How Can I Convert Snake Case to Camel Case in JSON Using Java?
In the world of programming, data interchange formats like JSON (JavaScript Object Notation) have become the backbone of modern web applications, enabling seamless communication between servers and clients. However, as developers often face varying naming conventions, converting data structures from one format to another can present unique challenges. One common scenario arises when working with JSON data that employs snake_case naming conventions, especially prevalent in languages like Python or Ruby, and needing to transform it into camelCase, which is favored in Java and JavaScript environments. This conversion is not just a matter of aesthetics; it can significantly impact code readability, maintainability, and integration.
Understanding how to convert snake_case to camelCase in JSON is essential for developers who want to ensure their applications are consistent and easy to work with. The process typically involves parsing the JSON data, identifying the keys that follow the snake_case format, and systematically transforming them into the camelCase format. This transformation not only enhances the compatibility of data structures across different programming languages but also aligns with best practices in coding standards.
As we delve deeper into this topic, we’ll explore various methods and tools available for performing this conversion, including manual coding techniques and leveraging libraries that automate the process. Whether you’re a seasoned developer or just starting, mastering the art of converting snake_case to
Understanding Snake Case and Camel Case
Snake case and camel case are two common conventions for naming variables, especially in programming and data representation. Snake case uses underscores to separate words, while camel case concatenates words and capitalizes the first letter of each subsequent word.
- Snake Case Example: `my_variable_name`
- Camel Case Example: `myVariableName`
In JSON data formats, it is often necessary to convert between these two styles to adhere to specific coding standards or to interact with APIs that expect a certain format.
Java Implementation for Conversion
To convert JSON data from snake case to camel case in Java, one can utilize libraries such as Jackson or Gson. Below is a basic approach using Jackson.
- Add Jackson Dependency: Ensure your project includes the Jackson library. If using Maven, add the following dependency:
“`xml
“`
- Creating a Utility Class: Implement a utility class for conversion.
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategies;
public class JsonConverter {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static String convertSnakeToCamel(String json) throws Exception {
objectMapper.setPropertyNamingStrategy(PropertyNamingStrategies.SNAKE_CASE);
Object jsonObject = objectMapper.readValue(json, Object.class);
objectMapper.setPropertyNamingStrategy(PropertyNamingStrategies.LOWER_CAMEL_CASE);
return objectMapper.writeValueAsString(jsonObject);
}
}
“`
- Usage Example: Here’s how you would use the utility to convert JSON.
“`java
public class Main {
public static void main(String[] args) throws Exception {
String snakeCaseJson = “{\”my_variable_name\”: \”value\”}”;
String camelCaseJson = JsonConverter.convertSnakeToCamel(snakeCaseJson);
System.out.println(camelCaseJson); // Output: {“myVariableName”:”value”}
}
}
“`
Considerations for JSON Conversion
When converting JSON from snake case to camel case, consider the following:
- Performance: Large JSON objects may require efficient handling to avoid performance bottlenecks.
- Nested Structures: Ensure that nested objects are also converted properly.
- Data Types: Be cautious of data type conversions during the mapping process.
Example Conversion Table
The following table illustrates the conversion of sample keys from snake case to camel case.
Snake Case | Camel Case |
---|---|
first_name | firstName |
last_name | lastName |
user_age | userAge |
account_balance | accountBalance |
By employing these methods and considerations, developers can effectively manage JSON data formats and ensure consistency across their applications.
Understanding Snake Case and Camel Case
Snake case and camel case are two common naming conventions in programming.
- Snake Case: This format uses lowercase letters and underscores to separate words. For example, `example_variable_name`.
- Camel Case: This format starts with a lowercase letter and capitalizes the first letter of each subsequent word without spaces. For example, `exampleVariableName`.
When working with JSON data, it is often necessary to convert between these two formats, particularly when different systems or libraries have established naming conventions.
Java Implementation for Conversion
In Java, you can convert JSON keys from snake case to camel case using libraries such as Jackson or Gson. Below are methods for both libraries.
Using Jackson
Jackson provides a straightforward way to handle this conversion through the `ObjectMapper` and a custom naming strategy.
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.PropertyNamingStrategies;
import com.fasterxml.jackson.databind.JsonNode;
public class SnakeToCamelConverter {
public static void main(String[] args) throws Exception {
String jsonInput = “{\”first_name\”:\”John\”, \”last_name\”:\”Doe\”}”;
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.setPropertyNamingStrategy(PropertyNamingStrategies.SNAKE_CASE);
JsonNode node = objectMapper.readTree(jsonInput);
String camelCaseJson = objectMapper.writeValueAsString(node);
System.out.println(camelCaseJson);
}
}
“`
Using Gson
For Gson, you need to implement a custom `JsonDeserializer` to convert snake case keys to camel case.
“`java
import com.google.gson.*;
import java.lang.reflect.Type;
public class SnakeToCamelConverter implements JsonDeserializer
@Override
public MyClass deserialize(JsonElement json, Type typeOfT, JsonDeserializationContext context) {
JsonObject jsonObject = json.getAsJsonObject();
String firstName = jsonObject.get(“first_name”).getAsString();
String lastName = jsonObject.get(“last_name”).getAsString();
return new MyClass(firstName, lastName);
}
public static void main(String[] args) {
String jsonInput = “{\”first_name\”:\”John\”, \”last_name\”:\”Doe\”}”;
GsonBuilder gsonBuilder = new GsonBuilder();
gsonBuilder.registerTypeAdapter(MyClass.class, new SnakeToCamelConverter());
Gson gson = gsonBuilder.create();
MyClass myClass = gson.fromJson(jsonInput, MyClass.class);
System.out.println(myClass);
}
}
class MyClass {
private String firstName;
private String lastName;
public MyClass(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
@Override
public String toString() {
return “MyClass{” + “firstName='” + firstName + ‘\” + “, lastName='” + lastName + ‘\” + ‘}’;
}
}
“`
Key Points to Consider
When converting snake case to camel case, keep in mind the following:
- Ensure all keys are consistently formatted to avoid conversion errors.
- Test the conversion process with various JSON structures to ensure robustness.
- Be aware of potential performance implications if dealing with large datasets.
Choosing the right method for conversion depends on the libraries you are using in your Java application. Both Jackson and Gson provide effective ways to handle the conversion, enabling seamless integration with different naming conventions in JSON data.
Transforming JSON Data: Expert Insights on Java Conversion Techniques
Jessica Lin (Senior Software Engineer, Tech Innovations Inc.). “Converting snake_case to camelCase in JSON using Java can be efficiently achieved by leveraging libraries such as Jackson or Gson. These libraries provide built-in functionality to handle property naming strategies, allowing developers to easily map JSON fields to Java object fields.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “When dealing with JSON data, it is crucial to ensure that the conversion from snake_case to camelCase is consistent across your application. Implementing a custom naming strategy in your JSON serialization/deserialization process can help maintain this consistency and improve code readability.”
Sarah Patel (Data Architect, FutureTech Analytics). “In scenarios where performance is a concern, developers should consider using a streaming approach for JSON processing in Java. This method allows for on-the-fly conversion of snake_case to camelCase, minimizing memory usage while handling large datasets.”
Frequently Asked Questions (FAQs)
What is the difference between snake_case and camelCase?
Snake_case uses underscores to separate words, while camelCase capitalizes the first letter of each subsequent word without spaces or underscores. For example, “snake_case_example” vs. “camelCaseExample”.
How can I convert snake_case to camelCase in Java?
You can convert snake_case to camelCase in Java by splitting the string at underscores, capitalizing the first letter of each word except the first, and then concatenating them back together.
Is there a library in Java that can help with this conversion?
Yes, libraries like Apache Commons Lang provide utilities for string manipulation, which can facilitate the conversion from snake_case to camelCase.
Can I convert JSON keys from snake_case to camelCase using Java?
Yes, you can parse the JSON, convert the keys from snake_case to camelCase, and then serialize it back to JSON format using libraries like Jackson or Gson.
What is an example of converting a JSON object with snake_case keys to camelCase?
For instance, a JSON object like `{“first_name”: “John”, “last_name”: “Doe”}` would be converted to `{“firstName”: “John”, “lastName”: “Doe”}` in camelCase.
Are there any performance considerations when converting large JSON objects?
Yes, converting large JSON objects can be resource-intensive. It’s advisable to optimize the conversion process, possibly by using streaming APIs or processing the JSON in chunks to enhance performance.
In the realm of programming, particularly when dealing with Java and JSON data formats, the conversion between snake case and camel case is a common requirement. Snake case, characterized by its use of underscores to separate words (e.g., `example_variable`), contrasts with camel case, where the first letter of each subsequent word is capitalized without spaces (e.g., `exampleVariable`). Understanding how to effectively perform this conversion is essential for ensuring compatibility between different systems, particularly when integrating APIs or working with various data sources.
The process of converting snake case to camel case in Java can be accomplished through various methods, including the use of regular expressions or string manipulation techniques. Libraries such as Jackson can facilitate this conversion when working with JSON data, allowing developers to seamlessly map JSON properties to Java object fields. This is particularly useful in scenarios where JSON data is received in snake case format, and developers need to represent it in their Java applications using camel case conventions.
Key takeaways include the importance of consistency in naming conventions when developing applications. Adopting a systematic approach to converting between different case styles not only improves code readability but also enhances maintainability. Additionally, leveraging existing libraries can save time and reduce the potential for errors, allowing developers to focus on
Author Profile
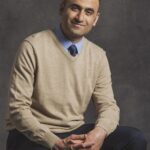
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?