Why Am I Encountering ‘Java: Compilation Failed: Internal Java Compiler Error’ and How Can I Fix It?
In the world of Java programming, encountering errors is an inevitable part of the development process. Among these, the phrase “java: compilation failed: internal java compiler error” can strike fear into the hearts of even seasoned developers. This cryptic message not only halts progress but also leaves many scratching their heads, wondering what went wrong in their code. As Java continues to evolve, understanding the nuances of its compilation process becomes crucial for troubleshooting and enhancing coding efficiency.
This article delves into the complexities surrounding internal Java compiler errors, shedding light on their causes and implications. We will explore the various scenarios that can lead to such errors, from subtle bugs in the code to deeper issues within the Java compiler itself. Additionally, we will discuss effective strategies for diagnosing and resolving these frustrating compilation failures, empowering developers to tackle them head-on.
By the end of this exploration, you will not only gain insight into the nature of internal compiler errors but also equip yourself with practical knowledge to prevent and address them in your own Java projects. Whether you are a novice programmer or an experienced developer, understanding these errors can significantly enhance your coding journey and improve your overall productivity.
Understanding Internal Java Compiler Errors
Internal Java compiler errors are serious issues that indicate a malfunction in the Java compiler itself rather than problems with the code being compiled. These errors can be challenging to diagnose and resolve, as they often stem from bugs in the Java Development Kit (JDK) or the configuration of the development environment.
Common causes for internal compiler errors include:
- JDK Bugs: Specific versions of the JDK may contain bugs that lead to compilation failures.
- Corrupted Installation: A corrupted JDK installation can cause the compiler to behave unpredictably.
- Incompatible Libraries: Using libraries that are not compatible with the version of the JDK can trigger these errors.
- Complex Code Constructs: Certain complex code structures or patterns may expose bugs in the compiler.
Troubleshooting Steps
When faced with an internal compiler error, it is essential to follow a systematic approach to troubleshoot the issue effectively:
- Check JDK Version: Ensure you are using a stable version of the JDK. Consider upgrading to the latest version or downgrading to a previously stable release.
- Reinstall JDK: If you suspect corruption, uninstall the current JDK and reinstall it. This process may resolve underlying issues caused by a faulty installation.
- Review Code Changes: Analyze recent changes in the codebase that might have triggered the error. Simplifying complex expressions or refactoring problematic sections can often help.
- Examine Compiler Flags: Some compiler flags may exacerbate or trigger internal compiler errors. Review any custom flags and consider removing them to see if the issue persists.
- Use Alternative IDEs: Sometimes, the integrated development environment (IDE) might introduce its own issues. Try compiling the code using a different IDE or directly through the command line.
- Check for Known Issues: Visit the Java bug database or relevant forums to see if others have encountered the same issue. Community discussions may provide insights or workarounds.
Common Workarounds
While internal compiler errors are often indicative of deeper issues, there are several workarounds that developers can employ to mitigate the impact:
- Split Code into Smaller Parts: Dividing large classes or methods into smaller, more manageable pieces can sometimes avoid triggering compiler bugs.
- Remove Annotations: Temporarily removing annotations from code can help identify whether they are causing the issue.
- Use Java Compiler API: If you’re developing a tool that uses the Java compiler programmatically, switching to the Java Compiler API (JSR 199) may offer more stability.
Example of Compiler Error Output
When an internal compiler error occurs, the output may look something like this:
“`
javac: compilation failed: internal java compiler error
Error: An unexpected error occurred while compiling
at com.sun.tools.javac.main.Main.compile(Main.java:633)
at com.sun.tools.javac.main.Main.compile(Main.java:452)
“`
In such cases, developers should focus on the stack trace and any accompanying messages to gather context about the error.
Comparison of JDK Versions
To understand the potential differences across JDK versions, the following table summarizes common features and stability across select versions:
JDK Version | Release Date | Stability | Notable Features |
---|---|---|---|
8 | March 2014 | High | Lambda Expressions, Stream API |
11 | September 2018 | Very High | Long-Term Support, New HTTP Client |
17 | September 2021 | High | Sealed Classes, Pattern Matching for `instanceof` |
By staying informed about the JDK versions and their respective stability, developers can make more educated decisions when faced with internal compiler errors.
Common Causes of Internal Java Compiler Errors
Internal Java compiler errors can be frustrating and may arise from various issues. Understanding these causes can help in troubleshooting effectively.
- JDK Bugs: Occasionally, the Java Development Kit (JDK) may have bugs that manifest as internal compiler errors. Keeping the JDK up to date can mitigate this risk.
- Corrupted Installation: A corrupted JDK installation can lead to unexpected behaviors, including compilation failures.
- Complex Code Constructs: Highly complex or unconventional code structures may trigger compiler errors. Refactoring such code can often resolve the issue.
- Incompatible Libraries: Using libraries that are not compatible with the current version of the JDK can lead to internal errors.
- Outdated IDE: An outdated Integrated Development Environment (IDE) can sometimes cause issues. Updating the IDE can resolve discrepancies between the IDE and the JDK.
Troubleshooting Steps
When encountering an internal Java compiler error, following a systematic troubleshooting approach can help identify and resolve the issue.
- Update the JDK:
- Ensure that you are using the latest stable version of the JDK.
- Check the release notes for known issues and fixes.
- Check for Code Issues:
- Review the code for any complex constructs or syntax errors.
- Simplify code where possible, especially around the area indicated in the error message.
- Verify IDE Configuration:
- Ensure that the IDE is configured to use the correct JDK version.
- Update the IDE to the latest version.
- Clean and Rebuild:
- Perform a clean build of the project to clear any cached files that may be causing issues.
- Examine Dependencies:
- Check for any incompatible or outdated libraries.
- Update or replace libraries as necessary.
Handling Compiler Errors in IDEs
Different IDEs have their own methods of displaying and handling internal compiler errors. Here’s how to manage these errors in some popular IDEs:
IDE | Error Handling Steps |
---|---|
IntelliJ IDEA |
|
Eclipse |
|
NetBeans |
|
Reporting Internal Compiler Errors
If internal compiler errors persist despite troubleshooting, consider reporting the issue. When doing so, include the following information:
- JDK Version: Specify the exact version of the JDK being used.
- Operating System: Mention the OS and its version.
- Code Sample: Provide a minimal, reproducible example of the code that triggers the error.
- Stack Trace: Include any stack trace or error messages received during compilation.
This information helps developers understand and resolve the issue more efficiently.
Expert Insights on Resolving Internal Java Compiler Errors
Dr. Emily Carter (Senior Software Engineer, Java Innovations Inc.). “Internal Java compiler errors often indicate issues within the Java Development Kit (JDK) or problems with the source code that are not easily identifiable. It is crucial to ensure that you are using a stable version of the JDK and to check for any syntax errors or unsupported features in your code.”
Mark Thompson (Lead Java Developer, CodeCraft Solutions). “When encountering a ‘compilation failed: internal java compiler error’, I recommend first cleaning and rebuilding the project. Sometimes, residual files can cause unexpected behavior. Additionally, examining the stack trace for any clues can lead to a quicker resolution.”
Linda Garcia (Java Compiler Specialist, TechReview Magazine). “These internal errors can be symptomatic of deeper issues, such as memory constraints or conflicts with third-party libraries. It is essential to monitor your system’s resources and review any recent changes to dependencies that might affect compilation.”
Frequently Asked Questions (FAQs)
What does “java: compilation failed: internal java compiler error” mean?
This error indicates that the Java compiler encountered an unexpected issue during the compilation process, which is not typically related to your code but rather to the compiler itself.
What are common causes of internal Java compiler errors?
Common causes include bugs in the Java Development Kit (JDK), incorrect installation of the JDK, or issues with the project configuration or dependencies.
How can I resolve an internal Java compiler error?
To resolve this error, try updating to the latest version of the JDK, ensuring that your project settings are correct, and cleaning and rebuilding your project.
Can IDE settings affect the occurrence of this error?
Yes, incorrect IDE settings, such as compiler compliance level or build paths, can contribute to internal compiler errors. Verify your IDE configurations to ensure they align with your project’s requirements.
Is it possible to get more detailed information about the error?
Yes, enabling verbose output in your IDE or command line can provide more detailed information about the compilation process, which may help identify the root cause of the error.
Should I report internal compiler errors to the Java community?
Yes, if you encounter a reproducible internal compiler error, consider reporting it to the Java community or the maintainers of the JDK, as it may indicate a bug that needs to be addressed.
The error message “java: compilation failed: internal java compiler error” indicates a serious issue occurring within the Java compiler itself during the compilation process. This type of error is not typically related to the source code written by the developer but rather suggests a malfunction or bug within the Java Development Kit (JDK) or the environment in which the code is being compiled. Such errors can arise from various factors, including outdated JDK versions, corrupted installation files, or conflicts with third-party libraries.
To address this error, developers should first ensure that they are using the latest stable version of the JDK, as updates often include bug fixes and improvements to the compiler. Additionally, it is advisable to check for any corrupted files in the JDK installation and to consider reinstalling the JDK if necessary. Developers should also review their code for any unusual constructs or configurations that might be triggering the compiler’s internal mechanisms.
In summary, encountering an internal Java compiler error can be frustrating, but it is often resolvable through systematic troubleshooting. Key takeaways include the importance of maintaining an updated development environment, the need for thorough checks of the code and configurations, and the potential benefits of seeking assistance from community forums or official documentation when faced with persistent issues.
Author Profile
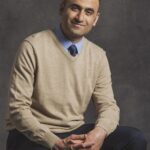
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?