How Can I Use JavaScript to Automatically Delete Form Entries?
In the fast-paced digital world, efficiency is key, especially when it comes to managing online forms. Whether you’re running a small business, a community organization, or a personal project, handling form submissions can quickly become overwhelming. This is where JavaScript comes into play, offering a powerful solution to streamline your workflow. Imagine a scenario where form entries that are no longer needed are automatically deleted, freeing you from the clutter and ensuring that your data remains relevant and manageable. In this article, we will explore how you can leverage JavaScript to automate the deletion of form entries, enhancing your data management process and improving user experience.
As online forms become an integral part of our digital interactions, the need for effective data management grows. Many users may submit forms, but not all entries are valuable or necessary over time. Manually sifting through submissions to delete outdated or irrelevant entries can be a tedious task. This is where JavaScript automation shines, allowing developers to create scripts that can intelligently remove entries based on specific criteria, such as age or status. By implementing such solutions, you can save time and resources, enabling you to focus on what truly matters: engaging with your audience and driving your objectives forward.
Moreover, automating the deletion of form entries not only enhances efficiency but also improves the
Understanding Automatic Deletion of Form Entries
Automatic deletion of form entries can significantly streamline data management in applications. This functionality helps maintain clean datasets by removing outdated or unnecessary entries. Implementing this feature in JavaScript involves setting up conditions under which entries will be deleted automatically.
Implementation Strategies
There are several strategies for automating the deletion of form entries. The choice of strategy may depend on specific requirements such as user interaction or time-based criteria.
- Time-Based Deletion: Entries can be set to delete after a certain period.
- User-Triggered Deletion: Users can specify which entries to delete, often through a button or link.
- Conditional Deletion: Entries that meet certain criteria can be automatically removed.
Example Code for Automatic Deletion
Below is a simple example of how to implement a time-based deletion mechanism using JavaScript. This script checks for entries older than a specified duration and deletes them.
“`javascript
// Assuming ‘formEntries’ is an array of entry objects with a ‘timestamp’ property
const formEntries = [
{ id: 1, data: ‘Entry 1’, timestamp: Date.now() – 86400000 }, // 1 day old
{ id: 2, data: ‘Entry 2’, timestamp: Date.now() – 3600000 }, // 1 hour old
];
const deleteOldEntries = (entries, maxAge) => {
const currentTime = Date.now();
return entries.filter(entry => (currentTime – entry.timestamp) < maxAge);
};
const maxAge = 86400000; // 1 day in milliseconds
const validEntries = deleteOldEntries(formEntries, maxAge);
console.log(validEntries);
```
This code filters entries to retain only those that are less than one day old.
Considerations for Automatic Deletion
When implementing automatic deletion of form entries, consider the following aspects:
- User Experience: Ensure that users are aware of the automatic deletion policy.
- Data Recovery: Implement a method to recover deleted entries if necessary.
- Performance: Test the performance impacts of frequent deletions on your application.
Best Practices
To ensure effective management of form entries, adhere to these best practices:
- Communicate Policies: Clearly communicate any deletion policies to users.
- Backup Data: Regularly back up data to prevent loss from automatic deletions.
- Testing: Rigorously test the deletion functionality to avoid unintended data loss.
Configuration Table
The following table outlines configurations that can be adjusted for the deletion process:
Configuration | Description | Default Value |
---|---|---|
Max Age | The maximum duration an entry can exist before deletion. | 1 day (86400000 ms) |
User Notification | Flag to notify users before deletion. | true |
Backup Frequency | Interval for backing up entries. | Daily |
By adhering to these strategies and best practices, developers can effectively implement automatic deletion of form entries, ensuring better data management and user satisfaction.
Implementing Automatic Deletion of Form Entries
To implement automatic deletion of form entries using JavaScript, you can utilize event listeners to detect specific actions, such as form submissions or timeouts. The following sections outline practical methods for achieving this.
Using JavaScript Timers
You can set up a timer to clear form entries after a certain period of inactivity. This method is useful for forms that may be left open by users.
“`javascript
let timer;
const form = document.getElementById(‘myForm’);
form.addEventListener(‘input’, () => {
clearTimeout(timer);
timer = setTimeout(() => {
form.reset(); // Resets all form fields
}, 60000); // 60 seconds
});
“`
- Event Listeners: Attach to form inputs to reset the timer on user interaction.
- Timeout: Specify a duration after which the form will be cleared.
Deleting Entries on Form Submission
Sometimes, you may want to automatically delete entries after a form is submitted. This can be particularly useful for temporary data that should not persist.
“`javascript
form.addEventListener(‘submit’, (event) => {
event.preventDefault(); // Prevents the default form submission
// Process form data here
// After processing, reset the form
form.reset();
});
“`
- Prevent Default Submission: Ensures that the form data is handled by JavaScript.
- Form Reset: Clears the entries immediately after processing.
Scheduled Deletion of Form Entries
If you want to delete form entries on a schedule, you can use `setInterval` to periodically clear the fields.
“`javascript
setInterval(() => {
form.reset(); // Clears form fields every 5 minutes
}, 300000); // 5 minutes in milliseconds
“`
- Interval Function: Sets a recurring task to reset the form.
- Custom Timing: Adjust the timing as necessary for your application’s needs.
Conditional Deletion Based on User Input
You can implement conditional logic to determine when to delete form entries based on specific criteria, such as input values.
“`javascript
const inputField = document.getElementById(‘inputField’);
inputField.addEventListener(‘input’, () => {
if (inputField.value.length === 0) {
form.reset(); // Clears form if input is empty
}
});
“`
- Input Length Check: Automatically deletes entries if certain conditions are met.
- Dynamic Behavior: Enhances user experience by providing immediate feedback.
Best Practices for Automatic Deletion
When implementing automatic deletion of form entries, consider the following best practices:
- User Notification: Inform users when their data will be deleted to avoid confusion.
- Undo Options: Offer a mechanism to restore deleted entries if necessary.
- Data Validation: Ensure that data is validated before deletion to prevent accidental loss of important information.
- Testing: Thoroughly test the functionality to ensure it behaves as expected across different scenarios.
Utilizing JavaScript for the automatic deletion of form entries can enhance the user experience and maintain data integrity. By implementing the methods described, you can create a more efficient and user-friendly form handling process.
Expert Insights on Automating Form Entry Deletion with JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Implementing JavaScript to automatically delete form entries can significantly enhance user experience by reducing clutter. However, it is crucial to ensure that users are informed about the deletion process to avoid confusion or data loss.”
Michael Thompson (Web Development Specialist, CodeCraft Academy). “While automating the deletion of form entries using JavaScript can streamline data management, developers must prioritize security measures. Proper validation and user confirmation are essential to prevent accidental deletions.”
Linda Garcia (UX/UI Designer, User Experience Lab). “From a design perspective, automating the deletion of form entries should be intuitive. Users should have clear options to undo actions or restore entries, ensuring that the interface remains user-friendly and accessible.”
Frequently Asked Questions (FAQs)
What is the purpose of using JavaScript to automatically delete form entries?
JavaScript can be used to enhance user experience by automatically clearing form entries after submission or after a specified time, ensuring that users do not accidentally resubmit data or retain outdated information.
How can I implement automatic deletion of form entries using JavaScript?
You can implement automatic deletion by using event listeners on form submission or input fields, combined with functions that reset the form or clear specific fields after a defined action occurs.
Is it possible to set a timer for automatic deletion of form entries?
Yes, you can use the `setTimeout` function in JavaScript to clear form entries after a specified period, allowing for automatic deletion without user intervention.
Are there any potential drawbacks to automatically deleting form entries?
Automatic deletion can lead to user frustration if not implemented thoughtfully, especially if users need time to review their entries. It’s essential to provide clear feedback or confirmation before deletion occurs.
Can I restrict automatic deletion to certain fields in the form?
Yes, you can target specific fields for automatic deletion by selecting them individually in your JavaScript code, allowing for more control over which entries are cleared.
How do I ensure that automatic deletion does not interfere with form validation?
To prevent conflicts, ensure that the automatic deletion logic is executed only after successful validation. This can be achieved by integrating the deletion function within the validation callback or event handlers.
In summary, utilizing JavaScript to automatically delete form entries can significantly enhance user experience and data management. This functionality can be implemented through various methods, such as setting timeouts for input fields or providing a clear button that resets the form. By automating the deletion process, developers can ensure that forms remain clean and prevent the accumulation of outdated or irrelevant data.
Moreover, the implementation of such features can lead to improved data integrity and usability. Users are less likely to submit forms with stale information, and the overall efficiency of data collection processes can be increased. Additionally, providing feedback mechanisms, such as alerts or notifications when entries are deleted, can further engage users and clarify the actions taken on their input.
incorporating JavaScript for automatic deletion of form entries not only streamlines the data entry process but also fosters a more organized and user-friendly environment. As web applications continue to evolve, leveraging such techniques will be essential for maintaining high standards of data management and user satisfaction.
Author Profile
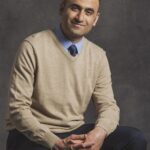
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?