Why Is My Jest Config Custom Environment TestEnvironment Not Working or Cannot Be Found?
In the realm of JavaScript testing, Jest has emerged as a powerful and flexible framework, favored by developers for its simplicity and efficiency. However, as projects grow in complexity, so too do the challenges associated with configuring Jest to meet specific testing needs. One common hurdle that developers encounter is the elusive “custom environment” setting within the Jest configuration. When the test environment fails to work or cannot be found, it can lead to confusion and frustration, stalling the development process. In this article, we will delve into the intricacies of Jest’s configuration, exploring the nuances of custom environments and how to troubleshoot the issues that may arise.
Understanding Jest’s configuration options is crucial for any developer looking to tailor their testing environment. The test environment is a pivotal aspect of Jest that dictates how tests are executed and how the code behaves during testing. By default, Jest provides a standard environment, but many projects require a specialized setup to accommodate unique requirements or dependencies. This is where custom environments come into play, allowing developers to define their own testing scenarios. However, misconfigurations or missing files can lead to the dreaded “not found” error, leaving developers scratching their heads.
As we navigate through the potential pitfalls of setting up a custom test environment in Jest, we will address common mistakes and
Understanding Custom Test Environments in Jest
Configuring a custom test environment in Jest requires precise attention to detail. If you encounter issues where the specified environment cannot be found, it may stem from several common misconfigurations. The custom environment can be defined in the Jest configuration file, typically `jest.config.js` or `package.json`, under the `testEnvironment` key.
When setting up a custom environment, ensure you follow these guidelines:
- File Structure: The path to your custom environment file must be correct relative to the Jest configuration file.
- Exporting the Class: The custom environment should be a class that extends `NodeEnvironment` or `JSDOMEnvironment`. Make sure it is properly exported in your module.
- Module Resolution: If using TypeScript or Babel, confirm that the module resolution is properly set up to recognize the environment file.
Common Causes for Test Environment Issues
Several factors can lead to Jest failing to locate or correctly utilize a custom test environment. Below are some common causes:
- Incorrect Path: Ensure that the path to the custom environment is correct. A relative path issue can prevent Jest from locating the file.
- Improper Export: The environment file must export a class correctly; otherwise, Jest will not be able to instantiate it.
- Dependency Conflicts: Conflicts with other packages or Jest versions can sometimes lead to unexpected behavior.
- Cache Issues: Cached files can cause Jest to use outdated configurations. Consider clearing the cache using `jest –clearCache`.
Issue | Solution |
---|---|
Incorrect Path | Verify the path in the `jest.config.js` file to ensure it points to the correct location. |
Improper Export | Check the environment file to confirm it exports the class correctly. |
Dependency Conflicts | Review package.json for version conflicts and update as necessary. |
Cache Issues | Run `jest –clearCache` to remove any stale cache. |
Debugging Steps for Custom Environments
When troubleshooting a custom test environment setup, consider the following debugging steps:
- Verbose Logging: Run Jest with the `–verbose` flag to get detailed output about what Jest is doing during the test run.
- Check Jest Configuration: Double-check the Jest configuration file for any typos or misconfigurations.
- Unit Test the Environment: Create a simple test case that directly uses the custom environment to confirm it is being recognized.
- Use Console Logs: Add console logs within the custom environment’s setup and teardown methods to track its execution flow.
By systematically following these steps and addressing common issues, you can effectively resolve problems related to custom test environments in Jest.
Understanding Jest Custom Test Environments
Jest allows developers to define custom test environments that can be tailored to specific requirements. However, issues can arise when configuring these environments, particularly with the `testEnvironment` option in Jest’s configuration file.
Common Issues with Custom Test Environments
When a custom test environment is not found or fails to work, the following factors may contribute to the issue:
- Incorrect Path: Ensure that the path to the custom environment file is correct. Jest expects a relative path from the root of the project.
- File Naming Conventions: The custom environment file should have the proper naming conventions (e.g., `.js` or `.ts` extension depending on your setup).
- Export Statement: The test environment file must correctly export a class that extends Jest’s `Environment` class.
Configuration Example
Here is an example of how to set a custom test environment in Jest:
“`json
{
“jest”: {
“testEnvironment”: “
}
}
“`
Ensure that `customEnvironment.js` correctly defines the environment class:
“`javascript
const NodeEnvironment = require(‘jest-environment-node’);
class CustomEnvironment extends NodeEnvironment {
async setup() {
await super.setup();
// Custom setup code
}
async teardown() {
// Custom teardown code
await super.teardown();
}
}
module.exports = CustomEnvironment;
“`
Debugging Steps
If the custom environment is still not recognized, consider the following debugging steps:
- Run Jest with Verbose Logging: Use the `–verbose` flag to get more detailed output. This can help identify where the configuration might be failing.
- Check for Typos: Ensure that there are no typos in the Jest configuration or the custom environment file.
- Dependencies: Verify that all necessary dependencies are installed and compatible with your version of Jest.
Alternative Solutions
If issues persist, consider these alternatives:
- Use Built-in Environments: Utilize Jest’s built-in environments (e.g., `jsdom`, `node`) to see if the tests pass under those configurations.
- Community Packages: Look for community-contributed packages that may offer similar functionality without needing a custom environment.
- Documentation Review: Check Jest’s official documentation for any updates or changes regarding custom environments.
By following the configuration guidelines and debugging steps outlined above, developers can effectively troubleshoot issues related to Jest’s custom test environments.
Resolving Jest Config Custom Environment Issues
Dr. Emily Carter (Senior Software Engineer, Testing Innovations Inc.). “When encountering issues with Jest configuration, particularly with custom environments, it is crucial to ensure that the specified environment is correctly referenced in the configuration file. Double-check the ‘testEnvironment’ property for any typos or incorrect paths that may lead to the error ‘cannot be found’.”
Michael Chen (Lead Developer, Code Quality Solutions). “A common pitfall in Jest configurations is the mismatch between the expected environment and the installed packages. If you are using a custom environment, verify that the corresponding package is installed and correctly listed in your dependencies. This often resolves the issue of Jest not recognizing the custom environment.”
Sarah Thompson (Software Testing Consultant, Agile Testers Group). “In scenarios where ‘testEnvironment’ is not working as expected, consider clearing the Jest cache or running Jest with the ‘–no-cache’ option. This can sometimes resolve hidden issues that prevent Jest from recognizing the custom environment specified in your configuration.”
Frequently Asked Questions (FAQs)
What is a custom environment in Jest?
A custom environment in Jest allows developers to define specific settings and behaviors for their tests that differ from the default Node environment. This can be useful for testing applications that require a specific runtime or context.
How do I configure a custom environment in Jest?
To configure a custom environment, you need to specify the environment in your Jest configuration file (jest.config.js) using the `testEnvironment` property. Set it to the path of your custom environment file.
Why is my custom environment not being recognized by Jest?
If your custom environment is not recognized, ensure that the path specified in the `testEnvironment` property is correct and that the environment file is properly exported. Additionally, check for any typos in the configuration.
What should I do if Jest cannot find my custom environment file?
If Jest cannot find your custom environment file, verify that the file exists in the specified location and that the path is relative to the Jest configuration file. Also, ensure that the file has the correct permissions for Jest to access it.
Can I use third-party libraries with a custom Jest environment?
Yes, you can use third-party libraries with a custom Jest environment. However, ensure that the libraries are compatible with the environment you have set up and that they are correctly imported within your test files.
What are common issues when setting up a custom test environment in Jest?
Common issues include incorrect file paths, missing dependencies, and misconfigured Jest settings. Additionally, ensure that the custom environment extends from a valid Jest environment class, such as `NodeEnvironment` or `JsDomEnvironment`, to avoid compatibility issues.
In the context of configuring Jest for testing environments, the issue of a custom environment specified in the Jest configuration not being found is a common challenge faced by developers. This often arises from misconfigurations in the Jest setup, such as incorrect paths, missing dependencies, or improperly defined environment files. Ensuring that the custom environment is correctly implemented and accessible within the project’s structure is crucial for seamless test execution.
One of the key takeaways is the importance of verifying the configuration settings in the Jest configuration file, typically `jest.config.js` or `package.json`. Developers should ensure that the `testEnvironment` property points to the correct custom environment file. Additionally, it is essential to check for the presence of the required dependencies and that they are correctly installed in the project. This can prevent the common pitfalls associated with missing or misconfigured environments.
Another valuable insight is the necessity of thorough documentation and community resources when dealing with custom Jest environments. Engaging with the Jest community through forums or GitHub can provide solutions and best practices that can help mitigate these issues. Regularly updating Jest and its associated packages can also resolve compatibility problems that may lead to the environment not being found.
Author Profile
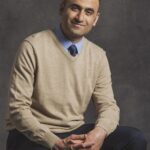
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?