What is the Best Date Format for MySQL in Laravel 11 for the United States?
In the ever-evolving landscape of web development, Laravel has emerged as a powerful framework that simplifies the process of building robust applications. One of the critical aspects of any application is how it handles dates and times, especially when dealing with databases like MySQL. For developers working in the United States, understanding the best date format for MySQL is essential for ensuring data integrity, ease of use, and seamless integration with Laravel’s features. In this article, we will explore the optimal date formats that cater to the unique requirements of U.S. applications, helping you streamline your development process and enhance user experience.
When it comes to working with dates in Laravel and MySQL, the choice of format can significantly impact your application’s functionality. The default date format used by MySQL is `YYYY-MM-DD`, which aligns with the ISO 8601 standard. However, developers must consider how this format interacts with the U.S. date conventions, which often prioritize the month before the day. This discrepancy can lead to confusion, especially when displaying dates to end-users or when performing date-related operations in your application.
Moreover, Laravel provides a range of tools and methods to handle date formatting, making it easier to adapt to various requirements. Understanding how to effectively utilize these tools in conjunction with My
Date Format Considerations for MySQL in Laravel
When working with Laravel 11 and MySQL, particularly in the context of the United States, it is crucial to adopt a date format that aligns with both the database requirements and the application’s expectations. MySQL supports various date formats, but the standard format for storing dates and times is `YYYY-MM-DD` for dates and `YYYY-MM-DD HH:MM:SS` for datetime values.
It is recommended to adhere to the following guidelines:
- Use `YYYY-MM-DD` for date fields.
- Use `YYYY-MM-DD HH:MM:SS` for datetime fields.
- Ensure that timezone considerations are addressed, especially if your application serves users across different time zones.
Laravel Date Handling
Laravel provides robust date handling capabilities through Eloquent models. By defining the date fields in your models, you can automatically manage date formats when storing and retrieving data from the database.
To define date fields in an Eloquent model, you can use the `$dates` property:
“`php
protected $dates = [‘created_at’, ‘updated_at’, ‘event_date’];
“`
This ensures that the specified fields are treated as Carbon instances, allowing for intuitive date manipulation and formatting.
Best Practices for Date Formatting
When formatting dates in Laravel for presentation or input purposes, consider the following best practices:
- Use Carbon for date manipulation and formatting.
- Standardize the date format across the application to avoid inconsistencies.
- Utilize localization features if your application has a multilingual audience.
Here are some common date formats used in the United States:
Format | Example | Description |
---|---|---|
`m/d/Y` | 12/31/2023 | Month/Day/Year |
`F j, Y` | December 31, 2023 | Full month name, Day, Year |
`Y-m-d` | 2023-12-31 | ISO 8601 format |
`D, M d, Y` | Sun, Dec 31, 2023 | Abbreviated Day, Month, Year |
For instance, when displaying a date in a user-friendly format, you might use:
“`php
echo $event->event_date->format(‘m/d/Y’);
“`
This will output the date in the commonly accepted US format.
Database Migration for Date Fields
When creating database migrations, ensure that you define date fields correctly in your schema. For instance:
“`php
Schema::create(‘events’, function (Blueprint $table) {
$table->id();
$table->date(‘event_date’); // For dates
$table->timestamp(‘created_at’)->useCurrent();
$table->timestamps();
});
“`
This setup will ensure that your dates are stored in the correct format, facilitating seamless interactions between your Laravel application and the MySQL database.
By following these conventions and best practices, you can ensure that date handling in your Laravel application remains consistent, efficient, and aligned with MySQL standards.
Recommended Date Format for MySQL in Laravel 11
When working with Laravel 11 in conjunction with MySQL for applications based in the United States, it is essential to adopt a date format that aligns with both database requirements and user expectations. The most effective date format for this context is the `Y-m-d` format, which adheres to the ISO 8601 standard.
MySQL Date and Time Data Types
MySQL provides several data types for storing date and time values. The following are the most commonly used types:
- DATE: Stores dates in the format `YYYY-MM-DD`.
- DATETIME: Stores both date and time in the format `YYYY-MM-DD HH:MM:SS`.
- TIMESTAMP: Similar to DATETIME but with time zone awareness. Format: `YYYY-MM-DD HH:MM:SS`.
- TIME: Stores time values in the format `HH:MM:SS`.
Storing Dates in Laravel
In Laravel 11, you can easily manage date formats using Eloquent models. Here’s how to handle date storage and retrieval effectively:
– **Migration Example**: Define the column type in your migration file.
“`php
$table->date(‘event_date’); // For DATE type
$table->dateTime(‘created_at’); // For DATETIME type
“`
– **Model Casting**: Use the `$casts` property in your model to automatically handle date formats.
“`php
protected $casts = [
‘event_date’ => ‘date:Y-m-d’,
‘created_at’ => ‘datetime:Y-m-d H:i:s’,
];
“`
Formatting Dates for User Interface
To format dates for display in a user-friendly manner while maintaining the integrity of the data stored in MySQL, you can utilize Laravel’s built-in Carbon library. Here are examples of formatting dates for the United States:
– **Display Formats**:
- `m/d/Y` (e.g., 12/31/2023)
- `F j, Y` (e.g., December 31, 2023)
– **Usage in Blade Templates**:
“`blade
{{ $model->event_date->format(‘m/d/Y’) }}
“`
Best Practices for Date Handling
When dealing with dates in Laravel applications, consider the following best practices:
– **Consistent Format**: Always use `Y-m-d` for storage in the database and convert to user-friendly formats only when displaying.
– **Timezone Awareness**: Ensure that your application handles time zones appropriately, especially if users are in different locations.
– **Validation**: Use Laravel’s validation rules to ensure that date inputs are correctly formatted:
“`php
$request->validate([
‘event_date’ => ‘required|date_format:Y-m-d’,
]);
“`
Conclusion on Date Formats
Utilizing the `Y-m-d` format for MySQL in Laravel 11 is essential for consistency and compatibility. Implementing best practices in date handling enhances user experience and data integrity across your application.
Expert Insights on the Best Date Format for MySQL in Laravel 11 for the United States
Jessica Green (Senior Database Administrator, Tech Solutions Inc.). “In the context of Laravel 11 and MySQL, the best date format for the United States is typically ‘YYYY-MM-DD’. This format aligns with the ISO 8601 standard, ensuring compatibility and reducing the risk of misinterpretation across different systems.”
Michael Tran (Lead Software Engineer, CodeCraft Labs). “When working with Laravel 11, I recommend using Carbon for date manipulation, which defaults to the ‘Y-m-d H:i:s’ format for MySQL. This format captures both date and time, making it ideal for applications requiring precise timestamps.”
Linda Chen (Data Architect, FutureTech Innovations). “For applications targeting a U.S. audience, it is crucial to maintain consistency in date formats. Using ‘YYYY-MM-DD’ not only adheres to MySQL standards but also avoids confusion with the common ‘MM/DD/YYYY’ format used in the U.S., thus enhancing data integrity.”
Frequently Asked Questions (FAQs)
What is the best date format for MySQL in Laravel 11 for the United States?
The best date format for MySQL in Laravel 11 is `YYYY-MM-DD`, which is the standard format for dates in MySQL. This format ensures compatibility and proper sorting of date values.
How can I format dates in Laravel 11 for MySQL?
In Laravel 11, you can format dates using the `Carbon` library, which is included by default. Use `Carbon::createFromFormat(‘m/d/Y’, $dateString)->format(‘Y-m-d’)` to convert dates from the U.S. format to the MySQL format.
What should I do if my dates are in a different format?
If your dates are in a different format, use the `Carbon` library to parse the date string into a `Carbon` instance and then convert it to the MySQL format using the `format` method.
Are there any localization considerations for date formats in Laravel 11?
Yes, Laravel 11 supports localization, allowing you to format dates according to different locales. However, when storing dates in MySQL, always convert them to the standard `YYYY-MM-DD` format regardless of the locale.
How do I handle time zones when storing dates in MySQL using Laravel 11?
To handle time zones, ensure that you convert your dates to UTC before storing them in MySQL. Laravel provides built-in support for time zones, which can be configured in the `config/app.php` file.
Can I use Eloquent to automatically handle date formatting in Laravel 11?
Yes, Eloquent automatically casts date attributes to instances of `Carbon`, allowing you to work with dates easily. You can specify date attributes in your model using the `$dates` property, and Eloquent will handle the formatting for you.
In the context of Laravel 11 and MySQL, selecting the best date format for applications in the United States is crucial for ensuring data consistency and ease of use. The recommended format is ‘Y-m-d H:i:s’, which aligns with MySQL’s default datetime format. This format not only adheres to ISO 8601 standards but also facilitates seamless integration between Laravel’s Eloquent ORM and MySQL databases, ensuring that date and time data is stored and retrieved accurately.
Utilizing the ‘Y-m-d H:i:s’ format allows developers to avoid common pitfalls associated with date handling, such as ambiguity in date representation. In the United States, where date formats can vary significantly (e.g., MM/DD/YYYY vs. DD/MM/YYYY), adopting a standardized format helps mitigate confusion and enhances the overall user experience. Furthermore, this format is beneficial for sorting and querying dates within the database, as it maintains a logical order.
In summary, when working with Laravel 11 and MySQL in a U.S. context, the ‘Y-m-d H:i:s’ date format is the best practice. It ensures compatibility, reduces errors, and promotes clarity in data management. By adhering to this format, developers can create robust applications that
Author Profile
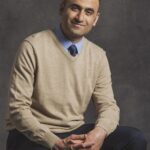
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?