How to Resolve Java Net ConnectException: Why Does Connection Time Out in Java?
In the world of Java programming, network connectivity issues can be a significant hurdle for developers and system administrators alike. One common error that often leaves users scratching their heads is the `java.net.ConnectException: Connection timed out`. This seemingly cryptic message can disrupt applications and halt progress, but understanding its underlying causes is essential for troubleshooting and resolution. Whether you’re building a web application, connecting to a database, or integrating with external APIs, grasping the nuances of this error can empower you to maintain robust and resilient systems.
As you delve into the intricacies of the `ConnectException`, it’s crucial to recognize that this error typically arises when a Java application attempts to establish a connection to a remote server but fails to do so within a specified time frame. This timeout can occur for various reasons, including network configuration issues, server unavailability, or even firewall restrictions. By identifying these potential pitfalls, developers can take proactive measures to ensure seamless connectivity and enhance the overall user experience.
Furthermore, understanding how to effectively debug and resolve connection timeouts can save valuable time and resources in the development process. From examining network settings to implementing retry mechanisms, there are numerous strategies to mitigate the impact of this error. In the following sections, we will explore the common causes, troubleshooting techniques, and
Understanding Connection Timeout in Java
A `ConnectException` in Java, particularly one indicating a “connection timed out,” typically arises when a network connection is attempted but not established within a specified timeout period. This can occur due to various reasons, including server unavailability, network issues, or incorrect configuration.
When a connection attempt exceeds the timeout limit set by the application, a `ConnectException` is thrown, signaling that the remote host is unreachable or that there are underlying network problems.
Common Causes of Connection Timeout
The primary reasons for a connection timeout in Java applications include:
- Server Unavailability: The target server may be down or not listening on the specified port.
- Network Issues: Firewalls or routers may be blocking access to the server or port.
- Incorrect Address/Port: The hostname or port number may be incorrect.
- Heavy Load: The server might be under heavy load, causing delays in response.
- DNS Resolution Failure: Failure to resolve the hostname to an IP address can also lead to timeouts.
Configuring Timeout Settings
In Java, you can configure connection timeout settings when establishing a connection using `Socket` or `URLConnection`. Here’s how to set a timeout for a `Socket` connection:
“`java
Socket socket = new Socket();
socket.connect(new InetSocketAddress(“hostname”, port), timeout);
“`
For `URLConnection`, use:
“`java
URLConnection urlConnection = url.openConnection();
urlConnection.setConnectTimeout(timeout);
“`
Handling Connection Timeout Exceptions
To effectively manage `ConnectException`, it’s essential to implement error handling in your Java code. Here’s a typical approach:
“`java
try {
// Attempt to connect
} catch (ConnectException e) {
System.err.println(“Connection timed out: ” + e.getMessage());
// Handle the exception
} catch (IOException e) {
System.err.println(“IO Exception: ” + e.getMessage());
}
“`
Best Practices for Preventing Connection Timeouts
To minimize the risk of connection timeouts in Java applications, consider the following best practices:
- Set Appropriate Timeouts: Use realistic timeout values based on expected network conditions.
- Use Connection Pooling: Implement connection pooling to manage multiple connections efficiently.
- Monitor Network Performance: Regularly check network health and server performance.
- Implement Retry Logic: Consider implementing a retry mechanism for transient failures.
- Log Connection Attempts: Maintain logs of connection attempts for troubleshooting.
Example of Timeout Configuration
The following table summarizes the timeout configuration for different connection types in Java:
Connection Type | Timeout Configuration |
---|---|
Socket | socket.connect(new InetSocketAddress(“hostname”, port), timeout); |
URLConnection | urlConnection.setConnectTimeout(timeout); |
HttpClient | RequestConfig.custom().setConnectTimeout(timeout).build(); |
By adhering to these practices and understanding the underlying causes of `ConnectException`, developers can create more robust Java applications that handle network connectivity issues effectively.
Understanding Connection Timed Out Errors
A `java.net.ConnectException: Connection timed out` error occurs when a Java application attempts to connect to a remote server but fails due to the connection request not receiving a response within a specified time period. This exception indicates that the application is unable to establish a connection, typically due to network issues or server unavailability.
Common Causes of Connection Timed Out
Several factors can lead to a connection timeout in Java:
- Network Configuration Issues: Incorrect firewall settings or network configurations can block access to the server.
- Server Unavailability: The target server may be down or overloaded, preventing it from responding to connection requests.
- Incorrect Host or Port: Specifying the wrong hostname or port number in the connection string can lead to timeouts.
- Slow Network: High latency or slow network conditions can delay the connection establishment beyond the timeout threshold.
- Proxy Server Issues: If a proxy server is misconfigured or down, it may impede connections to external servers.
Timeout Configuration in Java
Java provides options to configure connection timeouts using the `URLConnection` class or networking libraries like Apache HttpClient. Here’s how you can set timeouts:
Method | Description |
---|---|
setConnectTimeout(int timeout) | Sets the timeout for establishing a connection. |
setReadTimeout(int timeout) | Sets the timeout for waiting for data after establishing a connection. |
Example of setting timeouts using `HttpURLConnection`:
“`java
URL url = new URL(“http://example.com”);
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setConnectTimeout(5000); // 5 seconds
connection.setReadTimeout(5000); // 5 seconds
“`
Debugging Connection Timed Out Issues
To effectively troubleshoot a connection timed out error, consider the following steps:
- Ping the Server: Use command-line tools to ping the target server to check its availability.
- Check Firewall Settings: Ensure that local and remote firewall settings allow traffic on the specified port.
- Validate Host and Port: Double-check the hostname and port number in your code.
- Test Network Connectivity: Use tools like `traceroute` to identify any network issues between your application and the server.
- Review Application Logs: Look for additional error messages in your application logs that may provide insights.
Best Practices for Handling Connection Timeouts
Implementing best practices can help mitigate connection timeout issues:
- Increase Timeout Values: Adjust timeout settings based on network conditions and server response times.
- Use Retry Logic: Implement retry mechanisms to handle transient network issues.
- Asynchronous Calls: Consider using asynchronous calls to prevent blocking the main application thread.
- Graceful Error Handling: Ensure your application gracefully handles connection exceptions and provides meaningful feedback to users.
By understanding the causes and solutions for `java.net.ConnectException: Connection timed out`, developers can create more robust applications that handle network issues effectively.
Understanding Java Net ConnectException: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A Java Net ConnectException indicating a connection timed out is often the result of network issues, such as firewall restrictions or incorrect server configurations. It is crucial to ensure that the target server is reachable and that the necessary ports are open.”
Mark Thompson (Network Architect, Global Tech Solutions). “When encountering a connection timed out error in Java, it is essential to analyze both client-side and server-side logs. This will help identify whether the issue lies within the application code or the network infrastructure.”
Lisa Nguyen (Java Developer Advocate, Open Source Community). “To mitigate connection timeout issues in Java applications, developers should implement proper error handling and retry mechanisms. Additionally, configuring appropriate timeout settings in the application can prevent abrupt failures during network latency.”
Frequently Asked Questions (FAQs)
What does the error “java.net.ConnectException: Connection timed out” mean?
This error indicates that a connection attempt to a remote server has failed because the server did not respond within the expected time frame. It usually occurs when the server is unreachable or not listening on the specified port.
What are common causes of a “Connection timed out” error in Java?
Common causes include network issues, incorrect server addresses, firewalls blocking the connection, server downtime, or the server being overloaded and unable to accept new connections.
How can I troubleshoot a “Connection timed out” error in my Java application?
To troubleshoot, check the server address and port for correctness, ensure the server is running and reachable, verify network connectivity, and inspect firewall settings. Additionally, consider increasing the timeout settings in your code.
Can a proxy server cause a “Connection timed out” error in Java?
Yes, if your application is configured to use a proxy server, and the proxy is misconfigured or down, it can lead to a “Connection timed out” error. Ensure the proxy settings are correct and the proxy server is operational.
What steps can I take to increase the timeout duration in Java?
You can increase the timeout duration by setting the connection timeout and read timeout properties when creating a connection. For example, use `URLConnection.setConnectTimeout(int timeout)` and `URLConnection.setReadTimeout(int timeout)` methods to specify the desired timeout values.
Is it possible to handle “Connection timed out” exceptions gracefully in Java?
Yes, you can handle this exception using try-catch blocks. Implementing a retry mechanism or providing user feedback can enhance user experience when a timeout occurs. Always log the exception for further analysis.
In summary, the `java.net.ConnectException: Connection timed out` error in Java typically indicates that a connection attempt to a remote server has failed due to the server being unreachable within a specified time frame. This situation can arise from various factors, including network issues, incorrect server addresses, firewall restrictions, or server downtime. Understanding the underlying causes of this exception is crucial for troubleshooting and resolving connectivity problems effectively.
Key takeaways include the importance of verifying network configurations and ensuring that the target server is operational. Developers should check the correctness of the server’s IP address and port number, as well as confirm that any firewalls or security groups are properly configured to allow traffic. Additionally, implementing proper error handling and timeout settings in the Java application can provide more informative feedback and enhance the robustness of the application.
Furthermore, it is advisable to utilize tools such as `ping` or `telnet` to test connectivity to the server before attempting to establish a connection through the Java application. By proactively diagnosing network issues and applying best practices in error handling, developers can significantly reduce the occurrence of connection timeouts and improve the overall reliability of their applications.
Author Profile
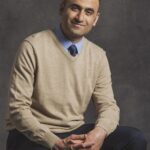
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?