How to Resolve ‘java.sql.SQLException: Illegal Mix of Collations for Operation’ in Your Database Queries?
In the world of database management, the seamless interaction between Java applications and SQL databases is crucial for maintaining data integrity and ensuring smooth operations. However, developers often encounter a perplexing error: `java.sql.SQLException: illegal mix of collations for operation`. This issue can disrupt workflows and lead to significant debugging time, particularly when dealing with multiple character sets and collations. Understanding the root causes and implications of this error is essential for any developer aiming to create robust, error-free applications.
When working with databases, collation refers to the set of rules that determine how string comparison is performed. Different collations can lead to unexpected behavior during SQL operations, especially when combining data from various sources or when performing queries that involve string comparisons. The `illegal mix of collations` error typically arises when the database engine encounters strings with incompatible collations, making it impossible to execute the intended operation. This situation can be particularly common in multilingual applications or when integrating legacy systems with modern databases.
Navigating this error requires a solid understanding of both Java and SQL, as well as the underlying principles of collation and character encoding. By delving into the specifics of how collations work, the potential pitfalls developers face, and the strategies for resolving these issues, we can empower developers to tackle
Understanding Collation in SQL
Collation in SQL refers to a set of rules that determine how data is sorted and compared. It affects string comparison operations, including those in WHERE clauses, ORDER BY clauses, and more. When collations mismatch, especially during comparisons, SQL engines may throw exceptions, such as `java.sql.SQLException: illegal mix of collations for operation`.
Key aspects of collation include:
- Character Set: Defines the set of characters that can be stored.
- Sorting Rules: Specifies how string comparison should occur (e.g., case sensitivity, accent sensitivity).
- Locale: Affects the sorting order based on language or regional preferences.
Understanding these components is crucial for database design and querying.
Common Causes of Collation Issues
Collation issues often arise from:
- Mixed Collation Settings: When tables or columns use different collations.
- String Literals: If a string literal has a different collation than the column it is being compared to.
- Database Configuration: The default collation of the database can differ from that of individual tables or columns.
A typical scenario leading to collation issues is when joining tables with different collation settings, which may result in an exception.
Resolving Collation Conflicts
To resolve collation conflicts, you can take several approaches:
- Explicit Collation Specification: Use the `COLLATE` keyword in your SQL queries to specify the collation for a particular column or string.
- Uniform Collation Setting: Ensure all relevant tables and columns share the same collation by altering them if necessary.
- Database Configuration: Set a consistent default collation for the entire database to minimize conflicts.
Example of explicit collation specification:
“`sql
SELECT * FROM table1
JOIN table2
ON table1.name COLLATE utf8_general_ci = table2.name COLLATE utf8_general_ci;
“`
Best Practices for Managing Collation
To prevent collation issues from arising, consider the following best practices:
- Standardization: Establish a standard collation for your database schema and adhere to it consistently across all tables and columns.
- Documentation: Keep thorough documentation of the collations used throughout your database to aid in troubleshooting.
- Regular Audits: Periodically review your database schema for collation mismatches to ensure ongoing compliance.
Action | Description |
---|---|
Check Collation | Review the collation settings of tables and columns regularly. |
Use COLLATE | Specify collation explicitly in queries to avoid conflicts. |
Alter Collation | Change the collation of a table or column to match others if needed. |
By adhering to these practices, you can significantly reduce the risk of encountering collation-related exceptions in your SQL operations.
Understanding Collation in SQL
Collation in SQL refers to the set of rules that determine how string comparison is performed. It affects sorting, case sensitivity, and accent sensitivity. Different collations can lead to discrepancies when combining or comparing data from various sources.
Key aspects of collation include:
- Character Set: The collection of characters that can be used.
- Sorting Rules: How characters are ordered in queries.
- Case Sensitivity: Whether ‘A’ and ‘a’ are treated as the same character.
- Accent Sensitivity: Whether characters with accents are treated as different from their non-accented counterparts.
Causes of the ‘Illegal Mix of Collations’ Error
The `java.sql.SQLException: illegal mix of collations` error occurs primarily due to incompatible collation settings in the database. Common causes include:
- Different Collations in Queries: Attempting to compare or manipulate strings with different collations in a SQL statement.
- Database/Table/Column Collation Mismatch: When the collation of a column does not match the collation of the query or the other columns being compared.
- Default Collation Conflicts: The default collation of the database may differ from the collation specified in queries.
Resolving Collation Conflicts
To address the `illegal mix of collations` error, consider the following strategies:
- Identify Collation Settings: Use the following SQL queries to check the collation of your database, tables, and columns.
“`sql
SHOW VARIABLES LIKE ‘collation_database’;
SHOW TABLE STATUS WHERE Name = ‘your_table_name’;
SHOW FULL COLUMNS FROM your_table_name;
“`
- Modify Collation in Queries: Explicitly specify the collation in your SQL queries using the `COLLATE` clause. For example:
“`sql
SELECT * FROM your_table WHERE your_column COLLATE utf8mb4_general_ci = ‘value’;
“`
- Change Column Collation: If necessary, alter the column collation to match the desired collation for your queries:
“`sql
ALTER TABLE your_table MODIFY your_column VARCHAR(255) COLLATE utf8mb4_general_ci;
“`
- Consistency Across Database: Ensure that all database elements (tables, columns, and even the database itself) use the same collation settings.
Best Practices for Managing Collations
Adopting best practices in managing collations can prevent errors and improve database performance:
- Use a Consistent Collation: Choose a collation for the entire database and stick to it for all tables and columns.
- Document Collation Choices: Maintain clear documentation of collation settings to facilitate troubleshooting.
- Test Queries: Always test new queries for collation conflicts in a development environment before deploying to production.
- Regularly Review Settings: Periodically review and update collation settings as needed, especially after upgrades or migrations.
While the `java.sql.SQLException: illegal mix of collations` error can be frustrating, understanding the underlying principles of collation and taking proactive measures can help mitigate such issues. By ensuring consistency and clarity in collation settings, developers can create a more robust and error-resistant database environment.
Understanding SQL Collation Issues in Java Applications
Dr. Emily Carter (Database Systems Architect, Tech Solutions Inc.). “The error ‘java.sql.sqlexception: illegal mix of collations for operation’ typically arises when there are conflicting character sets or collations in SQL queries. It is crucial to ensure that all string comparisons and operations in your database use the same collation to avoid such issues.”
Michael Chen (Senior Java Developer, CodeCraft Ltd.). “In my experience, this error often surfaces when joining tables or performing operations on string data types with differing collations. To resolve this, one should explicitly define the collation in the SQL query or modify the database schema to maintain consistency across tables.”
Sarah Patel (Lead Database Administrator, DataGuard Solutions). “When encountering an ‘illegal mix of collations’ error, it is essential to review the database settings and application configurations. Ensuring that the database connection string specifies the correct character encoding can prevent these types of collation conflicts.”
Frequently Asked Questions (FAQs)
What does the error ‘java.sql.SQLException: illegal mix of collations’ indicate?
This error indicates that there is a conflict between different character set collations in your SQL query. It typically occurs when comparing or combining strings from different collation settings in a database.
How can I identify the collations of my database columns?
You can identify the collations of your database columns by executing a query that retrieves the collation information. For example, in MySQL, you can use `SHOW FULL COLUMNS FROM table_name;` to display the collation for each column in the specified table.
What steps can I take to resolve this error?
To resolve this error, ensure that all string literals and columns involved in the operation use the same collation. You can either change the collation of the columns or explicitly convert the collations in your query using the `COLLATE` clause.
Can this error occur in JOIN operations?
Yes, this error can occur in JOIN operations when the columns being joined have different collations. It is essential to ensure that the collations match to avoid this issue.
Is it possible to set a default collation for my database?
Yes, you can set a default collation for your database at the time of its creation or alter it later using the `ALTER DATABASE` command. However, existing tables and columns will need to be updated individually to reflect the new default collation.
What are the implications of changing a column’s collation?
Changing a column’s collation can affect how data is sorted and compared. It may also impact existing queries, indexes, and application logic that rely on the original collation. Always ensure to back up your data before making such changes.
The error message “java.sql.SQLException: illegal mix of collations for operation ‘in'” typically arises in database operations when there is a conflict between different character set collations used in SQL queries. Collation refers to the set of rules that determine how string comparison is performed in a database, including how strings are sorted and compared for equality. When a query attempts to compare or operate on strings with different collations, the database engine cannot resolve the conflict, leading to this exception.
To address this issue, developers should ensure that all string literals and database columns involved in the operation use the same collation. This can be achieved by explicitly defining the collation for string literals in the SQL query or by altering the database schema to standardize the collation across relevant tables and columns. Additionally, it is advisable to review the database configuration settings to ensure that the default collation aligns with the intended use cases of the application.
In summary, the “illegal mix of collations” error serves as a reminder of the importance of consistency in character set collation within SQL queries. Understanding and managing collations effectively can prevent runtime errors and ensure smoother database interactions. Developers should prioritize collation compatibility during the design phase of database schemas and when writing SQL
Author Profile
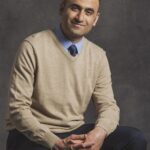
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?