Why Am I Seeing ‘java.lang.OutOfMemoryError: GC Overhead Limit Exceeded’ and How Can I Fix It?
In the world of Java programming, encountering errors is an inevitable part of the development journey. One of the more perplexing and frustrating issues developers face is the notorious `java.lang.OutOfMemoryError: GC overhead limit exceeded`. This error can bring even the most robust applications to a grinding halt, leaving developers scrambling to diagnose and resolve the underlying problems. Understanding this error not only helps in troubleshooting but also enhances your overall proficiency in memory management within Java applications.
At its core, the `GC overhead limit exceeded` error signals that the Java Virtual Machine (JVM) is spending too much time performing garbage collection while recovering very little memory. This situation often arises in applications that are either poorly optimized or that have reached their memory limits. As the JVM struggles to reclaim memory, it can lead to performance bottlenecks and ultimately result in application crashes. Recognizing the signs and symptoms of this error is crucial for developers who wish to maintain application stability and performance.
In this article, we will delve into the intricacies of the `java.lang.OutOfMemoryError: GC overhead limit exceeded`, exploring its causes, implications, and effective strategies for resolution. Whether you’re a seasoned Java developer or just starting out, understanding this error will empower you to build more resilient applications and enhance
Understanding the Error
The `java.lang.OutOfMemoryError: GC overhead limit exceeded` error occurs in Java applications when the garbage collector is spending too much time attempting to free up memory, while only managing to recover a minimal amount. This situation typically arises when the application is running low on memory and cannot allocate new objects, leading to performance degradation and ultimately causing the application to fail.
This error is often indicative of underlying memory management issues, which can be attributed to various factors:
- Memory Leaks: Objects that are no longer needed but are still referenced, preventing the garbage collector from reclaiming memory.
- Insufficient Heap Space: The allocated heap space may not be enough to handle the application’s demands.
- High Object Creation Rate: Excessive instantiation of objects, leading to frequent garbage collection cycles.
Common Causes
Identifying the specific cause of the `GC overhead limit exceeded` error is crucial for effective resolution. The primary causes include:
- Inefficient Code: Poorly designed algorithms or data structures that lead to unnecessary object creation.
- Large Data Sets: Processing large collections or datasets without proper management can overwhelm the heap.
- Improper Configuration: Suboptimal JVM settings, such as inadequate heap size or garbage collection parameters.
Diagnosing the Issue
Diagnosing the `GC overhead limit exceeded` error requires a systematic approach. Here are some strategies:
- Heap Dumps: Analyze heap dumps to identify memory leaks or objects consuming excessive memory.
- Profiling Tools: Utilize Java profiling tools (e.g., VisualVM, YourKit) to monitor memory usage and garbage collection behavior.
- Garbage Collection Logs: Enable GC logging to assess the frequency and duration of garbage collection cycles.
Tool | Purpose | Notes |
---|---|---|
VisualVM | Monitor and analyze Java applications | Comes with JDK; user-friendly interface |
JProfiler | Comprehensive profiling tool | Commercial; offers detailed analysis |
MAT (Memory Analyzer Tool) | Analyze heap dumps for memory leaks | Open-source; focuses on memory issues |
Solutions and Workarounds
Addressing the `GC overhead limit exceeded` error often involves multiple strategies to optimize memory usage:
- Increase Heap Size: Adjust the JVM options to allocate more memory to the heap using parameters like `-Xms` (initial heap size) and `-Xmx` (maximum heap size).
- Optimize Code: Refactor code to reduce unnecessary object creation and improve algorithm efficiency.
- Utilize Caching: Implement caching mechanisms to store frequently accessed data and minimize redundant processing.
- Garbage Collector Tuning: Explore different garbage collection algorithms (e.g., G1, ZGC) and tune their parameters to suit the application’s workload.
By systematically diagnosing and applying these solutions, developers can mitigate the `java.lang.OutOfMemoryError: GC overhead limit exceeded` error, leading to improved application performance and stability.
Understanding the GC Overhead Limit Exceeded Error
The `java.lang.OutOfMemoryError: GC overhead limit exceeded` error indicates that the Java Virtual Machine (JVM) is spending too much time in garbage collection (GC) while failing to free enough memory. This typically occurs when the application is using a substantial amount of memory and the GC is unable to recover sufficient space for new objects.
Key characteristics of this error include:
- High GC Activity: The JVM is performing garbage collection frequently, usually more than 98% of the time.
- Insufficient Memory Recovery: Less than 2% of the heap memory is being freed by the GC efforts.
- Memory Constraints: The application is likely under-provisioned in terms of memory allocation.
Common Causes
Several factors can lead to this error:
- Memory Leaks: Inefficient code that retains references to objects that should be garbage collected.
- Insufficient Heap Size: The allocated heap space may be too small for the application’s needs.
- Large Object Creation: Frequent creation of large objects can lead to fragmentation and increased GC overhead.
- Inefficient Data Structures: Using memory-heavy data structures that do not efficiently manage memory can exacerbate the issue.
Diagnostic Steps
When encountering this error, it is essential to perform a systematic diagnosis:
- Heap Dump Analysis: Generate a heap dump and analyze it using tools like Eclipse MAT or VisualVM to identify memory usage patterns.
- GC Logs: Enable GC logging by using JVM flags such as `-Xloggc:gc.log` and analyze the logs for GC performance metrics.
- Profiling: Use profiling tools (e.g., YourKit, JProfiler) to monitor memory usage and identify potential leaks or high memory consumption areas.
- Monitoring Tools: Implement monitoring solutions like Prometheus or Grafana to keep track of JVM metrics.
Solutions and Best Practices
To mitigate the `GC overhead limit exceeded` error, consider the following strategies:
- Increase Heap Size: Adjust the heap size parameters using `-Xms` and `-Xmx` flags to allocate more memory to the application.
- Optimize Code: Review and refactor code to eliminate memory leaks and optimize data structures.
- Adjust GC Settings: Experiment with different GC algorithms (e.g., G1, CMS) or tune GC parameters to improve performance.
- Use Soft/Weak References: Utilize soft or weak references for large cache objects to allow for better memory management by the GC.
Example Configuration
Here is an example of adjusting JVM parameters to handle memory more effectively:
Parameter | Description | Example Value |
---|---|---|
`-Xms` | Initial heap size | `-Xms512m` |
`-Xmx` | Maximum heap size | `-Xmx2048m` |
`-XX:+UseG1GC` | Enable G1 Garbage Collector | `-XX:+UseG1GC` |
`-XX:G1HeapRegionSize` | Size of the G1 heap region | `-XX:G1HeapRegionSize=16m` |
`-XX:MaxGCPauseMillis` | Maximum pause time for GC | `-XX:MaxGCPauseMillis=200` |
By implementing these solutions and best practices, you can significantly reduce the likelihood of encountering the `GC overhead limit exceeded` error and enhance the performance of your Java applications.
Understanding `java.lang.outofmemoryerror: gc overhead limit exceeded` from Expert Perspectives
Dr. Emily Carter (Senior Java Performance Engineer, Tech Innovations Inc.). “The `java.lang.outofmemoryerror: gc overhead limit exceeded` error typically indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection while making very little progress in freeing up memory. This can often be a sign of memory leaks or insufficient heap size, necessitating a review of the application’s memory management practices.”
Michael Chen (Lead Software Architect, Cloud Solutions Group). “When encountering the `gc overhead limit exceeded` error, it is crucial to analyze the application’s memory usage patterns. Tools such as VisualVM or Java Mission Control can provide insights into memory allocation and help identify objects that are not being properly released, leading to excessive garbage collection.”
Sarah Johnson (Java Development Consultant, CodeCraft Experts). “Addressing the `java.lang.outofmemoryerror: gc overhead limit exceeded` requires a multi-faceted approach. Increasing the heap size may provide a temporary fix, but it is essential to conduct a thorough code review to optimize memory usage and ensure that the application is designed to handle its workload efficiently.”
Frequently Asked Questions (FAQs)
What does the error “java.lang.outofmemoryerror: gc overhead limit exceeded” mean?
This error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection and recovering very little memory. Specifically, it means that more than 98% of the total time is spent on garbage collection, and less than 2% of the heap memory is reclaimed over a certain period.
What causes the “gc overhead limit exceeded” error?
This error typically arises from insufficient heap memory allocation for the application, memory leaks, or inefficient memory usage patterns. It can also occur when the application requires more memory than is available, leading to excessive garbage collection efforts.
How can I resolve the “gc overhead limit exceeded” error?
To resolve this error, consider increasing the heap size allocated to the JVM using the `-Xmx` option. Additionally, review your code for memory leaks, optimize memory usage, and analyze object creation patterns to reduce memory consumption.
Can I disable the GC overhead limit check?
Yes, you can disable the GC overhead limit check by using the JVM option `-XX:-UseGCOverheadLimit`. However, this is generally not recommended as it may lead to performance degradation and prolonged application downtime without addressing the underlying memory issues.
What tools can help diagnose memory issues related to this error?
Tools such as Java VisualVM, Eclipse Memory Analyzer (MAT), and JProfiler can help diagnose memory issues. These tools allow you to analyze heap dumps, monitor memory usage, and identify potential memory leaks or inefficient memory usage patterns.
Is the “gc overhead limit exceeded” error specific to any Java version?
No, the “gc overhead limit exceeded” error can occur in any version of Java that uses garbage collection. However, the behavior and thresholds may vary slightly between different Java versions and garbage collection algorithms, so tuning parameters may differ accordingly.
The error message “java.lang.OutOfMemoryError: GC overhead limit exceeded” is a common issue encountered in Java applications that indicates the Java Virtual Machine (JVM) is spending too much time performing garbage collection and is unable to reclaim sufficient memory. This typically occurs when the application is running low on heap space, and the garbage collector is unable to free up enough memory to meet the demands of the application. As a result, the performance of the application degrades significantly, leading to potential application crashes or unresponsiveness.
Several factors can contribute to this error, including memory leaks, improper configuration of the JVM heap size, or an application that requires more memory than what is allocated. Developers should investigate the memory usage patterns of their applications, utilizing profiling tools to identify memory leaks and optimize memory consumption. Additionally, adjusting the heap size parameters, such as increasing the maximum heap size, can help mitigate this issue, allowing the application to function more efficiently.
It is essential for developers to implement best practices in memory management to prevent encountering this error. Regularly monitoring application performance and memory usage, conducting thorough testing, and employing efficient coding practices can significantly reduce the likelihood of running into the “GC overhead limit exceeded” error. By taking proactive measures
Author Profile
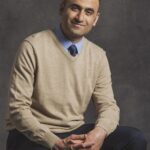
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?