How Can I Use jQuery to Mimic Chat Assistant Responses?
In the ever-evolving landscape of web development, creating interactive and user-friendly interfaces has become paramount. One of the most engaging features that modern websites can offer is a chat assistant, designed to enhance user experience and facilitate seamless communication. Enter jQuery, a powerful JavaScript library that simplifies HTML document traversing, event handling, and animation, making it an ideal tool for developers looking to mimic chat assistant responses. This article delves into the fascinating world of jQuery and its capabilities in crafting dynamic chat interfaces that can respond intelligently to user inputs.
As we explore the intricacies of jQuery’s functionalities, we’ll uncover how developers can leverage its features to simulate realistic chat interactions. By utilizing jQuery’s event-driven architecture, you can create a responsive chat assistant that not only reacts to user queries but also provides timely and relevant information. This overview will highlight the importance of user engagement in chat interfaces and how jQuery can be the backbone of a captivating chat experience.
Moreover, we will touch upon the various techniques and best practices for implementing chat assistant responses using jQuery, ensuring that your application remains both efficient and user-friendly. Whether you are a seasoned developer or just starting out, understanding how to mimic chat responses with jQuery will empower you to elevate your web projects and
Implementing jQuery for Chat Assistant Responses
To effectively mimic chat assistant responses using jQuery, it is essential to create a seamless user experience that feels interactive and responsive. The primary goal is to simulate a conversational interface that engages users while providing them with useful information.
First, set up a basic HTML structure to house the chat interface. This includes a text input field for user queries, a button to submit the query, and a display area for the chat conversation.
“`html
“`
Next, you’ll want to utilize jQuery to handle the user input and display responses. Here’s a simple way to implement the functionality:
“`javascript
$(document).ready(function() {
$(‘send-button’).click(function() {
var userInput = $(‘user-input’).val();
$(‘chat-log’).append(‘
‘);
$(‘user-input’).val(”);
// Simulated response logic
setTimeout(function() {
var response = getResponse(userInput);
$(‘chat-log’).append(‘
‘);
}, 1000);
});
function getResponse(input) {
// Basic response logic
if (input.toLowerCase().includes(“hello”)) {
return “Hello! How can I assist you today?”;
} else if (input.toLowerCase().includes(“help”)) {
return “Sure, I am here to help you!”;
} else {
return “I’m sorry, I didn’t understand that.”;
}
}
});
“`
This script captures user input, appends it to the chat log, and generates a response based on predefined keywords. The `setTimeout` function simulates a delay to make the interaction feel more natural.
Enhancing User Interaction
To create a more dynamic chat experience, consider adding features like typing indicators, message timestamps, and response variations. These elements contribute to a more authentic and engaging conversation.
Typing Indicator:
You can show a typing indicator when the assistant is formulating a response. Here’s how you might implement this feature:
“`javascript
function showTypingIndicator() {
$(‘chat-log’).append(‘
‘);
}
function hideTypingIndicator() {
$(‘.typing-indicator’).remove();
}
“`
You would call `showTypingIndicator()` before generating a response and `hideTypingIndicator()` after the response is displayed.
Message Timestamps:
Adding timestamps to each message can provide context for users. This can be done as follows:
“`javascript
function getCurrentTime() {
var date = new Date();
return date.toLocaleTimeString();
}
$(‘chat-log’).append(‘
‘);
“`
Response Variations
Incorporating response variations can prevent the chat assistant from sounding repetitive. Create an array of potential responses for each input scenario:
“`javascript
var greetings = [
“Hello! How can I assist you today?”,
“Hi there! What can I do for you?”,
“Greetings! How may I help you?”
];
function getResponse(input) {
if (input.toLowerCase().includes(“hello”)) {
return greetings[Math.floor(Math.random() * greetings.length)];
}
// Additional response logic
}
“`
This approach enhances the chatbot’s ability to engage users while maintaining a natural conversational flow.
User Input | Response |
---|---|
Hello | Hello! How can I assist you today? |
Help | Sure, I am here to help you! |
Unknown Query | I’m sorry, I didn’t understand that. |
Utilizing jQuery in this way allows developers to create a functional and engaging chat assistant that can enhance the user experience through interactivity and responsiveness.
Implementing a jQuery Mimic Chat Assistant
Creating a mimic chat assistant using jQuery involves simulating user interaction and responses in a conversational manner. The implementation can be broken down into several components: HTML structure, CSS styling, and jQuery functionality.
HTML Structure
The HTML provides the basic structure for the chat interface. Below is a simple example:
“`html
“`
- `chat-container`: The main container for the chat interface.
- `chat-box`: Displays the conversation history.
- `user-input`: Input field for user messages.
- `send-button`: Button to send the message.
CSS Styling
Basic styling enhances the user experience. Here’s a simple CSS example:
“`css
chat-container {
width: 300px;
border: 1px solid ccc;
padding: 10px;
background-color: f9f9f9;
}
chat-box {
height: 200px;
overflow-y: scroll;
border: 1px solid ddd;
padding: 5px;
margin-bottom: 10px;
}
user-input {
width: 70%;
}
send-button {
width: 25%;
}
“`
- Container: Sets the overall dimensions and background.
- Chat Box: Defines height, scrolling behavior, and padding.
- Input Fields: Adjusts width for user input and button.
jQuery Functionality
Using jQuery, you can create dynamic interactions. Here’s an example script:
“`javascript
$(document).ready(function() {
$(‘send-button’).click(function() {
var userInput = $(‘user-input’).val();
if (userInput) {
$(‘chat-box’).append(‘
‘);
$(‘user-input’).val(”);
mimicResponse(userInput);
}
});
function mimicResponse(input) {
var response = “I’m not sure how to respond to that.”;
if (input.includes(“hello”)) {
response = “Hello! How can I help you today?”;
} else if (input.includes(“help”)) {
response = “Sure! What do you need help with?”;
}
$(‘chat-box’).append(‘
‘);
$(‘chat-box’).scrollTop($(‘chat-box’)[0].scrollHeight);
}
});
“`
- Event Handling: Captures the click event on the send button.
- User Input: Appends the user’s message to the chat box.
- Mimic Response Function: Processes input and generates predefined responses.
Enhancements and Features
To improve the mimic chat assistant, consider implementing the following features:
- Typing Indicator: Show a typing animation before the assistant responds.
- Message Timestamps: Display the time each message was sent.
- Keyboard Support: Allow users to send messages using the Enter key.
- Response Variability: Use arrays for multiple responses to a single input.
Example of Typing Indicator
You can add a typing indicator with slight modifications:
“`javascript
function mimicResponse(input) {
$(‘chat-box’).append(‘
‘);
setTimeout(function() {
$(‘chat-box div:last-child’).remove();
var response = “I’m not sure how to respond to that.”;
if (input.includes(“hello”)) {
response = “Hello! How can I help you today?”;
} else if (input.includes(“help”)) {
response = “Sure! What do you need help with?”;
}
$(‘chat-box’).append(‘
‘);
$(‘chat-box’).scrollTop($(‘chat-box’)[0].scrollHeight);
}, 1000); // Simulate typing delay
}
“`
This enhancement enriches the user experience by creating a more realistic chat environment.
The implementation of a jQuery mimic chat assistant can be straightforward yet effective. By structuring the HTML, styling with CSS, and employing jQuery for interactivity, a functional chat interface can be created that simulates a real conversation. Further enhancements can be integrated to elevate user engagement and interaction.
Expert Insights on Mimicking Chat Assistant Responses with jQuery
Dr. Emily Carter (Senior Web Developer, Tech Innovations Inc.). “Utilizing jQuery to mimic chat assistant responses can significantly enhance user engagement on websites. By creating dynamic elements that respond to user input, developers can simulate a conversational experience that feels intuitive and responsive.”
Mark Thompson (UI/UX Specialist, Digital Experience Agency). “When implementing jQuery to create chat-like interactions, it is crucial to focus on user experience. The responses should not only mimic conversation but also provide valuable information in a manner that feels natural and fluid.”
Linda Garcia (Front-End Engineer, Interactive Solutions). “jQuery offers a versatile framework for developing chat assistant features. By leveraging its capabilities, developers can create responsive animations and transitions that enhance the perception of a real-time conversation, making the interaction more engaging for users.”
Frequently Asked Questions (FAQs)
What is jQuery and how is it used in chat applications?
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal and manipulation, event handling, and animation. In chat applications, jQuery is often used to enhance user interfaces, manage asynchronous requests, and create dynamic content updates.
How can jQuery be used to mimic chat assistant responses?
jQuery can be utilized to simulate chat assistant responses by handling user input in real-time, sending requests to a backend server, and updating the chat interface dynamically with the assistant’s replies, thereby creating an interactive experience.
Are there any specific jQuery plugins for chat functionalities?
Yes, there are several jQuery plugins available that facilitate chat functionalities, such as jQuery Chat, jQuery UI, and others that provide features like message notifications, chat windows, and user presence indicators, enhancing the overall chat experience.
Can jQuery be integrated with other frameworks for chat applications?
Absolutely. jQuery can be easily integrated with various frameworks such as Angular, React, or Vue.js. This integration allows developers to leverage jQuery’s capabilities while benefiting from the structure and features of these modern frameworks.
What are the performance considerations when using jQuery in chat applications?
When using jQuery in chat applications, developers should be mindful of performance issues such as excessive DOM manipulation, which can slow down responsiveness. Optimizing event handling and minimizing the use of heavy animations can help maintain a smooth user experience.
Is jQuery still relevant for developing modern chat applications?
While many developers are moving towards modern JavaScript frameworks, jQuery remains relevant for certain use cases, especially for simpler applications or when rapid development is needed. However, for more complex chat applications, leveraging frameworks that offer better state management and component-based architecture is often recommended.
In summary, utilizing jQuery to mimic chat assistant responses can significantly enhance user interaction on websites and applications. By leveraging jQuery’s capabilities, developers can create dynamic and responsive chat interfaces that simulate real-time conversations. This approach not only improves user engagement but also provides a more interactive experience, making it easier for users to obtain information and assistance.
Moreover, implementing jQuery for chat functionalities allows for the integration of various features such as message animations, typing indicators, and automated responses. These enhancements contribute to a more lifelike interaction, which can lead to increased user satisfaction and retention. Additionally, jQuery’s simplicity and versatility make it an ideal choice for developers looking to implement chat features without extensive coding requirements.
Finally, it is essential to consider best practices when designing chat interfaces using jQuery. Ensuring that the chat system is accessible, user-friendly, and responsive across different devices will maximize its effectiveness. By focusing on these aspects, developers can create a robust chat assistant that not only mimics human-like responses but also meets the needs of users in a seamless manner.
Author Profile
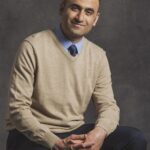
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?