How to Use LINQ Lambda to Select the Maximum Column Value in Entity Framework Core?
In the realm of modern application development, efficient data manipulation is paramount. As developers strive to create responsive and dynamic applications, the need for robust querying capabilities becomes increasingly vital. Enter LINQ (Language Integrated Query) and Lambda expressions, two powerful tools that seamlessly integrate with Entity Framework Core, allowing developers to harness the full potential of their data. Whether you’re aggregating results, filtering datasets, or extracting maximum values from specific columns, mastering these techniques can significantly enhance your application’s performance and user experience.
At the heart of LINQ is its ability to provide a concise and readable syntax for querying collections, making it an indispensable asset for developers working with Entity Framework Core. By leveraging Lambda expressions, you can create expressive queries that not only improve code clarity but also optimize execution time. One common requirement in data manipulation is the need to retrieve the maximum value from a particular column, a task that can be elegantly accomplished using LINQ’s `Select` and `Max` methods. Understanding how to effectively implement these functions can streamline your data retrieval processes and empower you to build more intelligent applications.
As we delve deeper into the intricacies of LINQ and Lambda expressions, we will explore practical examples and best practices for selecting maximum column values within Entity Framework Core. Whether you’re a seasoned developer or just starting your
Using LINQ with Lambda Expressions
LINQ (Language Integrated Query) in Entity Framework Core allows developers to efficiently retrieve data from databases using a syntax that is integrated into C. Lambda expressions provide a concise way to represent anonymous methods, making it easier to write queries. When dealing with collections of data, you can use the `Select` method in conjunction with `Max` to obtain the maximum value of a specified column.
To retrieve the maximum value from a particular column, you can combine these methods in a fluent way. For example, if you have a list of entities and you want to find the maximum value in a specific field, you would typically structure your query like this:
“`csharp
var maxValue = dbContext.Entities
.Select(e => e.ColumnName)
.Max();
“`
This code snippet queries the `Entities` table and selects the values from `ColumnName`, then retrieves the maximum value from that selection.
Example Scenario
Consider a scenario where you have a `Products` entity with a `Price` column, and you need to find the maximum price among all products. The LINQ query would look like this:
“`csharp
var maxPrice = dbContext.Products
.Select(p => p.Price)
.Max();
“`
This retrieves the maximum price value from the `Products` table.
Multiple Criteria with Max
When you need to apply conditions before fetching the maximum value, you can use the `Where` clause along with `Select` and `Max`. For instance, if you want to find the maximum price of products that are in stock, the query can be structured as follows:
“`csharp
var maxInStockPrice = dbContext.Products
.Where(p => p.InStock > 0)
.Select(p => p.Price)
.Max();
“`
This effectively filters the products to only those that are in stock before calculating the maximum price.
Performance Considerations
When executing queries, particularly those involving `Max`, it’s important to consider the following:
- Indexes: Ensure that the column being queried (e.g., `Price`) is indexed for optimal performance.
- Database Load: Complex queries may increase load on the database server, especially with large datasets.
- Memory Usage: Be mindful of how much data is being pulled into memory; using `Max` directly on the database can reduce memory overhead.
Example Table
Here’s a simple representation of a `Products` table that illustrates the query:
ProductId | ProductName | Price | InStock |
---|---|---|---|
1 | Product A | 20.00 | 5 |
2 | Product B | 15.50 | 0 |
3 | Product C | 30.00 | 3 |
In this table, if you run the maximum price query, the result would be `30.00`, considering that `Product C` has the highest price and is in stock.
By leveraging LINQ and lambda expressions, developers can effectively query and manipulate data in a clear and efficient manner within Entity Framework Core.
Using LINQ with Lambda Expressions to Select Maximum Column Values
In Entity Framework Core, retrieving the maximum value from a specific column can be efficiently accomplished using LINQ with lambda expressions. The `Max` method is particularly useful for this purpose.
Example Scenario
Assume you have an entity named `Product` with properties such as `Id`, `Name`, and `Price`. The goal is to find the maximum price among all products.
Sample Code
The following example demonstrates how to use LINQ with a lambda expression to achieve this:
“`csharp
using (var context = new YourDbContext())
{
var maxPrice = context.Products.Max(p => p.Price);
Console.WriteLine($”The maximum price is: {maxPrice}”);
}
“`
Breakdown of the Code
– **Context Initialization**: The `YourDbContext` is instantiated, representing the database context.
– **Max Method**: The `Max` method is called on the `Products` DbSet.
– **Lambda Expression**: The lambda expression `p => p.Price` specifies that the maximum value should be retrieved from the `Price` property of the `Product` entity.
Additional Considerations
When using `Max`, consider the following:
– **Null Values**: If the column may contain null values, ensure you handle them appropriately. The `Max` method will ignore nulls, but you may want to check for an empty result set.
– **Asynchronous Operations**: For better performance in web applications, consider using the asynchronous version:
“`csharp
var maxPriceAsync = await context.Products.MaxAsync(p => p.Price);
“`
Filtering Results
You can also filter results before finding the maximum value. For instance, if you want the maximum price of products that are in stock:
“`csharp
var maxPriceInStock = context.Products
.Where(p => p.InStock)
.Max(p => p.Price);
“`
Table of LINQ Methods for Aggregation
Method | Description |
---|---|
Max | Returns the maximum value of a specified column. |
Min | Returns the minimum value of a specified column. |
Sum | Returns the sum of a specified column. |
Average | Returns the average of a specified column. |
Count | Returns the number of elements in a collection. |
Conclusion of LINQ Max Usage
Using LINQ with lambda expressions in Entity Framework Core allows for efficient querying of maximum values from database tables. This approach is straightforward and integrates well with other LINQ methods for more complex data manipulations.
Maximizing Efficiency with LINQ and Entity Framework Core
Dr. Emily Carter (Data Architect, Tech Innovations Inc.). “Utilizing LINQ with lambda expressions in Entity Framework Core allows developers to efficiently retrieve the maximum value from a specified column. This approach not only enhances performance but also simplifies the codebase, making it more maintainable.”
James Liu (Senior Software Engineer, CodeCraft Solutions). “When working with Entity Framework Core, leveraging the ‘Select’ method in combination with ‘Max’ can significantly reduce the amount of data processed. This is particularly beneficial in large datasets where performance optimization is crucial.”
Sarah Thompson (Lead Developer, Modern Web Technologies). “Incorporating lambda expressions within LINQ queries not only streamlines the process of finding maximum values but also enhances readability. This is essential for teams that prioritize clean code practices while working with Entity Framework Core.”
Frequently Asked Questions (FAQs)
How can I use LINQ to select the maximum value of a column in Entity Framework Core?
You can use the `Max` method in LINQ to select the maximum value of a specific column. For example: `context.Entities.Max(e => e.ColumnName);` retrieves the maximum value from the specified column.
Can I use lambda expressions with LINQ to filter results before selecting the maximum value?
Yes, you can chain a `Where` clause before the `Max` method to filter the results. For example: `context.Entities.Where(e => e.Condition).Max(e => e.ColumnName);` applies the filter before calculating the maximum.
What is the difference between using `Max` and `OrderByDescending` followed by `FirstOrDefault`?
Using `Max` directly retrieves the maximum value efficiently, while `OrderByDescending` sorts the entire collection and retrieves the first element, which is less efficient for large datasets.
Is it possible to select the maximum value along with other columns in Entity Framework Core?
Yes, you can use an anonymous type or a DTO to select multiple columns. For example: `var result = context.Entities.Select(e => new { MaxValue = e.ColumnName, e.OtherColumn }).OrderByDescending(e => e.MaxValue).FirstOrDefault();`.
What should I do if my query returns null when using `Max`?
If your query returns null, it may indicate that there are no records in the dataset or that all entries have null values in the specified column. You can handle this by checking for null before performing operations on the result.
Can I use `Max` with grouped data in Entity Framework Core?
Yes, you can use `GroupBy` in combination with `Max` to find the maximum value within each group. For example: `context.Entities.GroupBy(e => e.GroupColumn).Select(g => new { Group = g.Key, MaxValue = g.Max(e => e.ColumnName) });` retrieves the maximum value for each group.
In the context of Entity Framework Core, utilizing LINQ (Language Integrated Query) with lambda expressions provides a powerful and efficient way to query data from a database. When seeking to retrieve the maximum value from a specific column, the combination of the `Select` method and the `Max` function facilitates a streamlined approach. This method allows developers to focus on specific properties of entities, enabling precise data retrieval and manipulation.
By employing a lambda expression within the `Select` method, developers can specify the exact column from which they wish to extract the maximum value. This not only enhances code readability but also optimizes performance by reducing the amount of data processed. The use of `Max` further simplifies the operation, allowing for an immediate return of the highest value found in the specified column.
In summary, leveraging LINQ with lambda expressions in Entity Framework Core for selecting the maximum column value is an efficient practice that enhances both performance and clarity in data queries. This approach empowers developers to write concise and effective code, ultimately leading to better database interactions and improved application performance.
Author Profile
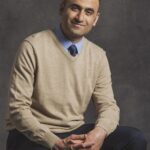
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?