How Can I Convert a JSON String to a JSON Object in Java?
In the world of software development, data interchange formats play a crucial role in enabling seamless communication between systems. Among these formats, JSON (JavaScript Object Notation) has emerged as a favorite due to its simplicity and readability. Java developers, in particular, often find themselves needing to convert JSON strings into JSON objects for various applications, whether it’s for processing API responses, storing configurations, or manipulating data structures. Understanding how to effectively perform this conversion is essential for any Java programmer looking to harness the full power of JSON in their applications.
Converting a JSON string to a JSON object in Java is a straightforward yet vital task that involves parsing the string representation of data into a usable object format. This process not only allows developers to access and manipulate the data more efficiently but also ensures that the data adheres to the expected structure and types defined within the JSON format. Java offers several libraries, such as Jackson, Gson, and org.json, each providing unique features and capabilities that facilitate this conversion.
As we delve deeper into this topic, we’ll explore the various libraries available for JSON processing in Java, the steps involved in converting a JSON string to a JSON object, and best practices to consider during implementation. Whether you’re a seasoned Java developer or just starting your journey, mastering this conversion process will
Understanding JSON Strings and JSON Objects
In Java, a JSON string is a textual representation of data structured in JavaScript Object Notation (JSON). This format is widely used for data interchange between a server and a web application. A JSON object, on the other hand, is a data structure that represents the same information but in a format that Java can manipulate programmatically.
To convert a JSON string into a JSON object, Java developers commonly utilize libraries such as Jackson or Gson. Both libraries provide methods to parse JSON strings and create corresponding Java objects or data structures.
Using Jackson to Convert JSON String to JSON Object
Jackson is a powerful library that allows for easy data binding between JSON and Java objects. Here’s how to use it:
- Add Jackson Dependency: First, include the Jackson library in your project. For Maven, add the following dependency to your `pom.xml`:
“`xml
“`
- Convert JSON String: Use the `ObjectMapper` class to convert a JSON string to a JSON object.
“`java
import com.fasterxml.jackson.databind.ObjectMapper;
import java.util.Map;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
Map
System.out.println(jsonObject);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Using Gson to Convert JSON String to JSON Object
Gson is another popular library for converting Java objects to JSON and vice versa. The steps to convert a JSON string to a JSON object using Gson are as follows:
- Add Gson Dependency: For Maven projects, add the Gson dependency:
“`xml
“`
- Convert JSON String: Use the `Gson` class to perform the conversion.
“`java
import com.google.gson.Gson;
import java.util.Map;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
Gson gson = new Gson();
Map
System.out.println(jsonObject);
}
}
“`
Comparison of Jackson and Gson
When choosing between Jackson and Gson, consider the following aspects:
Feature | Jackson | Gson |
---|---|---|
Performance | Generally faster for large data sets | Good performance but may lag behind Jackson |
Data Binding | Supports data binding with annotations | Simple and easy data binding |
Streaming API | Provides a powerful streaming API | Basic streaming support |
Customization | Highly customizable through modules | Less customizable compared to Jackson |
Both libraries are effective for converting JSON strings to JSON objects, and the choice often comes down to specific project requirements and personal preference.
Converting JSON String to JSON Object in Java
To convert a JSON string into a JSON object in Java, developers commonly utilize libraries such as Jackson, Gson, or org.json. Each library offers straightforward methods for parsing JSON strings and constructing JSON objects.
Using Jackson Library
Jackson is a widely-used library for processing JSON in Java. Here’s how to convert a JSON string to a JSON object using Jackson:
- Add Jackson Dependency: If you are using Maven, include the following in your `pom.xml`:
“`xml
“`
- Code Example:
“`java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
ObjectMapper objectMapper = new ObjectMapper();
try {
JsonNode jsonObject = objectMapper.readTree(jsonString);
System.out.println(jsonObject.get(“name”).asText()); // Outputs: John
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Using Gson Library
Gson is another popular library for converting JSON to Java objects. To use Gson for this conversion:
- Add Gson Dependency: Include the following in your `pom.xml`:
“`xml
“`
- Code Example:
“`java
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JsonObject jsonObject = JsonParser.parseString(jsonString).getAsJsonObject();
System.out.println(jsonObject.get(“name”).getAsString()); // Outputs: John
}
}
“`
Using org.json Library
The org.json library provides simple methods to handle JSON data in Java. To convert a JSON string to a JSON object using this library:
- Add org.json Dependency: If using Maven, add the following dependency:
“`xml
“`
- Code Example:
“`java
import org.json.JSONObject;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject.getString(“name”)); // Outputs: John
}
}
“`
Choosing the Right Library
When deciding which library to use, consider the following factors:
Factor | Jackson | Gson | org.json |
---|---|---|---|
Performance | High | Moderate | Moderate |
Features | Advanced features, streaming | Simple and easy to use | Basic functionality |
Learning Curve | Steeper due to features | Easier for beginners | Simple API |
Size | Larger due to features | Moderate | Small |
Selecting the right library depends on your project requirements and the complexity of the JSON data being handled.
Transforming JSON Strings to JSON Objects in Java: Expert Insights
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Converting a JSON string to a JSON object in Java is a straightforward process, especially with libraries like Jackson and Gson. These libraries provide robust methods to parse JSON strings efficiently, allowing developers to focus on application logic rather than data handling.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “When dealing with JSON in Java, it is crucial to choose the right library based on your project requirements. Gson is excellent for simple tasks, while Jackson offers more advanced features such as streaming and data binding, which can significantly enhance performance for larger datasets.”
Lisa Patel (Technical Architect, FutureTech Systems). “Proper error handling during the conversion of JSON strings to JSON objects cannot be overlooked. Implementing try-catch blocks ensures that your application can gracefully handle malformed JSON, which is a common issue in real-world applications.”
Frequently Asked Questions (FAQs)
What is the difference between a JSON string and a JSON object in Java?
A JSON string is a text representation of data formatted in JSON (JavaScript Object Notation), while a JSON object is a data structure that represents the JSON data in a form that can be manipulated programmatically in Java.
How can I convert a JSON string to a JSON object in Java?
You can convert a JSON string to a JSON object in Java using libraries such as `org.json`, `Gson`, or `Jackson`. For example, with `org.json`, you would use `JSONObject jsonObject = new JSONObject(jsonString);`.
What libraries are commonly used for JSON parsing in Java?
Common libraries for JSON parsing in Java include `org.json`, `Gson` by Google, and `Jackson`. Each library has its own methods and features for handling JSON data.
Is it necessary to handle exceptions when converting a JSON string to a JSON object?
Yes, it is necessary to handle exceptions, as the conversion process can throw exceptions such as `JSONException` if the JSON string is malformed or invalid. Proper exception handling ensures robust code.
Can I convert a JSON string directly into a Java object?
Yes, you can convert a JSON string directly into a Java object using libraries like `Gson` or `Jackson`. For instance, with `Gson`, you would use `MyClass obj = gson.fromJson(jsonString, MyClass.class);`.
What should I do if my JSON string contains nested objects?
If your JSON string contains nested objects, ensure your Java classes are structured to match the JSON hierarchy. Use appropriate data types and annotations (if applicable) to facilitate the conversion process.
In Java, converting a JSON string to a JSON object is a common task that can be accomplished using various libraries, with the most popular being Jackson and Gson. These libraries provide straightforward methods for parsing JSON strings and converting them into usable Java objects or JSON objects. The choice of library often depends on the specific requirements of the project, such as performance, ease of use, or the need for advanced features.
Both Jackson and Gson offer robust APIs that simplify the process of deserialization. For instance, with Jackson, one can utilize the `ObjectMapper` class to read a JSON string and convert it into a `JsonNode` or any other Java object. Similarly, Gson provides a `Gson` class that can parse a JSON string into a `JsonObject` or a custom Java class. These libraries handle various data types and structures, making them versatile tools for developers.
understanding how to convert a JSON string to a JSON object in Java is essential for developers working with web services, APIs, or any application that involves data interchange in JSON format. By leveraging libraries like Jackson or Gson, developers can efficiently manage JSON data, ensuring their applications can easily read and manipulate structured information. This knowledge not only enhances coding efficiency
Author Profile
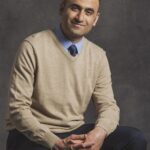
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?