How Can I Use Laravel Faker to Generate Random Dates?
When developing applications with Laravel, one of the most crucial yet often overlooked aspects is the importance of testing and seeding your database with realistic data. Enter Faker, a powerful library that allows developers to generate fake data effortlessly. Among its many features, the ability to create random dates can be particularly useful for simulating real-world scenarios in your application. Whether you’re building a blog, an e-commerce platform, or a social media site, incorporating random dates can help you test your application’s functionality more effectively and ensure it behaves as expected under various conditions.
In this article, we will explore how to leverage Laravel’s integration with Faker to generate random dates for your database seeding and testing processes. Understanding how to create realistic timeframes, whether for past events or future appointments, can enhance the credibility of your application and provide a better user experience. We’ll delve into the various methods available within Faker to generate these dates, as well as best practices for implementing them in your Laravel projects.
As we navigate through the intricacies of generating random dates, you’ll discover how to customize date formats, set specific ranges, and utilize Faker’s extensive capabilities to align with your application’s requirements. By the end of this guide, you’ll be equipped with the knowledge to create dynamic and engaging data that reflects the complexities of
Generating Random Dates with Laravel Faker
Laravel’s Faker library provides a powerful way to generate fake data for testing and seeding your applications. Among the various data types it can generate, random dates are particularly useful for populating databases with realistic data.
To generate a random date using Laravel Faker, you can leverage the `dateTimeBetween` method, which allows you to specify a range for the generated dates. The basic syntax is as follows:
“`php
$faker->dateTimeBetween($startDate = ‘-1 year’, $endDate = ‘now’);
“`
This method returns a `DateTime` object that represents a random date within the specified range. You can customize the start and end dates according to your needs.
Common Methods to Generate Random Dates
Faker provides several methods for generating random dates, each suited for different requirements. Here are some of the most commonly used methods:
- `dateTime()`: Returns a random date and time.
- `date()`: Returns a random date in the format `Y-m-d`.
- `time()`: Returns a random time in the format `H:i:s`.
- `dateTimeBetween()`: Returns a random `DateTime` object between two specified dates.
- `dateTimeThisDecade()`: Returns a random date within the current decade.
- `dateTimeThisMonth()`: Returns a random date within the current month.
Example Usage in Laravel Seeders
When using Laravel Seeders, you can easily incorporate Faker to populate your database with random dates. Below is an example of how to use Faker to generate random dates in a seeder:
“`php
use Illuminate\Database\Seeder;
use Faker\Factory as Faker;
class UsersTableSeeder extends Seeder
{
public function run()
{
$faker = Faker::create();
foreach (range(1, 50) as $index) {
DB::table(‘users’)->insert([
‘name’ => $faker->name,
’email’ => $faker->email,
‘created_at’ => $faker->dateTimeBetween(‘-1 year’, ‘now’),
‘updated_at’ => now(),
]);
}
}
}
“`
This example demonstrates how to create 50 users with random names, emails, and creation dates within the last year.
Table of Date Formats
To further assist in understanding the various date formats generated by Faker, refer to the following table:
Method | Return Type | Format |
---|---|---|
dateTime() | DateTime | Random date and time |
date() | String | Y-m-d |
time() | String | H:i:s |
dateTimeBetween() | DateTime | Random date between specified dates |
dateTimeThisDecade() | DateTime | Random date this decade |
dateTimeThisMonth() | DateTime | Random date this month |
By utilizing these methods and understanding their formats, developers can efficiently generate random dates for various applications in Laravel, ensuring that their testing and seeding processes are both effective and realistic.
Using Laravel Faker to Generate Random Dates
Laravel’s Faker library provides a straightforward way to generate dummy data, including random dates. This can be particularly useful for seeding databases or testing applications. To effectively generate random dates, you can utilize various Faker methods.
Basic Random Date Generation
Faker offers several methods to generate random dates, including:
- `date()`: Generates a random date in the format `Y-m-d`.
- `dateTime()`: Produces a random date and time, returning a `DateTime` object.
- `dateTimeThisCentury()`: Returns a random `DateTime` within this century.
- `dateTimeBetween($startDate, $endDate)`: Generates a random `DateTime` between two specified dates.
Example Usage in Laravel Seeders
In a typical Laravel seeder, you can use Faker to populate a database table with random dates. Below is an example of how to implement this in a seeder class:
“`php
use Illuminate\Database\Seeder;
use Faker\Factory as Faker;
class UsersTableSeeder extends Seeder
{
public function run()
{
$faker = Faker::create();
foreach (range(1, 50) as $index) {
DB::table(‘users’)->insert([
‘name’ => $faker->name,
’email’ => $faker->unique()->safeEmail,
‘created_at’ => $faker->dateTimeBetween(‘-1 year’, ‘now’),
‘updated_at’ => $faker->dateTimeBetween(‘-1 year’, ‘now’),
]);
}
}
}
“`
In this example, `created_at` and `updated_at` fields are populated with random dates generated within the past year.
Generating Specific Date Formats
If you need a specific date format, you can convert the `DateTime` object to a string using the `format()` method. Here’s an example of how to do this:
“`php
$date = $faker->dateTimeBetween(‘-1 year’, ‘now’)->format(‘Y-m-d H:i:s’);
“`
This line generates a random date and formats it as `Y-m-d H:i:s`.
Generating Dates with Specific Constraints
Faker allows you to set specific constraints on the generated dates. For example, you might want to ensure that the date falls within a certain range:
“`php
$startDate = ‘2020-01-01’;
$endDate = ‘2023-01-01’;
$randomDate = $faker->dateTimeBetween($startDate, $endDate)->format(‘Y-m-d’);
“`
This generates a random date between January 1, 2020, and January 1, 2023.
Table of Commonly Used Faker Date Methods
Method | Description |
---|---|
`date()` | Generates a random date in `Y-m-d` format. |
`dateTime()` | Returns a random `DateTime` object. |
`dateTimeThisCentury()` | Random date and time within this century. |
`dateTimeBetween($start, $end)` | Generates a random date between two dates. |
`dateTimeThisYear()` | Returns a random date and time within this year. |
Each method has its own use case, allowing for flexibility based on your specific needs in data generation.
Generating Random Dates with Laravel Faker: Expert Insights
Julia Thompson (Senior Software Engineer, CodeCraft Solutions). “When using Laravel Faker to generate random dates, it is essential to leverage the built-in date methods. The `dateTimeBetween` method allows developers to specify a range, ensuring that the generated dates are realistic and contextually appropriate for the application.”
Michael Chen (Lead Developer, Tech Innovators Inc.). “Faker’s ability to generate random dates can significantly enhance testing scenarios. By utilizing the `dateTime` method, developers can create timestamps that mimic real-world data, which is crucial for validating date-related functionalities in applications.”
Sarah Patel (Data Scientist, Analytics Pro). “Integrating Laravel Faker for random date generation not only aids in testing but also in seeding databases with realistic data. Using methods like `dateTimeThisYear` or `dateTimeThisDecade` provides flexibility, allowing for precise control over the generated data’s temporal context.”
Frequently Asked Questions (FAQs)
How can I generate a random date using Laravel Faker?
You can generate a random date using Laravel Faker by calling the `dateTime` method. For example, `$faker->dateTime($max = ‘now’, $min = ‘2000-01-01’)` will give you a random date between January 1, 2000, and the current date.
What formats can I use for random dates in Laravel Faker?
Laravel Faker allows you to generate random dates in various formats. You can use methods like `date`, `dateTime`, or `dateTimeBetween` to specify the desired format or range for the generated date.
Can I specify a date range when generating random dates with Laravel Faker?
Yes, you can specify a date range using the `dateTimeBetween` method. For instance, `$faker->dateTimeBetween($startDate = ‘-1 years’, $endDate = ‘now’)` generates a random date between one year ago and the current date.
Is it possible to generate random dates in the future using Laravel Faker?
Yes, you can generate random future dates using the `dateTimeBetween` method. For example, `$faker->dateTimeBetween($startDate = ‘now’, $endDate = ‘+1 years’)` generates a random date between now and one year in the future.
What is the difference between `date` and `dateTime` methods in Laravel Faker?
The `date` method generates a random date in the format ‘YYYY-MM-DD’, while the `dateTime` method generates a random date and time in the format ‘YYYY-MM-DD HH:MM:SS’. Use `dateTime` when you need both date and time components.
Can I customize the format of the generated random date in Laravel Faker?
Yes, you can customize the format of the generated date by using the `format` method along with `date`. For example, `$faker->date(‘Y-m-d’)` will give you the date in the specified format.
In summary, generating random dates in Laravel using the Faker library is a straightforward process that can significantly enhance the development and testing of applications. The Faker library provides a robust set of tools for creating fake data, including dates, which can be customized to meet specific requirements. By utilizing the `dateTime` or `dateTimeBetween` methods, developers can easily create random dates within defined ranges, facilitating more realistic data generation for testing purposes.
One of the key takeaways is the flexibility that Faker offers in terms of date generation. Developers can specify the range of dates, allowing for the creation of past, present, or future dates as needed. This capability is particularly useful for applications that require date-related functionalities, such as event scheduling or historical data analysis. Furthermore, integrating Faker into Laravel’s seeders can streamline the process of populating databases with test data, ensuring that applications are well-prepared for various scenarios.
Additionally, understanding how to effectively use Faker to generate random dates can improve the overall quality of application testing. By simulating real-world data conditions, developers can identify potential issues and optimize their applications before deployment. Ultimately, leveraging Laravel Faker for random date generation not only enhances the development workflow but also contributes to more reliable and robust
Author Profile
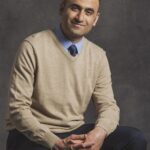
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?