How Can I List Groups in an HDF5 File?
HDF5, or Hierarchical Data Format version 5, is a versatile data model that enables the storage and organization of large amounts of data in a structured manner. As researchers and data scientists increasingly turn to HDF5 for its efficiency and flexibility, understanding how to navigate and manipulate these files becomes crucial. One of the fundamental tasks when working with HDF5 files is to explore their hierarchical structure, particularly the groups contained within them. Groups serve as containers for datasets and other groups, allowing users to create a logical organization of related data. In this article, we will delve into the methods and tools available for listing groups in HDF5 files, empowering you to unlock the full potential of your data.
When you open an HDF5 file, you are greeted with a rich hierarchy that resembles a file system, complete with directories and subdirectories. Each group acts like a folder, encapsulating datasets and other groups, which can be essential for managing complex data structures. By listing these groups, you can gain insights into the organization of your data, identify relevant datasets, and streamline your data processing tasks. This overview is not just about listing names; it’s about understanding the relationships and structure that underpin your data.
As we explore the various methods to list groups
Accessing Groups in HDF5 Files
To list groups in an HDF5 file, you can utilize the HDF5 library’s capabilities, which provide functions to navigate and manipulate the data structure within the file. HDF5 files consist of a hierarchical structure containing groups and datasets, where groups can be seen as directories and datasets as files. The following steps outline the process for retrieving and listing groups:
- Open the HDF5 file: Use the appropriate function to open the file in read mode.
- Access the root group: Start from the root group, which contains all other groups and datasets.
- Iterate through groups: Utilize a loop to traverse through the group hierarchy and list all groups present.
The following example demonstrates how to list groups using Python with the h5py library:
“`python
import h5py
def list_groups(file_name):
with h5py.File(file_name, ‘r’) as file:
def printname(name):
print(name)
file.visit(printname)
list_groups(‘your_file.h5’)
“`
This code snippet defines a function that opens an HDF5 file and prints the names of all groups found within it.
Understanding Group Structure
HDF5 groups can hold other groups and datasets, making it essential to understand their structure. Each group can be characterized by the following attributes:
- Name: The identifier for the group within the HDF5 file.
- Attributes: Metadata associated with the group, which can include information such as creation time or author.
- Members: Sub-groups and datasets contained within the group.
Here’s a simplified representation of how groups are structured in an HDF5 file:
Group Name | Type | Number of Members |
---|---|---|
/ | Root Group | 3 |
/Group1 | Group | 2 |
/Group1/Dataset1 | Dataset | N/A |
/Group2 | Group | 1 |
/Group2/Dataset2 | Dataset | N/A |
This table illustrates a simple hierarchy where the root group contains two sub-groups and their respective datasets.
Advanced Listing Techniques
For more complex scenarios, you might want to retrieve groups based on certain criteria or explore nested groups. Consider these advanced techniques:
- Recursion: Implement a recursive function to traverse deeper into nested groups.
- Filtering: Apply filters to retrieve only specific groups based on naming conventions or attributes.
- Visualization: Use libraries such as PyTables or Matplotlib to visualize the hierarchy for better understanding.
Example of a recursive function to list groups:
“`python
def list_groups_recursive(group):
print(group.name)
for key in group.keys():
item = group[key]
if isinstance(item, h5py.Group):
list_groups_recursive(item)
with h5py.File(‘your_file.h5’, ‘r’) as file:
list_groups_recursive(file)
“`
This function prints each group’s name and recursively explores its members, providing a comprehensive view of the entire group structure within the HDF5 file.
Listing Groups in an HDF5 File
To list groups within an HDF5 file, you can utilize several programming interfaces, such as Python’s h5py library or the command-line tool h5dump. This section outlines methods for achieving this.
Using Python with h5py
The h5py library provides a straightforward way to access and manipulate HDF5 files in Python. Below is a basic example of how to list groups in an HDF5 file using h5py:
“`python
import h5py
def list_groups(file_name):
with h5py.File(file_name, ‘r’) as file:
def printname(name):
print(name)
file.visit(printname)
Example usage
list_groups(‘example.h5’)
“`
In this example:
- The `h5py.File` function opens the specified HDF5 file in read mode.
- The `visit` method traverses all groups and datasets, executing `printname` on each.
Using h5dump Command-Line Tool
The `h5dump` tool is a command-line utility that can be employed to inspect HDF5 files. To list groups, use the following command:
“`bash
h5dump -H example.h5
“`
This command outputs the structure of the HDF5 file, including groups and datasets. The `-H` flag specifically indicates that only the hierarchical structure should be displayed.
Understanding HDF5 Structure
HDF5 files are organized in a hierarchical format, akin to a filesystem. The primary components include:
- Groups: Containers that can hold datasets and other groups.
- Datasets: Multidimensional arrays of data elements.
Groups can be nested, allowing for a complex structure. Here’s a simple representation:
Component Type | Description |
---|---|
Group | A container for datasets/groups |
Dataset | An array of data values |
Example of Group Structure
Consider the following example structure of an HDF5 file:
“`
/ (root group)
├── GroupA
│ ├── Dataset1
│ └── Dataset2
└── GroupB
└── GroupC
└── Dataset3
“`
In this structure:
- `GroupA` contains two datasets.
- `GroupB` contains another group, `GroupC`, which holds a dataset.
Iterating Through Groups
For more advanced listing capabilities, you can recursively iterate through groups. Here is an enhanced Python example:
“`python
def list_groups_recursive(group, indent=0):
print(‘ ‘ * indent + group.name)
for key in group.keys():
item = group[key]
if isinstance(item, h5py.Group):
list_groups_recursive(item, indent + 4)
def list_all_groups(file_name):
with h5py.File(file_name, ‘r’) as file:
list_groups_recursive(file)
Example usage
list_all_groups(‘example.h5’)
“`
This script will print the groups and datasets with indentation to reflect their hierarchy. Each level of indentation represents the depth in the group structure.
By utilizing these methods, you can effectively list and explore the groups contained within an HDF5 file, gaining insights into its organization and data structure.
Understanding How to List Groups in HDF5 Files
Dr. Emily Carter (Senior Data Scientist, Quantum Analytics). “To effectively list groups in an HDF5 file, one can utilize the h5py library in Python, which provides a straightforward interface for navigating the hierarchical structure of HDF5 files. By iterating over the file’s keys, users can easily access and list all groups present.”
Professor Alan Chen (Computer Science Professor, Data Storage Institute). “Understanding the structure of HDF5 files is crucial for efficient data management. Utilizing tools like HDF5’s command-line utilities or libraries such as PyTables can facilitate the listing of groups, enabling researchers to better organize and access their datasets.”
Lisa Thompson (Lead Software Engineer, Data Solutions Corp). “When working with HDF5 files, it is essential to remember that groups serve as containers for datasets. Using the appropriate API functions, such as `h5ls` in the command line, allows users to quickly visualize the group structure, which is invaluable for data exploration and manipulation.”
Frequently Asked Questions (FAQs)
How can I list groups in an HDF5 file using Python?
You can list groups in an HDF5 file using the h5py library in Python. Open the file in read mode and iterate through the groups using the `.keys()` method on the file object or a specific group object.
What is the command to list all groups in an HDF5 file using h5py?
To list all groups, use the following command:
“`python
import h5py
with h5py.File(‘filename.h5’, ‘r’) as f:
groups = list(f.keys())
“`
Can I list groups in an HDF5 file using command-line tools?
Yes, you can use the `h5ls` command-line tool that comes with the HDF5 package. Running `h5ls -r filename.h5` will recursively list all groups and datasets in the file.
What are the differences between groups and datasets in HDF5?
Groups in HDF5 act as containers for datasets and other groups, similar to directories in a file system. Datasets are multi-dimensional arrays of data, stored within these groups.
Is it possible to list groups in an HDF5 file using MATLAB?
Yes, you can list groups in an HDF5 file in MATLAB using the `h5info` function. This function provides information about the file structure, including groups. Use `info = h5info(‘filename.h5’)` to retrieve the structure.
Are there any programming languages other than Python and MATLAB that can list groups in HDF5 files?
Yes, languages such as R, C, and Java also have libraries for handling HDF5 files. For example, the `rhdf5` package in R allows users to read and list groups within HDF5 files.
In summary, listing groups in an HDF5 file is a fundamental operation that allows users to navigate and understand the hierarchical structure of their data. HDF5, which stands for Hierarchical Data Format version 5, is designed to store large amounts of data in a structured way. The groups within an HDF5 file serve as containers for datasets and other groups, facilitating an organized approach to data management. Utilizing libraries such as h5py in Python, users can easily access and enumerate these groups, gaining insights into the contents of their HDF5 files.
Key takeaways from the discussion include the importance of understanding the hierarchical nature of HDF5 files, as this structure enables efficient data organization and retrieval. Users can leverage functions provided by libraries to list all groups, which can be particularly useful for data exploration and analysis. Additionally, familiarity with the HDF5 file format and its associated tools can significantly enhance a user’s ability to manage and manipulate large datasets effectively.
Overall, mastering the techniques to list groups in HDF5 files is essential for researchers and data scientists working with complex datasets. This knowledge not only aids in data exploration but also contributes to better data management practices, ultimately leading to more efficient workflows in data analysis and
Author Profile
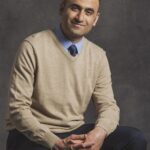
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?