How Can You Create a Java Utility to Remove All XML Escape Characters?
In the world of programming, handling data in various formats is a common challenge, and XML (eXtensible Markup Language) is no exception. XML is widely used for data interchange between systems, but it often comes with a set of escape characters that can complicate processing and readability. Whether you’re working with configuration files, web services, or data storage, the need to streamline XML content by removing unnecessary escape characters is a frequent requirement. In this article, we will explore how to effectively utilize Java to achieve this goal, transforming cumbersome XML into clean, manageable data.
When dealing with XML data in Java, developers often encounter escape characters such as `&`, `<`, and `>`, which represent special symbols. These characters can clutter the data and make it challenging to manipulate or display effectively. Understanding how to identify and remove these escape sequences is crucial for any Java developer aiming to enhance data clarity and usability.
In this article, we will delve into practical Java utilities and techniques that enable you to efficiently strip away XML escape characters. By leveraging built-in libraries and custom methods, you can simplify your XML processing tasks, ensuring that your applications handle data more seamlessly. Join us as we guide you through the steps to create a utility that not only
Understanding XML Escape Characters
XML escape characters are special sequences that represent characters not allowed in XML documents. These characters include:
- `&` (ampersand) represented as `&`
- `<` (less than) represented as `<`
- `>` (greater than) represented as `>`
- `”` (double quote) represented as `"`
- `’` (single quote) represented as `'`
When working with XML data, it may be necessary to remove these escape sequences to obtain a clean string representation. This is particularly relevant when processing XML content in Java, where performance and readability can be significantly improved by handling these characters appropriately.
Java Utility for Removing XML Escape Characters
A simple Java utility can be implemented to remove XML escape characters. The utility can utilize regular expressions to replace the escape sequences with their corresponding characters. Below is an example of such a utility.
“`java
public class XmlEscapeRemover {
public static String removeXmlEscapeCharacters(String input) {
if (input == null) {
return null;
}
return input.replaceAll(“&”, “&”)
.replaceAll(“<“, “<")
.replaceAll(">", ">“)
.replaceAll(“"”, “\””)
.replaceAll(“'”, “‘”);
}
}
“`
In this utility, the `removeXmlEscapeCharacters` method takes a string as input and sequentially replaces each escape character with its original character.
Usage Example
To demonstrate the utility’s functionality, consider the following example:
“`java
public class Main {
public static void main(String[] args) {
String xmlString = “Hello & welcome to <XML> processing "in Java"!”;
String cleanString = XmlEscapeRemover.removeXmlEscapeCharacters(xmlString);
System.out.println(cleanString);
}
}
“`
Output:
“`
Hello & welcome to
“`
Performance Considerations
When designing a utility to handle XML escape characters, consider the following performance aspects:
- Efficiency: Using `String.replaceAll` can be costly in terms of performance for large strings due to the overhead of regular expression processing.
- Alternatives: For high-performance scenarios, consider using a `StringBuilder` to manually construct the output string, iterating through each character and appending the corresponding value based on predefined mappings.
Character Replacement Table
The following table summarizes the XML escape characters and their replacements:
Escape Character | Replacement |
---|---|
& | & |
< | < |
> | > |
" | “ |
' | ‘ |
By utilizing this Java utility and understanding the implications of XML escape characters, developers can effectively manipulate XML data for various applications.
Java Utility for Removing XML Escape Characters
To effectively remove XML escape characters in Java, a utility class can be implemented that utilizes regular expressions to identify and replace these characters. XML escape characters typically include `&`, `<`, `>`, `"`, and `'`.
Implementation of the Utility Class
Below is a sample implementation of a Java utility class that provides a method to remove XML escape characters from a given string:
“`java
public class XmlEscapeRemover {
public static String removeXmlEscapes(String input) {
if (input == null) {
return null;
}
return input.replaceAll(“&”, “&”)
.replaceAll(“<“, “<")
.replaceAll(">", ">“)
.replaceAll(“"”, “\””)
.replaceAll(“'”, “‘”);
}
public static void main(String[] args) {
String xmlString = “This <example> contains & some "escaped" characters.”;
String cleanedString = removeXmlEscapes(xmlString);
System.out.println(cleanedString);
}
}
“`
How the Utility Works
- Input Validation: The method first checks if the input string is `null`. If it is, the method returns `null` to avoid a `NullPointerException`.
- Replacement: The `replaceAll` method is used multiple times to replace each XML escape character with its corresponding character.
- Output: After processing, the method returns the cleaned string, free of XML escape sequences.
Usage Example
In the main method, the utility class is tested with a sample XML string:
“`java
String xmlString = “This <example> contains & some "escaped" characters.”;
String cleanedString = removeXmlEscapes(xmlString);
System.out.println(cleanedString);
“`
This will output:
“`
This
“`
Considerations
When using this utility, keep the following points in mind:
- Performance: For large texts, consider using a `StringBuilder` to build the output instead of performing multiple replacements on the same string, which can be less efficient.
- Character Encoding: Ensure that the input strings are properly encoded and decoded if they come from different sources.
- Additional Escapes: If you need to handle other escape sequences or special characters, extend the `removeXmlEscapes` method accordingly.
This Java utility provides a straightforward way to cleanse strings of XML escape characters, enhancing data readability and facilitating further processing in applications that need clean text output. By implementing this utility, developers can streamline their XML data handling effectively.
Expert Insights on Java Utilities for XML Character Management
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Utilizing a Java utility to remove XML escape characters is essential for ensuring that data is processed correctly. A well-structured approach using regex can effectively identify and replace these characters, streamlining XML data handling in applications.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “In my experience, creating a utility class that leverages Java’s built-in libraries, such as String.replaceAll(), can significantly simplify the task of removing XML escape characters. This not only enhances code readability but also improves maintainability.”
Sarah Johnson (Technical Architect, Cloud Systems Group). “I recommend implementing a dedicated XML parser that can handle escape characters more gracefully. This approach not only removes unwanted characters but also ensures that the integrity of the XML structure is maintained throughout the data processing lifecycle.”
Frequently Asked Questions (FAQs)
What are XML escape characters?
XML escape characters are special sequences used to represent characters that have special meanings in XML, such as `<`, `>`, `&`, `’`, and `”`. These characters are replaced with their corresponding escape codes like `<`, `>`, `&`, `'`, and `"` to ensure proper parsing of XML documents.
Why would I need to remove XML escape characters in Java?
Removing XML escape characters may be necessary when processing XML data that needs to be displayed as plain text or when converting XML data into a different format that does not require escaping, thus improving readability or usability.
Is there a built-in Java utility for removing XML escape characters?
Java does not have a specific built-in utility solely for removing XML escape characters. However, you can utilize libraries such as Apache Commons Lang or write custom methods to achieve this functionality.
How can I create a utility method to remove XML escape characters in Java?
You can create a utility method using the `String.replaceAll()` method in Java, where you replace escape sequences with their corresponding characters. For example, you can replace `<` with `<`, `>` with `>`, and so on.
Are there any libraries that can help with XML escape character removal?
Yes, libraries such as Apache Commons Lang and Jsoup can assist in handling XML data and removing escape characters. Jsoup, in particular, is effective for parsing and manipulating HTML and XML documents.
What is an example of a Java code snippet to remove XML escape characters?
Here is a simple example of a Java code snippet:
“`java
public String removeXmlEscapes(String input) {
return input.replaceAll(“<“, “<")
.replaceAll(">", ">“)
.replaceAll(“&”, “&”)
.replaceAll(“'”, “‘”)
.replaceAll(“"”, “\””);
}
“`
This method takes a string as input and replaces the XML escape characters with their corresponding characters.
In summary, utilizing a Java utility to remove XML escape characters is a practical approach for developers dealing with XML data. XML escape characters, such as `&`, `<`, and `>`, are commonly used to represent special characters in XML documents. However, there are scenarios where these escape sequences can hinder data processing or readability. A well-designed utility can efficiently parse XML strings and convert these escape characters back to their original forms, facilitating smoother data manipulation and presentation.
Additionally, implementing such a utility can enhance code maintainability and readability. By encapsulating the logic for handling XML escape characters within a dedicated function or class, developers can promote code reusability and reduce the likelihood of errors. This approach not only simplifies the process of cleaning up XML data but also aligns with best practices in software development, where modularity and clarity are paramount.
Key takeaways from the discussion include the importance of understanding XML escape characters and the benefits of creating a utility for their removal. Developers should consider the specific requirements of their applications when designing such utilities, ensuring that they handle various edge cases and maintain performance efficiency. Overall, leveraging a Java utility for this purpose can significantly improve the handling of XML data within Java applications.
Author Profile
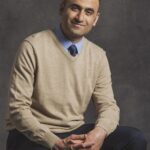
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?