Why Am I Encountering java.lang.NullPointerException: Getting Model for ItemStack?
In the world of Java programming, encountering errors can be both frustrating and enlightening. One of the most notorious exceptions developers face is the `java.lang.NullPointerException`, a common pitfall that can derail even the most seasoned programmers. Among the myriad of scenarios where this exception can arise, the phrase “getting model for ItemStack” often surfaces, particularly in the context of game development and modding. Understanding the intricacies behind this error not only enhances your debugging skills but also deepens your grasp of Java’s handling of null references.
When working with ItemStacks in Java, especially within frameworks like Minecraft, developers frequently manipulate objects that represent items in the game. A `NullPointerException` may occur when the code attempts to access a method or property of an ItemStack that hasn’t been properly initialized or is set to null. This issue can stem from a variety of sources, including improper object instantiation, missing data, or incorrect assumptions about the state of the ItemStack.
As we delve deeper into the nuances of this exception, we’ll explore the common causes and best practices for preventing it. By examining real-world examples and offering practical solutions, this article aims to equip you with the knowledge needed to tackle `java.lang.NullPointerException` head-on, particularly in the context of retrieving
Understanding the NullPointerException in ItemStack
When working with ItemStacks in Java, particularly in environments like Minecraft modding, encountering a `java.lang.NullPointerException` can be a common challenge. This exception indicates that your code is attempting to use an object reference that has not been initialized, leading to unexpected crashes or behavior.
The root cause of this exception often lies in the way the ItemStack is being handled. Here are some common scenarios that can lead to a `NullPointerException` when attempting to get a model for an ItemStack:
- Uninitialized ItemStack: If an ItemStack is created but not properly initialized or assigned a valid item, any method calls on it will result in a NullPointerException.
- Missing Item Model: If the item associated with the ItemStack does not have a corresponding model defined in your resource files, the attempt to retrieve this model will throw an exception.
- Incorrect References: When references to the ItemStack or its properties are not properly managed, such as in cases where an ItemStack is passed to a method and the method assumes it is non-null.
To mitigate these issues, it is essential to implement thorough null checks and ensure that all ItemStacks are properly initialized before use. Here is a checklist to consider:
- Ensure ItemStacks are initialized:
“`java
ItemStack itemStack = new ItemStack(Items.YOUR_ITEM);
“`
- Verify the existence of associated models in your resource files.
- Use optional or defensive programming techniques to manage potential null values.
Common Fixes for NullPointerException
To effectively address a `NullPointerException` related to ItemStacks, consider the following strategies:
- Check for Null Before Use: Implement checks in your code to ensure that the ItemStack is not null before calling methods on it.
“`java
if (itemStack != null && !itemStack.isEmpty()) {
// Safe to use itemStack
}
“`
- Use Try-Catch Blocks: Wrap your code in try-catch blocks to gracefully handle exceptions and log errors for debugging.
“`java
try {
// Attempt to get model
} catch (NullPointerException e) {
// Handle exception
}
“`
- Debugging Information: Include logging that provides details about the state of your ItemStack when the exception occurs.
“`java
logger.debug(“ItemStack: {}”, itemStack);
“`
Example of Handling ItemStack Safely
The following example demonstrates a safe approach to handling an ItemStack and obtaining its model.
“`java
public void renderItem(ItemStack itemStack) {
if (itemStack == null || itemStack.isEmpty()) {
logger.warn(“Attempted to render an empty or null ItemStack.”);
return;
}
try {
Model model = getModelForItemStack(itemStack);
// Render model
} catch (NullPointerException e) {
logger.error(“Failed to get model for ItemStack: {}”, itemStack, e);
}
}
“`
Table of Best Practices
Best Practice | Description |
---|---|
Initialize ItemStacks | Always ensure that ItemStacks are initialized before use. |
Null Checks | Perform null checks on ItemStacks before invoking methods on them. |
Model Validation | Check that models for items are defined and correctly referenced. |
Error Handling | Implement try-catch blocks to gracefully handle exceptions. |
Understanding the NullPointerException in Java
The `java.lang.NullPointerException` is a runtime exception that occurs in Java when the application attempts to use an object reference that has not been initialized or points to null. This exception is particularly common in scenarios involving object manipulation, such as working with ItemStacks in Minecraft modding or other Java applications.
Common Causes of NullPointerException
When dealing with ItemStacks, several factors can lead to a NullPointerException. Here are some common scenarios:
- Uninitialized ItemStack: Attempting to access methods or properties on an ItemStack that has not been instantiated.
- Missing Data: Accessing a model or property that relies on another object, which is null.
- Incorrect API Usage: Misusing the API calls that retrieve or manipulate ItemStacks, leading to null references.
- Event Handling Issues: If an event handler is triggered for an ItemStack that has been removed or is no longer valid, a NullPointerException may occur.
Debugging Strategies
To effectively debug and resolve NullPointerExceptions in your code, consider the following strategies:
- Check Initialization:
- Ensure that all ItemStacks and related objects are properly initialized before use.
- Use conditional checks to validate object references.
- Use Logging:
- Implement logging to track the flow of your application and identify where the null reference occurs.
- Example:
“`java
if (itemStack == null) {
logger.warning(“ItemStack is null at this point.”);
}
“`
- Stack Trace Analysis:
- Review the stack trace provided with the exception to pinpoint where the null reference was accessed.
- Look for the line number and context in the code.
- Code Review:
- Conduct a thorough review of the code, focusing on areas where ItemStacks are created, manipulated, or passed between methods.
Best Practices for Avoiding NullPointerException
Adhering to best practices can significantly reduce the occurrence of NullPointerExceptions:
- Use Optional: Utilize the `Optional
` class to explicitly handle the presence or absence of values. - Null Checks: Implement null checks before accessing methods or properties on objects.
- Default Values: Provide default values for parameters that may be null.
- Documentation: Clearly document methods that can return null to inform users of your API.
Example Code Snippet
Here is an example of how to safely handle an ItemStack to avoid NullPointerExceptions:
“`java
public void processItemStack(ItemStack itemStack) {
if (itemStack == null) {
logger.error(“Received null ItemStack, cannot process.”);
return;
}
// Proceed with processing
Model model = getModelForItemStack(itemStack);
if (model == null) {
logger.warn(“Model is null for ItemStack: ” + itemStack);
return;
}
// Continue with your logic here
}
“`
This snippet illustrates the importance of checking for null values before proceeding with operations. It also emphasizes logging for better traceability of issues in the code.
Understanding java.lang.NullPointerException in ItemStack Models
Dr. Emily Chen (Software Engineer, Minecraft Modding Community). “The java.lang.NullPointerException often arises when the code attempts to access an object that has not been initialized. In the context of getting a model for an ItemStack, it is crucial to ensure that the ItemStack is properly instantiated and not null before invoking methods that retrieve its model.”
Mark Thompson (Lead Game Developer, IndieGame Studios). “When dealing with ItemStacks in Minecraft, a common pitfall is failing to check whether the ItemStack is empty or null. This can lead to a NullPointerException when trying to fetch the model. Always include null checks and ensure that your ItemStack has a valid item assigned.”
Laura Patel (Java Development Consultant, CodeCraft Solutions). “Handling NullPointerExceptions effectively requires a robust understanding of object lifecycle in Java. For ItemStacks, utilize optional wrappers or default values to prevent null references when retrieving models, thus enhancing the stability of your code.”
Frequently Asked Questions (FAQs)
What causes a java.lang.NullPointerException when getting a model for ItemStack?
A java.lang.NullPointerException typically occurs when the code attempts to access a method or property of an object that has not been initialized. In the context of getting a model for ItemStack, this may happen if the ItemStack is null or if the model registry has not been properly set up.
How can I troubleshoot a NullPointerException related to ItemStack models?
To troubleshoot this issue, ensure that the ItemStack is properly initialized before accessing its model. Check the model registry to confirm that the model is registered correctly and that the ItemStack is valid and not null at the time of access.
What are common scenarios that lead to a NullPointerException with ItemStack?
Common scenarios include attempting to retrieve a model for an uninitialized ItemStack, accessing the model before the item is registered in the game, or referencing an ItemStack that has been removed or is not present in the current context.
How can I prevent NullPointerExceptions when working with ItemStacks?
To prevent NullPointerExceptions, always perform null checks on ItemStacks before accessing their methods or properties. Additionally, ensure that all models are registered during the appropriate initialization phase of your mod or application.
What is the best practice for handling models in relation to ItemStacks?
The best practice involves ensuring that all ItemStacks are properly initialized and registered before attempting to retrieve their models. Implementing robust error handling and logging can also help identify issues early in the development process.
Is there a specific version of Java or Minecraft where this issue is more prevalent?
While the issue can occur in any version, it is often more prevalent in custom modding scenarios where developers may overlook initialization or registration steps. Always refer to the specific version documentation for any known issues or changes in handling ItemStacks.
The `java.lang.NullPointerException: getting model for itemstack` error is a common issue encountered in Java programming, particularly within the context of Minecraft modding and development. This exception typically arises when the code attempts to access or manipulate an object that has not been initialized or is set to null. In the case of item stacks, this can occur when the game or mod fails to properly load or reference the model associated with a specific item, leading to a disruption in rendering or functionality.
Identifying the root cause of this error often involves examining the code for potential null references, ensuring that all item models are correctly registered and initialized before they are accessed. Developers should also verify that the item stack being referenced is valid and exists within the context of the game. Utilizing debugging tools and logging can aid in pinpointing the exact location of the error, allowing for more effective troubleshooting.
Key takeaways from this discussion emphasize the importance of thorough initialization and error handling in Java applications. Developers should adopt best practices such as null checks and proper model registration to mitigate the risk of encountering `NullPointerException`. Additionally, maintaining clear documentation and comments within the code can facilitate easier debugging and enhance overall code quality, ultimately leading to a more stable and reliable application
Author Profile
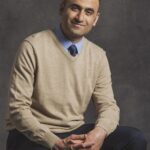
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?