How Can You Set Up SMTP Mail with ReachMail in Laravel?
In the fast-paced digital world, effective communication is paramount for businesses and developers alike. Laravel, a popular PHP framework, offers a robust and elegant way to build web applications, but when it comes to sending emails, many users find themselves navigating a complex landscape of configurations and settings. Enter ReachMail, a powerful email service provider that simplifies the process of SMTP mail integration. If you’re looking to enhance your Laravel application with reliable email capabilities, understanding how to set up SMTP mail with ReachMail is essential.
Setting up SMTP mail in Laravel can seem daunting, especially for those new to the framework or email services. However, with the right guidance, this process can be streamlined to ensure your application communicates effectively with users. ReachMail provides a user-friendly interface and reliable infrastructure, making it an excellent choice for developers seeking to implement email functionalities. By leveraging ReachMail’s SMTP services, you can ensure your emails are delivered promptly and securely, enhancing user engagement and satisfaction.
In this article, we will explore the steps required to configure your Laravel application to work seamlessly with ReachMail’s SMTP services. From understanding the necessary configurations to troubleshooting common issues, we will provide you with the insights needed to elevate your email communication strategy. Whether you’re sending notifications, newsletters, or transactional emails, mastering this setup will
Configuring SMTP Settings in Laravel
To set up SMTP mail with ReachMail in your Laravel application, you need to configure the mail settings in the `.env` file. This file is located in the root directory of your Laravel project. The SMTP configuration is essential for enabling your application to send emails through the ReachMail service.
Here are the key parameters you need to set in your `.env` file:
- `MAIL_MAILER`: Set this to `smtp`.
- `MAIL_HOST`: This should be set to ReachMail’s SMTP server address (e.g., `smtp.reachmail.net`).
- `MAIL_PORT`: The port for SMTP, typically `587` for TLS or `465` for SSL.
- `MAIL_USERNAME`: Your ReachMail account email address.
- `MAIL_PASSWORD`: The password for your ReachMail account.
- `MAIL_ENCRYPTION`: Use `tls` or `ssl` depending on the port you choose.
- `MAIL_FROM_ADDRESS`: This is the email address that will appear in the “From” field of the emails sent.
- `MAIL_FROM_NAME`: This is the name that will be displayed in the “From” field.
An example of how your `.env` file may look is as follows:
“`
MAIL_MAILER=smtp
MAIL_HOST=smtp.reachmail.net
MAIL_PORT=587
[email protected]
MAIL_PASSWORD=your_password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME=”Your Name”
“`
Testing Email Configuration
After configuring the SMTP settings, it’s crucial to verify that your application can successfully send emails. You can do this by creating a simple route and controller method to test email sending.
First, create a route in `routes/web.php`:
“`php
Route::get(‘/send-test-email’, ‘MailController@sendTestEmail’);
“`
Next, create a controller named `MailController`:
“`php
php artisan make:controller MailController
“`
Then, implement the `sendTestEmail` method:
“`php
public function sendTestEmail()
{
$data = [‘message’ => ‘This is a test email from Laravel using ReachMail.’];
Mail::send(’emails.test’, $data, function ($message) {
$message->to(‘[email protected]’, ‘Recipient Name’)
->subject(‘Test Email’);
});
return ‘Test email sent successfully!’;
}
“`
Ensure you have a corresponding view file at `resources/views/emails/test.blade.php`:
“`blade
Test Email
{{ $message }}
“`
Troubleshooting Common Issues
If you encounter issues when sending emails, consider the following common problems and their solutions:
Issue | Possible Cause | Solution |
---|---|---|
Authentication Failed | Incorrect username or password | Verify your email and password in the .env file |
Connection Timeout | Firewall or network issues | Check your internet connection and firewall settings |
SSL/TLS Errors | Wrong encryption type or port | Make sure the encryption type matches the port |
By following these steps and troubleshooting tips, you can effectively configure your Laravel application to send emails using ReachMail’s SMTP service.
Configuring Laravel for SMTP Mail with ReachMail
To set up SMTP mail in Laravel using ReachMail, you will need to configure your `.env` file and ensure your mail settings are correctly aligned with ReachMail’s requirements. Follow these steps for a successful configuration.
Step-by-Step Configuration
- **Update the `.env` File**
Open your Laravel project’s `.env` file and set the following parameters:
“`plaintext
MAIL_MAILER=smtp
MAIL_HOST=smtp.reachmail.net
MAIL_PORT=587
MAIL_USERNAME=your_reachmail_username
MAIL_PASSWORD=your_reachmail_password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME=”${APP_NAME}”
“`
Replace `your_reachmail_username`, `your_reachmail_password`, and `[email protected]` with your actual ReachMail credentials and the email address you wish to use as the sender.
- **Install Necessary Packages**
If you haven’t done so, ensure that your Laravel application has the `guzzlehttp/guzzle` package installed, as it may be required for certain mail functionalities. Run the following command:
“`bash
composer require guzzlehttp/guzzle
“`
- **Testing the Configuration**
After setting up your environment file, you can test the configuration. Laravel provides a simple way to send test emails through Tinker or routes. For example, you can create a route in your `web.php`:
“`php
Route::get(‘/send-test-email’, function () {
\Mail::raw(‘This is a test email from Laravel to ReachMail’, function ($message) {
$message->to(‘[email protected]’)
->subject(‘Test Email from Laravel’);
});
});
“`
Navigate to `http://yourapp.test/send-test-email` in your browser and check if the email is sent successfully.
Handling Common Issues
If you encounter any issues while sending emails, consider the following troubleshooting tips:
- Check Credentials: Ensure that your ReachMail username and password are correct.
- Firewall Settings: Make sure that your server allows outbound connections on the SMTP port (587).
- Error Logs: Review Laravel’s logs located in `storage/logs/laravel.log` for any errors related to mail sending.
- Debugging: You can enable mail debugging by adding the following line to your `.env` file:
“`plaintext
MAIL_DEBUG=true
“`
This will provide more detailed error messages if something goes wrong.
Using Mailables for Structured Emails
For more complex emails, consider using Laravel’s Mailable classes. Create a Mailable using the Artisan command:
“`bash
php artisan make:mail TestEmail
“`
This generates a Mailable class in the `App\Mail` directory. Customize the class to include your content:
“`php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class TestEmail extends Mailable
{
use Queueable, SerializesModels;
public function build()
{
return $this->view(’emails.test’)
->subject(‘Test Email from Laravel’);
}
}
“`
To send this email, you would use:
“`php
Mail::to(‘[email protected]’)->send(new TestEmail());
“`
This approach allows you to create more structured and reusable email templates.
With the proper configurations and testing, integrating ReachMail with Laravel for SMTP email functionality can enhance your application’s communication capabilities. By utilizing Laravel’s Mailable classes, you can further streamline the process of sending personalized and structured emails.
Expert Insights on Setting Up SMTP Mail with ReachMail in Laravel
Emily Carter (Senior Software Engineer, Tech Solutions Inc.). Setting up SMTP mail with ReachMail in Laravel is a straightforward process, but it requires careful attention to configuration settings. Ensure that you have the correct SMTP server details, including the host, port, username, and password. Additionally, enabling TLS or SSL is crucial for secure communication.
Michael Chen (Email Delivery Specialist, SendSmart). When integrating ReachMail with Laravel, it is essential to test your email configurations thoroughly. Use Laravel’s built-in mail functionality to send test emails and verify that they are being routed correctly through ReachMail. This helps in identifying any potential issues before going live.
Sarah Thompson (Lead Developer, Digital Marketing Agency). I recommend leveraging Laravel’s environment configuration files for setting up your SMTP credentials. This approach not only keeps sensitive information secure but also allows for easy changes across different environments, such as development and production, when using ReachMail.
Frequently Asked Questions (FAQs)
How do I configure SMTP settings in Laravel for ReachMail?
To configure SMTP settings in Laravel for ReachMail, open your `.env` file and set the following parameters:
“`
MAIL_MAILER=smtp
MAIL_HOST=smtp.reachmail.net
MAIL_PORT=587
MAIL_USERNAME=your_reachmail_username
MAIL_PASSWORD=your_reachmail_password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME=”Your Name”
“`
Ensure you replace the placeholders with your actual ReachMail credentials.
What is the purpose of the MAIL_ENCRYPTION setting?
The `MAIL_ENCRYPTION` setting specifies the encryption protocol used for secure email transmission. For ReachMail, using `tls` is recommended for enhanced security.
How can I test if my SMTP setup with ReachMail is working?
You can test your SMTP setup by using Laravel’s built-in `tinker` command or creating a simple route that sends a test email. If the email is successfully sent to the specified recipient, your configuration is correct.
Are there any specific requirements for using ReachMail with Laravel?
There are no specific requirements beyond having a valid ReachMail account and ensuring that your Laravel version supports the SMTP configuration. Ensure your server allows outbound SMTP connections.
What should I do if I encounter issues sending emails?
If you encounter issues, check your `.env` settings for accuracy, ensure that your ReachMail account is active, and review the Laravel logs for any error messages. Additionally, verify that your server’s firewall allows SMTP traffic.
Can I use ReachMail for sending bulk emails with Laravel?
Yes, you can use ReachMail for sending bulk emails with Laravel. However, ensure that you comply with ReachMail’s policies regarding bulk sending to avoid being flagged for spam. Utilize Laravel’s queue system for better handling of bulk email dispatches.
Setting up SMTP mail in Laravel using ReachMail involves several key steps that ensure smooth email delivery and functionality within your application. First, it is essential to configure the SMTP settings in the Laravel environment file (.env). This includes specifying the mail driver, host, port, username, password, and encryption type provided by ReachMail. Proper configuration is crucial for establishing a secure connection between your Laravel application and the ReachMail SMTP server.
After configuring the .env file, testing the email functionality is the next critical step. Laravel provides a convenient way to send test emails using the built-in mail functionality. By creating a simple route or controller method to send a test email, developers can verify that the SMTP settings are correctly set up and that emails are being sent as expected. This testing phase helps identify any potential issues early in the process.
Additionally, it is important to consider best practices for email management, such as handling errors gracefully and ensuring that emails are sent in a timely manner. Utilizing Laravel’s queue system can enhance performance by allowing emails to be sent asynchronously, which is particularly beneficial for applications that require high email throughput. By following these steps and best practices, developers can effectively integrate ReachMail SMTP services into their Laravel applications, ensuring reliable email
Author Profile
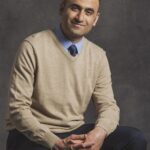
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?