Why Am I Seeing ‘Illegal Start of Expression’ Errors in My Java Compiler?
Navigating the world of Java programming can be both exhilarating and challenging, especially when you encounter cryptic error messages that halt your progress. One such message that can leave even seasoned developers scratching their heads is the dreaded “illegal start of expression.” This seemingly innocuous phrase can signal a range of issues in your code, from simple syntax errors to more complex logical missteps. Understanding the nuances behind this error is crucial for any Java programmer looking to refine their skills and enhance their coding efficiency.
When you see the “illegal start of expression” error, it typically indicates that the Java compiler has stumbled upon something unexpected in your code. This could be due to a misplaced character, an improperly formatted statement, or even a missing component that disrupts the flow of your program. As you delve deeper into the intricacies of Java syntax and structure, you’ll uncover the common pitfalls that lead to this error and how to avoid them in your future projects.
In this article, we will explore the various scenarios that can trigger the “illegal start of expression” error, providing you with practical insights and troubleshooting tips. By the end, you’ll not only be equipped to identify and resolve this error with confidence but also gain a deeper appreciation for the elegance of Java programming. So, let’s embark
Understanding the “Illegal Start of Expression” Error
The “illegal start of expression” error in Java typically occurs when the Java compiler encounters a syntactical issue that disrupts the flow of code. This can happen for various reasons, often related to misplacement of characters, incorrect syntax, or the use of reserved keywords where they are not expected.
Common causes of this error include:
- Missing semicolons at the end of statements.
- Incorrect variable declarations.
- Misuse of parentheses, brackets, or braces.
- Trying to declare variables outside of a method or class context.
- Using keywords incorrectly or inappropriately.
Examples of Common Scenarios
To better understand this error, consider the following examples:
Example 1: Missing Semicolon
“`java
int number = 5
System.out.println(number);
“`
In this instance, the absence of a semicolon after the variable declaration will trigger the error.
Example 2: Misplaced Braces
“`java
public class Test {
public static void main(String[] args) {
System.out.println(“Hello World”)
}
}
“`
Here, the missing semicolon after the `println` statement will lead to the “illegal start of expression” error.
Example 3: Incorrect Variable Declaration
“`java
int 2number = 10; // Invalid variable name
“`
Starting a variable name with a digit is not allowed in Java, causing the compiler to throw an error.
How to Fix the Error
To resolve the “illegal start of expression” error, follow these steps:
- Check Syntax: Ensure all statements are correctly terminated with a semicolon.
- Review Variable Declarations: Make sure that variable names follow Java naming conventions.
- Inspect Control Structures: Validate that all braces `{}` and parentheses `()` are correctly placed and balanced.
- Look for Unintended Characters: Sometimes, stray characters can cause confusion for the compiler.
- Refactor Code: If the error persists, consider restructuring your code for clarity.
Table of Common Causes and Solutions
Cause | Solution |
---|---|
Missing semicolon | Add a semicolon at the end of the statement. |
Incorrect variable name | Rename the variable to follow Java naming rules. |
Misplaced braces | Ensure all opening braces have a corresponding closing brace. |
Invalid use of keywords | Verify that keywords are used correctly in the context. |
By addressing these common issues and applying the outlined solutions, developers can effectively troubleshoot and rectify the “illegal start of expression” error in Java.
Understanding the “Illegal Start of Expression” Error
The “illegal start of expression” error in Java compilation typically arises when the Java compiler encounters code that does not conform to the syntactical rules of the language. This error signifies that the code structure is incorrect, which prevents the compiler from interpreting it properly. Below are common causes and examples of this error.
Common Causes of the Error
- Missing or Extra Symbols:
- A missing semicolon (`;`) at the end of a statement.
- Extra brackets or parentheses that disrupt the flow of the code.
- Incorrect Method or Variable Declarations:
- Declaring a variable or method without a proper return type or visibility modifier.
- Misplaced Keywords:
- Using keywords in an inappropriate context, such as placing `return` outside of a method.
- Invalid Characters:
- Including invalid or unsupported characters in the code.
Examples of the Error
Below are code snippets illustrating common scenarios that lead to the “illegal start of expression” error.
Code Snippet | Explanation |
---|---|
public class Test { public void method() { int a = 5 } } |
Missing semicolon after the variable declaration. |
public class Test { public void method() { return 5; } } |
Incorrect use of the `return` keyword outside of a method context. |
public class Test { int 1variable = 10; } |
Variable names cannot start with a digit. |
How to Fix the Error
To resolve the “illegal start of expression” error, consider the following steps:
- Check for Syntax Errors:
- Review the code for any missing symbols, such as semicolons or braces.
- Verify Declarations:
- Ensure all variables and methods are declared correctly with appropriate types and modifiers.
- Inspect Keywords:
- Confirm that keywords are placed correctly within the structure of the code.
- Remove Invalid Characters:
- Eliminate any unsupported characters or symbols that may have been inadvertently included.
- Use an IDE:
- Utilize an Integrated Development Environment (IDE) which can highlight syntax errors and provide real-time feedback.
Resolving the “illegal start of expression” error requires careful examination of the code for syntax and structural integrity. By following proper coding conventions and utilizing development tools, developers can quickly identify and rectify these issues, ensuring smoother compilation processes.
Understanding the ‘Illegal Start of Expression’ Error in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘illegal start of expression’ error in Java typically arises from syntax issues, such as misplaced brackets or incorrect variable declarations. It is crucial for developers to meticulously check their code structure to avoid such errors, especially in complex applications.”
Michael Chen (Java Programming Instructor, Code Academy). “This error can often confuse beginners, as it may not directly point to the root cause. I advise students to familiarize themselves with Java’s syntax rules and utilize IDE features that highlight syntax errors, which can significantly reduce the occurrence of this error.”
Sarah Thompson (Lead Developer, Open Source Projects). “In my experience, many developers encounter the ‘illegal start of expression’ error when they forget to close a string or comment properly. Code reviews and pair programming can be effective strategies to catch these common pitfalls before they escalate into bigger issues.”
Frequently Asked Questions (FAQs)
What does “illegal start of expression” mean in Java?
The “illegal start of expression” error in Java typically indicates that the compiler has encountered a syntax issue, such as a misplaced symbol, missing operators, or incorrect statement formatting that prevents it from interpreting the code correctly.
What are common causes of the “illegal start of expression” error?
Common causes include missing semicolons, incorrect use of parentheses or brackets, misplaced keywords, and using reserved keywords as variable names. Additionally, using an invalid expression format or incorrect method declarations can lead to this error.
How can I troubleshoot the “illegal start of expression” error?
To troubleshoot, carefully review the line indicated by the compiler error message, check for syntax mistakes, and ensure that all statements are properly terminated. It is also helpful to examine the surrounding lines for context that may affect the expression.
Can formatting issues lead to this error in Java?
Yes, formatting issues such as improper indentation, missing line breaks, or incorrect use of whitespace can lead to the “illegal start of expression” error. Ensuring consistent formatting can help the compiler interpret the code accurately.
Does this error occur only at the start of an expression?
No, the error may not necessarily occur at the start of an expression. It may appear anywhere in the code where the compiler detects an unexpected token or invalid syntax, leading to confusion in the expression parsing.
What steps should I take if I cannot find the source of the error?
If the source of the error is unclear, consider simplifying the code by commenting out sections to isolate the problematic area. Additionally, using an Integrated Development Environment (IDE) with syntax highlighting and error detection tools can assist in identifying the issue more easily.
The error message “illegal start of expression” in Java typically indicates a syntax issue within the code. This error occurs when the compiler encounters a statement that does not conform to the expected structure of the Java language. Common causes include misplaced punctuation, incorrect use of keywords, or improperly defined variables and methods. Understanding the context in which this error arises is crucial for effective debugging and code correction.
To resolve the “illegal start of expression” error, developers should carefully review their code for common pitfalls. This includes checking for missing semicolons, incorrect brackets, and ensuring that all statements are properly formed. Additionally, it is beneficial to consult Java documentation or utilize integrated development environments (IDEs) that provide real-time syntax checking and error highlighting to identify issues more efficiently.
In summary, addressing the “illegal start of expression” error requires a thorough understanding of Java syntax and careful attention to detail. By following best practices in coding and leveraging available tools, developers can minimize the occurrence of this error and enhance their programming proficiency. Continuous learning and practice are essential to mastering Java and avoiding common syntax errors in the future.
Author Profile
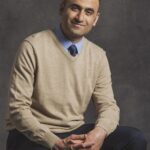
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?