Why Am I Getting a ‘Java Illegal Start of Expression’ Error?
Java, one of the most widely-used programming languages in the world, is known for its robustness and versatility. However, even seasoned developers can encounter perplexing errors that halt their progress. One such common error is the “illegal start of expression” message, which can leave programmers scratching their heads in confusion. This error often serves as a frustrating roadblock, but understanding its causes and solutions can empower developers to write cleaner, more efficient code. In this article, we will delve into the intricacies of this error, exploring its common triggers and providing practical tips for resolution.
When you see the “illegal start of expression” error in Java, it typically indicates a syntax issue in your code. Java’s strict syntax rules mean that even a small oversight, such as a misplaced semicolon or an incorrect use of parentheses, can lead to this error. As you navigate through your code, it’s essential to recognize the common patterns that lead to this message, as well as the specific scenarios where it might arise. By familiarizing yourself with these pitfalls, you can significantly reduce the likelihood of encountering this frustrating issue.
In addition to syntax errors, this message can also arise from more complex situations, such as issues with variable declarations or control structures. Understanding the context in which the error
Common Causes of the Illegal Start of Expression Error
The “illegal start of expression” error in Java typically indicates that there is a syntax issue within the code. This error can arise from various common mistakes made during coding. Understanding these causes is essential for efficient debugging and writing of Java programs.
- Missing Semicolons: Each statement in Java should end with a semicolon. Forgetting to include one can lead to this error.
- Incorrectly Placed Braces: Mismatched or misplaced curly braces `{}` can confuse the compiler, leading to syntax errors.
- Improper Variable Declarations: If a variable is declared incorrectly or outside of a method or class, it can trigger this error.
- Using Reserved Keywords: Attempting to use Java reserved keywords as variable names or identifiers will produce this error.
- Incorrect Method Definitions: Defining methods without the correct syntax (for instance, missing return types) can result in this error message.
Examples Illustrating the Error
To better understand the “illegal start of expression” error, consider the following examples:
Example 1: Missing Semicolon
“`java
int x = 10 // Missing semicolon here
int y = 20;
“`
This will result in an “illegal start of expression” error because of the missing semicolon after the first statement.
Example 2: Mismatched Braces
“`java
public class Test {
public void display() {
System.out.println(“Hello World!”
} // Missing closing brace for the method
“`
The absence of a closing parenthesis and brace will lead to a syntax error.
How to Resolve the Error
To effectively resolve the “illegal start of expression” error, follow these steps:
- Check Syntax: Review the code for any missing semicolons, parentheses, or braces.
- Validate Variable Declarations: Ensure that all variables are properly declared and initialized within the correct scope.
- Review Method Definitions: Confirm that all methods have the appropriate return types and are correctly defined.
- Use an IDE: Integrated Development Environments (IDEs) can highlight syntax errors, making it easier to identify and correct issues.
Common Mistake | Example | Correction |
---|---|---|
Missing Semicolon | int x = 10 | int x = 10; |
Mismatched Braces | if (x > 0) { System.out.println(“Positive”; } | if (x > 0) { System.out.println(“Positive”); } |
Using Reserved Keyword | int class = 5; | int myClass = 5; |
By addressing these potential issues, developers can effectively eliminate the “illegal start of expression” error from their Java programs.
Common Causes of “Illegal Start of Expression” Error
The “illegal start of expression” error in Java typically indicates that there is a syntax issue within the code. Below are common causes:
- Incorrect Use of Keywords: Using reserved keywords incorrectly can lead to this error.
- Missing or Extra Brackets: Mismatched parentheses or braces can confuse the parser.
- Improper Variable Declaration: Variables must be declared correctly before use.
- Incorrect Method Definitions: Methods must follow proper syntax, including return types.
Examples of Code Leading to the Error
The following examples illustrate situations that can generate the “illegal start of expression” error.
“`java
// Example 1: Incorrect use of a keyword
int public = 5; // ‘public’ is a reserved keyword
// Example 2: Missing brackets
if (x > 10 // Missing closing parenthesis
System.out.println(“X is greater than 10”);
// Example 3: Improper variable declaration
String name; // Proper declaration
name = “John”; // Assigning value correctly
String age = 30; // This will cause an error
// Example 4: Incorrect method definition
void myMethod() int x { // Incorrect placement of return type
System.out.println(x);
}
“`
Troubleshooting Steps
To resolve the “illegal start of expression” error, follow these troubleshooting steps:
- Review the Code Syntax:
- Check for missing semicolons at the end of statements.
- Ensure all parentheses and braces are correctly matched.
- Check Variable and Method Declarations:
- Verify that all variables are declared with proper types.
- Ensure methods are defined with the correct syntax.
- Examine Reserved Keywords:
- Avoid using Java reserved keywords as variable names or identifiers.
- Utilize an IDE:
- Use an Integrated Development Environment (IDE) that highlights syntax errors to identify issues quickly.
Best Practices to Avoid the Error
Implementing best practices can minimize the risk of encountering this error:
- Consistent Indentation: Properly indent code to improve readability and help identify mismatched brackets.
- Commenting: Use comments to temporarily disable code blocks for testing purposes without deleting them.
- Code Reviews: Have peers review your code to catch potential syntax issues.
- Testing Incrementally: Test code in smaller sections to isolate errors more easily.
Further Resources
For deeper understanding and additional troubleshooting techniques, consider the following resources:
Resource Title | Description |
---|---|
Java Documentation | Official documentation provides comprehensive details on syntax and keywords. |
Stack Overflow | Community-driven Q&A platform for specific coding issues. |
Java Coding Standards | Guides on best practices in Java programming. |
By adhering to these guidelines and utilizing provided resources, developers can effectively manage and resolve the “illegal start of expression” error in their Java applications.
Understanding Java’s Illegal Start of Expression Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘illegal start of expression’ error in Java typically arises from syntax issues, such as missing semicolons or incorrect placement of braces. Developers must ensure that their code adheres strictly to Java’s syntax rules to avoid this common pitfall.”
James Liu (Java Programming Instructor, Code Academy). “This error can often confuse beginners, as it may not always point directly to the line where the issue occurs. It’s crucial to review the preceding lines for any misplaced punctuation or keywords that could lead to such an error in Java.”
Sarah Thompson (Lead Java Developer, Future Tech Solutions). “In my experience, the ‘illegal start of expression’ error can also stem from attempting to declare variables or methods incorrectly. Ensuring that all declarations follow Java’s conventions will significantly reduce the chances of encountering this error.”
Frequently Asked Questions (FAQs)
What does “illegal start of expression” mean in Java?
The “illegal start of expression” error in Java indicates that there is a syntax issue in the code, often caused by misplaced symbols, incorrect keywords, or missing elements that disrupt the expected structure of the code.
What are common causes of the “illegal start of expression” error?
Common causes include missing semicolons, incorrect use of parentheses or braces, misplaced keywords (such as `public`, `static`, or `void`), and improper variable declarations or initializations.
How can I fix the “illegal start of expression” error?
To fix this error, carefully review the code for syntax mistakes, ensure that all statements are properly terminated, and verify that all brackets and parentheses are correctly matched and placed.
Can comments cause an “illegal start of expression” error?
Yes, improperly formatted comments can lead to this error. For example, forgetting to close a multi-line comment or placing a comment in a location where it disrupts the flow of code can trigger the error.
Is the “illegal start of expression” error specific to certain versions of Java?
No, this error is not specific to any version of Java. It is a general syntax error that can occur in any version when the code does not adhere to Java’s syntax rules.
How can I identify the location of the “illegal start of expression” error?
The Java compiler typically provides the line number where the error occurs. Check the indicated line and the surrounding lines for potential syntax issues that may have led to the error.
The “illegal start of expression” error in Java is a common compilation issue that developers encounter. This error typically arises when the Java compiler encounters code that does not conform to the expected syntax rules. Common causes include misplaced punctuation, incorrect use of keywords, or improper structuring of statements. Understanding the context in which this error occurs is crucial for effective debugging and code correction.
One key takeaway is the importance of adhering to Java’s syntax rules. Developers should ensure that all expressions are correctly formed, with appropriate use of semicolons, brackets, and parentheses. Additionally, recognizing the significance of proper variable declarations and method definitions can help prevent this error. Utilizing an Integrated Development Environment (IDE) can also facilitate the identification of syntax issues, as many IDEs provide real-time feedback and suggestions.
Another valuable insight is the role of careful code review and testing in minimizing such errors. Engaging in thorough code reviews can help catch syntax mistakes before compilation. Furthermore, writing unit tests can aid in identifying logical errors that may not directly trigger a compilation error but could lead to runtime issues. Overall, a proactive approach to coding practices can significantly reduce the occurrence of the “illegal start of expression” error in Java development.
Author Profile
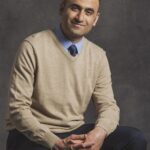
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?