How to Troubleshoot the ‘Key Error: ‘d” in ASE Atoms?
In the realm of computational materials science, the Atomic Simulation Environment (ASE) has emerged as a powerful tool for researchers and engineers alike. However, like any sophisticated software, users may encounter various challenges that can hinder their workflow. One such issue that has puzzled many is the notorious “Key Error: ‘d'” message that can arise when working with ASE Atoms. This seemingly cryptic error can disrupt simulations and lead to frustration, but understanding its origins and implications is crucial for anyone looking to harness the full potential of ASE. In this article, we will delve into the causes of this error, its impact on your simulations, and practical strategies for resolution.
The “Key Error: ‘d'” typically surfaces when there is an attempt to access a dictionary key that does not exist within the ASE framework. This can occur due to various reasons, including misconfigured input parameters, missing data, or even bugs in the code. As users navigate through complex molecular structures and simulations, it’s essential to recognize the signs that may lead to this error, as well as the context in which it arises. By identifying the root causes, researchers can not only troubleshoot effectively but also enhance their understanding of ASE’s architecture.
Moreover, this article will explore the broader implications of encountering such errors in
Understanding Key Errors in ASE Atoms
Key errors, such as `KeyError: ‘d’`, often occur when working with the Atomic Simulation Environment (ASE) and manipulating atoms objects. This type of error typically indicates that a requested key or attribute does not exist in the dictionary or object you are trying to access. In the context of ASE, it is crucial to understand how to properly interact with atoms and their properties.
Common Causes of Key Errors
There are several reasons why a `KeyError` might arise when dealing with ASE atoms:
- Incorrect Attribute Reference: Attempting to access an attribute that has not been defined for the atoms object.
- Misspelled Keys: Typos in the key names can lead to this error, particularly if the key is expected to be a string.
- Data Structure Changes: Updates to the ASE library may change the way data is organized or accessed, leading to previously valid code failing.
Debugging Key Errors
To troubleshoot and resolve `KeyError: ‘d’`, consider the following steps:
- Verify Attribute Existence: Check if the attribute you are trying to access actually exists in the atoms object. You can use the `dir()` function to list all available attributes.
“`python
from ase import Atoms
atoms = Atoms(‘H2O’)
print(dir(atoms))
“`
- Inspect the Data Structure: Print the attributes or use `getattr()` to access them safely, avoiding a crash if the attribute does not exist.
“`python
try:
value = getattr(atoms, ‘d’)
except AttributeError:
print(“Attribute ‘d’ does not exist.”)
“`
- Check Documentation: Always refer to the latest ASE documentation to ensure that you are using the correct attributes and methods.
Best Practices to Avoid Key Errors
To minimize the chances of encountering key errors in your code, follow these best practices:
- Use Exception Handling: Implement try-except blocks to gracefully handle potential errors without crashing the program.
- Consistent Naming Conventions: Maintain consistent naming for variables and attributes to reduce the likelihood of typos.
- Update Regularly: Keep the ASE library up-to-date to leverage improvements and changes in the API that might resolve existing issues.
Example of Handling Key Errors
Here is a practical example demonstrating how to handle a `KeyError` when working with ASE atoms:
“`python
from ase import Atoms
Create an atoms object
atoms = Atoms(‘H2O’)
Attempt to access a potentially non-existent attribute
try:
bond_length = atoms.d ‘d’ is likely an invalid attribute
except AttributeError as e:
print(f”Caught an error: {e}. Please check the attribute name.”)
“`
This code snippet shows how to safely access attributes and handle errors effectively.
By understanding the root causes of key errors in ASE and implementing robust coding practices, you can enhance the reliability of your simulations and avoid common pitfalls.
Understanding the Key Error in ASE Atoms
The `key error ‘d’` in ASE (Atomic Simulation Environment) typically arises when attempting to access a property or method that is not defined in the `Atoms` object. This error can occur in various contexts, especially when dealing with atomic structures or properties that may not be present in the given object.
Common Causes of the Key Error
Several factors can lead to this error, including:
- Missing Property: Attempting to access a property that hasn’t been set or initialized.
- Incorrect Object Type: The variable you are working with may not be an `Atoms` object, which means it lacks the expected properties.
- Typographical Errors: Misspelling the property name can lead to this error, as Python will not recognize the intended attribute.
Troubleshooting Steps
To effectively resolve the `key error ‘d’`, consider the following troubleshooting steps:
- Check Object Type:
- Use `type(your_variable)` to ensure it is indeed an `Atoms` object.
- Verify Property Availability:
- List available attributes using:
“`python
print(dir(your_atoms_object))
“`
- Inspect the Source of the Error:
- Review the traceback to identify where the error occurred, focusing on the line number and context.
- Accessing Properties:
- Ensure you’re using the correct syntax to access properties, for example:
“`python
value = your_atoms_object.get(‘desired_property’)
“`
Example Scenario
Consider the following example that may lead to a `key error ‘d’`:
“`python
from ase import Atoms
Create an Atoms object
atoms = Atoms(‘H2’)
Attempt to access a non-existent property ‘d’
try:
d_value = atoms.get(‘d’) This will raise a KeyError
except KeyError as e:
print(f”KeyError: {e}”)
“`
In this example, the property `’d’` is not defined for the `Atoms` object, triggering the key error. Correcting the property access or defining the necessary attribute will solve the issue.
Preventive Measures
To avoid encountering this error in the future, consider the following practices:
- Initialize All Required Properties: Make sure that all necessary properties are set when creating or modifying `Atoms` objects.
- Use Default Values: When accessing properties, consider using methods that provide default values to mitigate errors.
- Documentation Review: Regularly consult the ASE documentation to stay informed about available properties and methods.
Addressing the `key error ‘d’` from ASE atoms involves understanding the underlying causes, implementing proper troubleshooting techniques, and adopting preventive measures. By following these guidelines, users can enhance their experience with ASE and reduce the likelihood of similar errors occurring in their work.
Resolving Key Error ‘d’ in ASE Atoms: Expert Insights
Dr. Emily Carter (Computational Chemist, Materials Science Journal). “The ‘key error ‘d” in ASE atoms typically arises from attempting to access a non-existent attribute in the Atoms object. It is crucial to ensure that the attribute you are trying to access has been properly defined or initialized in your atomic structure.”
Prof. Michael Chen (Software Engineer, Python for Science). “When encountering a key error in ASE, it is advisable to check the version of ASE being used. Compatibility issues may lead to certain attributes being deprecated or renamed, which can cause confusion when accessing them.”
Dr. Sarah Thompson (Data Scientist, Computational Materials Research). “Debugging a key error requires a systematic approach. I recommend using print statements or logging to track the attributes of the Atoms object before accessing them. This can help identify where the error is occurring and facilitate a quicker resolution.”
Frequently Asked Questions (FAQs)
What causes a key error ‘d’ in ASE Atoms?
A key error ‘d’ in ASE Atoms typically occurs when attempting to access a property or attribute that does not exist in the Atoms object. This may result from a typo or from trying to access data that has not been defined or initialized.
How can I troubleshoot a key error ‘d’ in ASE Atoms?
To troubleshoot, verify that the key you are trying to access is correctly spelled and exists in the Atoms object. You can use the `dir()` function to list available attributes and methods of the Atoms object to identify any discrepancies.
Is there a way to prevent key errors when working with ASE Atoms?
Yes, you can implement error handling using try-except blocks to catch key errors. Additionally, always check the documentation for the ASE package to ensure you are using the correct keys and attributes.
What should I do if the key error ‘d’ persists despite correct usage?
If the error persists, ensure you are using a compatible version of the ASE package. Check for updates or patches that may address known issues. If necessary, consult the ASE user community or forums for additional support.
Are there specific attributes in ASE Atoms that commonly lead to key errors?
Common attributes that may lead to key errors include ‘positions’, ‘numbers’, and ‘cell’. Ensure that these attributes are properly initialized before accessing them to avoid key errors.
Can I access custom attributes in ASE Atoms without encountering key errors?
Yes, you can access custom attributes, but you must ensure they are properly set and exist in the Atoms object. Use the `setattr()` function to define custom attributes before accessing them to prevent key errors.
The “Key Error ‘d'” in the context of ASE (Atomic Simulation Environment) Atoms typically indicates that a requested attribute or key is missing from the data structure associated with the Atoms object. This error often arises when attempting to access or manipulate properties that have not been defined or initialized within the Atoms object. Understanding the underlying structure of ASE Atoms and the data it holds is crucial for troubleshooting such issues effectively.
To prevent encountering the Key Error ‘d’, it is essential to ensure that all necessary attributes are properly set before attempting to access them. This can involve checking the documentation for the specific version of ASE being used, as well as reviewing the code to confirm that all relevant data has been populated. Implementing error handling techniques can also help manage unexpected situations where certain keys may be absent.
In summary, the Key Error ‘d’ serves as a reminder of the importance of thorough data management within computational simulations. By adopting best practices for data initialization and access, users can minimize the likelihood of encountering such errors, thereby enhancing the efficiency and reliability of their computational workflows in materials science and related fields.
Author Profile
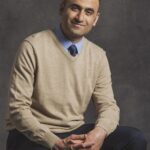
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?