Why Am I Getting a Java Lang OutOfMemoryError: GC Overhead Limit Exceeded?
In the world of Java programming, few errors can be as perplexing and frustrating as the `java.lang.OutOfMemoryError: GC overhead limit exceeded`. This error often emerges unexpectedly, leaving developers scrambling to diagnose the underlying issues that led to this memory crisis. As applications grow in complexity and scale, understanding this error becomes crucial for maintaining performance and reliability. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and strategies for effective resolution.
Overview
The `OutOfMemoryError: GC overhead limit exceeded` is a signal that the Java Virtual Machine (JVM) is struggling to manage memory effectively. Specifically, it indicates that the garbage collector is spending an excessive amount of time attempting to reclaim memory but is only able to free a minimal amount. This situation typically arises in environments where memory usage is high, leading to performance degradation and application instability.
Understanding the root causes of this error is essential for developers. Factors such as memory leaks, inadequate heap size, or inefficient data structures can all contribute to the problem. By identifying these issues early, developers can implement best practices in memory management and optimize their applications to prevent this error from occurring. As we explore the solutions and preventive measures, you’ll gain valuable insights
Understanding OutOfMemoryError: GC Overhead Limit Exceeded
OutOfMemoryError: GC overhead limit exceeded is a common error encountered in Java applications. It signifies that the Java Virtual Machine (JVM) has spent too much time performing garbage collection without successfully freeing up sufficient memory. Specifically, the JVM throws this error when more than 98% of the total time is spent in garbage collection and less than 2% of the heap memory is reclaimed.
This condition often indicates a serious memory leak or inefficient memory management within the application. It is crucial to identify the root cause to prevent application crashes and performance degradation.
Common Causes
Several factors can lead to this error, including:
- Memory Leaks: Unreleased object references that prevent the garbage collector from reclaiming memory.
- Large Data Structures: Using oversized collections or data structures that consume excessive heap space.
- Improper Configuration: Inadequate JVM settings for heap size leading to insufficient memory allocation.
- High Object Creation Rates: Rapid instantiation of objects without sufficient garbage collection cycles.
Diagnosing the Issue
To diagnose the root cause of the OutOfMemoryError, consider the following approaches:
- Heap Dumps: Analyze heap dumps to identify objects consuming large amounts of memory.
- Profiling Tools: Utilize profiling tools such as VisualVM, YourKit, or Eclipse Memory Analyzer to monitor memory usage.
- JVM Flags: Enable the following JVM flags to gather more information:
- `-XX:+PrintGCDetails`
- `-XX:+PrintGCTimeStamps`
These flags can help log detailed garbage collection activities.
Solutions and Best Practices
Implementing best practices can significantly mitigate the risk of encountering the GC overhead limit exceeded error. Some effective solutions include:
- Increasing Heap Size: Adjust the heap size settings using `-Xmx` and `-Xms` parameters.
- Optimizing Code: Refactor code to minimize unnecessary object creation and avoid memory leaks.
- Using Weak References: For large caches, consider using weak references that allow the garbage collector to reclaim memory.
- Garbage Collection Tuning: Adjust garbage collection settings to suit the application’s needs, using parameters like `-XX:GCTimeRatio` to balance between application performance and memory reclamation.
Monitoring Memory Usage
Regular monitoring of memory usage can help preemptively address potential issues. Key metrics to track include:
Metric | Description |
---|---|
Heap Size | The total memory allocated for the JVM heap. |
Garbage Collection Count | The number of times garbage collection has run. |
Average GC Pause Time | Average time taken by the garbage collector. |
Live Object Size | The total size of objects still in memory. |
Incorporating monitoring tools such as JMX, Prometheus, or Grafana can provide real-time insights into memory usage patterns.
Addressing OutOfMemoryError: GC overhead limit exceeded requires a comprehensive understanding of memory management in Java applications. By implementing proactive measures, optimizing code, and utilizing diagnostic tools, developers can significantly reduce the likelihood of encountering this error and improve overall application performance.
Understanding `OutOfMemoryError` and GC Overhead Limit Exceeded
The `java.lang.OutOfMemoryError: GC overhead limit exceeded` error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection (GC) with little success in reclaiming memory. This typically occurs when the application is running out of heap space and the garbage collector is unable to free enough memory for the application to continue functioning optimally.
Causes of the Error
- Memory Leak: Objects are being retained in memory longer than necessary, preventing the garbage collector from reclaiming that space.
- Insufficient Heap Size: The application may require more memory than what is allocated to the JVM.
- High Object Creation Rate: Excessive creation of objects can lead to rapid memory consumption.
- Poor GC Tuning: Default garbage collection settings may not be suitable for your application’s workload.
Symptoms
- Frequent GC pauses.
- Increased application latency.
- Slow performance, often accompanied by spikes in CPU usage.
Recommended Solutions
- Increase Heap Size: Adjust the maximum heap size using JVM options:
“`bash
-Xmx
“`
- Analyze Memory Usage:
- Use tools like VisualVM, Eclipse Memory Analyzer, or JProfiler to identify memory leaks and analyze object retention.
- Optimize Code:
- Review and refactor code to reduce unnecessary object creation.
- Ensure proper disposal of resources, such as closing database connections and streams.
- Adjust GC Settings: Modify the garbage collector settings for better performance:
- Use the G1 Garbage Collector:
“`bash
-XX:+UseG1GC
“`
- Set the `-XX:GCTimeRatio` to adjust GC thresholds.
Configuration Example
Parameter | Description | Example Value |
---|---|---|
`-Xms` | Initial heap size | `-Xms512m` |
`-Xmx` | Maximum heap size | `-Xmx2g` |
`-XX:+UseG1GC` | Use G1 Garbage Collector | `-XX:+UseG1GC` |
`-XX:GCTimeRatio` | Ratio of time spent in GC | `-XX:GCTimeRatio=4` |
`-XX:MaxGCPauseMillis` | Maximum pause time for GC | `-XX:MaxGCPauseMillis=100` |
Monitoring and Maintenance
- Implement regular monitoring of the application’s memory usage to catch potential issues early.
- Set up alerts for unusual GC activity or memory usage patterns.
- Conduct periodic reviews of application performance and resource utilization.
Conclusion
Addressing the `OutOfMemoryError: GC overhead limit exceeded` requires a multi-faceted approach, focusing on memory management, code optimization, and appropriate JVM configurations. Implementing these strategies will enhance application stability and performance.
Understanding Java’s OutOfMemoryError: Insights from Experts
Dr. Emily Chen (Senior Java Developer, Tech Innovations Inc.). “The ‘GC overhead limit exceeded’ error typically indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection and not enough time executing the application. This often suggests that the application is either using too much memory or has memory leaks that need to be addressed.”
Mark Thompson (Lead Software Architect, Cloud Solutions Group). “When encountering the ‘java.lang.OutOfMemoryError: GC overhead limit exceeded’, it is crucial to analyze the heap memory usage. Tools such as VisualVM or Java Mission Control can help identify memory usage patterns and pinpoint areas where optimizations can be made to reduce memory consumption.”
Lisa Patel (Performance Engineer, Enterprise Software Solutions). “To mitigate the ‘GC overhead limit exceeded’ error, consider increasing the heap size or optimizing the application’s memory usage. Additionally, reviewing the code for inefficient data structures or unnecessary object creations can significantly improve performance and reduce the likelihood of this error occurring.”
Frequently Asked Questions (FAQs)
What does the error “java lang outofmemoryerror gc overhead limit exceeded” mean?
This error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection and is unable to reclaim sufficient memory, leading to an OutOfMemoryError.
What causes the “gc overhead limit exceeded” error?
The error is typically caused by insufficient heap memory allocated to the JVM, memory leaks in the application, or an excessive number of objects being created and retained in memory.
How can I resolve the “gc overhead limit exceeded” error?
To resolve this error, consider increasing the heap size using JVM options like `-Xmx` and `-Xms`, optimizing your code to reduce memory consumption, and identifying and fixing memory leaks.
What JVM options can help manage garbage collection?
You can use options such as `-XX:+UseG1GC` for the G1 garbage collector, `-XX:+UseConcMarkSweepGC` for the CMS collector, or adjust parameters like `-XX:GCTimeRatio` to fine-tune garbage collection behavior.
How can I monitor memory usage in a Java application?
You can monitor memory usage using tools like VisualVM, JConsole, or Java Mission Control, which provide insights into heap memory, garbage collection activity, and object allocation.
What are the best practices to avoid “gc overhead limit exceeded” errors?
Best practices include optimizing data structures, minimizing object creation, using weak references where appropriate, and regularly profiling your application to identify memory usage patterns and potential leaks.
The Java `OutOfMemoryError: GC overhead limit exceeded` error indicates that the Java Virtual Machine (JVM) is spending too much time performing garbage collection (GC) but is unable to reclaim sufficient memory. This situation typically arises when the application is running with insufficient heap space or is experiencing memory leaks, leading to frequent GC cycles that consume CPU resources without freeing up enough memory for the application to function effectively.
To address this issue, developers should first analyze the memory usage of their applications. Tools such as Java VisualVM or profilers can help identify memory leaks or objects that are consuming excessive memory. Increasing the heap size using JVM options like `-Xmx` can provide immediate relief, but it is essential to ensure that the application is optimized for memory usage to prevent the error from recurring.
Another critical takeaway is the importance of optimizing code to manage memory efficiently. This includes practices such as using appropriate data structures, avoiding unnecessary object creation, and ensuring that unused objects are dereferenced. Additionally, monitoring the application’s performance and memory usage in production environments can help detect potential issues before they escalate into more significant problems.
In summary, the `GC overhead limit exceeded` error serves as a crucial indicator of underlying memory
Author Profile
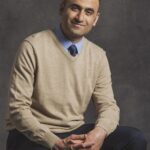
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?