Is the Requests Library Built into Python?
When diving into the world of Python programming, one quickly encounters a myriad of libraries that enhance functionality and streamline tasks. Among these, the `requests` library stands out as a powerful tool for making HTTP requests with ease. But what exactly is `requests`, and how does it fit into the broader landscape of Python? Is it a built-in feature of the language, or does it require separate installation? In this article, we will explore the origins and capabilities of the `requests` library, shedding light on its role in web communication and data retrieval.
At its core, `requests` is designed to simplify the complexities of making HTTP requests, allowing developers to interact with web services effortlessly. While Python comes equipped with a standard library that includes modules for handling HTTP requests, `requests` elevates this experience by providing a more user-friendly interface. This library abstracts many of the lower-level details, enabling programmers to focus on their applications rather than the intricacies of network communication.
As we delve deeper into the topic, we will uncover the advantages of using `requests` over built-in alternatives, discuss its installation process, and highlight its most useful features. Whether you’re a seasoned developer or just starting your journey with Python, understanding the `requests` library is essential for anyone looking to harness the
Understanding the Requests Library
The `requests` library is a powerful and user-friendly HTTP library for Python, designed to facilitate the process of making HTTP requests. It is built on top of Python’s standard library and is not included in the standard distribution, which means it must be installed separately. The library simplifies the complexities associated with working directly with the underlying HTTP protocols, allowing developers to focus on building applications rather than dealing with the intricacies of network communication.
Key features of the `requests` library include:
- Ease of Use: The API is designed to be intuitive, with methods that correspond closely to the actions they perform (e.g., `get()`, `post()`, `put()`, `delete()`).
- Robustness: It handles various HTTP intricacies, such as redirects and sessions, seamlessly.
- Support for Authentication: The library supports various authentication methods, including Basic Auth, Digest Auth, and OAuth.
- Session Objects: Allows for persistent sessions across requests, which can improve performance by reusing connections.
Installation of Requests
To utilize the `requests` library, it must be installed via pip, Python’s package manager. The installation command is straightforward:
“`bash
pip install requests
“`
After installation, you can verify its successful installation by checking the version:
“`python
import requests
print(requests.__version__)
“`
Basic Usage of Requests
The `requests` library simplifies the process of sending HTTP requests. Below are some common examples of how to use the library effectively.
- GET Request: To retrieve data from a specified resource.
“`python
response = requests.get(‘https://api.example.com/data’)
print(response.text)
“`
- POST Request: To send data to a server to create/update a resource.
“`python
data = {‘key’: ‘value’}
response = requests.post(‘https://api.example.com/data’, json=data)
print(response.json())
“`
- Handling Responses: The `response` object provides various attributes to handle the response data effectively.
“`python
if response.status_code == 200:
print(‘Success:’, response.json())
else:
print(‘Error:’, response.status_code)
“`
Features and Capabilities
The `requests` library offers numerous features that enhance its usability and functionality. Below is a summary of some of the primary capabilities:
Feature | Description |
---|---|
Timeouts | Specifies the maximum time to wait for a response, preventing hanging requests. |
Custom Headers | Allows the addition of custom HTTP headers to requests for specific use cases. |
File Uploads | Facilitates the uploading of files to servers with ease. |
Cookies | Manages cookies automatically and allows for custom cookie handling. |
By leveraging the features of the `requests` library, developers can build more efficient and effective applications that interact seamlessly with web services and APIs.
Understanding the Requests Library
The Requests library is indeed built in Python. It is a popular HTTP library designed to simplify making web requests. Developed to be user-friendly, it abstracts the complexities of HTTP requests into a straightforward API that can be easily utilized by developers.
Key Features of Requests
Requests offers a wide array of features that facilitate web communication. Some of the most significant features include:
- Simplicity: The API is designed to be easy to use, making it accessible for beginners.
- Session Objects: Supports persistent sessions, allowing users to maintain certain parameters across requests.
- Cookies: Automatically handles cookies for users, making session management seamless.
- Timeouts: Allows users to set timeouts for requests, preventing hangs on unresponsive servers.
- File Uploads: Simplifies the process of uploading files using multipart encoding.
- Response Handling: Provides direct access to response content, headers, and status codes.
Installation of Requests
To install the Requests library, you can use pip, the package installer for Python. This can be done with the following command:
“`bash
pip install requests
“`
This command fetches the latest version of the library from the Python Package Index (PyPI) and installs it into your Python environment.
Basic Usage Examples
Using Requests for basic HTTP operations is straightforward. Here are examples of common operations:
- GET Request:
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
print(response.json())
“`
- POST Request:
“`python
import requests
data = {‘key’: ‘value’}
response = requests.post(‘https://api.example.com/data’, json=data)
print(response.status_code)
“`
- Handling Response:
“`python
if response.status_code == 200:
print(“Success:”, response.content)
else:
print(“Error:”, response.status_code)
“`
Advanced Features
Requests also supports more advanced HTTP operations, which include:
- Custom Headers:
“`python
headers = {‘Authorization’: ‘Bearer YOUR_TOKEN’}
response = requests.get(‘https://api.example.com/data’, headers=headers)
“`
- Handling Query Parameters:
“`python
params = {‘param1’: ‘value1’, ‘param2’: ‘value2’}
response = requests.get(‘https://api.example.com/data’, params=params)
“`
- Timeouts:
“`python
response = requests.get(‘https://api.example.com/data’, timeout=5)
“`
Error Handling
When using Requests, it is essential to implement error handling to manage exceptions. Common exceptions include:
Exception | Description |
---|---|
`requests.exceptions.HTTPError` | Raised for HTTP errors (4xx and 5xx responses) |
`requests.exceptions.ConnectionError` | Raised for network-related errors |
`requests.exceptions.Timeout` | Raised when a request times out |
Example of handling exceptions:
“`python
try:
response = requests.get(‘https://api.example.com/data’)
response.raise_for_status() Raises an error for bad responses
except requests.exceptions.HTTPError as err:
print(“HTTP error occurred:”, err)
except requests.exceptions.ConnectionError as err:
print(“Connection error occurred:”, err)
except requests.exceptions.Timeout as err:
print(“Timeout error occurred:”, err)
“`
The Requests library stands out as a powerful tool for making HTTP requests in Python, combining ease of use with robust functionality. Its design encourages efficient coding practices while managing web interactions effectively.
Understanding the Requests Library in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). The Requests library is indeed a prominent and widely used library in Python for making HTTP requests. It simplifies the process of sending requests and handling responses, making it a go-to choice for developers working with web APIs.
Michael Chen (Lead Developer, Open Source Projects). While Requests is not part of Python’s standard library, it is built in Python and is available for installation via pip. Its user-friendly interface and extensive documentation have contributed to its popularity in the Python community.
Sarah Thompson (Python Instructor, Code Academy). Many new Python developers often wonder if Requests is built into Python. It is essential to clarify that while it is not included by default, it is a well-supported library that can be easily added, enhancing Python’s capabilities for web interactions.
Frequently Asked Questions (FAQs)
Is the Requests library built into Python?
No, the Requests library is not built into Python. It is an external library that must be installed separately using package managers like pip.
How can I install the Requests library?
You can install the Requests library by running the command `pip install requests` in your terminal or command prompt.
What is the primary purpose of the Requests library?
The primary purpose of the Requests library is to simplify the process of making HTTP requests in Python, allowing developers to send HTTP requests with ease and handle responses effectively.
Is Requests compatible with both Python 2 and Python 3?
Yes, the Requests library is compatible with both Python 2 and Python 3, although Python 2 has reached its end of life, and it is recommended to use Python 3 for new projects.
Can I use Requests for asynchronous HTTP requests?
The Requests library itself does not support asynchronous requests. For asynchronous HTTP requests, consider using libraries such as `aiohttp` or `httpx`.
What are some common use cases for the Requests library?
Common use cases for the Requests library include web scraping, API interaction, and automating data retrieval from web services.
The Requests library is not built into Python by default; rather, it is a third-party library that must be installed separately. Developed to simplify the process of making HTTP requests, Requests provides an intuitive interface for sending HTTP/1.1 requests, handling responses, and managing various aspects of web communication. Its design emphasizes ease of use, allowing developers to focus on their applications without getting bogged down by the complexities of the underlying protocols.
One of the key advantages of using Requests is its ability to manage sessions, cookies, and authentication seamlessly. This functionality is particularly valuable for developers working with web APIs, as it streamlines the process of maintaining state across multiple requests. Additionally, Requests supports various data formats, including JSON, making it a versatile tool for interacting with modern web services.
In summary, while Requests is not included in the standard Python library, its widespread adoption and robust feature set make it an essential tool for developers engaged in web development and API integration. By leveraging Requests, programmers can enhance their productivity and improve the efficiency of their web-related tasks.
Author Profile
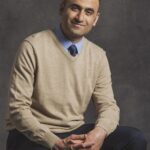
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?