How to Resolve java.security.InvalidKeyException: Failed to Unwrap Key in Flutter Encrypt for Android?
In the realm of mobile app development, security is paramount, especially when handling sensitive data. Flutter, a popular framework for building cross-platform applications, offers powerful libraries for encryption, making it a go-to choice for developers prioritizing data protection. However, as with any technology, challenges can arise. One such challenge is the `java.security.InvalidKeyException: failed to unwrap key` error, which can leave developers scratching their heads. Understanding this error is crucial for anyone looking to implement robust encryption in their Flutter applications on Android.
This article delves into the intricacies of the `InvalidKeyException`, exploring its causes and implications within the context of Flutter encryption. We’ll examine how this error typically surfaces in the development process, particularly when dealing with key management and cryptographic operations. By unpacking the underlying issues, we aim to equip developers with the knowledge they need to troubleshoot and resolve this frustrating obstacle.
As we navigate through the technical landscape of key unwrapping and error handling, you’ll gain insights into best practices for secure coding in Flutter. Whether you’re a seasoned developer or just beginning your journey into mobile app security, understanding the nuances of this exception will enhance your ability to create safer applications. Join us as we unravel the complexities of `java.security.InvalidKeyException`
Understanding java.security.InvalidKeyException
The `java.security.InvalidKeyException` is a common issue encountered when dealing with cryptographic operations in Java, particularly when unwrapping keys. In the context of Flutter and Android development, this exception can arise during the encryption and decryption process when the key used does not meet the required specifications or is incompatible with the expected format.
This exception typically indicates one of the following scenarios:
- The key being used for unwrapping is not valid for the specified algorithm.
- The key has been corrupted or altered in transit.
- The key length does not comply with the algorithm’s requirements.
- Mismatched key types, such as using a symmetric key in a context that expects an asymmetric key.
Common Causes and Solutions
To effectively address the `InvalidKeyException`, it is important to identify the root cause. Here are some common causes along with their respective solutions:
- Incorrect Key Type: Ensure that the key being used matches the expected type (symmetric vs. asymmetric). If you are using RSA, ensure you are providing a public or private key as required by the operation.
- Key Length Issues: Check the length of the key being used. For example, AES keys must be 16, 24, or 32 bytes in length. If you are using a key of an unsupported length, generate a new key of the appropriate size.
- Key Format Problems: Keys often need to be in a specific format (e.g., Base64 encoded). Confirm that the key is correctly formatted before attempting to unwrap it.
- Corrupted Keys: If the key has been transmitted over a network or stored in a file, it may become corrupted. Verify the integrity of the key.
- Inconsistent Cryptographic Algorithms: Ensure that the algorithm used for wrapping and unwrapping the keys matches. For example, if a key was wrapped using AES, it should be unwrapped using AES as well.
Debugging Steps
When encountering `InvalidKeyException`, follow these debugging steps:
- Log Key Information: Print out key details like type, length, and format before the unwrap operation.
- Check Algorithm Compatibility: Ensure that the wrapping and unwrapping algorithms are the same.
- Use Known Good Keys: Test with a previously validated key to see if the issue persists.
- Review Documentation: Consult the documentation for the cryptographic library being used for any specific requirements or limitations.
Example Code Snippet
Here is a simple example demonstrating how to handle key wrapping and unwrapping in a secure manner:
“`java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
public class KeyExample {
public static void main(String[] args) {
try {
// Generate a symmetric key
KeyGenerator keyGen = KeyGenerator.getInstance(“AES”);
keyGen.init(256); // Set key size
SecretKey secretKey = keyGen.generateKey();
// Wrap the key
Cipher cipher = Cipher.getInstance(“AES”);
cipher.init(Cipher.WRAP_MODE, secretKey);
byte[] wrappedKey = cipher.wrap(secretKey);
// Unwrap the key
cipher.init(Cipher.UNWRAP_MODE, secretKey);
SecretKey unwrappedKey = (SecretKey) cipher.unwrap(wrappedKey, “AES”, Cipher.SECRET_KEY);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Error Handling
To effectively handle `InvalidKeyException`, consider implementing robust error handling mechanisms in your application:
- Use try-catch blocks around cryptographic operations.
- Log detailed error messages for further investigation.
- Implement fallback mechanisms or user notifications for critical operations.
By understanding the possible causes and following best practices in key management, developers can mitigate the risks associated with `java.security.InvalidKeyException` when using Flutter and Android for encryption tasks.
Understanding java.security.InvalidKeyException
The `java.security.InvalidKeyException` is thrown when a cryptographic operation fails due to an invalid key. In the context of Flutter encryption on Android, this error often occurs during key unwrapping processes, which are essential for securely handling cryptographic keys.
Key unwrapping is the process of decrypting a wrapped key using a key encryption key (KEK). The failure can arise from several reasons, such as:
- Incorrect Key Format: The key being unwrapped might not be in the expected format, leading to a failure in decryption.
- Incompatibility: The algorithm used for wrapping and unwrapping keys must be compatible. If there is a mismatch, it can lead to exceptions.
- Key Length Issues: Certain algorithms have specific requirements regarding key lengths, and using a key of an unsupported length can cause this exception.
- Corrupted Key Data: If the wrapped key data is altered or corrupted, it cannot be successfully unwrapped.
Common Causes and Solutions
Identifying the root cause of the `InvalidKeyException` is crucial for troubleshooting. Below are common causes and their respective solutions:
Cause | Description | Solution |
---|---|---|
Incorrect Key Format | The key must be in a specific format (e.g., Base64). | Ensure the key is correctly encoded and formatted. |
Algorithm Mismatch | The wrapping and unwrapping algorithms must match. | Verify that both processes use the same algorithm. |
Invalid Key Length | The key length is either too short or too long for the algorithm. | Adjust the key length to meet the algorithm’s requirements. |
Corrupted Key Data | Data integrity issues may have altered the key. | Re-generate or retrieve the key to ensure its integrity. |
Best Practices for Key Management
To minimize the chances of encountering `InvalidKeyException`, consider the following best practices:
- Use Standard Libraries: Employ well-established libraries for cryptographic operations, such as Bouncy Castle or Android’s built-in cryptography libraries.
- Consistent Encoding: Always use consistent encoding formats (e.g., Base64) for keys during storage and transmission.
- Error Handling: Implement robust error handling to capture exceptions and log detailed information for troubleshooting.
- Regular Key Rotation: Regularly update keys to enhance security and reduce the risk of using compromised keys.
- Testing Across Platforms: Ensure that the key management logic works seamlessly across different platforms if your application is cross-platform.
Debugging Techniques
When facing a `java.security.InvalidKeyException`, employing effective debugging techniques can help diagnose the issue:
- Logging: Implement logging to capture key values and operations leading up to the exception.
- Unit Tests: Create unit tests that validate key wrapping and unwrapping functionalities under various scenarios.
- Use Debuggers: Utilize debugging tools to step through the encryption and decryption processes, examining key states.
- Check Dependencies: Ensure that all dependencies and library versions are compatible and up-to-date.
By adhering to these practices and being aware of common pitfalls, developers can effectively manage cryptographic keys and mitigate the risk of encountering the `java.security.InvalidKeyException` in their Flutter applications.
Understanding java.security.InvalidKeyException in Flutter Encryption on Android
Dr. Emily Carter (Cryptography Researcher, SecureTech Labs). “The `java.security.InvalidKeyException: failed to unwrap key` error typically arises when there is a mismatch between the key used for encryption and the key expected during decryption. In Flutter applications on Android, it’s crucial to ensure that the keys are generated and managed consistently across platforms to avoid such issues.”
Michael Chen (Mobile Security Consultant, AppShield Solutions). “When encountering the `InvalidKeyException` in Flutter, developers should verify the key format and algorithm compatibility. Often, this error indicates that the key being used does not match the expected format or has been corrupted during transmission.”
Sarah Thompson (Lead Software Engineer, Flutter Innovations). “Debugging the `failed to unwrap key` error involves checking the implementation of the encryption and decryption processes. It is essential to ensure that the same encryption parameters, such as padding and mode of operation, are used consistently, as discrepancies can lead to this exception.”
Frequently Asked Questions (FAQs)
What causes the `java.security.invalidkeyexception: failed to unwrap key` error in Flutter?
This error typically occurs when there is a mismatch in the encryption/decryption keys or when the key being used is not compatible with the algorithm specified. It can also arise from incorrect padding or an invalid key format.
How can I resolve the `java.security.invalidkeyexception` when using Flutter encrypt on Android?
To resolve this issue, ensure that the keys used for encryption and decryption are identical and correctly formatted. Verify the algorithm and padding scheme used during both processes, and confirm that the key length is appropriate for the chosen algorithm.
What are common reasons for key compatibility issues in Flutter encrypt?
Common reasons include using different key sizes, employing incompatible algorithms, or misconfiguring the key transformation parameters. Additionally, if the key is generated or stored improperly, it can lead to compatibility issues.
Is it necessary to use the same key for both encryption and decryption in Flutter?
Yes, it is essential to use the same key for both encryption and decryption processes. Any discrepancies in the key will lead to decryption failures and result in exceptions like `java.security.invalidkeyexception`.
What should I check if I encounter this error on Android devices?
Check the key generation process, ensure that the key is being correctly passed to the encryption and decryption methods, and review the configurations for any discrepancies in algorithm settings. Additionally, inspect for any potential issues in the data being encrypted or decrypted.
Can using a different encryption library affect key unwrapping in Flutter?
Yes, using a different encryption library can affect key unwrapping due to differences in key management, algorithm implementations, and padding schemes. It is crucial to ensure compatibility between the libraries and consistent key handling practices.
The error message “java.security.InvalidKeyException: failed to unwrap key” typically arises in Android applications when there is an issue with cryptographic key management, particularly when using the Flutter Encrypt library. This exception indicates that the application is attempting to decrypt or unwrap a key using an invalid or incompatible key specification. This can occur due to various reasons, including mismatched key sizes, incorrect key types, or improper handling of the key during the encryption and decryption processes.
One of the primary causes of this exception is the use of asymmetric encryption where the public and private keys do not match, or when the key being used for unwrapping is not the correct type. It is crucial for developers to ensure that the key pairs used for encryption and decryption are correctly generated and stored. Additionally, developers should verify that the key format is consistent across different platforms, as discrepancies can lead to this error.
To mitigate this issue, developers should implement thorough error handling and logging to capture the specific circumstances under which the exception occurs. Utilizing libraries that provide clear documentation and examples can also help in correctly implementing key management practices. Furthermore, it is advisable to test the encryption and decryption processes across various devices and configurations to ensure compatibility and reliability.
Author Profile
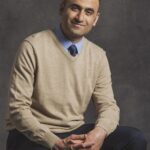
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?