Why Am I Getting the Error: ‘Java Package Does Not Exist’?
In the world of Java programming, encountering errors is an inevitable part of the development journey. One of the most common yet perplexing issues that developers face is the dreaded message: “package does not exist.” This seemingly simple error can halt progress and lead to frustration, especially for those new to the language. Understanding the root causes of this error is essential for any Java developer, as it not only impacts productivity but also hinders the learning process. In this article, we will delve into the intricacies of this error, exploring its common triggers and offering insights on how to resolve it effectively.
The “package does not exist” error typically arises when the Java compiler cannot locate the specified package in your project. This can stem from a variety of factors, including incorrect file paths, missing libraries, or even issues with your IDE configuration. As Java developers, we rely heavily on packages to organize our code and utilize external libraries, making it crucial to understand how package management works within the Java ecosystem.
Moreover, this error serves as a valuable learning opportunity, prompting developers to refine their understanding of Java’s structure and dependencies. By addressing the underlying issues that lead to this error, you can enhance your coding practices and ensure smoother project workflows. Join us as we unpack the nuances
Understanding Package Visibility
In Java, packages are used to group related classes and interfaces. When you encounter the error message indicating that a package does not exist, it often stems from visibility and accessibility issues. This can happen for various reasons, including:
- The package is not correctly imported.
- The package is not part of the classpath.
- The package name is misspelled.
To resolve these issues, ensure that the package you are trying to access is correctly declared and imported in your Java file. For example, if you are trying to use classes from the `java.util` package, your code should include:
“`java
import java.util.*;
“`
Classpath Configuration
The classpath is a parameter that tells the Java Virtual Machine (JVM) and Java compiler where to find the user-defined classes and packages. If the package is not found, it may not be included in the classpath. You can configure the classpath in several ways:
- Command Line: Use the `-cp` or `-classpath` option when compiling and running Java applications.
- Environment Variable: Set the `CLASSPATH` environment variable to include the paths to your packages.
- IDE Settings: Most Integrated Development Environments (IDEs) allow you to set the classpath in project settings.
Here’s how to set the classpath via the command line:
“`bash
javac -cp /path/to/package YourClass.java
java -cp /path/to/package YourClass
“`
Common Causes of the Error
Understanding the common causes can help in troubleshooting:
Cause | Description |
---|---|
Misspelled Package Name | Check for typos in the package name in your import statement. |
Missing JAR Files | Ensure that all required JAR files containing the package are included in the classpath. |
Incorrect Directory Structure | Java packages must reflect the directory structure; ensure directories match the package name. |
Version Mismatch | Verify that you are using compatible versions of libraries that include the package. |
Debugging Steps
When debugging this issue, follow these steps:
- Verify Package Declaration: Check if the package is declared correctly in the source files.
- Check Imports: Ensure that the relevant classes are imported properly.
- Inspect Classpath: Confirm that the classpath is configured correctly for your application.
- Review IDE Configuration: If using an IDE, check the project settings for any misconfigurations.
- Compile and Run: Use the command line to compile and run your Java files to see if the issue persists outside the IDE.
By following these guidelines, you can effectively troubleshoot and resolve the “java package does not exist” issue, allowing for smoother development in your Java projects.
Common Causes of “Java Package Does Not Exist”
The error message “Java package does not exist” can arise due to several reasons, which typically involve issues with the project structure, dependencies, or classpath settings. Understanding these causes is crucial for troubleshooting effectively.
- Incorrect Package Name: Ensure that the package name in your Java file matches the directory structure. For example, if your package is declared as `package com.example;`, the file should be in the `com/example` directory.
- Missing Dependencies: If your project relies on external libraries, make sure that all dependencies are included in your build configuration. For Maven projects, check the `pom.xml` file; for Gradle projects, review the `build.gradle` file.
- Classpath Issues: The classpath must include all the necessary libraries and directories where your packages are located. If you’re using an IDE, ensure that the project configuration includes the correct source folders.
- Build Path Configuration: In IDEs like Eclipse or IntelliJ IDEA, verify that the build path is correctly set. Missing or misconfigured source folders can lead to this error.
- File Naming Conventions: Java requires that the filenames match the public class names declared within them. If there’s a mismatch, the compiler may not find the package.
Troubleshooting Steps
To resolve the “Java package does not exist” error, follow these troubleshooting steps:
- Check Package Declaration: Verify that your Java source file begins with the correct package declaration.
- Inspect Directory Structure: Confirm that the physical directory structure aligns with the declared package.
- Review Build Configuration: Examine `pom.xml` or `build.gradle` for any missing dependencies.
- Update IDE Settings: In your IDE, check the project settings to ensure all source directories are included in the build path.
- Rebuild Project: Sometimes, simply rebuilding the project can resolve issues caused by stale caches.
Example Scenarios
Understanding specific scenarios can aid in diagnosing the problem effectively. Below are a few examples:
Scenario | Description |
---|---|
Missing JAR Files | An external library is not included in the classpath, leading to unresolved packages. |
Incorrect Module Configuration | In multi-module projects, ensure that the module dependencies are correctly defined. |
Source Folder Exclusion | A source folder may be excluded from the build process, causing the package not to compile. |
Tools and Best Practices
Utilizing the right tools and adhering to best practices can prevent package-related errors.
- IDE Features: Use your IDE’s features like “Organize Imports” and “Fix Imports” to manage package imports automatically.
- Build Tools: Utilize Maven or Gradle for dependency management, ensuring that all required libraries are included and updated.
- Version Control: Maintain a consistent project structure in version control systems to avoid discrepancies.
- Documentation: Regularly consult documentation for the libraries and frameworks in use to ensure compatibility and proper setup.
By addressing the common causes, following troubleshooting steps, and implementing best practices, developers can effectively resolve the “Java package does not exist” error and maintain a smooth development workflow.
Understanding the “Java Package Does Not Exist” Error
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “The ‘java package does not exist’ error typically arises due to incorrect package declarations or classpath issues. It is crucial to ensure that the package structure in your project matches the directory structure on the file system.”
James Liu (Software Architect, CodeCraft Solutions). “This error can also occur when the required libraries are not included in the build path. Always verify that all dependencies are correctly configured in your IDE or build tool.”
Sarah Thompson (Java Programming Instructor, Online Learning Academy). “For beginners, this error can be confusing. I recommend checking the spelling of the package name and ensuring that the Java files are compiled properly. A clean build often resolves such issues.”
Frequently Asked Questions (FAQs)
What does it mean when a Java package does not exist?
When a Java package does not exist, it indicates that the Java compiler cannot find the specified package in the classpath. This can occur due to incorrect package naming, missing dependencies, or improper directory structure.
How can I resolve the “package does not exist” error in Java?
To resolve this error, ensure that the package name is correctly spelled, verify that the required libraries are included in the classpath, and check that the directory structure matches the package declaration in your Java files.
What should I check if I encounter this error in an IDE?
In an IDE, check the project settings to ensure that the libraries are correctly added to the build path. Additionally, verify that the source folders are configured properly and that there are no typos in the package declaration.
Can this error occur due to missing JAR files?
Yes, this error can occur if the required JAR files are not included in the classpath. Ensure that all necessary JAR files are added to your project dependencies.
Is it possible for this error to be caused by incorrect Java version?
Yes, using an incompatible Java version can lead to this error. Ensure that the Java version used for compiling matches the version specified in your project settings and dependencies.
How can I check the classpath in a Java project?
You can check the classpath in a Java project by inspecting the build configuration in your IDE, or by using the command line with the `java -cp` or `javac -cp` commands to see the current classpath settings.
The error message “java package does not exist” typically indicates that the Java compiler cannot find a specified package in the classpath. This issue often arises due to several common factors, including incorrect package naming, missing dependencies, or improperly configured build paths. Ensuring that the package is correctly defined and that all necessary libraries are included in the project setup is crucial for resolving this error.
Another significant aspect to consider is the organization of the project structure. Java packages must align with the directory structure of the project. If the directory structure does not match the package declaration in the source files, the compiler will not be able to locate the package. Additionally, using an Integrated Development Environment (IDE) can simplify package management and help avoid such errors by providing tools for managing dependencies and project configurations.
Moreover, it is essential to verify that the Java Development Kit (JDK) version being used is compatible with the libraries and packages required by the project. Mismatched versions can lead to further complications, including the inability to locate certain packages. Regularly updating the JDK and libraries can help mitigate these issues and ensure smoother project development.
the “java package does not exist” error can be effectively addressed by carefully checking
Author Profile
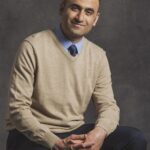
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?