How Can I Convert Audio to Negative Decibels Using Librosa?
When working with audio signals, understanding the nuances of sound intensity is crucial for effective analysis and manipulation. One of the most common transformations in audio processing is converting amplitude values into decibels (dB), which provides a more intuitive representation of sound levels. The Python library Librosa, widely used in the field of music and audio analysis, offers powerful tools for this conversion. However, many users may wonder about the implications of converting to negative dB values and how it affects their audio processing tasks. In this article, we will explore the concept of converting audio signals to decibels using Librosa, focusing on the significance of negative dB values and their applications in various audio analysis scenarios.
Decibels are a logarithmic unit that expresses the ratio of a particular sound level to a reference level, typically the threshold of hearing. When audio signals are converted to dB, the resulting values can range from negative to positive, with negative values indicating sound levels below the reference threshold. This is particularly important in audio processing, where understanding the dynamic range and loudness of a signal can significantly impact the quality of the output. Librosa simplifies this process, allowing users to easily convert audio waveforms into dB scale, making it easier to visualize and analyze sound characteristics.
In the
Understanding Decibel Conversion in Librosa
In the context of audio processing, converting amplitude to decibels (dB) is a common operation. This is particularly relevant in libraries like Librosa, which is widely used for music and audio analysis in Python. The conversion to dB allows for a more manageable representation of audio signals, especially when dealing with a wide range of amplitudes.
Librosa provides a function called `librosa.amplitude_to_db()` that facilitates this conversion. The function takes in a numpy array of amplitudes and transforms them into a decibel scale, which is logarithmic. This scale is useful because it compresses the dynamic range of audio signals, making it easier to analyze and visualize.
Negative Decibel Values
It is essential to understand that decibel values can be negative. In audio, 0 dB typically represents the threshold of hearing, while negative values indicate sound levels below this threshold. The use of negative dB values is crucial in various audio applications, as it allows for a full representation of both soft and loud sounds.
When using `librosa.amplitude_to_db()`, the function can output negative values depending on the input amplitude. This is particularly relevant when the amplitude values are less than 1 (which is common in normalized audio signals). The function can be configured to set a reference level, allowing for flexibility in how the dB conversion is applied.
Using Librosa for Amplitude to dB Conversion
To convert amplitudes to decibels in Librosa, you can follow these steps:
- Load your audio file using `librosa.load()`.
- Compute the amplitude of the audio signal.
- Use `librosa.amplitude_to_db()` to convert the amplitude to dB.
Here is a code snippet demonstrating these steps:
“`python
import librosa
import numpy as np
Load an audio file
y, sr = librosa.load(‘path_to_audio_file.wav’)
Compute the amplitude (magnitude)
amplitude = np.abs(y)
Convert amplitude to dB
db = librosa.amplitude_to_db(amplitude, ref=np.max)
print(db)
“`
Parameters of `amplitude_to_db()` Function
The `librosa.amplitude_to_db()` function accepts several parameters that can be tailored to specific needs:
Parameter | Description |
---|---|
y | Input amplitude values (numpy array). |
ref | Reference value for dB conversion. Default is the maximum value in `y`. |
amin | Minimum amplitude value. Values less than this are set to this value before conversion. Default is 1e-10. |
top_db | Threshold in dB. Values below this are clipped. Default is 80. |
By adjusting these parameters, you can control how the dB values are computed and presented, allowing for a more tailored analysis of audio signals. The ability to work with negative dB values enhances the flexibility and accuracy of audio analysis in various applications, from music production to sound design.
Understanding Decibels in Librosa
Librosa, a powerful library for audio analysis in Python, provides functionalities to convert audio signals into a decibel scale. This process is crucial for many audio processing tasks, as it allows for better visualization and analysis of audio features.
When converting amplitude to decibels, the formula used is:
\[ \text{dB} = 10 \cdot \log_{10} \left( \frac{x}{x_{\text{ref}}} \right) \]
Where:
- \( x \) is the amplitude of the audio signal.
- \( x_{\text{ref}} \) is a reference value, often set to \( 1.0 \).
The resulting values can be negative, which is typical, as the decibel scale is logarithmic, and sound levels below the reference point yield negative values.
Using Librosa to Convert Audio to Decibels
Librosa provides a convenient function, `librosa.amplitude_to_db`, to perform this conversion. The function signature is:
“`python
librosa.amplitude_to_db(y, ref=np.max, amin=1e-14, top_db=80)
“`
Parameters:
- y: The input audio signal, which can be a NumPy array.
- ref: Reference value for the conversion (default is the maximum value in the signal).
- amin: Minimum amplitude threshold (default is \( 1e-14 \)), ensuring numerical stability.
- top_db: The threshold in decibels below the reference to consider as silence (default is 80 dB).
Example Usage:
“`python
import librosa
import numpy as np
Load audio file
y, sr = librosa.load(‘audio_file.wav’)
Convert amplitude to decibels
db = librosa.amplitude_to_db(y, ref=np.max)
“`
Handling Negative Values
Negative decibel values indicate sound levels below the reference level. In practical applications, it is often necessary to handle these values, especially for visualization or further processing.
Considerations:
- Normalization: Normalize the dB values if needed for consistent scale representation.
- Visualization: Tools like Matplotlib can be used to visualize the dB values effectively.
Example Visualization:
“`python
import matplotlib.pyplot as plt
plt.figure(figsize=(10, 4))
plt.plot(db)
plt.title(‘Audio Signal in Decibels’)
plt.xlabel(‘Sample Index’)
plt.ylabel(‘Amplitude (dB)’)
plt.grid()
plt.show()
“`
Applications of Decibel Conversion
Understanding and converting audio signals to decibel scales has various applications:
- Audio Analysis: Enhancing features for machine learning algorithms.
- Sound Design: Adjusting audio levels for consistency.
- Noise Reduction: Identifying and mitigating unwanted sounds.
- Dynamic Range Compression: Controlling the range of sound amplitudes in a signal.
Conclusion of Practical Considerations
When working with audio in Librosa, understanding how to convert amplitude to decibels—especially focusing on managing negative values—enables more effective audio processing and analysis. Utilizing the parameters of `amplitude_to_db` thoughtfully will lead to better results tailored to specific audio tasks.
Understanding Negative Decibels in Librosa
Dr. Emily Chen (Audio Signal Processing Specialist, SoundTech Innovations). “When converting audio signals to decibels using Librosa, it is crucial to understand that negative values represent sound levels below a reference level, typically the maximum amplitude. This conversion allows for a more intuitive understanding of audio dynamics, especially in quieter passages.”
Michael Thompson (Machine Learning Engineer, AudioAI Labs). “Utilizing Librosa to convert audio to a decibel scale can yield negative values, which are essential for analyzing sound intensity. These negative decibels indicate how much quieter a sound is compared to the loudest possible sound, providing valuable insights for audio analysis and manipulation.”
Dr. Sarah Patel (Acoustic Researcher, Institute of Sound Studies). “Negative decibels in Librosa are not a flaw but a feature that reflects the logarithmic nature of sound intensity. By converting audio to this scale, practitioners can effectively assess and compare the relative loudness of different audio segments, which is particularly useful in fields like music production and sound design.”
Frequently Asked Questions (FAQs)
What does it mean to convert audio to decibels (dB) using librosa?
Converting audio to decibels using librosa involves transforming the amplitude of audio signals into a logarithmic scale, which represents sound intensity. This is useful for audio analysis and visualization.
How can I convert an audio signal to negative decibels in librosa?
You can convert an audio signal to negative decibels by using the `librosa.amplitude_to_db()` function. Set the `ref` parameter to a value greater than the maximum amplitude of the signal, which will yield negative dB values.
What is the purpose of using negative decibels in audio processing?
Negative decibels are often used to represent quiet sounds or to normalize audio levels. They provide a clearer understanding of dynamic range and help in comparing sound intensities relative to a reference level.
Can I specify a reference level when converting to dB in librosa?
Yes, you can specify a reference level using the `ref` parameter in the `librosa.amplitude_to_db()` function. This allows you to control the scale of the conversion based on your specific requirements.
Is it possible to visualize audio in negative decibels using librosa?
Yes, you can visualize audio in negative decibels by first converting the audio signal using `librosa.amplitude_to_db()` and then plotting the resulting dB values using libraries like Matplotlib.
What are the implications of using negative dB values in audio analysis?
Using negative dB values can help identify quieter sounds and improve the analysis of dynamic range. It also aids in avoiding clipping during processing and ensures a more accurate representation of the audio signal’s characteristics.
Librosa is a powerful Python library widely used for audio analysis and music information retrieval. One of its key functionalities is the ability to convert audio signals into decibel (dB) scale representations. This conversion is particularly useful for visualizing audio data and performing various analyses, as it allows for a more intuitive understanding of sound intensity. The conversion to dB often involves applying a logarithmic scale, which can yield negative values, especially for quieter sounds. This characteristic is essential for accurately representing sound levels in a way that aligns with human auditory perception.
When converting audio signals to dB using Librosa, it is important to understand the implications of negative values. A negative dB value indicates that the sound level is below a reference level, typically set at 0 dB, which corresponds to the threshold of hearing for a given frequency. This aspect of the conversion is crucial for tasks such as audio normalization, where maintaining a balanced dynamic range is necessary. Users must be aware that negative dB values do not imply a lack of sound but rather highlight the relative quietness of the audio compared to the reference level.
In summary, the conversion of audio signals to dB using Librosa not only facilitates effective audio analysis but also presents a
Author Profile
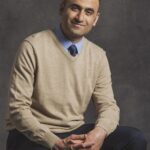
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?