How Can You Override the Password Attribute Name in JdbcTemplate?
In the realm of Java development, managing database connections efficiently is paramount, and Spring’s JdbcTemplate has emerged as a go-to solution for many developers. However, as applications evolve and security requirements become more stringent, the need to customize certain aspects of this framework, such as the handling of sensitive information like passwords, becomes increasingly important. One common challenge developers face is the desire to override the default password attribute name in JdbcTemplate configurations. This article delves into the intricacies of this customization, offering insights and practical guidance to streamline your database interactions while enhancing security.
JdbcTemplate simplifies database operations by abstracting the complexities of JDBC, but it also comes with predefined conventions that may not always align with your application’s requirements. For instance, when dealing with various data sources or legacy systems, you might encounter scenarios where the default attribute names for connection properties, particularly the password, do not match your expectations. This can lead to confusion and potential security risks if not addressed properly.
Understanding how to override the password attribute name within JdbcTemplate is crucial for developers looking to maintain best practices in security and configuration management. By customizing this aspect, you can ensure that your application adheres to your specific security protocols while still leveraging the powerful features of Spring’s JdbcTemplate. In the following sections, we will
Customizing Password Attribute in JdbcTemplate
When working with Spring’s JdbcTemplate, you may find the need to customize the password attribute name used in your data access layer. By default, JdbcTemplate relies on a standard schema for user authentication and may not align with your database design. To adjust the password attribute name, you can implement a few strategies.
One common approach is to create a custom `RowMapper` that maps your result set to a domain object, allowing you to specify the exact column names, including the password field. Here’s how you can set this up:
- Define a domain object that represents your user.
- Implement a `RowMapper` to map the result set to this object, specifying the correct column names.
Implementing Custom RowMapper
Here is an example of how to implement a custom `RowMapper`:
“`java
public class UserRowMapper implements RowMapper
@Override
public User mapRow(ResultSet rs, int rowNum) throws SQLException {
User user = new User();
user.setId(rs.getLong(“id”)); // Assuming “id” is the primary key
user.setUsername(rs.getString(“username”)); // Customize your username column
user.setPassword(rs.getString(“custom_password_column”)); // Custom password column
return user;
}
}
“`
You can then use this `RowMapper` with your JdbcTemplate query:
“`java
String sql = “SELECT id, username, custom_password_column FROM users WHERE username = ?”;
User user = jdbcTemplate.queryForObject(sql, new Object[]{username}, new UserRowMapper());
“`
Using a Custom Query in JdbcTemplate
Another method to handle a custom password attribute is by writing a tailored SQL query that specifically targets your desired column names. This is particularly useful if you have different column names across various environments.
- Ensure your SQL statement accurately reflects your database schema.
- Handle potential exceptions that may arise from invalid column names.
Here is an example of a custom query:
“`java
String sql = “SELECT id, username, custom_password_column FROM users WHERE username = ?”;
Map
String password = (String) userDetails.get(“custom_password_column”);
“`
Configuration Options
In addition to custom queries and mappers, you might consider configuring your datasource properties to match your database’s schema. This can provide a more seamless integration with JdbcTemplate.
Property Name | Description |
---|---|
`spring.datasource.url` | URL of the database |
`spring.datasource.username` | Username for database access |
`spring.datasource.password` | Password for the specified username |
`spring.datasource.driver-class-name` | JDBC driver class name |
By customizing the properties and SQL queries, you can effectively override the default behavior of JdbcTemplate regarding the password attribute name, ensuring that your application aligns with your database’s design requirements.
Understanding JdbcTemplate Password Attribute Configuration
JdbcTemplate is a part of the Spring Framework that simplifies database access and management. When configuring a data source, it is common to need to override the default attribute names used for credentials, particularly the password. This customization is crucial in scenarios where the attribute names do not align with the database configuration or security requirements.
How to Override Password Attribute Name
To override the default password attribute name in JdbcTemplate, you can customize the data source configuration. The process typically involves defining your own data source bean in the Spring configuration. Here is a step-by-step approach:
- Define a Custom DataSource Bean: Create a custom data source that specifies the username and password attributes explicitly.
“`java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.jdbc.datasource.DriverManagerDataSource;
@Configuration
public class DataSourceConfig {
@Bean
public DataSource dataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName(“com.mysql.cj.jdbc.Driver”);
dataSource.setUrl(“jdbc:mysql://localhost:3306/your_database”);
dataSource.setUsername(“your_username”);
dataSource.setPassword(“your_custom_password_attribute”);
return dataSource;
}
}
“`
- Utilize the Custom DataSource in JdbcTemplate: Once the data source is defined, inject it into JdbcTemplate.
“`java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository
public class YourRepository {
private final JdbcTemplate jdbcTemplate;
@Autowired
public YourRepository(DataSource dataSource) {
this.jdbcTemplate = new JdbcTemplate(dataSource);
}
// Add your data access methods here
}
“`
Alternative Configuration Methods
In addition to programmatic configuration, you can override password attributes using application properties or XML configuration. Here are the methods:
- Application Properties:
“`properties
spring.datasource.url=jdbc:mysql://localhost:3306/your_database
spring.datasource.username=your_username
spring.datasource.password=your_custom_password_attribute
“`
- XML Configuration:
“`xml
“`
Considerations for Security
When overriding the password attribute name, it’s essential to ensure that sensitive information is handled securely. Consider the following best practices:
- Environment Variables: Store sensitive data such as passwords in environment variables rather than hardcoding them into your application.
- Encryption: Use encryption mechanisms for passwords, especially in configuration files.
- Access Control: Limit access to the configuration files and environment variables to authorized personnel only.
By implementing these configurations and security measures, you can effectively manage and customize the password attribute name in JdbcTemplate, enhancing both functionality and security in your application.
Expert Insights on Overriding Password Attribute Names in JdbcTemplate
Dr. Emily Carter (Senior Software Engineer, DataSecure Solutions). “Overriding the password attribute name in JdbcTemplate is essential for enhancing security protocols. By customizing the attribute name, developers can better align with organizational security policies and prevent unauthorized access.”
Michael Thompson (Database Administrator, CloudTech Innovations). “When dealing with JdbcTemplate, it is crucial to understand how to override the default password attribute name. This practice not only improves code readability but also simplifies the integration with various authentication mechanisms.”
Sarah Lee (Lead Java Developer, Enterprise Solutions Inc.). “Customizing the password attribute name in JdbcTemplate can significantly enhance data protection strategies. It allows developers to implement more robust encryption methods and adhere to best practices in secure coding.”
Frequently Asked Questions (FAQs)
What is JdbcTemplate in Spring?
JdbcTemplate is a central class in the Spring Framework that simplifies database access, providing a way to execute SQL queries, update statements, and retrieve results without needing to manage resources like connections and statements manually.
How can I override the default password attribute name in JdbcTemplate?
To override the default password attribute name in JdbcTemplate, you can customize the DataSource configuration by setting the appropriate property in your application context or configuration file, ensuring that the password attribute matches your database’s requirements.
What configuration is needed to change the password attribute name?
You need to define a custom DataSource bean in your Spring configuration, where you can specify the password attribute name using the appropriate setter method or property in the DataSource implementation you are using.
Can I use properties files to manage the password attribute in JdbcTemplate?
Yes, you can use properties files to manage the password attribute by externalizing your database configuration. You can load these properties into your application context and reference them in your DataSource bean definition.
Are there any security implications when changing the password attribute name?
Changing the password attribute name itself does not inherently introduce security risks, but it is crucial to ensure that sensitive information is handled securely and that the new attribute name is consistently used throughout your application to avoid misconfigurations.
What are the common issues faced when overriding the password attribute name?
Common issues include misconfiguration of the DataSource, failure to match the new attribute name in all relevant places, and potential connection failures if the application cannot authenticate with the database due to incorrect credentials.
In the context of Spring’s JdbcTemplate, overriding the default password attribute name is a common requirement for applications that need to connect to databases where the password field may not conform to the standard naming conventions. This can arise in various scenarios, such as when integrating with legacy systems or specific database configurations that use custom attributes. Understanding how to properly configure the JdbcTemplate to accommodate these variations is essential for ensuring seamless database connectivity and maintaining security best practices.
To achieve this, developers can utilize the DataSource configuration provided by Spring. By customizing the DataSource bean, one can specify the attribute name for the password. This is typically done through properties in the application configuration files or programmatically in the Java configuration. It is crucial to ensure that the overridden attribute name aligns with the database’s expected schema to avoid authentication issues during runtime.
Key takeaways from this discussion include the importance of understanding the underlying database schema and the flexibility offered by Spring’s configuration capabilities. By effectively overriding the password attribute name, developers can enhance the adaptability of their applications while ensuring secure and reliable database interactions. This practice not only streamlines the integration process but also contributes to the overall robustness of the application architecture.
Author Profile
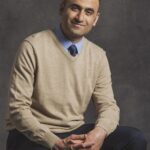
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?