How Can Java Implement SAML Protocol to Access Salesforce APIs?
In today’s interconnected digital landscape, securing access to cloud services while ensuring seamless integration is paramount for businesses. As organizations increasingly rely on platforms like Salesforce for their customer relationship management (CRM) needs, the demand for robust authentication mechanisms has surged. One such mechanism is the Security Assertion Markup Language (SAML) protocol, which offers a standardized way to authenticate users across different systems. For Java developers looking to harness the power of Salesforce APIs, understanding how to implement SAML can unlock new levels of security and efficiency.
SAML serves as a bridge between identity providers and service providers, allowing users to authenticate once and gain access to multiple applications without the need for repeated logins. This single sign-on (SSO) capability is particularly beneficial in enterprise environments where users interact with various systems throughout the day. When integrating Salesforce APIs, leveraging SAML not only enhances security but also improves user experience by streamlining the authentication process.
In this article, we will explore how Java developers can effectively implement SAML to consume Salesforce APIs. We will delve into the fundamental concepts of SAML, its architecture, and the steps necessary to establish a secure connection between your Java application and Salesforce. By the end of this guide, you will have a clearer understanding of how to utilize SAML to enhance your application’s
Understanding SAML in Salesforce API Integration
Security Assertion Markup Language (SAML) is an XML-based framework that allows for the exchange of authentication and authorization data between parties, particularly between an identity provider (IdP) and a service provider (SP). In the context of Salesforce, SAML can be used to authenticate users accessing Salesforce APIs.
When integrating Java applications with Salesforce APIs via SAML, it is essential to configure both the Salesforce environment and the Java application to handle SAML assertions properly. The process generally involves the following key steps:
- Setting up Salesforce for SAML:
- Enable SAML in your Salesforce organization.
- Create a new SAML Single Sign-On (SSO) configuration in Salesforce.
- Obtain the necessary metadata from Salesforce, including the SSO URL and the certificate for signing assertions.
- Configuring your Java application:
- Use a library like OpenSAML or Spring Security SAML to handle SAML assertions in your Java application.
- Implement the logic for generating SAML requests and processing SAML responses.
Creating a SAML Request in Java
To initiate a SAML authentication request from a Java application, you will typically create a SAML request object, sign it, and redirect the user to the Salesforce SSO URL. Below is an example of how this can be structured:
“`java
import org.opensaml.saml.saml2.core.AuthnRequest;
// Additional imports…
public class SAMLRequestGenerator {
public AuthnRequest createSAMLRequest() {
// Implementation to create and configure the SAML request
}
}
“`
You will need to ensure that the request is properly signed using the private key associated with your certificate. The signed request is then sent to the Salesforce endpoint.
Handling SAML Responses
Upon successful authentication, Salesforce redirects the user back to your Java application with a SAML response. Your application needs to process this response, validate its authenticity, and extract user information such as their Salesforce user ID.
Key points for handling SAML responses include:
- Validating the SAML Response:
- Ensure the response is signed by the expected IdP.
- Check the validity period of the assertion.
- Extracting User Information:
- Retrieve user attributes from the SAML response to establish a session within your application.
Example of a SAML Response Structure
Below is a simplified example of a SAML response:
Element | Description |
---|---|
Assertion | The main element that contains authentication statements. |
Subject | The user being authenticated. |
Conditions | Defines the validity period of the assertion. |
Signature | Used to verify the authenticity of the assertion. |
This structure is critical for your application to correctly interpret and process the response received from Salesforce.
Best Practices for SAML Integration
When integrating SAML for Salesforce API access, consider the following best practices:
- Always use HTTPS for secure transmission of SAML assertions.
- Regularly update and rotate your signing certificates to maintain security.
- Implement logging and monitoring to detect and respond to authentication issues.
- Validate all incoming SAML assertions against your IdP’s public key.
Following these guidelines will help ensure a secure and efficient integration between your Java application and Salesforce APIs using the SAML protocol.
Understanding SAML Protocol in Java
SAML (Security Assertion Markup Language) is an XML-based framework that allows for secure single sign-on (SSO) across different domains. When integrating with Salesforce APIs, using SAML can streamline authentication processes. Here are the key components involved in utilizing SAML with Java applications:
- Identity Provider (IdP): The system that authenticates users and provides SAML assertions.
- Service Provider (SP): The application (e.g., your Java application) that consumes the SAML assertions to grant access.
- SAML Assertion: A package of information that the IdP sends to the SP, containing the user’s authentication and authorization details.
Java Libraries for SAML Implementation
To implement SAML in Java, several libraries are available that simplify the process:
- OpenSAML: A robust library for creating and processing SAML messages.
- Spring Security SAML: An extension of Spring Security that integrates SAML authentication.
- Keycloak: An open-source identity and access management solution that supports SAML.
Library | Description | Use Case |
---|---|---|
OpenSAML | Comprehensive SAML processing library | Custom SAML implementations |
Spring Security SAML | Easy integration with Spring applications | Applications using Spring framework |
Keycloak | Identity management with SAML support | Full-featured identity solutions |
Configuring SAML Authentication with Salesforce
To configure SAML for Salesforce, you need to set up your Identity Provider properly. The following steps outline the process:
- Configure Salesforce as a Service Provider:
- Navigate to the Salesforce Setup menu.
- Search for “Single Sign-On Settings.”
- Click “New” to create a new SAML configuration.
- Set Up the Identity Provider:
- Obtain the SAML metadata from your Java application.
- Provide the required information, including the Entity ID, Assertion Consumer Service (ACS) URL, and certificate details.
- Create a SAML Assertion:
- In your Java application, generate a SAML assertion after user authentication.
- Ensure the assertion includes relevant user attributes and is signed properly.
- Testing the Configuration:
- Use a SAML testing tool to simulate assertions.
- Verify that users can log in to Salesforce through your Java application seamlessly.
Implementing SAML in Java Code
Here’s a brief overview of how to implement SAML authentication using OpenSAML in a Java application:
- Initialize OpenSAML:
“`java
static {
try {
InitializationService.initialize();
} catch (InitializationException e) {
e.printStackTrace();
}
}
“`
- Create a SAML Request:
“`java
AuthnRequest authnRequest = (AuthnRequest) buildSAMLObject(AuthnRequest.class);
// Set parameters such as ID, version, issuer, etc.
“`
- Send the Request to the IdP:
“`java
String redirectUrl = getRedirectUrl(authnRequest);
response.sendRedirect(redirectUrl);
“`
- Process the SAML Response:
“`java
SAMLResponse samlResponse = parseSAMLResponse(request.getParameter(“SAMLResponse”));
// Validate the response and extract user information.
“`
Handling SAML Responses
When your application receives a SAML response, it must be parsed and validated. Key steps include:
- Base64 Decode the SAML response.
- XML Parsing to extract assertion details.
- Signature Validation to ensure the response’s integrity.
- User Attribute Retrieval for user session creation.
This structured approach to handling SAML responses ensures secure and reliable authentication for users accessing Salesforce APIs through Java applications.
Expert Insights on Using SAML Protocol with Salesforce APIs in Java
Dr. Emily Carter (Lead Security Architect, TechSecure Solutions). “Implementing SAML for authentication when consuming Salesforce APIs in Java is crucial for maintaining a secure environment. It simplifies user management and enhances security by allowing single sign-on capabilities, which significantly reduces the risk of credential theft.”
Michael Chen (Senior Software Engineer, Cloud Integration Services). “When integrating Java applications with Salesforce APIs using SAML, it is essential to ensure that your SAML assertions are correctly configured. This includes setting up the correct audience and issuer attributes to avoid common pitfalls that can lead to authentication failures.”
Laura Smith (Principal Consultant, Enterprise Solutions Group). “The choice of libraries in Java for handling SAML can greatly affect the integration process with Salesforce APIs. Libraries like OpenSAML or Spring Security SAML provide robust solutions, but developers must be familiar with their configurations to leverage their full potential effectively.”
Frequently Asked Questions (FAQs)
What is SAML and how does it relate to Salesforce APIs?
SAML (Security Assertion Markup Language) is an open standard for exchanging authentication and authorization data between parties, particularly between an identity provider and a service provider. In the context of Salesforce APIs, SAML can be used to enable Single Sign-On (SSO), allowing users to authenticate once and access Salesforce services securely.
How can I implement SAML authentication in a Java application to access Salesforce APIs?
To implement SAML authentication in a Java application, you need to configure your application as a SAML service provider. This involves setting up a SAML library (such as OpenSAML or Spring Security SAML), creating a SAML request to send to the identity provider, and handling the SAML response to obtain user credentials for accessing Salesforce APIs.
What libraries are recommended for Java applications to use SAML with Salesforce?
Popular libraries for implementing SAML in Java applications include OpenSAML, Spring Security SAML, and pac4j. These libraries provide comprehensive support for SAML protocols and simplify the integration process with Salesforce APIs.
What are the prerequisites for using SAML with Salesforce APIs?
Prerequisites include a Salesforce account with API access, a configured identity provider that supports SAML, and a Java development environment with the necessary SAML libraries. Additionally, you must have a basic understanding of SAML concepts and Java programming.
What are the common challenges when using SAML with Salesforce APIs?
Common challenges include configuring the identity provider and service provider correctly, handling SAML assertions and responses, and ensuring proper security measures are in place. Debugging SAML-related issues can also be complex due to the nature of XML and the various configurations involved.
Is there any documentation available for implementing SAML with Salesforce APIs?
Yes, Salesforce provides comprehensive documentation on implementing SAML for Single Sign-On, which includes guidelines for setting up your identity provider, configuring Salesforce, and using SAML assertions. Additionally, the documentation for the chosen Java SAML library will offer insights on integration specifics.
In summary, integrating Java applications with Salesforce APIs using the Security Assertion Markup Language (SAML) protocol provides a robust framework for secure authentication and authorization. SAML facilitates Single Sign-On (SSO) capabilities, allowing users to access Salesforce services seamlessly without needing to manage multiple credentials. This approach enhances user experience while maintaining a high level of security, which is critical in enterprise environments.
Utilizing SAML with Java requires a solid understanding of both the SAML specifications and the Salesforce API architecture. Developers must implement SAML assertions and configure identity providers (IdPs) to communicate effectively with Salesforce as the service provider (SP). This integration not only streamlines authentication processes but also leverages existing security infrastructure, thereby reducing the overhead associated with user management.
Key takeaways from the discussion include the importance of proper configuration and adherence to security best practices when implementing SAML. Developers should ensure that their Java applications are capable of handling SAML requests and responses, and they must be familiar with libraries and frameworks that facilitate SAML integration. Additionally, thorough testing is essential to validate the SSO functionality and ensure that the integration meets organizational security requirements.
Author Profile
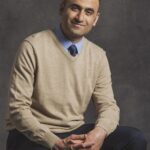
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?