How to Safely Retrieve a Double from a Java Object: Handle Null Values Effectively?
In the world of Java programming, handling data types effectively is crucial for building robust applications. One common challenge developers face is managing the nuances of object types, particularly when dealing with numerical values. The question of how to retrieve a double value from an object, or determine if that value is null, is a scenario that arises frequently in Java development. Whether you’re working with databases, APIs, or complex data structures, understanding how to navigate these situations can significantly enhance your coding efficiency and application reliability.
When you work with Java objects, the distinction between primitive types and their wrapper classes becomes paramount. The `Double` class, for instance, allows you to encapsulate a double value while also providing the flexibility of representing null. This duality can lead to complications, especially when you need to extract a double from an object that may or may not contain a valid value. Developers must employ best practices to ensure that they handle potential null values gracefully, avoiding runtime exceptions that could disrupt application flow.
Moreover, the approach you take to retrieve a double or null from an object can affect the overall design of your code. By leveraging techniques such as optional values, null checks, and default assignments, you can create a more resilient application that anticipates and handles potential data inconsistencies. As we delve deeper
Understanding Java Objects and Their Handling of Double Values
In Java, the handling of numeric values, particularly double types, can be nuanced due to the distinction between primitive types and their corresponding wrapper classes. The `Double` class is the wrapper for the primitive `double`, allowing for the representation of null values. Understanding how to retrieve a double value or handle nulls effectively is crucial for robust Java application development.
Retrieving Double Values from Objects
When working with objects in Java, particularly when you expect a `Double` value, it is essential to consider that the value might be absent (null). To retrieve a double value safely, you can use the following approaches:
- Using Wrapper Classes: The `Double` class can be used to check for null values.
- Using Optional: Java 8 introduced `Optional`, which provides a way to avoid null references.
Here’s an example of how to retrieve a double value safely:
“`java
public Double getDoubleValue(MyObject obj) {
if (obj != null && obj.getDoubleValue() != null) {
return obj.getDoubleValue();
}
return null; // or return 0.0 if a default is needed
}
“`
Using Optional for Safe Value Retrieval
The `Optional` class can streamline the process of checking for null values. This approach allows you to avoid null pointer exceptions while providing a clear API for handling absent values.
Example of using `Optional`:
“`java
public Optional
return Optional.ofNullable(obj)
.map(MyObject::getDoubleValue);
}
“`
This method returns an `Optional
“`java
Optional
value.ifPresentOrElse(System.out::println, () -> System.out.println(“Value is absent”));
“`
Comparison of Approaches
Approach | Advantages | Disadvantages |
---|---|---|
Direct Check | Simple and straightforward | Prone to NullPointerExceptions if not careful |
Optional | Safe handling of absent values | Slightly more verbose, may introduce additional overhead |
Conclusion on Handling Double Values
retrieving double values from objects in Java requires careful consideration of nullability. Utilizing wrapper classes and the `Optional` type provides developers with robust methods to handle potential null values, ensuring the stability and reliability of Java applications.
Handling Double Values in Java Objects
In Java, dealing with double values that may potentially be null requires careful consideration, especially when interacting with objects. This is particularly important because the primitive `double` type cannot hold a null value, while the `Double` class (the wrapper for the primitive type) can be null.
Retrieving Double Values
To retrieve a double value from an object that may or may not be null, you can follow these methods:
- Direct Access with Null Check:
Use a conditional check to determine if the object is null before attempting to access its double value.
“`java
Double myDouble = myObject.getDoubleValue();
if (myDouble != null) {
double value = myDouble;
// use value
} else {
// handle the null case
}
“`
- Using Optional:
Java’s `Optional` class can be beneficial for avoiding null checks and providing a default value if the object is null.
“`java
Double myDouble = Optional.ofNullable(myObject)
.map(MyObject::getDoubleValue)
.orElse(0.0); // default value
“`
- Try-Catch Block:
In situations where an exception might be thrown during value retrieval, a try-catch block can be implemented.
“`java
double value;
try {
value = myObject.getDoubleValue();
} catch (NullPointerException e) {
value = 0.0; // handle null case
}
“`
Conversion from String to Double
When converting strings to double, the potential for null or invalid inputs must be addressed.
- Using try-catch:
This method handles parsing errors effectively.
“`java
String doubleString = myObject.getDoubleString();
double value;
try {
value = Double.parseDouble(doubleString);
} catch (NumberFormatException | NullPointerException e) {
value = 0.0; // handle invalid or null string
}
“`
- Using Optional:
An alternative using `Optional` can streamline the process.
“`java
double value = Optional.ofNullable(myObject.getDoubleString())
.map(Double::parseDouble)
.orElse(0.0);
“`
Best Practices
- Always prefer using `Double` over `double` when there is a possibility of null values.
- Utilize `Optional` to reduce boilerplate code related to null checks and to express intent clearly.
- Ensure proper exception handling when dealing with user input or external data sources.
Summary Table of Methods
Method | Description | Example Code |
---|---|---|
Direct Access | Checks for null before accessing | `if (myDouble != null) { … }` |
Using Optional | Simplifies null handling with defaults | `Optional.ofNullable(…).orElse(0.0)` |
Try-Catch Block | Catches exceptions during retrieval/conversion | `try { … } catch (Exception e) { … }` |
By applying these methods and practices, developers can effectively manage double values in Java objects while ensuring robustness in their applications.
Expert Insights on Handling Double Values in Java Objects
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When dealing with Java objects that may return a double or null, it is crucial to implement proper null checks to avoid NullPointerExceptions. Utilizing Optional
can provide a more robust solution, allowing developers to handle the absence of a value gracefully.”
Michael Chen (Java Developer Advocate, CodeCraft). “In Java, the primitive type double cannot hold a null value, which poses challenges when working with objects. I recommend encapsulating the double in an object wrapper like Double, allowing for null representation. This approach enhances code readability and maintainability.”
Sarah Thompson (Lead Architect, FutureTech Solutions). “To effectively manage scenarios where a Java object might return a double or null, employing the ternary operator can simplify the logic. This allows developers to provide a default value when the object yields null, ensuring that the application remains stable and user-friendly.”
Frequently Asked Questions (FAQs)
How can I safely retrieve a double value from a Java object that might be null?
To safely retrieve a double value from a potentially null object, use the `Optional` class. For example, `Optional.ofNullable(object).map(MyObject::getDoubleValue).orElse(null);` returns the double value or null if the object is null.
What is the best way to handle null values when working with doubles in Java?
The best practice is to use `Optional
Can I use primitive double types with null values in Java?
No, primitive types like double cannot hold null values. Instead, use the wrapper class `Double`, which can be null, or use `Optional
What happens if I try to unbox a null Double to a double?
Attempting to unbox a null `Double` to a primitive `double` will throw a `NullPointerException`. Always check for null before unboxing.
Is there a way to set a default value when retrieving a double from an object that may be null?
Yes, you can use the `Optional` class with a default value. For instance, `Optional.ofNullable(object).map(MyObject::getDoubleValue).orElse(defaultValue);` will return the default value if the object is null.
How do I convert a string to a double while handling potential null values?
Use `Double.valueOf(string)` to convert a string to a `Double`. If the string is null, it will return a `NullPointerException`. Instead, check for null and handle it appropriately, possibly returning null or a default value.
In Java, handling objects that may or may not contain a double value is a common scenario, particularly when dealing with data that can be absent or uninitialized. The concept revolves around the use of wrapper classes, specifically the `Double` class, which can represent a double value or be null. This allows developers to differentiate between a valid double value and the absence of a value, thereby avoiding potential issues related to primitive data types.
When retrieving a double from an object, it is essential to implement checks to determine whether the object is null or if it contains a valid double value. This can be achieved through methods such as `Optional
Furthermore, the use of default values is a practical approach when dealing with potential nulls. If a double is expected but not present, developers can define a fallback value to ensure that the application continues to function smoothly without interruptions. This practice is particularly useful in scenarios involving calculations or data processing, where a missing value could lead to erroneous results.
In summary, managing the retrieval of double values
Author Profile
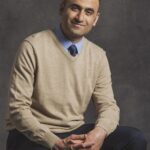
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?