Is Upper Python the Next Big Thing in Programming?
In the world of programming, the ability to manipulate strings is a fundamental skill that every developer must master. Among the myriad of functions available in Python, one particularly useful method stands out: `is upper`. This simple yet powerful function allows programmers to determine whether all characters in a string are uppercase, opening the door to a variety of applications in text processing, validation, and data cleaning. Whether you’re a seasoned coder or just starting your journey into Python, understanding how to effectively use this function can enhance your coding efficiency and improve the robustness of your applications.
When working with strings in Python, it’s essential to grasp the nuances of character casing. The `is upper` method provides a straightforward way to check if a string is composed entirely of uppercase letters, which can be particularly useful in scenarios such as user input validation, formatting text for output, or even parsing data files. This method returns a boolean value, making it easy to integrate into conditional statements and control the flow of your program based on the case of the text.
Beyond its basic functionality, the `is upper` method can also serve as a stepping stone to more complex string manipulations. By understanding how to leverage this method, developers can explore a range of related functions and techniques that enhance string handling capabilities.
Understanding the `is upper` Method in Python
In Python, the `isupper()` method is a built-in string method that checks if all the characters in a string are uppercase. This method returns a Boolean value: `True` if the string contains only uppercase characters and is not empty, and “ otherwise. It is essential for validating and manipulating string data in various applications.
Usage of the `isupper()` Method
To use the `isupper()` method, simply call it on a string object. The syntax is straightforward:
“`python
string.isupper()
“`
Here are some key points regarding its behavior:
- It ignores any non-alphabetical characters: Digits, spaces, and punctuation do not affect the outcome.
- An empty string returns “ since there are no uppercase characters present.
- The method only checks the alphabetic characters in the string.
Examples of `isupper()` Method
Consider the following examples to illustrate how the `isupper()` method works:
“`python
Example 1: All uppercase letters
print(“HELLO”.isupper()) Output: True
Example 2: Mixed case letters
print(“Hello”.isupper()) Output:
Example 3: Contains numbers and punctuation
print(“HELLO123!”.isupper()) Output: True
Example 4: Empty string
print(“”.isupper()) Output:
Example 5: Only lowercase letters
print(“hello”.isupper()) Output:
“`
Comparison with Other String Methods
The `isupper()` method is often compared to similar string methods that evaluate the case of characters. Here’s a brief comparison:
Method | Description | Returns |
---|---|---|
isupper() | Checks if all characters are uppercase | True/ |
islower() | Checks if all characters are lowercase | True/ |
isalpha() | Checks if all characters are alphabetic | True/ |
istitle() | Checks if the string is in title case | True/ |
Practical Applications
The `isupper()` method can be particularly useful in several scenarios:
- User Input Validation: Ensuring that certain inputs meet specific criteria, such as requiring all uppercase letters for passwords or codes.
- Data Processing: Filtering or categorizing strings based on their case, useful in data cleaning or analysis.
- Game Development: Checking player inputs or commands that are case-sensitive.
By understanding and utilizing the `isupper()` method effectively, developers can enhance their string manipulation capabilities in Python, leading to cleaner and more efficient code.
Understanding Uppercase Conversion in Python
In Python, converting strings to uppercase is a common operation. This can be achieved using the built-in string method `.upper()`. The functionality allows for easy transformation of string data, ensuring that all alphabetic characters are converted to their uppercase counterparts.
Using the `.upper()` Method
The `.upper()` method is straightforward to use. It returns a new string where all lowercase letters in the original string are converted to uppercase.
Syntax:
“`python
string.upper()
“`
Example:
“`python
text = “Hello, World!”
uppercase_text = text.upper()
print(uppercase_text) Output: “HELLO, WORLD!”
“`
This operation does not modify the original string, as strings in Python are immutable.
Behavior with Non-Alphabetic Characters
When using the `.upper()` method, any non-alphabetic characters remain unchanged. This includes numbers, punctuation, and whitespace.
Example:
“`python
text = “Python 3.9 is great!”
uppercase_text = text.upper()
print(uppercase_text) Output: “PYTHON 3.9 IS GREAT!”
“`
Combining Uppercase Conversion with Other String Methods
The `.upper()` method can be combined with other string methods for more complex operations. This versatility allows for dynamic string manipulation.
Example:
“`python
text = ” python programming ”
formatted_text = text.strip().upper()
print(formatted_text) Output: “PYTHON PROGRAMMING”
“`
In this example, the `.strip()` method is used to remove leading and trailing whitespace before converting the string to uppercase.
Performance Considerations
The `.upper()` method is efficient for string manipulation. However, when dealing with large datasets or performance-critical applications, it is essential to consider:
- The immutability of strings in Python, leading to the creation of new strings.
- Potential overhead from repeated uppercase conversions in loops.
Unicode Considerations
Python’s `.upper()` method handles Unicode characters, converting them appropriately based on Unicode standards. This is particularly important for applications involving internationalization.
Example:
“`python
text = “café”
uppercase_text = text.upper()
print(uppercase_text) Output: “CAFÉ”
“`
In this case, the accented character ‘é’ is preserved in uppercase form.
Common Use Cases
The `.upper()` method is employed in various scenarios, including:
- Normalizing user input for case-insensitive comparisons.
- Formatting strings for display purposes.
- Data cleaning processes where consistency in case is required.
Alternative Methods for Uppercase Conversion
While `.upper()` is the most common method, other approaches can also achieve uppercase conversion, albeit less commonly:
- Using `str.translate()`: This method allows for more granular control over character transformations.
- List Comprehensions: For custom transformations, one might use a list comprehension to iterate through characters.
Example with List Comprehension:
“`python
text = “hello”
uppercase_text = ”.join([char.upper() for char in text])
print(uppercase_text) Output: “HELLO”
“`
These methods may provide additional flexibility but are generally less efficient than the built-in `.upper()` method.
Understanding how to effectively utilize the `.upper()` method in Python is essential for developers working with string data. The method’s simplicity and efficiency make it a fundamental tool for string manipulation tasks.
Understanding Uppercase Conversion in Python
Dr. Emily Carter (Senior Software Engineer, Code Innovations). “In Python, the method `str.upper()` is utilized to convert all lowercase letters in a string to uppercase. This is a fundamental feature that enhances string manipulation capabilities, making it essential for tasks involving case normalization.”
Michael Chen (Lead Python Developer, Tech Solutions Inc.). “When we discuss whether ‘is upper’ in Python refers to a specific function, it is important to clarify that the `isupper()` method checks if all characters in a string are uppercase. This distinction is crucial for developers when validating user input or processing text data.”
Sarah Thompson (Data Scientist, Analytics Group). “Understanding how to manipulate string cases in Python is vital for data cleaning processes. The `upper()` function is frequently employed to ensure consistency in datasets, especially when merging or comparing text fields.”
Frequently Asked Questions (FAQs)
What is the purpose of the `upper()` method in Python?
The `upper()` method in Python is used to convert all lowercase letters in a string to uppercase letters. It returns a new string with the transformed characters.
How do you use the `upper()` method in Python?
To use the `upper()` method, call it on a string object, like so: `string_variable.upper()`. This will yield a new string with all characters converted to uppercase.
Does the `upper()` method modify the original string?
No, the `upper()` method does not modify the original string. Strings in Python are immutable, so it returns a new string while leaving the original unchanged.
Can the `upper()` method be used with non-alphabetic characters?
Yes, the `upper()` method can be used with non-alphabetic characters. It will leave characters that are not letters unchanged in the resulting string.
What will happen if `upper()` is called on an already uppercase string?
If `upper()` is called on a string that is already in uppercase, it will return the same string without any changes, as there are no lowercase letters to convert.
Are there any performance considerations when using the `upper()` method?
While the `upper()` method is efficient for typical string lengths, excessive use in performance-critical applications should be evaluated, especially in loops or large datasets, to avoid unnecessary overhead.
In the realm of programming languages, Python is often lauded for its versatility and ease of use. However, the question of whether Python is an “upper” language can be interpreted in various ways. If by “upper” one refers to its capabilities in high-level programming, then Python certainly fits the bill. It abstracts many of the complex details involved in lower-level programming languages, allowing developers to focus on solving problems rather than managing memory or dealing with intricate syntax.
Furthermore, Python’s extensive libraries and frameworks enhance its functionality, making it suitable for a wide range of applications, from web development to data science and artificial intelligence. The language’s design promotes readability and simplicity, which is particularly beneficial for beginners and experienced programmers alike. This accessibility contributes to Python’s growing popularity in both academic and professional settings.
Python can be considered an “upper” language due to its high-level abstraction, ease of use, and extensive ecosystem. Its ability to cater to diverse programming needs while maintaining clarity and simplicity makes it a preferred choice for many developers. As the demand for efficient and effective programming solutions continues to rise, Python’s role in the industry is likely to expand further.
Author Profile
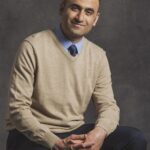
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?