Understanding Java IO: What Causes an IOException Due to a Broken Pipe?
In the world of Java programming, handling input and output operations is a fundamental skill that every developer must master. However, even seasoned programmers can encounter perplexing errors that disrupt their flow, one of which is the notorious `IOException: Broken pipe`. This error can arise unexpectedly during network communication or file operations, leaving developers scratching their heads and searching for solutions. Understanding the nuances of this error is crucial for effective debugging and ensuring robust application performance. In this article, we will delve into the intricacies of the `IOException: Broken pipe`, exploring its causes, implications, and strategies for resolution.
When working with Java’s I/O streams, developers often rely on sockets for network communication or file streams for data handling. A `Broken pipe` error typically indicates that a write operation has been attempted on a socket that has been closed on the other end, leading to a disruption in the data flow. This can happen in various scenarios, such as when a client disconnects unexpectedly or when there is a timeout during data transmission. Understanding the context in which this error occurs is essential for diagnosing the root cause and implementing effective error handling.
Moreover, the `IOException: Broken pipe` serves as a reminder of the complexities inherent in I/O operations, particularly in a multi-threaded environment
Understanding IOException
IOException is a general class of exceptions produced by failed or interrupted I/O operations. In Java, it is crucial for developers to handle these exceptions effectively to ensure robust applications. An IOException can occur during reading, writing, or closing a file, and it indicates that an input-output operation has failed or been interrupted.
The IOException class has several subclasses that provide more specific details about the kind of error that occurred. For instance:
- FileNotFoundException: Thrown when an attempt to open the file denoted by a specified pathname has failed.
- EOFException: Signals that an end of file or end of stream has been reached unexpectedly during input.
- SocketException: A subclass of IOException that indicates an error in the underlying protocol, such as a TCP error.
Broken Pipe Exception
A broken pipe exception is a specific type of IOException that occurs when one end of a pipe or socket tries to write data while the other end has been closed. This usually manifests in client-server architectures where the client attempts to send data after the server has already closed the connection.
Key Characteristics of Broken Pipe Exception:
- Nature: It is typically thrown when writing to an output stream that has been closed by the peer.
- Common Scenario: In network programming, if a server closes the connection and the client continues to send data, a broken pipe error occurs.
- Handling: It is essential to implement proper exception handling to gracefully manage such situations in applications.
Handling IOException in Java
Java provides several mechanisms for handling IOExceptions, especially when dealing with broken pipes. Here are some best practices:
- Try-Catch Blocks: Always wrap I/O operations in try-catch blocks to catch IOExceptions.
- Resource Management: Use `try-with-resources` statement to ensure that resources are closed automatically.
- Logging: Implement logging for exceptions to help diagnose issues during runtime.
Here’s an example of how to handle an IOException:
“`java
try (Socket socket = new Socket(“localhost”, 8080);
PrintWriter out = new PrintWriter(socket.getOutputStream(), true)) {
out.println(“Hello, Server!”);
} catch (IOException e) {
System.err.println(“IOException occurred: ” + e.getMessage());
}
“`
Common Causes of Broken Pipe Exceptions
Understanding the common causes of broken pipe exceptions can help in preventing them. Here are some typical scenarios:
Cause | Description |
---|---|
Server Timeout | The server closes the connection due to inactivity. |
Network Issues | Intermittent network connectivity can lead to premature closure. |
Client Error | The client tries to write after closing the connection. |
Application Logic | Flaws in application logic that fail to check the connection state. |
By being aware of these causes, developers can implement checks and balances in their applications to minimize the chances of encountering a broken pipe exception.
In summary, handling IOException, particularly broken pipe exceptions, is essential in Java programming. Proper exception management and awareness of potential pitfalls can significantly enhance the reliability of applications.
Understanding IOException and Broken Pipe Errors
IOException in Java occurs when an input or output operation fails or is interrupted. A common scenario is the “broken pipe” error, which typically arises during network communication or file handling. This error indicates that a process is attempting to write to a pipe that has no reader on the other end.
Causes of Broken Pipe Errors
- Disconnected Socket: The most frequent cause is a disconnected socket when one end of a connection has been closed while the other is still attempting to send data.
- Timeouts: If a read or write operation takes too long, the operating system may close the connection, leading to a broken pipe.
- Resource Limitations: Operating system limits on the number of simultaneous connections can lead to abrupt terminations.
- Application Bugs: Logic errors in the application can lead to attempts to write to a closed stream or socket.
Handling IOException in Java
To effectively manage IOException, particularly broken pipe scenarios, developers can implement several best practices:
- Try-Catch Blocks: Utilize try-catch blocks to handle exceptions gracefully.
“`java
try {
// Code that may throw IOException
} catch (IOException e) {
// Handle the IOException
}
“`
- Check Connection Status: Before writing data, ensure that the connection is still open.
“`java
if (socket.isConnected() && !socket.isClosed()) {
// Proceed with writing data
}
“`
- Implement Retry Logic: In cases where the broken pipe might be temporary, implementing a retry mechanism may help.
Example of Broken Pipe Handling
Here’s an example of how to handle a broken pipe in a socket communication scenario:
“`java
Socket socket = new Socket(“example.com”, 1234);
try {
OutputStream out = socket.getOutputStream();
out.write(data);
out.flush();
} catch (IOException e) {
if (e.getMessage().contains(“Broken pipe”)) {
// Handle broken pipe error specifically
System.err.println(“Attempted to write to a closed connection.”);
} else {
// Handle other IOException
e.printStackTrace();
}
} finally {
socket.close();
}
“`
Best Practices for Avoiding Broken Pipe Errors
- Graceful Disconnection: Always ensure that connections are closed properly to avoid abrupt terminations.
- Timeout Configuration: Set reasonable timeouts for socket operations to prevent long waits.
- Use Buffered Streams: Buffered streams can help reduce the number of write operations, minimizing the risk of broken pipes.
Conclusion on Best Practices
By understanding the causes of IOException related to broken pipes and employing robust exception handling techniques, developers can create more resilient Java applications. Properly managing connections and using sound coding practices will significantly reduce the occurrence of these errors.
Understanding Java IO and Handling IOException: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘broken pipe’ IOException in Java typically occurs when a process attempts to write to a socket that has been closed by the other end. This often signifies that the client has disconnected unexpectedly, and it’s crucial for developers to implement proper error handling to manage such scenarios gracefully.”
James Liu (Lead Java Developer, Cloud Solutions Group). “In my experience, encountering a broken pipe IOException can be frustrating. It is essential to monitor network connections and ensure that both client and server are synchronized in their communication. Implementing timeouts and retries can significantly reduce the frequency of these errors in production environments.”
Sarah Thompson (Technical Architect, Enterprise Systems). “When dealing with IOException related to broken pipes, it’s important to understand the underlying network architecture. Analyzing the conditions leading to disconnections, such as network latency or server overload, can provide insights into preventing these issues. Additionally, logging detailed error messages can aid in troubleshooting and improving system resilience.”
Frequently Asked Questions (FAQs)
What does a “broken pipe” error mean in Java IO?
A “broken pipe” error in Java IO typically occurs when a process attempts to write to a socket that has been closed by the other end. This results in an IOException being thrown, indicating that the connection is no longer valid.
What causes a broken pipe exception in Java?
A broken pipe exception can be caused by various factors, including network issues, abrupt termination of the receiving process, or timeouts. When the sender tries to write data to a closed connection, the system raises this exception.
How can I handle a broken pipe IOException in my Java application?
To handle a broken pipe IOException, you should implement proper exception handling using try-catch blocks. Additionally, consider checking the connection status before writing data and implementing retries or fallback mechanisms as necessary.
Is a broken pipe IOException recoverable in Java?
In many cases, a broken pipe IOException is not recoverable, as it indicates a fundamental issue with the connection. However, you can catch the exception and attempt to re-establish the connection or notify the user, depending on the application’s requirements.
What are best practices to avoid broken pipe exceptions in Java?
To avoid broken pipe exceptions, ensure that you manage socket connections properly, implement timeout settings, and regularly check the health of the connection. Additionally, gracefully handle disconnections and notify users of any issues.
Can a broken pipe exception occur in other contexts outside of Java IO?
Yes, a broken pipe exception can occur in various programming environments and languages, particularly in network programming. It generally signifies that a write operation was attempted on a closed communication channel.
In Java, an IOException related to a broken pipe typically occurs when a program attempts to write to a socket or stream that has been closed on the other end. This situation often arises in network programming, where the connection between a client and server can be disrupted due to various reasons, such as network issues, timeouts, or the remote application terminating unexpectedly. Understanding the context of this exception is crucial for developers to implement robust error handling and recovery strategies.
One of the key takeaways is the importance of proper resource management in Java applications. Developers should ensure that they are closing streams and sockets appropriately to prevent resource leaks. Additionally, implementing retry logic or fallback mechanisms can help mitigate the impact of broken pipe exceptions, allowing applications to recover gracefully from transient network issues.
Furthermore, logging detailed information when an IOException occurs can provide valuable insights into the state of the application and the network at the time of the error. This practice not only aids in debugging but also helps in understanding patterns that may lead to such exceptions. Overall, being proactive in handling IOExceptions and understanding their implications can significantly enhance the reliability and stability of Java applications.
Author Profile
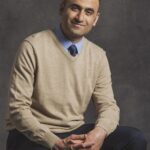
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?