Is the Print Function in Python a Command or a Function?
In the world of Python programming, clarity and precision are paramount, especially when it comes to understanding the fundamental building blocks of the language. Among these building blocks, the `print` function stands out as one of the most frequently used tools for outputting information to the console. But is it merely a command, or does it hold the status of a full-fledged function? This seemingly simple question opens the door to a deeper exploration of Python’s syntax and semantics, inviting both novice and experienced programmers to delve into the nuances of how Python operates.
At first glance, the `print` function might appear to be just another command in a sea of programming jargon. However, it embodies the principles of function-based programming that Python champions. Understanding whether `print` is a command or a function requires us to examine its role within the broader context of Python’s design philosophy. This distinction is not just academic; it influences how developers interact with the language and leverage its capabilities to produce dynamic outputs.
As we navigate through this topic, we will uncover the intricacies of the `print` function, its syntax, and its behavior in various contexts. By dissecting its characteristics, we will clarify why it is categorized as a function in Python, and how this classification impacts programming practices. Join us as
Understanding the Print Function in Python
In Python, the `print()` function is an essential built-in function used to display output to the console. Unlike commands in other programming languages that may execute a specific task without returning a value, Python’s `print()` is distinctly categorized as a function. This classification is crucial for understanding how it operates within Python’s syntax and structure.
The primary characteristics of the `print()` function include:
- Return Value: The `print()` function returns `None`, indicating that its primary purpose is not to compute a value but to produce output.
- Syntax: The basic syntax is `print(*objects, sep=’ ‘, end=’\n’, file=None, flush=)`, where:
- `*objects`: The values to be printed, which can be of any type.
- `sep`: A string inserted between the values, defaulting to a space.
- `end`: A string appended after the last value, defaulting to a newline.
- `file`: An optional file-like object where the output can be redirected.
- `flush`: A boolean that, when set to `True`, forces the output to be flushed.
Key Differences Between Commands and Functions
To better understand why `print()` is categorized as a function, it is useful to compare its properties with those of commands in other languages.
Aspect | Command | Function |
---|---|---|
Execution | Performs an action immediately | May return a value or perform an action |
Syntax | Often simpler, may not require parameters | Requires specific parameters and syntax |
Return Value | May not return any value | Typically returns a value (even if it’s `None`) |
Scope | Limited to specific contexts | Can be reused across the program |
The distinction is significant in programming paradigms, especially in Python, where functions are first-class citizens. This means functions like `print()` can be passed as arguments, returned from other functions, and assigned to variables, enhancing modular programming capabilities.
Examples of Using the Print Function
Here are some practical examples that illustrate the versatility of the `print()` function:
- Basic Output:
python
print(“Hello, World!”)
- Multiple Arguments:
python
print(“The sum of 2 and 3 is”, 2 + 3)
- Custom Separator and End Character:
python
print(“Python”, “is”, “fun”, sep=”-“, end=”!\n”)
- Redirecting Output to a File:
python
with open(“output.txt”, “w”) as file:
print(“This will be written to a file.”, file=file)
This flexibility makes the `print()` function a fundamental tool for debugging and user interaction in Python applications.
Understanding the Print Function in Python
In Python, the `print` function serves as a fundamental tool for outputting data to the console. It is classified as a built-in function rather than a command. This distinction is crucial for understanding its usage and functionality within the language.
Characteristics of the Print Function
The `print` function displays the specified message or data to the standard output device, typically the screen. Here are key characteristics:
- Syntax: The basic syntax of the `print` function is as follows:
python
print(*objects, sep=’ ‘, end=’\n’, file=sys.stdout, flush=)
- Parameters:
- `*objects`: The values to be printed. Can be multiple items separated by commas.
- `sep`: A string inserted between the objects (default is a space).
- `end`: A string appended after the last value (default is a newline).
- `file`: An object with a `write(string)` method; defaults to the console.
- `flush`: A boolean indicating whether to forcibly flush the stream.
Function vs. Command
To differentiate between a function and a command in Python:
Feature | Function | Command |
---|---|---|
Definition | A reusable block of code that performs a specific task. | An instruction to the interpreter to perform an action. |
Syntax | Requires parentheses (e.g., `print()`). | Can be a simple statement (e.g., `exit`). |
Return Value | Can return a value (e.g., `print()` returns `None`). | Generally does not return a value. |
Thus, the `print` function is a function because it encapsulates behavior and can accept parameters, allowing for versatile output formatting.
Examples of Print Function Usage
The following examples illustrate various usages of the `print` function:
- Basic Output:
python
print(“Hello, World!”)
- Multiple Arguments:
python
print(“Python”, “is”, “fun”)
- Custom Separator:
python
print(“A”, “B”, “C”, sep=”-“) # Output: A-B-C
- Changing End Parameter:
python
print(“Hello”, end=”, “)
print(“World!”) # Output: Hello, World!
Print Functionality
The `print` function is essential in Python for displaying output, functioning as a built-in feature that enhances interaction with users and aids in debugging. Its flexibility allows developers to customize output effectively, making it a powerful tool in a programmer’s toolkit.
Understanding the Nature of Python’s Print Function
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). The print function in Python is unequivocally a function. It is defined in the Python standard library and follows the principles of functional programming, allowing for arguments to be passed and returning values, which is characteristic of functions rather than commands.
Michael Chen (Lead Software Engineer, CodeCraft Solutions). In Python, the print function serves as a built-in function that outputs data to the console. Unlike commands in other programming languages that may execute a single action without returning a value, Python’s print function can be used flexibly within expressions, reinforcing its classification as a function.
Sarah Thompson (Python Educator, LearnPythonNow Academy). It is important to recognize that the print function in Python is indeed a function. This distinction is crucial for learners, as it emphasizes the importance of understanding function parameters and return values, which are foundational concepts in programming.
Frequently Asked Questions (FAQs)
Is the print function in Python a command or a function?
The print function in Python is a built-in function used to output data to the console. It is not a command in the traditional sense, as it requires parentheses and can take various arguments.
How do you use the print function in Python?
To use the print function, you simply call it with the desired arguments inside parentheses, such as `print(“Hello, World!”)`. You can also pass multiple items separated by commas.
What are some common parameters of the print function?
Common parameters of the print function include `sep`, which defines the separator between items, `end`, which specifies what to print at the end of the output, and `file`, which determines where the output is sent.
Can you override the default behavior of the print function?
Yes, you can override the default behavior of the print function by using its parameters. For example, you can change the default separator from a space to a comma by using `print(“Hello”, “World”, sep=”, “)`.
Is the print function the same in Python 2 and Python 3?
No, the print function is different in Python 2 and Python 3. In Python 2, print is a statement and does not require parentheses, while in Python 3, it is a function that requires parentheses.
Can you use the print function to output to a file?
Yes, you can use the print function to output to a file by specifying the `file` parameter. For example, `print(“Hello, World!”, file=my_file)` will write the output to the specified file object.
The print function in Python is classified as a built-in function, rather than a command. This distinction is important as it highlights the nature of how print operates within the Python programming environment. Functions in Python are defined blocks of code that perform a specific task when called, and the print function exemplifies this by taking input arguments and outputting them to the console or other specified outputs.
One of the key aspects of the print function is its versatility. It allows for various parameters, such as the ability to format strings, specify end characters, and control the output stream. This flexibility makes it an essential tool for developers when debugging or providing user feedback. Understanding the print function’s capabilities can significantly enhance a programmer’s efficiency and effectiveness in writing Python code.
In summary, recognizing the print function as a built-in function rather than a command underscores its role in Python programming. It serves as a fundamental tool for outputting information, and its various features provide developers with the ability to customize their output. Mastery of the print function is a vital skill for anyone looking to become proficient in Python.
Author Profile
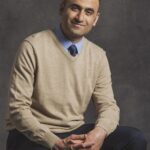
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?