Can jQuery Be Used to Mimic AI Chat Responses Effectively?
In the rapidly evolving landscape of web development, the intersection of artificial intelligence and user experience is becoming increasingly significant. One particularly intriguing aspect of this convergence is the use of jQuery to create dynamic, AI-like chat responses that can enhance user interaction on websites. Imagine a scenario where a simple webpage transforms into an engaging conversational partner, seamlessly mimicking the responses of a human chat agent. This article delves into the fascinating world of jQuery and its capabilities in crafting chat interfaces that not only respond intelligently but also adapt to user inputs in real-time, creating a more immersive online experience.
As businesses strive to improve customer engagement and satisfaction, the demand for interactive chat solutions has surged. By leveraging jQuery, developers can build chatbots that simulate human-like conversations, providing users with instant answers and assistance. This approach not only streamlines communication but also allows for a more personalized interaction, making users feel heard and valued. The ability to mimic AI chat responses through jQuery opens up a realm of possibilities, from enhancing e-commerce platforms to providing support in educational environments.
Moreover, the integration of jQuery with other technologies can further amplify the effectiveness of these chat systems. By utilizing APIs and machine learning algorithms, developers can create sophisticated chatbots capable of understanding context, sentiment, and
Understanding jQuery in Chat Applications
jQuery is a powerful JavaScript library that simplifies HTML document traversing, event handling, and animation, making it an excellent choice for enhancing user interfaces in chat applications. By integrating jQuery, developers can create dynamic, responsive chat features that mimic artificial intelligence (AI) chat responses effectively.
Key functionalities of jQuery in chat applications include:
- DOM Manipulation: jQuery allows for easy manipulation of the Document Object Model (DOM), enabling developers to update chat interfaces in real-time without requiring page reloads.
- Event Handling: With jQuery, you can handle user interactions such as clicks and key presses efficiently, making it easier to trigger chat responses based on user input.
- AJAX Support: jQuery simplifies AJAX calls, allowing for seamless communication between the client and server, which is crucial for retrieving AI-generated responses without interrupting the user experience.
Creating AI-Like Responses with jQuery
To mimic AI chat responses, developers can utilize jQuery to create a responsive interface that interacts with a backend AI service. The process typically involves the following steps:
- Capture User Input: Use jQuery to capture the user’s message when they submit it.
- Send the Input to the Server: Implement an AJAX call to send the user’s message to an AI service or backend endpoint that processes the input.
- Receive and Display AI Responses: Once the server processes the input, it returns a response, which can be dynamically added to the chat interface using jQuery.
Here is a simple structure of how this workflow can be implemented:
“`javascript
$(document).ready(function() {
$(‘sendButton’).click(function() {
var userInput = $(‘userInput’).val();
$.ajax({
url: ‘https://your-ai-service.com/api/chat’,
type: ‘POST’,
data: JSON.stringify({ message: userInput }),
contentType: ‘application/json’,
success: function(response) {
$(‘chatWindow’).append(‘
‘);
}
});
});
});
“`
Example Implementation: Chatbot Interface
To illustrate how jQuery can be used to create a chatbot interface that mimics AI responses, consider the following example:
“`html
“`
The above HTML provides a basic structure for a chat interface. The accompanying jQuery script allows for interaction and response handling.
Benefits of Using jQuery for Chat Applications
Utilizing jQuery for developing chat applications provides several advantages:
- Ease of Use: jQuery’s simple syntax allows developers to implement complex features quickly.
- Cross-Browser Compatibility: jQuery handles many inconsistencies across browsers, ensuring a uniform experience for users.
- Rich Plugin Ecosystem: A wide array of plugins are available, extending jQuery’s functionality for chat applications.
Feature | Benefit |
---|---|
DOM Manipulation | Real-time updates to chat interface |
Event Handling | Responsive user interactions |
AJAX Support | Seamless data exchange with the server |
By leveraging jQuery’s capabilities, developers can create sophisticated chat applications that provide users with an engaging and interactive experience, closely resembling a natural conversation with AI.
Understanding jQuery for AI Chat Mimicking
jQuery is a powerful JavaScript library that simplifies HTML document manipulation, event handling, and Ajax interactions. When integrating jQuery to mimic AI chat responses, several key aspects must be considered:
- Dynamic Content Handling: jQuery can dynamically update the chat interface by appending messages as they are sent or received.
- Event Management: Use jQuery to manage user inputs and trigger responses based on interactions, such as pressing the “Enter” key.
- Ajax Calls: jQuery simplifies making asynchronous requests to a server, allowing for real-time chat functionalities.
Setting Up the Chat Interface
Creating a user-friendly chat interface involves both HTML and CSS, supplemented by jQuery for interactivity. Below is a simple structure:
“`html
“`
CSS for Basic Styling:
“`css
chat-window {
width: 300px;
border: 1px solid ccc;
padding: 10px;
background-color: f9f9f9;
}
messages {
height: 200px;
overflow-y: scroll;
border-bottom: 1px solid ccc;
margin-bottom: 10px;
}
.message {
margin: 5px 0;
}
“`
Implementing jQuery for Interaction
To handle user input and simulate AI responses, the following jQuery code can be utilized:
“`javascript
$(document).ready(function() {
$(‘send-button’).click(function() {
let userMessage = $(‘user-input’).val();
appendMessage(userMessage, ‘user’);
$(‘user-input’).val(”);
simulateAIResponse(userMessage);
});
$(‘user-input’).keypress(function(event) {
if (event.which === 13) {
$(‘send-button’).click();
}
});
function appendMessage(message, sender) {
$(‘messages’).append(`
`);
$(‘messages’).scrollTop($(‘messages’)[0].scrollHeight);
}
function simulateAIResponse(userMessage) {
let aiResponse = generateAIResponse(userMessage);
setTimeout(() => {
appendMessage(aiResponse, ‘ai’);
}, 1000);
}
function generateAIResponse(message) {
// Simple simulation of AI response generation
return “AI: ” + message.split(”).reverse().join(”); // Example response
}
});
“`
Enhancing User Experience
To elevate the chat experience, consider implementing the following features:
- Typing Indicators: Show an indicator when the AI is “typing.”
- Voice Responses: Integrate text-to-speech for AI replies.
- Customizable Themes: Allow users to change the look and feel of the chat interface.
Feature | Description |
---|---|
Typing Indicators | Visual feedback when the AI is generating a response |
Voice Responses | Speech synthesis for a more interactive experience |
Customizable Themes | Options for users to personalize the chat interface |
Testing and Debugging
Testing the chat application is critical to ensure functionality and user satisfaction. Utilize browser developer tools to:
- Inspect elements and debug jQuery code.
- Monitor network requests to check Ajax functionality.
- Validate user inputs and handle errors gracefully.
Ensure comprehensive testing across multiple devices and browsers to verify compatibility and responsiveness.
Exploring the Possibilities of jQuery in AI Chat Response Simulation
Dr. Emily Carter (Lead AI Researcher, Tech Innovations Lab). “jQuery can indeed be utilized to mimic AI chat responses by facilitating dynamic content updates and user interactions. By leveraging its event handling capabilities, developers can create responsive interfaces that simulate conversational flows, enhancing user engagement.”
Michael Tran (Senior Front-End Developer, Interactive Solutions). “While jQuery is not inherently designed for AI functionalities, it can effectively manage asynchronous requests to backend AI services. This allows for real-time chat simulations that can provide users with a seamless experience, making it a valuable tool in the development of chat interfaces.”
Sarah Lee (UX/UI Designer, Future Tech Designs). “Incorporating jQuery into chat response simulations can significantly enhance the user interface. By utilizing animations and transitions, developers can create a more engaging and lifelike chat experience, which is essential for mimicking the fluidity of human conversation.”
Frequently Asked Questions (FAQs)
What is jQuery and how does it relate to AI chat responses?
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversing, event handling, and animation. It can be used to enhance user interfaces in AI chat applications, facilitating dynamic interactions.
Can jQuery be used to create AI chatbots?
While jQuery is not a dedicated AI framework, it can be utilized to build the front-end interface of chatbots, allowing for interactive elements and seamless user experience. However, the AI logic typically relies on backend technologies.
How can jQuery mimic AI chat responses?
jQuery can simulate AI chat responses by creating predefined response patterns and using AJAX to fetch data from a server. This enables the chatbot to respond dynamically to user inputs without reloading the page.
What are the limitations of using jQuery for AI chat applications?
jQuery primarily handles client-side scripting, which limits its ability to perform complex AI functions such as natural language processing. For advanced capabilities, integration with backend AI services is necessary.
Are there any libraries that complement jQuery for AI chat functionalities?
Yes, libraries such as Botpress, Rasa, or Dialogflow can be integrated with jQuery to enhance AI capabilities, allowing for more sophisticated conversational interfaces and machine learning features.
Is jQuery suitable for real-time chat applications?
jQuery can be used in real-time chat applications, but it is often more effective when combined with WebSocket or other technologies that support real-time communication, ensuring a smooth user experience.
In summary, utilizing jQuery to mimic AI chat responses involves leveraging the library’s capabilities to create interactive and dynamic web applications. By integrating jQuery with various APIs and libraries, developers can simulate conversational interfaces that respond to user inputs in a natural and engaging manner. This approach allows for the development of chatbots that can enhance user experience on websites and applications, providing immediate assistance and information to users.
Furthermore, the implementation of jQuery in creating AI chat responses can significantly streamline the development process. With its simplified syntax and robust features, jQuery enables developers to manipulate the Document Object Model (DOM) efficiently, handle events seamlessly, and manage asynchronous operations effectively. This results in a more responsive and user-friendly interface, which is crucial for maintaining user engagement in chat applications.
Key takeaways from this discussion include the importance of combining jQuery with AI technologies to create more sophisticated chatbots. By understanding how to utilize jQuery’s functionalities, developers can enhance the interactivity of their applications, making them not only more appealing but also more functional. Ultimately, the ability to mimic AI chat responses through jQuery opens up new avenues for innovation in user interaction and customer service solutions.
Author Profile
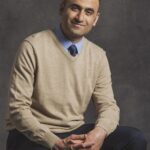
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?