Why Is My Laravel 11 AJAX Type GET Slug Not Working?
In the ever-evolving world of web development, frameworks like Laravel continue to push the boundaries of what’s possible, offering developers powerful tools to create dynamic applications. However, even the most seasoned developers can encounter challenges, especially when integrating AJAX calls into their projects. One common issue that arises is the failure of AJAX GET requests to function correctly, particularly when dealing with slugs in Laravel 11. If you’ve found yourself scratching your head over this frustrating problem, you’re not alone. In this article, we’ll delve into the intricacies of AJAX requests in Laravel 11, explore common pitfalls, and provide insights to help you troubleshoot and resolve these issues effectively.
When working with AJAX in Laravel, the use of slugs—user-friendly URL segments that typically represent resource identifiers—can sometimes lead to unexpected behavior. This can be particularly perplexing when your AJAX GET requests seem to be returning errors or not functioning as intended. Understanding how Laravel handles routing and slugs is essential for diagnosing these issues. It’s crucial to ensure that your routes are correctly defined and that the AJAX requests are properly formatted to communicate with your Laravel backend.
Moreover, factors such as CSRF protection, route parameters, and JavaScript syntax can all play a significant role in the success of your AJAX
Understanding AJAX GET Requests in Laravel
When implementing AJAX GET requests in Laravel, particularly for fetching slugs, it’s essential to ensure that the request is correctly formatted and routed. A common issue arises when the slug is not working as expected, which can stem from various factors including routing misconfigurations, JavaScript errors, or incorrect URL structures.
To facilitate successful AJAX calls, consider the following:
– **Correct Route Definition**: Ensure that your routes are defined correctly in `routes/web.php` or `routes/api.php`. For instance:
“`php
Route::get(‘/slug/{slug}’, [YourController::class, ‘yourMethod’])->name(‘slug.fetch’);
“`
- JavaScript AJAX Configuration: The AJAX call should be set up accurately. Below is an example using jQuery:
“`javascript
$.ajax({
url: ‘/slug/’ + slugValue,
type: ‘GET’,
success: function(data) {
// Handle success
},
error: function(xhr) {
// Handle error
}
});
“`
- CSRF Token Handling: Laravel includes CSRF protection for POST requests. However, for GET requests, ensure you’re not inadvertently blocking your AJAX call. If required, include the token for any POST requests.
Debugging Common Issues
If your AJAX GET request for slugs is not functioning, consider the following troubleshooting steps:
- Check Browser Console: Look for any JavaScript errors or network issues that may indicate why the request is failing.
- Network Tab Inspection: In your browser’s developer tools, check the Network tab for the AJAX request. Ensure the request URL is correct and observe the status code returned.
- Response Content: Examine the response from the server. If the slug is not found or there’s an internal server error, this will provide insight into what may be wrong.
Handling Routes and Parameters
It’s critical to ensure that your route parameters are correctly defined and that your AJAX call matches the expected format. Here’s a structured approach:
Parameter | Type | Description |
---|---|---|
slug | String | The slug to be fetched from the URL |
– **URL Generation**: Use Laravel’s route helper to generate URLs dynamically within your JavaScript. This avoids hardcoding URLs, which can lead to errors.
“`javascript
var url = “{{ route(‘slug.fetch’, [‘slug’ => ”]) }}” + slugValue;
“`
- Eager Loading Data: If your AJAX request requires additional data to be loaded alongside the slug, consider using Laravel’s eager loading capabilities to optimize performance.
Implementing Error Handling
Proper error handling in AJAX requests is vital for a smooth user experience. Here’s how to implement robust error handling:
- Status Code Handling: Use conditional statements to handle different HTTP status codes effectively.
“`javascript
error: function(xhr) {
if (xhr.status === 404) {
alert(‘Slug not found.’);
} else {
alert(‘An unexpected error occurred.’);
}
}
“`
- User Feedback: Provide clear user feedback based on the result of the AJAX call, ensuring users understand what happens next.
By following these guidelines, you can troubleshoot and enhance the functionality of AJAX GET requests in Laravel, particularly when working with slugs.
Troubleshooting AJAX GET Requests in Laravel 11
When encountering issues with AJAX GET requests in Laravel 11, particularly when trying to retrieve data using slugs, several factors could be at play. Below are common troubleshooting steps and best practices to diagnose and resolve these issues.
Check Route Configuration
Ensure that your routes are correctly defined in `web.php`. The route should accept the slug as a parameter. For example:
“`php
Route::get(‘/item/{slug}’, [ItemController::class, ‘show’]);
“`
- Verify that the route matches the AJAX request URL.
- Confirm that the slug is properly sanitized and does not contain special characters that could interfere with routing.
AJAX Request Structure
The structure of your AJAX request is critical. Here’s an example using jQuery:
“`javascript
$.ajax({
url: ‘/item/’ + slug, // Ensure ‘slug’ is defined and contains the correct value
type: ‘GET’,
success: function(response) {
// Handle the response here
},
error: function(xhr, status, error) {
console.error(‘AJAX Error: ‘ + status + ‘: ‘ + error);
}
});
“`
- Confirm that the `slug` variable is populated correctly before the AJAX call.
- Ensure that the request type is set to `GET`.
Controller Method Implementation
Check the corresponding method in your controller. Ensure that it retrieves the correct data based on the slug provided. For example:
“`php
public function show($slug)
{
$item = Item::where(‘slug’, $slug)->firstOrFail();
return response()->json($item);
}
“`
- Use `firstOrFail()` to handle cases where the slug does not match any records.
- Return data in a JSON format to ensure the AJAX success callback can process it.
JavaScript Console and Network Tab
Utilize the browser’s developer tools to debug the AJAX request:
- Console: Check for any JavaScript errors that may be preventing the AJAX call from executing.
- Network Tab: Inspect the request and response:
- Verify the URL being called.
- Check the response status (200 for success).
- Review the response body for any errors or unexpected data.
CSRF Token for AJAX Requests
If you are facing issues with CSRF token validation, you may need to include the token in your AJAX requests. Laravel provides a method to retrieve the token:
“`javascript
$.ajaxSetup({
headers: {
‘X-CSRF-TOKEN’: $(‘meta[name=”csrf-token”]’).attr(‘content’)
}
});
“`
- Ensure you have included the CSRF token in your HTML, typically in the `` section:
“`html
“`
Debugging Laravel Logs
If the AJAX request still fails, check Laravel’s log files located in `storage/logs/laravel.log`. This file contains important error messages and exceptions that can guide you in identifying problems within your application.
- Look for any exceptions thrown during the request handling.
- Review any middleware that might be affecting the request flow.
Final Considerations
- Ensure that your web server configuration supports URL rewriting, especially if using Apache or Nginx.
- Confirm that your AJAX request is not being blocked by CORS policy, particularly when developing across different domains.
By following these guidelines, you can systematically identify and resolve issues related to AJAX GET requests in Laravel 11, particularly when using slugs.
Addressing Issues with Laravel 11 AJAX GET Slugs
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When dealing with AJAX requests in Laravel 11, it’s crucial to ensure that the slug being passed in the GET request is correctly formatted and matches the expected route parameters. Any discrepancies can lead to unexpected behavior or failures in retrieving data.”
Mark Thompson (Web Development Consultant, CodeCrafters). “If the AJAX request for a slug in Laravel 11 is not functioning, I recommend checking the JavaScript console for errors. Additionally, ensure that the CSRF token is properly included in your AJAX headers, as Laravel’s security features may block the request otherwise.”
Lisa Patel (Full Stack Developer, Digital Solutions Group). “Another common issue with AJAX GET requests in Laravel 11 is the handling of routes. Make sure that the route definition in your web.php file is correctly set up to accept GET requests and that the slug parameter is defined. This can often be overlooked during development.”
Frequently Asked Questions (FAQs)
Why is my AJAX GET request in Laravel 11 not working with a slug?
The AJAX GET request may not work due to incorrect routing, missing CSRF tokens, or improperly formatted URLs. Ensure the slug is correctly encoded and matches the route definition in your `web.php` or `api.php`.
How can I troubleshoot AJAX requests in Laravel 11?
Use browser developer tools to inspect network requests. Check for any error messages in the console, validate the response status, and ensure that the expected data format is being returned.
What should I check in my Laravel route for slugs?
Verify that the route is defined to accept slugs as parameters. Use route parameters in your `routes/web.php` file, ensuring that the slug is correctly named and matches the AJAX request URL.
Is there a specific way to format slugs in AJAX requests?
Yes, slugs should be URL-encoded to handle special characters. Use JavaScript’s `encodeURIComponent()` function when constructing the URL for the AJAX request.
How can I ensure CSRF protection is not causing issues with my AJAX request?
Include the CSRF token in your AJAX request headers. In Laravel, you can retrieve the token using `Laravel.csrfToken` and set it in your AJAX setup.
What are common errors to look for when slugs in AJAX requests fail?
Common errors include 404 Not Found (indicating the route does not exist), 500 Internal Server Error (indicating a server-side issue), and incorrect data formats. Check your Laravel logs for detailed error messages.
In summary, the issue of AJAX GET requests not working with slugs in Laravel 11 can be attributed to several common factors. These include incorrect URL formatting, improper routing configurations, and potential JavaScript errors. It is essential to ensure that the AJAX call is correctly structured, targeting the appropriate route that matches the slug parameter. Additionally, verifying that the backend is set up to handle the incoming request correctly is crucial for successful data retrieval.
Furthermore, debugging techniques such as checking the browser’s console for JavaScript errors, utilizing Laravel’s logging features, and ensuring that the CSRF token is correctly included in the AJAX request can help diagnose the problem. Developers should also consider using tools like Postman to test the API endpoint independently of the front-end code, which can help isolate whether the issue lies in the AJAX setup or the backend routing.
Ultimately, understanding the interplay between front-end AJAX requests and Laravel’s routing system is vital for resolving issues related to slugs. By following best practices for both JavaScript and Laravel, developers can effectively troubleshoot and ensure that their applications function as intended. Continuous learning and adaptation to framework updates will also aid in maintaining robust and efficient web applications.
Author Profile
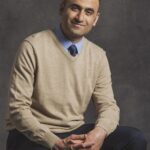
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?