How Can You Check if a File Exists Using JavaScript?
In the dynamic world of web development, ensuring that your application runs smoothly often hinges on the ability to manage files effectively. One common challenge developers face is determining whether a file exists before attempting to access or manipulate it. This seemingly simple task can lead to significant complications if not handled properly, resulting in errors that disrupt the user experience. In this article, we will explore the various methods available in JavaScript for checking the existence of files, equipping you with the knowledge to enhance your coding practices and streamline your projects.
When working with JavaScript, especially in environments like Node.js or the browser, the approach to checking for file existence can differ significantly. In server-side applications, developers can leverage built-in modules that provide straightforward methods for file system interactions. Conversely, in client-side JavaScript, the process may involve asynchronous operations and API calls, which require a different mindset and set of tools. Understanding these distinctions is crucial for implementing the right solution tailored to your specific context.
Moreover, the implications of file existence checks extend beyond mere error handling. They can play a vital role in optimizing performance, managing resources, and ensuring that your application behaves predictably. By mastering the techniques for checking file existence in JavaScript, you can not only avoid potential pitfalls but also enhance the overall robustness of
Checking for File Existence in JavaScript
In JavaScript, the ability to check if a file exists can vary based on the environment in which your code is running. Below are methods for both client-side and server-side checks.
Client-Side File Existence Check
In a browser environment, JavaScript does not have direct access to the file system for security reasons. However, you can check if a file exists by attempting to load it using an XMLHttpRequest or the Fetch API. Here’s how you can implement it:
**Using XMLHttpRequest:**
“`javascript
function checkFileExists(url, callback) {
const xhr = new XMLHttpRequest();
xhr.open(‘HEAD’, url, true);
xhr.onload = function() {
callback(xhr.status !== 404);
};
xhr.onerror = function() {
callback();
};
xhr.send();
}
// Usage example
checkFileExists(‘path/to/file.txt’, function(exists) {
console.log(‘File exists:’, exists);
});
“`
**Using Fetch API:**
“`javascript
function checkFileExists(url) {
return fetch(url, { method: ‘HEAD’ })
.then(response => response.ok)
.catch(() => );
}
// Usage example
checkFileExists(‘path/to/file.txt’).then(exists => {
console.log(‘File exists:’, exists);
});
“`
These methods send a request to the specified URL and check the response status. If the status is 200 (OK), the file exists; if it’s 404 (Not Found), it does not.
Server-Side File Existence Check
In a Node.js environment, you can check if a file exists using the `fs` (file system) module. Here are two common methods:
**Using `fs.existsSync`:**
“`javascript
const fs = require(‘fs’);
if (fs.existsSync(‘path/to/file.txt’)) {
console.log(‘File exists’);
} else {
console.log(‘File does not exist’);
}
“`
**Using `fs.access`:**
“`javascript
const fs = require(‘fs’);
fs.access(‘path/to/file.txt’, fs.constants.F_OK, (err) => {
console.log(err ? ‘File does not exist’ : ‘File exists’);
});
“`
The `fs.existsSync` method checks synchronously, blocking execution until the check is complete, while `fs.access` is asynchronous and preferred for non-blocking operations.
Comparison of Methods
Below is a comparison table highlighting the differences between the methods used for checking file existence in both environments:
Method | Environment | Asynchronous | Return Type |
---|---|---|---|
XMLHttpRequest | Client-Side | Yes | Callback |
Fetch API | Client-Side | Yes | Promise |
fs.existsSync | Server-Side | No | Boolean |
fs.access | Server-Side | Yes | Callback |
Understanding these methods will allow developers to effectively manage file existence checks in both client and server environments.
Checking File Existence in JavaScript
In JavaScript, checking if a file exists can vary depending on the environment in which the code is running. Below are methods to check for file existence in both Node.js (server-side) and browser environments.
Checking File Existence in Node.js
Node.js provides a straightforward way to check for file existence using the `fs` module. The `fs` module contains methods that allow you to interact with the file system.
**Using `fs.access`**:
“`javascript
const fs = require(‘fs’);
fs.access(‘path/to/file.txt’, fs.constants.F_OK, (err) => {
if (err) {
console.error(‘File does not exist’);
} else {
console.log(‘File exists’);
}
});
“`
Using `fs.existsSync`:
For synchronous operations, you can use `fs.existsSync`, which returns a boolean value.
“`javascript
const fs = require(‘fs’);
if (fs.existsSync(‘path/to/file.txt’)) {
console.log(‘File exists’);
} else {
console.log(‘File does not exist’);
}
“`
Method | Description | Asynchronous |
---|---|---|
`fs.access` | Checks the existence and permissions. | Yes |
`fs.existsSync` | Synchronously checks for existence. | No |
Checking File Existence in the Browser
In browser environments, checking if a file exists on the server is typically done via AJAX requests, as direct file system access is restricted for security reasons.
**Using `fetch`**:
“`javascript
fetch(‘path/to/file.txt’)
.then(response => {
if (response.ok) {
console.log(‘File exists’);
} else {
console.error(‘File does not exist’);
}
})
.catch(error => {
console.error(‘Error fetching the file:’, error);
});
“`
Using `XMLHttpRequest`:
Alternatively, you can use `XMLHttpRequest` for checking file existence.
“`javascript
const xhr = new XMLHttpRequest();
xhr.open(‘HEAD’, ‘path/to/file.txt’, true);
xhr.onreadystatechange = function () {
if (xhr.readyState === 4) {
if (xhr.status === 200) {
console.log(‘File exists’);
} else {
console.error(‘File does not exist’);
}
}
};
xhr.send();
“`
Method | Description | Browser Support |
---|---|---|
`fetch` | Modern way to make network requests. | All modern browsers |
`XMLHttpRequest` | Traditional method for handling requests. | All browsers |
Considerations
When checking for file existence, consider the following:
- Network Latency: When using `fetch` or `XMLHttpRequest`, network delays may affect the response time.
- Cross-Origin Requests: Ensure your server allows cross-origin requests if accessing files from different domains.
- Error Handling: Implement robust error handling to manage unexpected scenarios, such as network failures or permission issues.
These methods provide a reliable way to check for file existence across different JavaScript environments, ensuring efficient file handling in your applications.
Expert Insights on Checking File Existence in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In JavaScript, checking if a file exists can be approached differently depending on the environment. For Node.js, using the ‘fs’ module with ‘fs.existsSync’ or ‘fs.access’ is effective. However, in a browser context, you typically rely on server responses, as direct file system access is restricted for security reasons.”
Michael Chen (Lead Web Developer, Creative Solutions). “For web applications, verifying file existence often involves making an AJAX call to the server. If the server returns a 200 status code, the file exists; otherwise, it does not. This method is crucial for ensuring that resources are available before attempting to load them.”
Sarah Lopez (Full-Stack Developer, CodeCraft Labs). “In modern JavaScript frameworks, utilizing promises and async/await syntax can streamline the process of checking file existence. By wrapping file-checking logic in a promise, developers can handle both success and error states more elegantly, improving code readability and maintainability.”
Frequently Asked Questions (FAQs)
How can I check if a file exists in JavaScript?
You can check if a file exists in JavaScript using the `fs` module in Node.js. Use `fs.existsSync(filePath)` for synchronous checks or `fs.access(filePath, fs.constants.F_OK, callback)` for asynchronous checks.
Is there a way to check file existence in the browser using JavaScript?
In a browser environment, JavaScript does not have direct access to the file system for security reasons. However, you can check if a file exists on a server by making an HTTP request and checking the response status.
What does the `fs.existsSync` method do?
The `fs.existsSync` method synchronously checks if a given file or directory exists. It returns `true` if the file exists and “ otherwise.
Can I use `fetch` to check if a file exists on a server?
Yes, you can use the `fetch` API to check if a file exists on a server. By sending a request and checking the response status, you can determine if the file is available.
What is the difference between `fs.existsSync` and `fs.access`?
`fs.existsSync` is a synchronous method that blocks the execution until the check is complete, while `fs.access` is asynchronous and takes a callback function to handle the result, allowing other operations to continue during the check.
Are there any alternatives to check file existence in JavaScript?
In addition to `fs` methods in Node.js and `fetch` in the browser, you can use libraries like `axios` for HTTP requests or `fs.promises` for promise-based file system operations in Node.js.
checking if a file exists in JavaScript can be approached in various ways depending on the environment in which the code is executed. In a Node.js context, developers can utilize the built-in `fs` (file system) module, which provides methods such as `fs.existsSync()` for synchronous checks and `fs.access()` for asynchronous checks. These methods allow developers to efficiently verify the presence of files on the server’s file system.
In contrast, when working in a browser environment, direct file system access is restricted for security reasons. Instead, developers can check for the existence of files through user input, such as file uploads, or by making requests to a server to determine if a file is available at a specified URL. Utilizing the `fetch` API or XMLHttpRequest can help ascertain whether a file exists on a remote server.
Key takeaways include the importance of understanding the environment when implementing file existence checks in JavaScript. Developers should choose the appropriate method based on whether they are operating in a server-side or client-side context. Additionally, handling potential errors gracefully is crucial, especially when dealing with asynchronous operations or network requests, to ensure a smooth user experience.
Author Profile
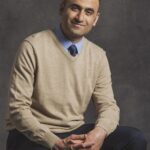
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?