Why Am I Getting a Java Net BindException: Address Already in Use?
If you’ve ever delved into the world of Java networking, you may have encountered the dreaded `BindException: Address already in use` error. This frustrating message can halt your application in its tracks, leaving you puzzled and searching for answers. Understanding the nuances of this error is crucial for developers, as it not only disrupts the flow of application development but also highlights the intricacies of network programming in Java. In this article, we will explore the causes of this common issue, its implications, and practical solutions to get your application back on track.
Overview
At its core, the `BindException` occurs when a Java application attempts to bind a socket to a specific IP address and port that is already in use by another process. This situation can arise in various scenarios, such as when multiple instances of an application are inadvertently launched or when a previous instance has not been properly terminated. Understanding the underlying mechanics of socket binding and the operating system’s role in managing network resources is essential for diagnosing and resolving this error.
Moreover, the implications of encountering a `BindException` extend beyond mere inconvenience. It can affect the reliability of your application, especially in environments where multiple services interact or compete for the same network resources. By gaining insight into the potential causes and remedies
Understanding BindException
A `BindException` in Java typically occurs when a program attempts to bind a socket to a local address or port that is already in use. This error can disrupt network operations and is crucial to address for maintaining smooth application performance. When a Java application encounters this exception, it usually indicates that the specified port is occupied by another process or has not been released properly.
Common Causes of BindException
Several factors can lead to a `BindException`:
- Port Already in Use: The most common reason is that another application is already using the same port.
- Improper Socket Closure: If a socket is not closed properly, the port remains in use until the operating system reclaims it.
- Firewall or Security Software: Sometimes, firewall settings or security software can interfere with socket binding.
- Rapid Restart of Services: Restarting services that use the same port too quickly can lead to binding issues.
How to Diagnose BindException
To diagnose a `BindException`, consider the following steps:
- Check Active Connections: Use tools like `netstat` to check which ports are currently in use.
- Identify Conflicting Applications: Determine if any other applications are using the same port.
- Inspect Firewall Settings: Review any firewall or security software settings that might block or interfere with the port binding.
- Log Exception Details: Capture the exception message and stack trace to identify the specific conditions leading to the error.
Resolving BindException
To resolve a `BindException`, follow these strategies:
- Change Port Number: Modify your application configuration to use a different port that is not in use.
- Ensure Proper Socket Management: Always close sockets when they are no longer needed. This can be done using a try-with-resources statement or explicitly closing sockets in a finally block.
- Wait for Release: If a port is temporarily occupied, wait a few moments and try again, as the OS may release the port after a short time.
- Terminate Conflicting Processes: If you identify a process occupying the port, terminate it if it’s not essential.
Resolution Method | Description |
---|---|
Change Port Number | Select an unused port for your application. |
Proper Socket Management | Ensure that sockets are closed properly after use. |
Wait for Release | Give the OS time to release the port. |
Terminate Conflicting Processes | End processes that are using the desired port. |
By following these guidelines, developers can effectively mitigate the issues associated with `BindException`, ensuring that their Java applications run smoothly without network interruptions.
Understanding BindException in Java Networking
`BindException` is a subclass of `SocketException`, which indicates that an error occurred while attempting to bind a socket to a local address and port. The specific message “address already in use” arises when the desired port is already occupied by another process.
Common Causes of BindException
Several factors can lead to this exception, including:
- Port Conflict: Another application is already using the same port.
- Improper Socket Closure: A socket was not properly closed, leaving the port in a TIME_WAIT state.
- Firewall or Security Software: Interference from security software that restricts port usage.
- Insufficient Permissions: The application might not have permission to bind to the specified port.
Diagnosing BindException
To troubleshoot a `BindException`, consider the following steps:
- Check Active Connections:
- Use command-line tools to identify which process is using the port.
- On Linux/Mac: `lsof -i :
` - On Windows: `netstat -ano | findstr :
`
- Kill the Process:
- Once identified, terminate the process if it’s safe to do so.
- On Linux/Mac: `kill
` - On Windows: `taskkill /PID
/F`
- Change Port:
- If the port is essential for another application, consider changing the port number in your application’s configuration.
- Socket Reuse Options:
- Consider using the `SO_REUSEADDR` socket option to allow your application to bind to a port that is in a TIME_WAIT state.
Example Code for Handling BindException
Below is a simple example demonstrating how to handle `BindException` gracefully in a Java application:
“`java
import java.net.ServerSocket;
import java.net.BindException;
public class Server {
public static void main(String[] args) {
int port = 8080;
while (true) {
try (ServerSocket serverSocket = new ServerSocket(port)) {
System.out.println(“Server started on port: ” + port);
// Server logic goes here
break; // Exit the loop if successful
} catch (BindException e) {
System.out.println(“Port ” + port + ” is already in use. Trying next port…”);
port++; // Increment port number and retry
} catch (Exception e) {
e.printStackTrace();
break; // Break on other exceptions
}
}
}
}
“`
Best Practices to Avoid BindException
To minimize the risk of encountering `BindException`, adhere to the following best practices:
- Port Management: Keep track of which ports are in use by your applications.
- Proper Resource Cleanup: Ensure sockets are closed appropriately in `finally` blocks or using try-with-resources.
- Use Dynamic Ports: Allow the system to choose an available port by specifying port `0` when creating a `ServerSocket`.
- Configuration Files: Store port numbers in configuration files, enabling easy adjustments without altering code.
Conclusion on Handling BindException
Addressing `BindException` effectively requires understanding its causes, diagnosing issues through system tools, and implementing best practices in code and configuration. By following the outlined strategies, developers can create robust Java networking applications less prone to binding issues.
Understanding Java Net BindException: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The `BindException: Address already in use` error in Java typically indicates that an application is attempting to bind to a port that is already occupied by another process. It is crucial to ensure that the port is free before starting the server, or to configure the application to use a different port.”
James Liu (Network Architect, Global Systems Solutions). “In many cases, this issue arises during development when multiple instances of an application are inadvertently launched. Developers should implement proper shutdown procedures to release the port or utilize tools like `netstat` to identify which process is occupying the port.”
Sarah Thompson (DevOps Specialist, CloudTech Enterprises). “To avoid encountering `BindException`, it is advisable to incorporate error handling in your Java application. This allows for graceful degradation and provides users with informative feedback when the desired port is unavailable, instead of crashing the application.”
Frequently Asked Questions (FAQs)
What does the `java.net.BindException: Address already in use` error mean?
This error indicates that an attempt to bind a socket to a specific IP address and port has failed because that address and port are already in use by another process or application.
How can I identify which process is using the port?
You can identify the process using the port by executing commands such as `netstat -ano` on Windows or `lsof -i :
What steps can I take to resolve the `BindException`?
To resolve the `BindException`, you can either terminate the process currently using the port, change your application to use a different port, or configure your application to handle port conflicts gracefully.
Can I configure my Java application to automatically select an available port?
Yes, you can configure your Java application to automatically select an available port by specifying port number `0` when creating a server socket. The system will then assign an available port.
Are there any common scenarios that lead to this error?
Common scenarios include running multiple instances of the same application, misconfigured services, or lingering processes from previous executions that have not released the port.
Does this error occur only in server applications?
No, this error can occur in both client and server applications whenever there is an attempt to bind a socket to an address and port that is already occupied.
The Java `BindException` indicating that an address is already in use typically arises when a network application attempts to bind a socket to a port that is already occupied by another process. This issue is common in server applications that listen for incoming connections, as they require exclusive access to a specific port. When a bind operation fails due to this error, it can disrupt the functionality of the application and lead to service interruptions.
To resolve this issue, developers should first identify which process is currently using the desired port. Tools such as `netstat`, `lsof`, or platform-specific utilities can help determine the offending process. Once identified, the developer can either terminate the conflicting process or choose a different port for their application. Additionally, implementing proper error handling and logging can aid in diagnosing and preventing future occurrences of this exception.
Understanding the underlying causes of a `BindException` and taking proactive measures can significantly enhance the robustness of network applications. It is crucial for developers to be aware of port management and to design applications that can gracefully handle such exceptions. By doing so, they can ensure better reliability and user experience in their software solutions.
Author Profile
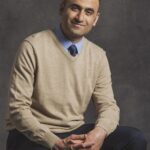
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?