Is a Tuple Mutable in Python? Unpacking the Truth!
In the world of Python programming, understanding data structures is crucial for effective coding and problem-solving. Among these structures, tuples often spark curiosity among both novice and experienced developers alike. With their unique characteristics, tuples present a fascinating contrast to other data types, particularly when it comes to mutability. This article delves into the intriguing question: Is a tuple mutable in Python? By exploring the nature of tuples, their behavior, and how they differ from other data structures, we aim to shed light on this essential aspect of Python programming.
At first glance, tuples may seem like a straightforward collection of elements, but their immutability sets them apart from lists and dictionaries. This inherent property of tuples not only influences how they are used in Python but also affects performance and memory management. Understanding whether tuples can be modified after creation is key to leveraging their full potential in your coding projects.
Throughout this article, we will explore the implications of tuple immutability, the scenarios in which they shine, and how they can be effectively utilized alongside other data structures. Whether you’re looking to optimize your code or simply enhance your understanding of Python, this examination of tuples will provide valuable insights that can elevate your programming skills.
Understanding Tuple Mutability in Python
Tuples in Python are a fundamental data structure that is often compared to lists. However, one of the most significant differences lies in their mutability.
A tuple is defined as immutable, meaning that once it is created, the elements within it cannot be modified, added, or removed. This characteristic lends itself to several important implications and advantages:
- Data Integrity: Since tuples cannot be altered, they can be used to ensure that data remains constant throughout the program.
- Performance: Tuples can be slightly more memory-efficient than lists. Their immutability allows for optimizations that can lead to better performance in certain scenarios.
- Hashability: Because tuples are immutable, they can be used as keys in dictionaries, whereas lists cannot.
Examples of Tuple Behavior
To illustrate the immutability of tuples, consider the following Python code examples:
“`python
Creating a tuple
my_tuple = (1, 2, 3)
Attempting to change an element
try:
my_tuple[0] = 10
except TypeError as e:
print(f”Error: {e}”)
“`
In the above example, trying to change the first element of `my_tuple` results in a `TypeError`, confirming that tuples cannot be modified.
Tuple vs. List: A Comparative Overview
The following table highlights the key differences between tuples and lists in Python:
Feature | Tuple | List |
---|---|---|
Mutability | Immutable | Mutable |
Syntax | (1, 2, 3) | [1, 2, 3] |
Performance | Generally faster | Slower due to mutability |
Use Cases | Fixed collections, keys in dictionaries | Dynamic collections, stack implementation |
When to Use Tuples
Considering their properties, tuples are best utilized in scenarios where:
- The data set is not intended to change.
- You want to ensure data integrity.
- You require a hashable type for keys in dictionaries.
- You are looking for a lightweight data structure for storage.
In summary, the immutability of tuples is a defining feature that not only differentiates them from lists but also provides specific advantages in terms of performance and data integrity. Understanding when and how to use tuples effectively is a key skill in Python programming.
Understanding Tuple Mutability in Python
In Python, a tuple is classified as an immutable sequence type. This characteristic means that once a tuple is created, its contents cannot be altered. The immutability of tuples has significant implications for how they are used in programming.
Key Characteristics of Tuples
- Immutable: The primary characteristic of a tuple is that it cannot be modified. This includes:
- No addition of new elements.
- No removal of existing elements.
- No modification of elements themselves.
- Fixed Size: Tuples have a fixed size after creation. The number of elements in a tuple is defined at the time of its creation.
- Heterogeneous: Tuples can contain elements of different data types, including integers, strings, lists, and other tuples.
- Indexable: Elements in a tuple can be accessed using indexing, similar to lists.
Examples of Tuple Behavior
To illustrate the immutability of tuples, consider the following examples:
“`python
Creating a tuple
my_tuple = (1, 2, 3)
Attempting to change an element (will raise an error)
try:
my_tuple[1] = 4
except TypeError as e:
print(e) Output: ‘tuple’ object does not support item assignment
Attempting to append an element (will raise an error)
try:
my_tuple.append(4)
except AttributeError as e:
print(e) Output: ‘tuple’ object has no attribute ‘append’
“`
Workarounds for Tuple Immutability
Although tuples are immutable, there are ways to work with them effectively:
- Creating New Tuples: You can create a new tuple by concatenating existing tuples or by including new elements.
“`python
new_tuple = my_tuple + (4, 5)
“`
- Converting to a List: If modification is necessary, convert the tuple to a list, make changes, and convert it back to a tuple.
“`python
temp_list = list(my_tuple)
temp_list.append(4)
my_tuple = tuple(temp_list)
“`
Performance Considerations
The immutability of tuples provides certain performance benefits:
- Memory Efficiency: Tuples use less memory than lists, making them more efficient for storing fixed collections of items.
- Hashable: Tuples can be used as keys in dictionaries due to their immutability, while lists cannot.
Feature | Tuple | List |
---|---|---|
Mutability | Immutable | Mutable |
Syntax | `()` | `[]` |
Memory Efficiency | More efficient | Less efficient |
Hashable | Yes | No |
When to Use Tuples
Tuples are particularly useful in scenarios where:
- A fixed collection of items is needed.
- Data integrity is a priority, and you want to prevent accidental modifications.
- You require a data structure that can serve as a dictionary key.
In contrast, lists are preferable when you need a dynamic collection that allows for modifications. Understanding these distinctions helps in selecting the appropriate data structure for specific programming needs.
Understanding Tuple Mutability in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). Tuples in Python are immutable, meaning once they are created, their elements cannot be modified, added, or removed. This characteristic makes tuples a reliable choice for fixed collections of items that should not change throughout the program’s execution.
Michael Chen (Lead Software Engineer, CodeCraft Solutions). The immutability of tuples is a fundamental aspect of Python’s design. This property not only enhances performance by allowing for optimizations but also ensures that data integrity is maintained when passing collections of values between functions.
Sarah Patel (Data Scientist, Analytics Hub). Understanding that tuples are immutable is crucial for Python developers. This immutability allows tuples to be used as keys in dictionaries and elements in sets, which is not possible with mutable data types like lists.
Frequently Asked Questions (FAQs)
Is a tuple mutable in Python?
No, tuples are immutable in Python. Once a tuple is created, its elements cannot be modified, added, or removed.
What is the difference between a list and a tuple in Python?
The primary difference is mutability. Lists are mutable, allowing modifications, while tuples are immutable, providing a fixed collection of elements.
Can you change the elements of a tuple?
No, you cannot change the elements of a tuple after it has been created. Any attempt to modify a tuple will result in a TypeError.
How can I create a tuple in Python?
You can create a tuple by placing comma-separated values inside parentheses, such as `my_tuple = (1, 2, 3)`.
Are tuples hashable in Python?
Yes, tuples are hashable if all their elements are hashable. This allows tuples to be used as keys in dictionaries and elements in sets.
What are some common use cases for tuples in Python?
Tuples are commonly used for returning multiple values from a function, storing fixed collections of items, and ensuring data integrity due to their immutability.
In Python, a tuple is an immutable data structure, which means that once a tuple is created, its elements cannot be modified, added, or removed. This characteristic distinguishes tuples from lists, which are mutable and allow for changes to their contents. The immutability of tuples ensures that the data they contain remains constant throughout their lifecycle, making them suitable for situations where a fixed collection of items is required.
One of the key advantages of using tuples is their efficiency in terms of performance. Since tuples are immutable, they can be stored in a more memory-efficient manner compared to lists. This can lead to faster access times and reduced overhead when dealing with large datasets. Additionally, tuples can be used as keys in dictionaries, whereas lists cannot, due to their mutable nature. This feature enhances the versatility of tuples in various programming scenarios.
In summary, understanding the immutability of tuples in Python is crucial for effective programming. While they offer several benefits, such as improved performance and usability as dictionary keys, developers should carefully consider when to use tuples versus lists based on the specific requirements of their applications. This knowledge contributes to writing more efficient and reliable Python code.
Author Profile
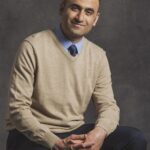
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?