How Can I Make a Java API Request and Wait for It to Finish?
In the fast-paced world of software development, efficient communication between different systems is paramount. Java, with its robust ecosystem and extensive libraries, is a popular choice for creating APIs that facilitate this interaction. However, as developers dive into crafting these APIs, they often encounter a common challenge: managing asynchronous requests. Understanding how to effectively wait for an API request to finish is crucial for ensuring that your application runs smoothly and provides a seamless user experience. In this article, we will explore the intricacies of handling API requests in Java, equipping you with the knowledge to tackle this challenge head-on.
When making API requests in Java, especially in asynchronous programming scenarios, it’s essential to grasp how to synchronize your code to wait for the completion of these requests. This involves understanding various techniques and tools available within the Java ecosystem, such as futures, callbacks, and reactive programming paradigms. Each of these approaches offers unique advantages and can significantly impact the performance and responsiveness of your application.
As we delve deeper into the topic, we will examine best practices for managing API requests, including error handling and optimizing response times. By the end of this article, you will be well-equipped with the strategies needed to ensure your Java applications can handle API requests efficiently, allowing you to focus on building robust and scalable solutions.
Understanding Java API Request Completion
When making API requests in Java, particularly asynchronous requests, it’s crucial to ensure that your application waits for the request to complete before proceeding with further processing. This can be accomplished through various mechanisms, depending on the libraries and frameworks in use.
Using Future and ExecutorService
One common approach is utilizing the `ExecutorService` along with `Future`. This allows you to submit tasks that run asynchronously and retrieve results once they are available. The `Future` interface provides methods to check the status of the task and to block until it is complete.
- Steps to use ExecutorService and Future:
- Create an instance of `ExecutorService`.
- Submit the API request as a callable task.
- Retrieve the `Future` object.
- Call `get()` on the `Future` object to wait for the completion.
Example code snippet:
“`java
ExecutorService executor = Executors.newSingleThreadExecutor();
Future
// Your API request logic here
return apiClient.makeRequest();
});
Response response = future.get(); // This line blocks until the request is finished
executor.shutdown();
“`
Using CompletableFuture
If you’re using Java 8 or later, `CompletableFuture` provides a more flexible approach to handle asynchronous programming. It allows you to write non-blocking code while still waiting for the result when needed.
- Key features of CompletableFuture:
- Allows chaining of multiple asynchronous operations.
- Provides methods to handle results and exceptions.
- Can combine multiple futures into a single future.
Example usage:
“`java
CompletableFuture
// Your API request logic here
return apiClient.makeRequest();
});
Response response = future.join(); // This blocks until the future is complete
“`
Blocking Calls with HttpURLConnection
If you prefer a more straightforward blocking call, using `HttpURLConnection` is another option. This method does not require threading or futures, as it will block until the response is received.
- Example of a blocking API request:
“`java
URL url = new URL(“http://example.com/api”);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod(“GET”);
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(connection.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
// Process the response
}
“`
Comparison Table: API Request Handling Methods
Method | Blocking | Asynchronous | Complexity |
---|---|---|---|
ExecutorService and Future | No | Yes | Medium |
CompletableFuture | No | Yes | High |
HttpURLConnection | Yes | No | Low |
By leveraging these methods, you can effectively manage API requests in Java, ensuring that your application behaves as expected in both synchronous and asynchronous environments.
Understanding API Requests in Java
In Java, making an API request typically involves using libraries such as `HttpURLConnection`, `OkHttp`, or `Apache HttpClient`. Each of these libraries provides mechanisms to execute requests and handle responses. However, the challenge often lies in ensuring that the program waits for the request to complete before proceeding.
Using HttpURLConnection
When using `HttpURLConnection`, the request is synchronous by default, meaning that the thread will block until the response is received. Here’s how to implement it:
“`java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class ApiRequest {
public static void main(String[] args) {
try {
URL url = new URL(“https://api.example.com/data”);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod(“GET”);
int responseCode = conn.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
BufferedReader in = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
} else {
System.out.println(“GET request not worked”);
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Using OkHttp
OkHttp provides a more modern approach and allows for both synchronous and asynchronous requests. To wait for a request to finish using OkHttp, you can use the `execute` method:
“`java
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class ApiRequest {
public static void main(String[] args) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(“https://api.example.com/data”)
.build();
try (Response response = client.newCall(request).execute()) {
if (response.isSuccessful()) {
System.out.println(response.body().string());
} else {
System.out.println(“Request failed: ” + response.code());
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Handling Asynchronous Requests
If you prefer asynchronous requests, you can use callbacks. Here’s how to implement it with OkHttp:
“`java
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class ApiRequest {
public static void main(String[] args) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(“https://api.example.com/data”)
.build();
client.newCall(request).enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
e.printStackTrace();
}
@Override
public void onResponse(Call call, Response response) throws IOException {
if (response.isSuccessful()) {
System.out.println(response.body().string());
} else {
System.out.println(“Request failed: ” + response.code());
}
}
});
}
}
“`
Using CompletableFuture for Asynchronous Control
Java’s `CompletableFuture` can also be utilized to handle asynchronous API requests effectively:
“`java
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
import java.util.concurrent.CompletableFuture;
public class ApiRequest {
public static CompletableFuture
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder().url(“https://api.example.com/data”).build();
return CompletableFuture.supplyAsync(() -> {
try (Response response = client.newCall(request).execute()) {
return response.isSuccessful() ? response.body().string() : “Request failed: ” + response.code();
} catch (Exception e) {
throw new RuntimeException(e);
}
});
}
public static void main(String[] args) {
fetchData().thenAccept(System.out::println);
}
}
“`
By using these methods, you can effectively manage API requests in Java and ensure your application waits for the necessary completion of those requests.
Expert Insights on Managing Java API Request Completion
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively wait for a Java API request to finish, developers should utilize the CompletableFuture class. This allows for asynchronous programming while providing a straightforward way to handle the completion of tasks without blocking the main thread.”
James Thompson (Lead Java Developer, CodeCraft Solutions). “Implementing synchronous calls using the HttpURLConnection class is a common approach. By invoking the connect() method and subsequently calling getInputStream(), developers can ensure that the request completes before proceeding with further processing.”
Linda Zhang (API Integration Specialist, Global Tech Systems). “Using libraries such as Retrofit or OkHttp can significantly simplify the handling of API requests in Java. These libraries provide built-in mechanisms for waiting for responses, allowing for cleaner and more maintainable code.”
Frequently Asked Questions (FAQs)
How can I ensure a Java API request completes before proceeding?
You can use synchronous methods provided by the API or utilize `Future` and `Callable` in Java. By calling `get()` on a `Future`, you can block the current thread until the API request is completed.
What is the difference between synchronous and asynchronous API requests in Java?
Synchronous requests block the execution of the program until the request is completed, while asynchronous requests allow the program to continue executing other tasks while waiting for the response.
How do I implement asynchronous API calls in Java?
You can use libraries like `CompletableFuture`, `ExecutorService`, or frameworks like Spring WebFlux to handle asynchronous API calls, which allow you to manage callbacks and handle responses without blocking the main thread.
What is the role of `Thread.sleep()` in waiting for an API request?
Using `Thread.sleep()` is generally not recommended for waiting on API requests as it blocks the thread unnecessarily. Instead, consider using proper synchronization mechanisms or asynchronous programming models.
Can I use callbacks to handle API responses in Java?
Yes, you can implement callbacks by defining an interface with a method to handle the response and passing an instance of that interface to the API request method, allowing you to process the response once it is available.
What libraries can help manage Java API requests and waiting for their completion?
Popular libraries include Apache HttpClient, OkHttp, and Spring RestTemplate. These libraries often provide built-in support for handling both synchronous and asynchronous requests efficiently.
In Java, managing API requests often involves asynchronous processing, which can lead to challenges in ensuring that a request completes before proceeding with subsequent operations. To effectively wait for an API request to finish, developers can utilize various techniques such as synchronous calls, callbacks, futures, or reactive programming. Each of these methods has its own advantages and use cases, allowing for flexibility in application design.
One common approach is to use synchronous requests, which block the executing thread until a response is received. This method is straightforward but may lead to performance bottlenecks if the API response time is unpredictable. Alternatively, employing futures allows for non-blocking calls where the main thread can continue executing while waiting for the response. This is particularly useful in applications that require high responsiveness.
Another effective strategy is to leverage callback mechanisms, which enable developers to define actions that should occur once the API request is completed. This promotes a more modular code structure and can enhance readability. For more advanced scenarios, reactive programming frameworks, such as RxJava, provide powerful tools for handling asynchronous data streams, allowing for more complex workflows and better resource management.
understanding how to wait for API requests to finish in Java is crucial for building efficient and responsive applications.
Author Profile
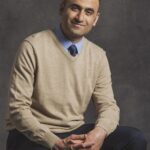
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?