How Can I Resolve the ‘java.lang.IllegalArgumentException: Unsupported Class File Major Version 65’ Error?
In the ever-evolving landscape of software development, Java remains a cornerstone language, powering everything from enterprise applications to mobile devices. However, as developers strive to leverage the latest features and improvements, they often encounter hurdles that can disrupt their workflow. One such obstacle is the notorious `java.lang.IllegalArgumentException: Unsupported class file major version 65`, a cryptic error message that can leave even seasoned programmers scratching their heads. Understanding the implications of this error is crucial for anyone working with Java, especially as the language continues to advance with new versions and capabilities.
This error typically arises when there is a mismatch between the Java version used to compile a class file and the version of the Java Runtime Environment (JRE) attempting to execute it. As Java evolves, each version introduces new class file major versions, and version 65 corresponds to Java 21. If your environment is running an older JRE, it simply won’t recognize the newer class file format, leading to this frustrating exception. The implications of this issue extend beyond mere inconvenience; they can significantly impact development timelines and application performance.
In this article, we will delve into the causes and solutions for the `Unsupported class file major version 65` error, equipping you with the knowledge to navigate this common pitfall
Understanding the Error
The error `java.lang.IllegalArgumentException: unsupported class file major version 65` indicates that your Java application is attempting to run class files that were compiled with a version of Java that is not supported by the runtime environment. The “major version 65” refers to classes that were compiled with Java 21. If you are using an older version of Java for runtime, this mismatch will lead to the error.
Java versions have specific major version numbers that correspond to the different releases. Here’s a quick reference:
Java Version | Major Version |
---|---|
Java 8 | 52 |
Java 9 | 53 |
Java 10 | 54 |
Java 11 | 55 |
Java 12 | 56 |
Java 13 | 57 |
Java 14 | 58 |
Java 15 | 59 |
Java 16 | 60 |
Java 17 | 61 |
Java 18 | 62 |
Java 19 | 63 |
Java 20 | 64 |
Java 21 | 65 |
Common Causes
Several common scenarios can lead to this error:
- Incompatible Runtime: The Java runtime being used is an older version that does not support the class files.
- Mismatched Compilation: The project may have been compiled with a newer version of Java without updating the runtime.
- Dependency Issues: Libraries or dependencies might have been compiled with a newer Java version than what your project is configured to use.
Resolving the Issue
To resolve the `Unsupported class file major version 65` error, consider the following approaches:
- Upgrade Java Runtime: Install a newer version of the Java Runtime Environment (JRE) that matches or exceeds the version used for compilation.
- Recompile the Code: If maintaining compatibility with an older JRE is necessary, recompile your code with the `-target` and `-source` options set to a lower version. For example:
“`bash
javac -source 11 -target 11 YourClass.java
“`
- Check Project Settings: Verify that the project settings in your IDE reflect the correct JDK version.
- Dependency Management: If you are using a build tool such as Maven or Gradle, ensure that all dependencies are compatible with your JDK version.
Best Practices
To prevent similar issues in the future, adhere to the following best practices:
- Consistent Environment: Maintain a consistent development and production environment with the same JDK version.
- Version Control: Use version control for your project and document the JDK version requirements clearly in your project’s README or documentation.
- Automated Build Systems: Implement CI/CD pipelines that verify compatibility during build and deployment processes.
By following these guidelines, you can minimize the risk of encountering class file compatibility issues and ensure smoother application development and deployment.
Understanding the Error
The error `java.lang.IllegalArgumentException: Unsupported class file major version 65` indicates that the Java runtime environment (JRE) is trying to load a class file compiled with a version of the Java compiler that it does not support. In this case, version 65 corresponds to Java 21. This mismatch often occurs in scenarios where older Java versions are used to run applications compiled with newer versions.
Common Causes
Several factors can lead to this error:
- Mismatched JDK/JRE Versions: The application is compiled with a newer version of Java than the version used to run it.
- IDE Configuration: Integrated Development Environments (IDEs) may be set to compile with a newer version while the runtime environment is still set to an older version.
- Build Tools: Tools like Maven or Gradle might be configured incorrectly, specifying a JDK version that is incompatible with the local JRE.
Resolving the Issue
To address the `Unsupported class file major version 65` error, consider the following steps:
- Update the JRE:
- Ensure that the runtime environment is updated to a version that supports the compiled class files. For example, install Java 21 or higher.
- Modify Project Settings:
- In your IDE, check the project settings and ensure that both the compiler and runtime versions are aligned.
- For example, in IntelliJ IDEA or Eclipse, adjust the project settings to match the JDK version used for compilation.
- Check Build Tool Configuration:
- For Maven, check the `pom.xml` file for the `maven-compiler-plugin` configuration:
“`xml
- For Gradle, ensure the following lines are set in your `build.gradle` file:
“`groovy
sourceCompatibility = ’21’
targetCompatibility = ’21’
“`
Verifying Java Versions
It is essential to verify the versions of Java installed on your system:
- Command Line: Use the following commands to check installed versions:
“`bash
java -version
javac -version
“`
- Environment Variables: Ensure that the `JAVA_HOME` environment variable points to the correct JDK installation.
Best Practices
To prevent similar issues in the future, follow these best practices:
- Consistent Environments: Maintain consistency across development, testing, and production environments regarding Java versions.
- Documentation: Document the required Java version for each project to ensure team members are aware of necessary configurations.
- Automated Build Processes: Use continuous integration (CI) tools to automate builds and verify compatibility with specified Java versions.
References for Further Reading
Resource | Description |
---|---|
Oracle’s Java Documentation | Official documentation for Java versions. |
Maven Documentation | Details on configuring Maven builds. |
Gradle User Manual | Comprehensive guide for Gradle configurations. |
IntelliJ IDEA Documentation | Instructions for managing project SDKs. |
Understanding java.lang.IllegalArgumentException: Unsupported Class File Major Version 65
Dr. Emily Chen (Senior Java Developer, Tech Innovations Inc.). “The error ‘java.lang.IllegalArgumentException: unsupported class file major version 65’ typically indicates that the Java Runtime Environment (JRE) being used is incompatible with the version of Java used to compile the class files. Specifically, major version 65 corresponds to Java 21, which may not be supported by older JRE installations.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “When encountering this exception, developers should first verify the version of the JDK used for compilation. If the project is built with a newer JDK, ensure that the runtime environment matches or is updated accordingly to avoid compatibility issues.”
Sarah Patel (Java Architect, FutureTech Labs). “To resolve the ‘unsupported class file major version 65’ error, it is crucial to check the build configurations and dependencies. Utilizing tools like Maven or Gradle can help manage versions effectively and prevent such discrepancies in the class file versions.”
Frequently Asked Questions (FAQs)
What does the error “java.lang.illegalargumentexception: unsupported class file major version 65” mean?
This error indicates that the Java Virtual Machine (JVM) is attempting to load a class file compiled with a version of Java that it does not support. Major version 65 corresponds to Java 21, which may not be compatible with older JVM versions.
How can I resolve the “unsupported class file major version 65” error?
To resolve this error, ensure that you are using a compatible version of the JVM that supports the class file version. Upgrade your JVM to at least Java 21 or recompile your code with an earlier version of Java that matches your current JVM.
What is the significance of class file major versions in Java?
Class file major versions indicate the version of the Java compiler used to generate the class file. Each major version corresponds to a specific version of the Java Development Kit (JDK). Compatibility between the compiled class files and the JVM is crucial for successful execution.
Can I run Java 21 class files on Java 11 or earlier versions?
No, Java 21 class files cannot be run on Java 11 or earlier versions. The JVM must match or exceed the major version of the class files to execute them correctly.
How can I check the major version of a class file?
You can check the major version of a class file by using the `javap` command-line tool included in the JDK. Running `javap -v YourClass.class` will display the version along with other details about the class file.
Is it possible to downgrade my project to avoid this error?
Yes, you can downgrade your project by changing the source and target compatibility in your build configuration (e.g., Maven, Gradle) to a version compatible with your current JVM. This will allow you to compile the code to a lower class file version.
The error message “java.lang.IllegalArgumentException: unsupported class file major version 65” typically indicates that the Java Runtime Environment (JRE) being used does not support the version of the class files being executed. In this context, major version 65 corresponds to Java 21, which suggests that the application was compiled with a newer version of Java than the JRE can handle. This discrepancy often arises when developers compile their code with the latest Java features but attempt to run it on an outdated runtime environment.
To resolve this issue, users should ensure that they are running a compatible version of the JRE that matches or exceeds the version used for compilation. Upgrading the JRE to a version that supports major version 65, such as Java 21, is essential for executing the compiled classes successfully. Additionally, developers should consider using build tools that specify the target Java version to avoid compatibility issues when deploying applications across different environments.
It is also important to be aware of the Java ecosystem’s rapid evolution, as new versions are frequently released with enhancements and new features. Regularly updating both the development and runtime environments can help mitigate such compatibility issues. Furthermore, maintaining clear documentation of the Java version requirements for any application can facilitate smoother deployment and
Author Profile
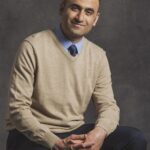
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?