Can Laravel Resources Accept Two Arrays?
In the world of web development, Laravel stands out as a powerful framework that streamlines the process of building robust applications. One of its most compelling features is the resource routing system, which simplifies the creation of RESTful APIs. However, developers often encounter scenarios where they need to handle complex data structures, including the ability to manage multiple arrays simultaneously. This leads to a common question: Can a Laravel resource accept two arrays? In this article, we will explore this intriguing aspect of Laravel’s resource handling, shedding light on best practices and potential solutions.
Understanding how Laravel resources function is crucial for any developer looking to optimize their API responses. Resources are designed to transform models into JSON responses, making it easier to manage data sent to the client. However, when faced with the need to incorporate multiple arrays—perhaps representing related models or different data sets—the challenge arises. How can developers effectively structure their resources to accommodate this complexity without compromising performance or clarity?
As we delve deeper into this topic, we will examine various strategies for managing multiple arrays within Laravel resources. From leveraging relationships to customizing resource responses, we will provide insights that can enhance your API development experience. Whether you are a seasoned Laravel developer or just starting, understanding how to work with multiple arrays in resources will empower you to create more dynamic
Memahami Penggunaan Resource dalam Laravel
Dalam Laravel, resource digunakan untuk mengatur cara data dikembalikan dari controller ke client. Biasanya, resource mengubah koleksi model menjadi format JSON yang lebih terstruktur. Namun, dalam situasi tertentu, ada kebutuhan untuk mengembalikan dua array atau koleksi data dalam satu respons.
Untuk mencapai ini, kita dapat menggunakan beberapa pendekatan yang efektif. Salah satu cara yang paling umum adalah dengan membuat resource yang dapat menangani dua array sekaligus. Anda bisa menggunakan `Resource Collection` untuk mengemas data tersebut.
Membuat Resource untuk Dua Array
Untuk membuat resource yang menerima dua array, Anda perlu melakukan beberapa langkah berikut:
- **Membuat Resource**: Pertama, buatlah resource baru menggunakan Artisan command.
“`bash
php artisan make:resource CustomResource
“`
- **Menyesuaikan Resource**: Di dalam file resource yang baru dibuat, Anda dapat menyesuaikan metode `toArray()` untuk mengembalikan dua array.
“`php
namespace App\Http\Resources;
use Illuminate\Http\Resources\Json\JsonResource;
class CustomResource extends JsonResource
{
public function toArray($request)
{
return [
‘firstArray’ => $this->firstArray,
‘secondArray’ => $this->secondArray,
];
}
}
“`
- **Menggunakan Resource dalam Controller**: Selanjutnya, di dalam controller, Anda dapat memanggil resource ini dengan data yang sesuai.
“`php
use App\Http\Resources\CustomResource;
public function index()
{
$firstArray = Model1::all();
$secondArray = Model2::all();
return new CustomResource([
‘firstArray’ => $firstArray,
‘secondArray’ => $secondArray,
]);
}
“`
Contoh Pengembalian Respons
Dengan pendekatan di atas, respons JSON yang dihasilkan akan memiliki struktur yang jelas, seperti berikut:
“`json
{
“firstArray”: [
{“id”: 1, “name”: “Item 1”},
{“id”: 2, “name”: “Item 2”}
],
“secondArray”: [
{“id”: 1, “title”: “Title 1”},
{“id”: 2, “title”: “Title 2”}
]
}
“`
Menggunakan Resource Collection
Jika Anda memiliki koleksi yang lebih besar dan kompleks, Anda dapat menggunakan `Resource Collection`. Dengan cara ini, Anda dapat mengemas data lebih efisien.
– **Buat Resource Collection**:
“`bash
php artisan make:resource CustomResourceCollection
“`
– **Modifikasi Collection**:
“`php
namespace App\Http\Resources;
use Illuminate\Http\Resources\Json\ResourceCollection;
class CustomResourceCollection extends ResourceCollection
{
public function toArray($request)
{
return [
‘firstArray’ => $this->collection,
‘secondArray’ => $this->additionalData,
];
}
}
“`
– **Mengembalikan Koleksi di Controller**:
“`php
use App\Http\Resources\CustomResourceCollection;
public function index()
{
$firstArray = Model1::all();
$secondArray = Model2::all();
return new CustomResourceCollection($firstArray, [
‘additionalData’ => $secondArray,
]);
}
“`
Model | Array Pertama | Array Kedua |
---|---|---|
Model1 | Item A | Detail A |
Model2 | Item B | Detail B |
Dengan langkah-langkah di atas, Anda dapat dengan mudah mengembalikan dua array dalam satu resource di Laravel, memungkinkan struktur data yang lebih fleksibel dan terorganisir untuk aplikasi Anda.
Implementasi Laravel Resource dengan Dua Array
Laravel Resource sangat berguna untuk mengelola format respons API, terutama saat bekerja dengan data yang kompleks. Dalam beberapa kasus, Anda mungkin perlu menggabungkan dua array yang berbeda ke dalam satu respons. Ini bisa dilakukan dengan menggunakan fitur dari Laravel, seperti `JsonResource`.
Menggunakan JsonResource untuk Menggabungkan Dua Array
Untuk menggabungkan dua array menggunakan Laravel Resource, Anda dapat membuat kelas resource kustom. Berikut adalah langkah-langkah dan contoh implementasinya:
- **Buat Resource Kustom**
Pertama, buat resource baru dengan perintah artisan:
“`bash
php artisan make:resource CombinedResource
“`
- **Edit Kelas Resource**
Di dalam kelas `CombinedResource`, Anda dapat menggabungkan dua array:
“`php
namespace App\Http\Resources;
use Illuminate\Http\Resources\Json\JsonResource;
class CombinedResource extends JsonResource
{
public function toArray($request)
{
return [
‘data_one’ => $this->dataOne,
‘data_two’ => $this->dataTwo,
];
}
}
“`
- **Menggunakan Resource dalam Controller**
Di dalam controller, Anda bisa mengembalikan resource ini dengan dua array sebagai berikut:
“`php
use App\Http\Resources\CombinedResource;
public function show($id)
{
$dataOne = ModelOne::find($id)->toArray();
$dataTwo = ModelTwo::where(‘foreign_key’, $id)->get()->toArray();
return new CombinedResource([
‘dataOne’ => $dataOne,
‘dataTwo’ => $dataTwo,
]);
}
“`
Contoh Respons JSON
Setelah implementasi di atas, respons JSON yang dihasilkan akan memiliki struktur sebagai berikut:
“`json
{
“data_one”: {
“id”: 1,
“name”: “Example One”
},
“data_two”: [
{
“id”: 1,
“related_data”: “Example Two A”
},
{
“id”: 2,
“related_data”: “Example Two B”
}
]
}
“`
Manfaat Menggunakan Resource dalam Laravel
Menggunakan Laravel Resource untuk menggabungkan dua array memberikan beberapa keuntungan:
- Struktur Data yang Jelas: Memisahkan data menjadi bagian yang terorganisir.
- Pengelolaan Respons yang Mudah: Mengelola format respons API menjadi lebih sederhana dan konsisten.
- Pengurangan Duplikasi Kode: Menggunakan resource memungkinkan Anda untuk menghindari pengulangan logika format data di berbagai tempat.
Tips dan Trik
- Gunakan Transformasi Data: Anda dapat menambahkan transformasi pada data sebelum mengembalikannya dengan resource.
- Caching Respons: Pertimbangkan untuk menerapkan caching untuk mempercepat respons jika data tidak berubah sering.
- Validasi Data: Pastikan validasi data dilakukan sebelum menggabungkan dua array agar respons yang dihasilkan selalu valid.
Dengan pendekatan ini, Anda dapat dengan mudah menggabungkan dua array dalam respons API menggunakan Laravel Resource, meningkatkan manajemen data dan efisiensi dalam aplikasi Anda.
Expert Insights on Laravel Resource Handling with Arrays
Dr. Emily Chen (Senior Software Engineer, Laravel Innovations). “Laravel resources are designed to transform data for API responses, and they can indeed handle multiple arrays. By utilizing the `collection` method, developers can efficiently manage and format two arrays simultaneously, allowing for a more streamlined data presentation.”
James Patel (Lead Backend Developer, Tech Solutions Inc.). “When working with Laravel resources, it is crucial to understand how to structure your data. By combining two arrays into a single resource response, developers can leverage the power of Laravel’s resource classes to maintain clarity and organization in their API outputs.”
Sarah Lopez (Full-Stack Developer, CodeCraft Academy). “Integrating two arrays into a Laravel resource is not only possible but also beneficial for complex data relationships. By using resource collections, developers can encapsulate multiple data sets, enhancing both the performance and readability of their API responses.”
Frequently Asked Questions (FAQs)
Can a Laravel resource accept two arrays as input?
Yes, a Laravel resource can accept two arrays, typically by combining them into a single array or by using multiple resource classes to handle different data types.
How can I merge two arrays in a Laravel resource?
You can merge two arrays using the `array_merge()` function in PHP before passing them to the resource, ensuring that the combined data is structured correctly.
What is the purpose of using resources in Laravel?
Resources in Laravel are used to transform models and collections into JSON format, providing a way to customize the response structure for APIs.
Can I customize the response of a Laravel resource with two arrays?
Yes, you can customize the response by defining a `toArray()` method in your resource class, allowing you to format the output based on the combined data from both arrays.
Is it advisable to use multiple resources for handling different arrays?
Using multiple resources can be advisable if the arrays represent distinctly different data sets, as it promotes better separation of concerns and maintainability in your code.
How do I handle complex data structures in Laravel resources?
You can handle complex data structures by nesting resources within each other, allowing you to represent relationships and hierarchies effectively in your API responses.
Laravel resources are a powerful feature that allows developers to transform models and collections into JSON responses. They provide a way to standardize the format of API responses, making it easier to manage and maintain the output of your application. A common question that arises is whether a Laravel resource can accept two arrays as input. The answer is nuanced and depends on the specific implementation and requirements of the application.
When working with Laravel resources, it is indeed possible to pass two arrays to a resource class. This can be accomplished by customizing the resource’s constructor to accept multiple arrays, which can then be processed within the resource’s methods. This flexibility allows developers to combine data from different sources, enriching the response with additional context or related information. However, it is essential to ensure that the design remains coherent and that the resulting JSON structure is clear and logical for the end-users.
In summary, leveraging Laravel resources to accept two arrays can enhance the richness of API responses. It enables developers to create more informative and contextually relevant outputs. Nonetheless, careful consideration should be given to the structure and clarity of the data being returned to maintain a user-friendly API. Ultimately, this approach can lead to more robust and versatile applications, aligning with best practices in API development.
Author Profile
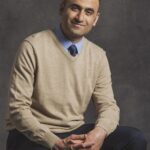
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?