Why Am I Facing a Java Net ConnectException: Connection Timed Out?
In the world of Java programming, connectivity issues can often lead to frustrating roadblocks, particularly when developers encounter the dreaded `java.net.ConnectException: Connection timed out`. This error message, while seemingly straightforward, can stem from a myriad of underlying causes that challenge even seasoned programmers. Understanding the nuances of this exception is crucial for troubleshooting and ensuring seamless network communication in your applications. Whether you’re developing a web service, a client-server application, or simply trying to connect to a database, grasping the intricacies of connection timeouts can save you hours of debugging and enhance your application’s reliability.
A `ConnectException` occurs when a Java application attempts to establish a connection to a remote server but fails to do so within a specified timeframe. This timeout can arise from various factors, including network issues, server unavailability, or misconfigured client settings. As developers, it’s essential to recognize the signs of a connection timeout and to systematically investigate the root causes. By doing so, you can implement effective solutions that not only resolve the immediate issue but also fortify your application against future connectivity challenges.
In the following sections, we will delve deeper into the common scenarios that lead to a `java.net.ConnectException`, explore best practices for diagnosing and resolving these issues, and provide insights into optimizing
Understanding Connection Timeout Exceptions
Connection timeout exceptions in Java, specifically the `java.net.ConnectException: Connection timed out`, occur when a connection attempt to a remote server fails due to the server not responding within a specified time frame. This can arise from various factors, including network issues, server downtime, or incorrect configuration settings.
The timeout duration is typically defined in the application’s networking code or configuration. If the server does not respond before this timeout period elapses, the Java runtime throws a `ConnectException`. Understanding how to handle these exceptions is crucial for building robust networked applications.
Common Causes of Connection Timeout
Several factors can contribute to a connection timeout exception in Java applications:
- Network Issues: Poor network connectivity, firewalls, or VPN settings that block the connection.
- Server Unavailability: The target server may be down or unreachable due to maintenance or network failures.
- Incorrect Configuration: An incorrect IP address, port number, or DNS issue can lead to connection failures.
- Heavy Server Load: If the server is overloaded with requests, it may not be able to respond in a timely manner.
- Timeout Settings: The default timeout settings may be too low for the specific application requirements.
Diagnosing Connection Timeout Issues
To diagnose connection timeout exceptions effectively, consider the following steps:
- Check Network Connectivity: Use tools like `ping` or `traceroute` to verify if the server is reachable.
- Review Application Logs: Analyze application logs for any specific error messages or patterns leading to the timeout.
- Verify Configuration Settings: Ensure that the IP address and port number are correct and that the server is configured to accept incoming connections.
- Monitor Server Health: Use monitoring tools to check if the server is under heavy load or experiencing downtime.
- Adjust Timeout Settings: Consider increasing the timeout settings if the server is known to respond slowly.
Handling Connection Timeouts in Java
When encountering a connection timeout, it is essential to implement proper error handling strategies to gracefully manage the exception. Below are typical approaches:
- Retry Mechanism: Implement a retry mechanism that attempts to reconnect after a brief delay.
- User Notifications: Inform users of connectivity issues and possible resolutions.
- Fallback Strategies: Use alternative servers or services if available.
Here’s a simple example of handling connection timeouts in Java:
“`java
import java.net.Socket;
import java.net.SocketException;
public class ConnectionManager {
public void connect(String host, int port) {
try {
Socket socket = new Socket();
socket.connect(new InetSocketAddress(host, port), 5000); // 5 seconds timeout
} catch (SocketException e) {
System.out.println(“Socket exception: ” + e.getMessage());
} catch (IOException e) {
System.out.println(“Connection timed out: ” + e.getMessage());
}
}
}
“`
Best Practices for Avoiding Connection Timeout
To minimize the risk of connection timeout exceptions, consider the following best practices:
- Use Connection Pools: Implement connection pooling to manage database or service connections efficiently.
- Optimize Server Performance: Ensure the server is well-optimized and capable of handling expected traffic loads.
- Regular Monitoring: Continuously monitor network and server performance to identify potential issues early.
- Proper Configuration: Review and configure timeout settings based on the application’s specific needs.
Cause | Solution |
---|---|
Network Issues | Check firewall settings and network routes. |
Server Unavailability | Ensure the server is up and reachable. |
Incorrect Configuration | Verify IP and port settings. |
Heavy Server Load | Scale the server resources or optimize request handling. |
Understanding Connection Timeout
Connection timeout occurs when a Java application attempts to connect to a server but does not receive a response within a specified time frame. This can result in a `java.net.ConnectException: Connection timed out` error. Several factors can contribute to this issue, including network configuration, server availability, and application settings.
Common Causes of Connection Timeout
- Network Issues: Poor network conditions or interruptions can lead to connection failures.
- Server Unavailability: The target server might be down or experiencing high load, causing it to be unresponsive.
- Firewall Restrictions: Firewalls can block outbound or inbound traffic, preventing successful connections.
- Incorrect Hostname or Port: Misconfigured connection parameters may lead to attempts to connect to the wrong address or port.
- DNS Resolution Failures: Problems with DNS can prevent the application from resolving the server’s hostname.
Troubleshooting Connection Timeout
To effectively troubleshoot and resolve connection timeout issues, consider the following steps:
- Check Network Connectivity:
- Use commands like `ping` or `traceroute` to verify connectivity to the server.
- Confirm that your local network is functioning properly.
- Verify Server Status:
- Ensure that the target server is running and accessible.
- Check server logs for any signs of issues or overload.
- Inspect Firewall Settings:
- Review firewall rules on both client and server sides.
- Temporarily disable firewalls to determine if they are causing the issue.
- Validate Connection Parameters:
- Ensure that the hostname and port number used in the connection string are correct.
- Test using a simple client to connect to the server.
- DNS Configuration:
- Verify that the DNS server settings are correct.
- Try using an alternative DNS service to rule out resolution problems.
Example Code to Handle Connection Timeout
Properly handling connection timeouts in Java can enhance application reliability. Here is an example of how to set a timeout when establishing a connection:
“`java
import java.net.Socket;
import java.net.InetSocketAddress;
import java.net.SocketTimeoutException;
public class ConnectionExample {
public static void main(String[] args) {
String host = “example.com”;
int port = 80;
int timeout = 5000; // 5 seconds
try (Socket socket = new Socket()) {
socket.connect(new InetSocketAddress(host, port), timeout);
System.out.println(“Connection established successfully.”);
} catch (SocketTimeoutException e) {
System.err.println(“Connection timed out: ” + e.getMessage());
} catch (Exception e) {
System.err.println(“An error occurred: ” + e.getMessage());
}
}
}
“`
This code attempts to connect to a specified host and port, incorporating a timeout setting to ensure that long waits are avoided.
Best Practices to Avoid Connection Timeout
- Set Appropriate Timeouts: Use reasonable timeout settings based on expected server response times.
- Implement Retry Logic: If a connection fails, implement retry mechanisms with exponential backoff.
- Monitor Network and Server Health: Regularly check the status of your network and server to preemptively address issues.
- Use Asynchronous Connections: Consider using non-blocking I/O or asynchronous calls to improve responsiveness.
Connection timeout errors can stem from various sources, and understanding these causes is essential for effective troubleshooting. By following best practices and implementing robust error handling, developers can significantly reduce the incidence of these issues in their applications.
Understanding Java Net ConnectException: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A Java Net ConnectException indicating a connection timed out usually suggests that the application is unable to reach the server within the expected time frame. This can be due to network issues, server overload, or incorrect configurations in the application settings.”
Michael Thompson (Network Security Analyst, CyberSafe Solutions). “When encountering a connection timed out error in Java, it is crucial to examine the firewall settings and network policies. Often, security measures can inadvertently block necessary traffic, leading to such exceptions.”
Linda Garcia (Java Development Consultant, CodeCraft Experts). “To effectively troubleshoot a connection timed out error in Java, developers should implement proper logging mechanisms. This allows for better visibility into the request lifecycle and can help identify where the timeout occurs, whether at the client or server side.”
Frequently Asked Questions (FAQs)
What causes a Java Net ConnectException: Connection Timed Out?
A Java Net ConnectException: Connection Timed Out occurs when a network connection attempt exceeds the specified timeout duration without establishing a connection. This can be due to network issues, incorrect IP addresses, or the target server being down.
How can I troubleshoot a Connection Timed Out error in Java?
To troubleshoot this error, check your network connectivity, verify the server’s availability, ensure the correct IP address and port are being used, and inspect firewall settings that may be blocking the connection.
What are common scenarios that lead to a Connection Timed Out in Java applications?
Common scenarios include attempting to connect to a server that is offline, misconfigured network settings, using an incorrect port number, or having a firewall that restricts access to the desired service.
Is there a way to increase the timeout duration in Java?
Yes, you can increase the timeout duration by setting the timeout parameter in the connection method. For example, when using `Socket` or `HttpURLConnection`, you can specify the timeout values using `setSoTimeout()` or `setConnectTimeout()` methods.
Can a Connection Timed Out error be caused by server-side issues?
Yes, server-side issues such as high load, misconfiguration, or resource exhaustion can lead to a Connection Timed Out error. If the server is unable to handle incoming connections, it may not respond within the expected timeframe.
What should I do if the issue persists after troubleshooting?
If the issue persists, consider reviewing server logs for errors, consulting with network administrators, or using network diagnostic tools to analyze the connection path for potential bottlenecks or failures.
The Java `Net ConnectException: Connection timed out` error typically indicates that a network connection attempt has failed due to the target server being unreachable within a specified time frame. This issue can arise from various factors, including network configuration problems, firewall restrictions, server downtime, or incorrect address specifications. Understanding the underlying causes is crucial for effectively troubleshooting and resolving the error.
To address this issue, it is essential to check the network connectivity between the client and the server. This may involve verifying that the server is operational, ensuring that the correct IP address and port are being used, and confirming that any firewalls or security settings are not blocking the connection. Additionally, examining the server logs can provide insights into whether the server is receiving connection requests and if any errors are being logged on the server side.
Another important takeaway is the significance of proper timeout settings in Java applications. Adjusting the timeout values can help manage expectations regarding connection attempts and ensure that applications do not hang indefinitely. Furthermore, implementing robust error handling can enhance the resilience of applications, allowing them to gracefully handle such exceptions and provide meaningful feedback to users.
Author Profile
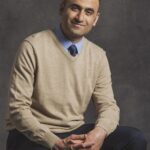
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?