How Can I Resolve the Java Lang OutOfMemoryError: GC Overhead Limit Exceeded?
In the world of Java programming, encountering errors is an inevitable part of the development process. Among these, the `java.lang.OutOfMemoryError: GC overhead limit exceeded` stands out as a particularly frustrating challenge. This error serves as a warning sign that your application is struggling to manage memory effectively, leading to performance bottlenecks and potential system crashes. As developers, understanding the root causes and implications of this error is crucial for maintaining robust and efficient applications. In this article, we will delve into the intricacies of this error, exploring its triggers, the underlying mechanics of garbage collection, and effective strategies for resolution.
The `OutOfMemoryError` is not just a simple notification; it reflects deeper issues within the Java Virtual Machine (JVM) memory management system. When the garbage collector is overburdened, it may spend excessive time trying to reclaim memory, resulting in diminished application performance. This error often indicates that your application is either consuming too much memory or that the memory allocation settings are not optimized for the workload. Understanding how the JVM handles memory allocation and garbage collection is key to diagnosing and fixing these issues.
As we navigate through the complexities of the `GC overhead limit exceeded` error, we will uncover the common scenarios that lead to its
Understanding OutOfMemoryError
OutOfMemoryError is a critical runtime exception in Java, indicating that the Java Virtual Machine (JVM) has run out of memory and cannot allocate an object because it is out of memory and has no more memory to reclaim. This error can manifest in different forms, with “GC overhead limit exceeded” being one of the most common.
When the JVM encounters this error, it typically means that the garbage collector is spending too much time trying to reclaim memory but is only able to free a small amount. This situation can severely degrade application performance and, if not addressed, can lead to application crashes.
Causes of GC Overhead Limit Exceeded
Several factors can contribute to the occurrence of the “GC overhead limit exceeded” error:
- Memory Leaks: Unused objects that are still referenced in the application prevent the garbage collector from reclaiming memory.
- Insufficient Heap Size: The allocated heap space for the application may not be enough for its demands.
- Inefficient Code: Poorly optimized code can create excessive temporary objects, leading to increased garbage collection activity.
- Large Data Structures: Using large collections or data structures can exhaust available memory quickly.
Identifying the Problem
To diagnose the cause of this error, the following strategies can be employed:
- Heap Dumps: Capture heap dumps during runtime to analyze memory usage.
- Profiling Tools: Utilize Java profiling tools (such as VisualVM, JProfiler, or YourKit) to monitor memory allocation and identify memory leaks.
- Garbage Collection Logs: Enable detailed GC logging to understand how much time is spent in garbage collection and the amount of memory reclaimed.
Tool | Purpose |
---|---|
VisualVM | Monitor application performance and memory usage in real-time. |
JProfiler | Profile memory usage and identify memory leaks. |
YourKit | Analyze memory and CPU usage, providing detailed insights. |
Solutions to Resolve GC Overhead Limit Exceeded
To mitigate the “GC overhead limit exceeded” error, several approaches can be implemented:
- Increase Heap Size: Adjust the JVM options to allocate more memory to the heap. This can be done using flags like `-Xmx` to set the maximum heap size.
- Optimize Code: Refactor code to reduce the number of temporary objects created, and ensure that collections are properly sized.
- Implement Cache Mechanisms: Use caching strategies to avoid repeated object creation.
- Garbage Collection Tuning: Modify garbage collection settings to optimize performance based on the application’s needs.
By carefully analyzing the application and applying these solutions, developers can effectively manage memory usage and minimize the risk of encountering the “GC overhead limit exceeded” error.
Understanding the OutOfMemoryError
The `java.lang.OutOfMemoryError: GC overhead limit exceeded` error typically occurs when the Java Virtual Machine (JVM) spends too much time performing garbage collection (GC) but is unable to reclaim sufficient memory. This situation often indicates that the application is running low on memory resources, leading to performance degradation and potential application failures.
Symptoms of the Error
- Frequent occurrences of garbage collection events
- Increased application latency
- Application crashes or hangs
- Unresponsive user interface
Causes of the Error
Several factors can contribute to this error, including:
- Memory Leaks: Objects that are no longer needed but are still referenced, preventing them from being garbage collected.
- Insufficient Heap Size: The allocated heap space may be too small for the application’s requirements.
- High Object Creation Rate: Continuous creation of objects without proper disposal leads to rapid memory consumption.
- Improper GC Configuration: Inappropriate garbage collection settings can exacerbate memory issues.
Diagnosing the Issue
To effectively address this error, it is crucial to diagnose the underlying causes. Several tools and techniques can aid in this process:
- Heap Dumps: Analyze heap dumps to identify memory consumption patterns and locate memory leaks.
- Profiling Tools: Utilize Java profiling tools such as VisualVM, YourKit, or Eclipse Memory Analyzer to monitor memory usage in real-time.
- JVM Flags: Enable verbose GC logging with flags like `-verbose:gc` and `-XX:+PrintGCDetails` to gain insights into garbage collection behavior.
Tool | Purpose |
---|---|
VisualVM | Monitor and analyze JVM performance |
YourKit | Detailed memory profiling |
Eclipse Memory Analyzer | Analyze heap dumps for memory leaks |
Resolving the OutOfMemoryError
Addressing the `GC overhead limit exceeded` error requires a multi-faceted approach:
- Increase Heap Size: Adjust JVM options to increase the maximum heap size, using parameters like `-Xmx` (e.g., `-Xmx2g` for 2 GB).
- Optimize Code: Refactor code to minimize object creation and enhance memory management practices.
- Garbage Collection Tuning: Experiment with different garbage collection algorithms (e.g., G1GC, CMS) and their associated tuning parameters.
- Identify Memory Leaks: Use heap dump analysis tools to locate and resolve memory leaks.
JVM Options for Tuning
Option | Description |
---|---|
-Xmx | Sets the maximum heap size |
-Xms | Sets the initial heap size |
-XX:+UseG1GC | Enables G1 garbage collector |
-XX:+UseConcMarkSweepGC | Enables CMS garbage collector |
-XX:GCTimeRatio=4 | Adjusts the ratio of time spent on GC |
Best Practices to Prevent OutOfMemoryError
Implementing best practices can significantly reduce the likelihood of encountering the `OutOfMemoryError`:
- Code Review: Regularly conduct code reviews to identify potential memory leaks.
- Object Pooling: Reuse objects where possible instead of creating new instances.
- Monitor Memory Usage: Continuously monitor application memory usage in production environments.
- Load Testing: Perform load testing to identify memory-related issues under stress.
By adhering to these practices and leveraging the appropriate tools, developers can mitigate the risk of `java.lang.OutOfMemoryError: GC overhead limit exceeded`, ensuring smoother application performance and reliability.
Understanding Java’s OutOfMemoryError: Insights from Experts
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “The ‘GC overhead limit exceeded’ error typically indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection without reclaiming enough memory. This often points to memory leaks or insufficient heap size, necessitating a thorough analysis of memory usage patterns in your application.”
Michael Chen (Lead Software Engineer, Cloud Solutions Corp.). “To effectively address the ‘OutOfMemoryError’, it is crucial to monitor the application’s memory consumption closely. Utilizing profiling tools can help identify memory bottlenecks and optimize object allocation, which can significantly reduce the frequency of this error in production environments.”
Sarah Patel (Java Performance Consultant, OptimizeIT). “When encountering the ‘GC overhead limit exceeded’ error, consider increasing the heap size or tuning the garbage collector settings. However, these are merely stopgap measures; the root cause must be addressed to prevent recurrence, which often involves refactoring code to manage memory more efficiently.”
Frequently Asked Questions (FAQs)
What does the error “java.lang.OutOfMemoryError: GC overhead limit exceeded” mean?
This error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection and recovering very little memory. Specifically, it signifies that the JVM has spent more than 98% of its total time in garbage collection and has recovered less than 2% of the heap memory.
What causes the “GC overhead limit exceeded” error?
The error is typically caused by insufficient heap memory allocation for the application, memory leaks, or inefficient memory usage patterns. It occurs when the application demands more memory than what is available, leading to excessive garbage collection attempts.
How can I resolve the “java.lang.OutOfMemoryError: GC overhead limit exceeded” error?
To resolve this error, consider increasing the heap size allocated to the JVM using the `-Xmx` parameter. Additionally, review your code for memory leaks, optimize data structures, and ensure that large objects are managed efficiently.
Can I disable the GC overhead limit check?
Yes, you can disable the GC overhead limit check by using the JVM option `-XX:-UseGCOverheadLimit`. However, this is not recommended as it may lead to other issues, such as application hangs or crashes due to excessive memory consumption.
What tools can help diagnose memory issues in a Java application?
Several tools can assist in diagnosing memory issues, including VisualVM, Eclipse Memory Analyzer (MAT), and Java Mission Control. These tools help analyze heap dumps, monitor memory usage, and identify memory leaks.
Is it possible to prevent the “GC overhead limit exceeded” error in future applications?
Yes, to prevent this error, implement best practices for memory management, such as using appropriate data structures, avoiding unnecessary object creation, and conducting regular performance testing. Additionally, monitor memory usage in production to identify potential issues early.
The Java `OutOfMemoryError: GC overhead limit exceeded` error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection (GC) with little success in reclaiming memory. This situation arises when the application is running low on memory, and the GC is unable to free up enough space to continue executing processes efficiently. It typically signals that the application requires more memory than is currently allocated, or that there may be memory leaks or inefficient memory usage patterns within the code.
To address this error, developers should first analyze the memory consumption of their applications. Tools such as Java VisualVM, Eclipse Memory Analyzer, or JProfiler can help identify memory usage patterns and potential leaks. Increasing the heap size allocated to the JVM can also provide a temporary solution, but it is crucial to investigate the underlying causes of excessive memory consumption to ensure long-term stability and performance of the application.
Another essential takeaway is the importance of optimizing code and resource management. Developers should adopt best practices such as using appropriate data structures, closing resources like database connections, and avoiding unnecessary object creation. Regular code reviews and performance testing can help identify areas for improvement and prevent the occurrence of the `OutOfMemoryError` in production environments.
Author Profile
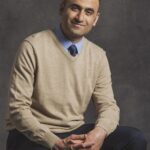
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?