Why Am I Encountering java.lang.NoClassDefFoundError: Could Not Initialize Class?
In the world of Java programming, encountering errors is an inevitable part of the development journey. Among these, the `java.lang.NoClassDefFoundError: Could not initialize class` stands out as a particularly perplexing and frustrating issue. This error not only signifies that the Java Virtual Machine (JVM) failed to load a class, but it also hints at deeper underlying problems that can disrupt the flow of your application. Whether you’re a seasoned developer or a newcomer to Java, understanding this error is crucial for maintaining robust and efficient code.
This article delves into the intricacies of the `NoClassDefFoundError`, shedding light on its causes and implications. At its core, this error often arises during runtime, signaling that while the class was present during compilation, it couldn’t be loaded when needed. This can stem from various factors, including classpath issues, static initialization failures, or even problems with the Java environment itself. By exploring these potential pitfalls, developers can better prepare themselves to troubleshoot and resolve this error effectively.
As we navigate through the common scenarios that trigger this error, we will also highlight best practices for preventing it in future projects. Understanding the nuances of class loading and initialization in Java can empower developers to create more resilient applications. Join us as we uncover
Understanding NoClassDefFoundError
The `NoClassDefFoundError` is an error that occurs in Java when the Java Virtual Machine (JVM) or a ClassLoader tries to load a class, but the definition of that class is not found. This error may arise due to various reasons, primarily during runtime, despite the class being present during compile time. It is crucial to differentiate it from `ClassNotFoundException`, which occurs when the class is not found at all.
Common reasons for `NoClassDefFoundError` include:
- The class file was deleted or moved after compilation.
- The class path is incorrectly set, leading to the JVM being unable to locate the class.
- Static initializers or static blocks within the class fail to execute properly, causing the class not to initialize.
Causes of NoClassDefFoundError
Several factors can contribute to the occurrence of this error. Below are some common causes:
- Static Initialization Failure: If a static block in the class throws an exception, the class will not be initialized, resulting in `NoClassDefFoundError`.
- Classpath Issues: Incorrectly configured classpath settings can lead to the JVM being unable to find the necessary class files.
- Jar File Corruption: If a jar file is corrupted, the JVM may not be able to load the classes contained within it.
- Version Mismatch: If the application expects a certain version of a library but finds an incompatible version, it may not load the class as expected.
Diagnosing the Error
To effectively diagnose `NoClassDefFoundError`, consider the following steps:
- Check the Stack Trace: Examine the stack trace provided by the JVM. It often indicates which class could not be initialized.
- Review Classpath Configuration: Ensure that the classpath is correctly set and that all required libraries are included.
- Inspect Static Initializers: Look for exceptions in static blocks or static variable initializations that may prevent class loading.
- Verify Class Files: Ensure that the required class files are present in the expected locations.
Example Scenario
Consider a situation where you have a class `MyClass` that contains a static initializer that throws an exception:
“`java
public class MyClass {
static {
if (true) {
throw new RuntimeException(“Initialization failed”);
}
}
}
“`
Attempting to use `MyClass` would result in:
“`
java.lang.NoClassDefFoundError: Could not initialize class MyClass
“`
This error indicates that while `MyClass` was found, its static initializer failed to execute, preventing the class from being properly initialized.
Handling NoClassDefFoundError
To handle `NoClassDefFoundError`, you can adopt the following strategies:
- Exception Handling: Implement try-catch blocks to handle exceptions in static initializers gracefully.
- Check Dependencies: Ensure that all dependencies are properly included in your project and that no version mismatches occur.
- Use Logging: Introduce logging within static blocks to gain insights into what might be causing initialization failures.
Cause | Solution |
---|---|
Static Initialization Failure | Review and fix any exceptions in static blocks |
Classpath Issues | Verify and correct classpath settings |
Jar File Corruption | Rebuild or replace the corrupted jar file |
Version Mismatch | Ensure compatible versions of libraries are used |
Understanding NoClassDefFoundError
NoClassDefFoundError is a runtime error in Java that indicates a class could not be initialized. This can occur due to various reasons, including issues related to classpath, dependency problems, or static initialization failures.
Common Causes
Several factors can contribute to the occurrence of NoClassDefFoundError:
- Classpath Issues: The class file might not be present in the expected location. This often happens when the class is not included in the build or runtime classpath.
- Static Initialization Failures: If a static block in the class throws an exception, it can prevent the class from being initialized properly.
- Version Mismatch: Incompatibilities between different versions of libraries can lead to this error if a class is removed or altered.
- Circular Dependencies: Classes that depend on each other for initialization can cause this error if one of the classes fails to initialize.
Troubleshooting Steps
To resolve NoClassDefFoundError, consider the following troubleshooting steps:
- Check Classpath:
- Ensure that the classpath includes all necessary JAR files and directories.
- Use the `java -cp` option to explicitly specify the classpath.
- Inspect Static Initializers:
- Review the static blocks in the class for any code that may throw exceptions.
- Log errors or exceptions that occur during initialization.
- Verify Library Versions:
- Confirm that all libraries are compatible and that the required classes are available in the expected versions.
- Check for dependency conflicts in your build tool (e.g., Maven, Gradle).
- Analyze Logs:
- Look for stack traces or error messages preceding the NoClassDefFoundError for clues about the root cause.
Example Scenario
Consider a scenario where a Java application relies on a library containing a class `DatabaseConnector`. If the class file for `DatabaseConnector` is missing from the classpath, the following error might occur:
“`
Exception in thread “main” java.lang.NoClassDefFoundError: Could not initialize class DatabaseConnector
“`
In this case, the solution would involve:
- Ensuring the JAR file containing `DatabaseConnector` is included in the project.
- Checking for any exceptions thrown during the static initialization of `DatabaseConnector`.
Best Practices
To prevent NoClassDefFoundError in future projects, adhere to these best practices:
- Consistent Environment Configuration: Use build tools to manage dependencies consistently across development and production environments.
- Comprehensive Logging: Implement logging within static initializers to catch and diagnose initialization issues early.
- Regular Dependency Updates: Keep your libraries and dependencies up-to-date to avoid compatibility issues.
- Automated Testing: Employ unit tests to validate class loading and initialization under various conditions.
Understanding and troubleshooting NoClassDefFoundError is essential for maintaining robust Java applications. By following best practices and thorough debugging, developers can significantly reduce the occurrence of this error.
Understanding java.lang.NoClassDefFoundError: Insights from Software Development Experts
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “The `java.lang.NoClassDefFoundError` often indicates that the Java Virtual Machine (JVM) was unable to find a class definition at runtime, which can be due to a variety of reasons such as classpath issues or problems during the class initialization phase. It’s crucial to ensure that all necessary libraries are included in the classpath and that there are no static initializers that might fail.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “When encountering `NoClassDefFoundError`, developers should investigate the environment where the application is running. This error can arise if the class was present during compile time but is missing at runtime, often due to discrepancies in the deployment environment or changes in the build process. A thorough review of the build logs and deployment configurations is essential.”
Lisa Patel (Java Architect, Enterprise Systems Corp.). “In many cases, `java.lang.NoClassDefFoundError` can be avoided by adhering to best practices in dependency management. Utilizing tools like Maven or Gradle can help manage class dependencies effectively and ensure that all required classes are available at runtime. Additionally, proper version control of libraries can prevent such issues from arising.”
Frequently Asked Questions (FAQs)
What does the error `java.lang.NoClassDefFoundError: Could not initialize class` indicate?
This error signifies that the Java Virtual Machine (JVM) was unable to initialize a class due to various reasons, such as static initialization failure, missing dependencies, or issues with the classpath.
What are common causes of `NoClassDefFoundError`?
Common causes include missing class files in the classpath, issues with static blocks or initializers, circular dependencies between classes, or incompatible versions of libraries.
How can I troubleshoot `NoClassDefFoundError`?
To troubleshoot, check the classpath for missing classes, review static initializers for exceptions, ensure all dependencies are correctly included, and verify that the correct versions of libraries are being used.
Can `NoClassDefFoundError` occur in a production environment?
Yes, it can occur in a production environment, particularly if there are discrepancies between the development and production classpaths, or if there are runtime changes that affect class loading.
Is `NoClassDefFoundError` the same as `ClassNotFoundException`?
No, they are different. `ClassNotFoundException` occurs when the class is not found at runtime, while `NoClassDefFoundError` indicates that the class was present during compile time but could not be initialized at runtime.
What steps can I take to prevent `NoClassDefFoundError`?
To prevent this error, ensure that all required classes and dependencies are included in the classpath, avoid complex static initializers, and conduct thorough testing to catch initialization issues early in the development process.
The error message `java.lang.NoClassDefFoundError: Could not initialize class` typically indicates that the Java Virtual Machine (JVM) encountered a problem when trying to initialize a class. This issue arises after the class has been found but fails to initialize due to various reasons, such as static initialization failures, missing dependencies, or classpath issues. Understanding the root cause of this error is essential for effective debugging and resolution.
One common cause of this error is a failure in the static initialization block or static variables of a class. If an exception is thrown during this process, the class will not be initialized, leading to the `NoClassDefFoundError`. Additionally, if the class relies on other classes that are not available or improperly configured in the classpath, this can also trigger the error. Developers should thoroughly check the stack trace and ensure that all dependencies are correctly included and accessible.
Another important aspect to consider is the environment in which the Java application is running. Differences between development and production environments, such as mismatched library versions or configuration settings, can lead to this error. It is crucial to maintain consistency across environments to minimize the risk of encountering such issues. Furthermore, proper exception handling during the initialization phase can provide more insight
Author Profile
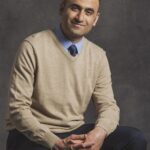
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?