutability strictly, allowing mutable types like lists and dictionaries.
Is functional programming in Python suitable for large-scale applications?
Functional programming can be suitable for large-scale applications in Python, particularly for tasks involving data processing and parallelism. However, it is often complemented with other paradigms to enhance readability and maintainability.
Python is a multi-paradigm programming language that supports various programming styles, including functional programming. While it is not a purely functional language like Haskell, Python incorporates several functional programming features that allow developers to adopt functional techniques. Key functional programming concepts such as first-class functions, higher-order functions, and the use of lambda expressions are integral to Python’s design, enabling programmers to write concise and expressive code.
One of the most notable aspects of Python’s functional capabilities is its support for higher-order functions, which can take other functions as arguments or return them as results. This feature allows for powerful abstractions and the creation of more modular code. Additionally, Python’s built-in functions like `map()`, `filter()`, and `reduce()` facilitate a functional approach to data processing, making it easier to work with collections in a declarative manner.
Despite its functional programming features, Python also emphasizes readability and simplicity, which can sometimes lead to a more imperative style of programming. Developers can choose to blend functional programming with object-oriented and procedural styles, allowing for flexibility in how they approach problem-solving. Ultimately, Python’s versatility makes it a valuable tool for programmers who wish to incorporate functional programming principles into their work.
In
Author Profile
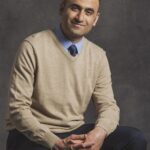
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?