How Can I Resolve the ‘Conflicting Types for Function’ Error in Linux Syscalls?
Navigating the intricate world of Linux programming can often feel like walking a tightrope, where a single misstep can lead to frustrating errors and unexpected behavior. One common pitfall developers encounter is the dreaded “conflicting types for function” error, particularly when dealing with system calls. This issue not only disrupts the flow of coding but can also obscure the underlying logic that drives Linux’s powerful capabilities. Understanding the nuances of this error is essential for any programmer looking to harness the full potential of Linux system calls.
As you delve deeper into the realm of Linux system calls, it becomes clear that they serve as the bridge between user applications and the kernel. Each system call is defined by a specific prototype, and any deviation from this can trigger a myriad of errors, including the notorious conflicting types error. This situation often arises when there is a mismatch in function declarations across different files or libraries, leading to confusion for the compiler.
In this article, we will explore the roots of the “conflicting types for function” error in the context of Linux system calls, shedding light on common scenarios that lead to this issue. By understanding the underlying principles and best practices for function declarations, you will be better equipped to troubleshoot and resolve these errors efficiently. Join us as we unravel
Understanding Conflicting Types in Linux Syscalls
When developing applications in Linux, it’s common to encounter errors related to conflicting types for functions, especially when dealing with system calls. These errors typically arise when the declared type of a function does not match its definition or the expected type in the context of a syscall. This can lead to compilation failures or runtime issues that can be difficult to debug.
Common Causes of Conflicting Types
Several factors can contribute to the occurrence of conflicting types for functions in Linux syscalls:
- Mismatched Function Signatures: If a function is declared with one signature but defined with another, the compiler will raise a type conflict. For example, if a function is declared to return an `int` but is defined to return a `void`, this will lead to an error.
- Header File Inconsistencies: Including different header files that define the same function with different signatures can result in conflicting types. It’s crucial to ensure that the correct header file is included and that the function declarations are consistent.
- C vs. C++ Differences: When using C++ to wrap C libraries, differences in type handling can cause type conflicts, especially with function pointers and overloading.
- Macro Definitions: Sometimes, macros can redefine types or functions in unexpected ways, leading to conflicts that are hard to trace.
Identifying and Resolving Errors
To resolve conflicting type errors, follow a systematic approach:
- Check Function Declarations and Definitions: Ensure that the function declaration matches its definition in terms of return type and parameters.
- Review Header Files: Verify that the correct header files are included and check for multiple inclusions that may lead to discrepancies.
- Use `extern “C”` in C++: When using C libraries in C++, encapsulate C function declarations with `extern “C”` to prevent name mangling and ensure type consistency.
- Inspect Macro Usage: Check for any macros that may redefine the function or its types and alter their expected signatures.
- Compiler Warnings: Enable compiler warnings to get detailed information on where the type conflicts are occurring. This can help in pinpointing the source of the issue.
Example of a Conflicting Type Error
Consider the following code snippet which demonstrates a conflicting type scenario:
“`c
// Header file: example.h
int my_function(int a);
// Source file: example.c
include “example.h”
void my_function(int a) { // Conflict: returns void instead of int
// Function implementation
}
“`
In this example, the declaration in the header file specifies that `my_function` returns an `int`, while the definition incorrectly specifies a return type of `void`. This discrepancy will lead to a compilation error.
Resolving the Example Conflict
To resolve the above conflict, ensure that both the declaration and definition agree on the return type. The corrected code should look like this:
“`c
// Corrected Source file: example.c
include “example.h”
int my_function(int a) { // Consistent return type
// Function implementation
return a;
}
“`
Helpful Tools
Utilizing tools can significantly aid in detecting and resolving type conflicts:
Tool | Description |
---|---|
GCC | GNU Compiler Collection, provides detailed error messages and warnings about conflicting types. |
Clang | A compiler that offers precise diagnostics about type conflicts and other issues. |
Static Analyzers | Tools like Coverity and SonarQube can identify potential type mismatches at a deeper level. |
By systematically addressing these aspects, developers can minimize the occurrence of conflicting type errors in their Linux system calls and ensure smoother compilation and runtime performance.
Understanding the Error
Conflicting types for a function in Linux syscall programming typically arise when the definition of a function or its prototype does not match the expected type. This can lead to compile-time errors, which can be frustrating when developing system-level applications.
Common causes of this error include:
- Mismatched Return Types: The declared return type in the function prototype differs from the actual return type of the function definition.
- Parameter Type Discrepancies: The types or number of parameters in the function prototype do not match those in the implementation.
- Inclusion of Headers: If you include a header file that has an incompatible function declaration, you may inadvertently introduce conflicting types.
Common Scenarios and Solutions
Here are some common scenarios that could lead to conflicting types and their respective solutions:
Scenario | Description | Solution |
---|---|---|
Inconsistent Return Types | The prototype declares a function returning `int`, but the definition returns `void`. | Ensure both declare the same return type. |
Parameter Type Mismatch | A function is declared to take `int`, but the definition uses `float`. | Update the prototype or definition to match types. |
Missing Header Files | A function is defined in a source file but not correctly declared in a header file. | Add the correct prototype in the header file. |
Conflicting Declarations | Two different header files declare the same function with different types. | Use `ifdef` guards or ensure a single consistent header. |
Debugging Steps
To troubleshoot and resolve conflicting type errors in your code, follow these debugging steps:
- Check Function Declarations: Review both the function prototype and definition for consistency in return types and parameter lists.
- Inspect Header Files: Identify any header files included in your source files that might contain conflicting declarations.
- Use Compiler Warnings: Enable compiler warnings (e.g., `-Wall` in GCC) to get detailed information about the type mismatches.
- Simplify Your Code: Temporarily comment out sections of your code to isolate the function causing the conflict.
- Search for Multiple Declarations: Look for multiple declarations of the same function in different files or libraries that may have differing types.
Example Scenario
Consider the following example that generates a conflicting types error:
“`c
// header.h
void myFunction(int);
// source.c
include “header.h”
void myFunction(float x) { // Error: conflicting types for myFunction
// Function implementation
}
“`
In this example, the function `myFunction` is declared in `header.h` as taking an `int` but defined in `source.c` as taking a `float`. To fix this, you must ensure both the declaration and definition match:
“`c
// header.h
void myFunction(float);
// source.c
include “header.h”
void myFunction(float x) {
// Function implementation
}
“`
By ensuring consistency across declarations and definitions, you can resolve the conflicting types error and maintain code integrity in your Linux syscall applications.
Understanding Linux Syscall Errors: Insights from Experts
Dr. Emily Carter (Senior Systems Architect, Open Source Solutions). “Conflicting types for function errors in Linux syscall implementations often stem from mismatched data types between the function declaration and its definition. It is crucial to ensure that the function signature is consistent across all declarations to avoid such issues.”
Mark Thompson (Linux Kernel Developer, Tech Innovations Inc.). “When encountering a syscall error related to conflicting types, developers should meticulously review the headers and source files involved. This type of error can lead to significant debugging challenges, especially in large codebases where function prototypes may be declared in multiple places.”
Lisa Chen (Software Engineer, Embedded Systems Expert). “In my experience, resolving conflicting types for function errors requires a systematic approach. It is essential to utilize compiler warnings effectively, as they can provide valuable insights into type mismatches that might not be immediately obvious during development.”
Frequently Asked Questions (FAQs)
What does “conflicting types for function” mean in Linux syscall programming?
This error indicates that the function’s declaration differs from its definition or the expected type, leading to type mismatches in the program.
How can I resolve conflicting types for a syscall function?
To resolve this issue, ensure that the function’s declaration matches its definition. Verify the return type and parameter types in both the declaration and definition.
What are common causes of conflicting types errors in Linux programming?
Common causes include mismatched data types, incorrect function prototypes, or including headers that define functions differently than intended.
Can conflicting types for a function affect program execution?
Yes, conflicting types can lead to behavior, crashes, or incorrect results during program execution due to type mismatches.
Is it necessary to include header files for syscalls in Linux?
Yes, including the appropriate header files is essential as they provide the correct function prototypes and type definitions necessary for proper compilation.
How can I debug conflicting types errors in my code?
To debug, review the function declarations and definitions, check included headers, and use compiler flags like `-Wall` to identify type-related warnings.
In the context of Linux system calls, encountering an error related to “conflicting types for function” typically arises during the compilation of C programs. This issue indicates that the function’s declaration does not match its definition, leading to ambiguity in the type expected by the compiler. Such discrepancies can occur due to variations in function signatures, including differences in return types, parameter types, or the number of parameters. Addressing these errors is essential for ensuring that the program compiles successfully and operates as intended.
One of the primary causes of this error is the improper inclusion of header files or the absence of necessary prototypes. Developers must ensure that all function declarations are consistent across their codebase. Utilizing `include` directives correctly and maintaining proper function prototypes can significantly reduce the likelihood of conflicting types. Additionally, it is advisable to check for any redefinitions or mismatches in type definitions that may stem from multiple included headers.
Another key takeaway is the importance of adhering to C programming conventions and best practices. Clear and consistent naming conventions, along with thorough documentation of function signatures, can help mitigate confusion and prevent type conflicts. Furthermore, leveraging compiler warnings and employing static analysis tools can aid developers in identifying potential issues early in the development process, thus enhancing code
Author Profile
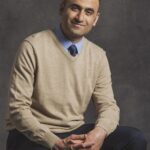
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?