How Can You Speed Up Jest Tests with All Async Code?
In the fast-paced world of software development, where time is of the essence, the efficiency of your testing suite can make or break your project. As developers increasingly rely on asynchronous operations to enhance application performance, the challenge of maintaining swift and reliable tests becomes paramount. Enter Jest, a powerful testing framework that not only simplifies the testing process but also offers a myriad of tools to optimize test execution. If you’re grappling with sluggish test runs due to an abundance of asynchronous code, you’re not alone. In this article, we’ll explore effective strategies to speed up your Jest tests while fully embracing the asynchronous nature of modern JavaScript.
Asynchronous testing can often lead to longer execution times, especially when tests are not optimized for performance. With Jest, however, there are several techniques you can employ to streamline your testing process without sacrificing accuracy. From leveraging built-in features like test concurrency to utilizing mock functions effectively, these strategies can significantly reduce the time it takes to run your entire test suite.
Moreover, understanding how to structure your tests and manage asynchronous operations can lead to a more efficient workflow. By implementing best practices tailored for asynchronous testing, you can ensure that your Jest tests run faster, allowing you to focus on what truly matters: delivering high-quality code. Join us as we delve deeper into these
Utilizing Jest’s Built-in Features for Async Testing
Jest offers several built-in features that can help streamline the process of testing asynchronous code, ultimately enhancing the speed and efficiency of your test suite. By leveraging these features, developers can reduce the time spent on setup and teardown, thereby speeding up test execution.
- Promise Handling: Jest automatically handles promises in your tests. By returning a promise from your test function, Jest will wait for it to resolve before completing the test. This eliminates the need for manual `done` callbacks in most scenarios.
- Async/Await Syntax: Utilizing `async` and `await` can lead to cleaner code and a more synchronous style of writing asynchronous tests. It can improve readability and help avoid common pitfalls associated with promise chaining.
Parallel Test Execution
By default, Jest runs tests in parallel, which can significantly speed up the execution time. Each test file runs in its own process, ensuring that tests do not interfere with one another. Here are some strategies to maximize parallel execution:
- Isolate Tests: Ensure that tests are independent of each other. Shared state can lead to race conditions, which may slow down tests or lead to flaky results.
- Split Tests into Separate Files: Organizing tests into multiple files allows Jest to utilize parallel processes effectively. Each file can be executed in isolation.
Optimizing Test Environment
The test environment can significantly impact the performance of Jest tests. Here are several optimizations to consider:
- Use `–runInBand` only when necessary: While this command forces Jest to run tests serially, it can be useful for debugging or when tests require shared resources. However, it should be avoided in regular runs to leverage parallel execution.
- Configure Jest’s Cache: Jest provides a caching mechanism that can prevent unnecessary re-runs of unchanged tests. Ensure that the cache is enabled and properly configured in your Jest setup.
Reducing Test Time with Mocking
Mocking is an essential practice in Jest that can lead to faster tests by isolating external dependencies. Here are some benefits and strategies for effective mocking:
- Mocking Network Requests: Instead of making actual API calls, use Jest’s mocking capabilities to simulate responses. This reduces the time spent waiting for network latency.
- Mocking Modules: Use `jest.mock()` to replace complex modules with simpler mocks, allowing tests to run faster and reducing the overhead associated with setup.
Mocking Strategy | Benefit |
---|---|
Network Requests | Eliminates latency and flakiness due to network issues |
Database Calls | Reduces time spent on setup and teardown of test data |
Complex Modules | Simplifies tests and reduces execution time |
By effectively utilizing Jest’s features for async testing, optimizing the test environment, and leveraging mocking strategies, you can significantly enhance the speed of your test suite while maintaining robustness and reliability in your test outcomes.
Optimizing Async Tests in Jest
When dealing with asynchronous tests in Jest, it’s crucial to adopt strategies that minimize wait times and optimize performance. Here are effective methods to speed up your tests:
Utilize Async/Await Properly
Using async/await syntax enhances readability and helps manage asynchronous code more effectively. Ensure that all async functions are awaited properly to avoid unintentional delays in test execution.
- Always use `await` when calling async functions.
- Ensure that your async functions return promises.
Limit Parallel Execution
Jest runs tests in parallel, but excessive parallelism can lead to resource contention. Limit the number of concurrent tests to improve overall speed.
- Use `–maxWorkers=
` to specify the number of worker threads. - Consider reducing the number of workers during resource-intensive operations.
Mock External Dependencies
Mocking external services or APIs can significantly speed up tests by eliminating network latency.
- Use Jest’s built-in mocking capabilities, such as `jest.mock()`, to replace real implementations with mock functions.
- Ensure that mocked functions return results quickly and realistically.
Optimize Test Structure
The organization of tests can impact performance. Consider the following:
- Group related tests to minimize setup and teardown overhead.
- Use `beforeAll` and `afterAll` for setup and teardown that only needs to happen once per test suite.
Reduce Test Data Size
Large datasets can slow down tests considerably. Use smaller datasets for testing wherever possible.
- Create minimal datasets that still cover all edge cases.
- Use fixtures or factories to generate only the necessary data.
Leverage Jest Configuration Options
Jest provides configuration options that can enhance performance.
Option | Description |
---|---|
`testTimeout` | Increase or decrease the default test timeout. |
`detectOpenHandles` | Disable this option if you are sure there are no open handles. |
`testEnvironment` | Choose a lighter test environment if feasible. |
Use `–runInBand` for Resource-Heavy Tests
When tests are resource-heavy, running them sequentially may yield better performance. The `–runInBand` option executes tests in the main thread.
- Command: `jest –runInBand`
- Useful for debugging and reducing resource contention.
Profile and Analyze Test Performance
Regularly assess test performance to identify bottlenecks. Jest provides useful tools for profiling.
- Use the `–detectOpenHandles` option to find unclosed resources.
- Analyze slow tests by using the `–reporters` option with performance metrics.
By implementing these strategies, you can significantly improve the speed of asynchronous tests in Jest, ensuring efficient and effective testing processes.
Strategies for Accelerating Async Tests in Jest
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To speed up tests with all async operations in Jest, it is crucial to minimize the use of unnecessary awaits. By leveraging Promise.all for concurrent operations, you can significantly reduce the overall execution time of your tests.”
Michael Thompson (Lead QA Analyst, Agile Testing Solutions). “Utilizing Jest’s built-in mocking capabilities can enhance test performance. By mocking external API calls or database interactions, you eliminate the latency associated with these operations, allowing your async tests to run much faster.”
Sarah Kim (Performance Engineer, CodeSpeed Labs). “Another effective method to speed up async tests in Jest is to run tests in parallel. Configuring Jest to utilize worker threads can help execute multiple tests simultaneously, leading to a significant reduction in total test time.”
Frequently Asked Questions (FAQs)
How can I identify slow tests in Jest?
You can identify slow tests in Jest by using the `–detectOpenHandles` flag when running your tests. This will help you find tests that are not properly closing asynchronous operations, which can lead to longer execution times.
What strategies can I use to speed up async tests in Jest?
To speed up async tests in Jest, consider using `Promise.all` to run multiple asynchronous operations concurrently, avoid unnecessary delays in your tests, and ensure that you are not using excessive mocking that can slow down execution.
Is it beneficial to use `jest.setTimeout` for async tests?
Yes, using `jest.setTimeout` can be beneficial for async tests, as it allows you to adjust the default timeout for specific tests that may require more time to complete. This can prevent tests from failing prematurely due to timeout errors.
How can I optimize database calls in my Jest tests?
You can optimize database calls in Jest tests by using in-memory databases, such as SQLite, for testing purposes. Additionally, consider mocking database interactions to avoid actual calls during tests, which can significantly reduce execution time.
Can I run tests in parallel to improve performance?
Yes, Jest runs tests in parallel by default. To further enhance performance, ensure that your tests are independent and do not share state, allowing them to execute concurrently without interference.
What role does proper teardown play in speeding up Jest tests?
Proper teardown is crucial for speeding up Jest tests as it ensures that all resources are released after tests run. This prevents memory leaks and reduces the time needed for subsequent tests, leading to overall faster test execution.
In the context of using Jest for testing asynchronous code, there are several strategies to enhance the speed and efficiency of test execution. One of the primary approaches involves leveraging Jest’s built-in capabilities for handling asynchronous operations. This includes utilizing async/await syntax, which can simplify the code and reduce the overhead associated with promise handling. By ensuring that tests are written in a clear and concise manner, developers can minimize execution time while maintaining readability.
Another effective method to speed up Jest tests is to optimize the test environment. This can be achieved by configuring Jest to run tests in parallel, thereby taking full advantage of available CPU cores. Additionally, using the `–runInBand` flag can be beneficial for debugging, but it may slow down test execution. Therefore, it is crucial to strike a balance between parallel execution and debugging needs. Furthermore, utilizing Jest’s `–watch` mode can help developers run only the tests that are affected by recent changes, further improving efficiency.
Lastly, it is essential to review and refactor tests regularly. This includes removing redundant tests, optimizing test data setup, and ensuring that mocks and spies are used judiciously. By keeping the test suite lean and focused, developers can significantly reduce the time it takes to
Author Profile
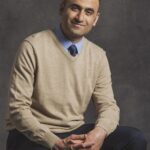
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?