How Can You Load Parameters into the Environment in R Studio?
In the world of data analysis and statistical computing, R has established itself as a powerhouse for researchers, analysts, and data enthusiasts alike. One of the key features that enhances the efficiency and effectiveness of R is its ability to manage and manipulate the environment seamlessly. A crucial aspect of this is the ability to load parameters into the environment, allowing users to streamline their workflows and enhance reproducibility. Whether you’re working on a complex data project or simply running a few analyses, understanding how to effectively load parameters can elevate your R programming experience.
Loading parameters into the R environment is not just a matter of convenience; it’s a fundamental practice that can significantly improve your coding efficiency. By storing and retrieving parameters from external files or scripts, you can maintain cleaner code, reduce redundancy, and make your analyses more transparent. This practice is especially beneficial when collaborating with others or revisiting projects after some time, as it allows for easier adjustments and updates to your analyses without the hassle of rewriting code.
Moreover, R provides various methods to load parameters, ranging from simple variable assignments to more complex configurations using packages designed for this purpose. Understanding these methods can empower users to create dynamic and adaptable R scripts that can respond to changing data or analysis requirements. As we delve deeper into the topic, we will explore the different
Loading Parameters into the Environment
Loading parameters into the R environment allows users to manage their workspace effectively. This is particularly useful for setting up analyses and ensuring that the environment is configured correctly to run scripts without modification. Below are common methods to load parameters into the R environment.
Using the `load()` Function
The `load()` function in R is designed to read R data files saved with the `.RData` extension. This method is efficient for loading multiple objects into the global environment at once.
- Syntax:
“`R
load(“path/to/your/file.RData”)
“`
- Example:
If you have a file named `my_data.RData`:
“`R
load(“my_data.RData”)
“`
This command imports all objects saved in that file into your current R session.
Using the `source()` Function
The `source()` function is useful for executing R scripts that may contain parameter definitions and other R code. This method is ideal for scripts that set up the environment or define functions.
- Syntax:
“`R
source(“path/to/your/script.R”)
“`
- Example:
To run a script called `setup.R`, you would use:
“`R
source(“setup.R”)
“`
This will execute the code within the script, loading any parameters defined there into the current environment.
Using Configuration Files
Configuration files, such as `.csv` or `.json`, can also be used to store parameters. These files can be read into R using functions like `read.csv()` or `fromJSON()` from the `jsonlite` package.
- Example with CSV:
“`R
params <- read.csv("params.csv")
```
- Example with JSON:
“`R
library(jsonlite)
params <- fromJSON("params.json")
```
Once loaded, parameters can be accessed as data frames or lists, depending on the file type.
Environment Management Best Practices
To maintain a clean working environment, consider the following practices:
- Clear the Environment: Use `rm(list = ls())` to remove all objects before loading new parameters.
- Use `.Rprofile`: Place frequently used parameter loading commands in an `.Rprofile` file, which R executes at startup.
- Document Changes: Keep a log of parameter changes in a README file for easy reference.
Common Parameter Types
Parameters can vary widely depending on the analysis. Here’s a quick overview of common types:
Parameter Type | Description |
---|---|
Data Paths | File locations for datasets or results |
Model Settings | Configurations for statistical models, such as hyperparameters |
Analysis Options | Flags for enabling or disabling features in analyses |
By employing these methods and best practices, users can effectively manage their R environment, leading to more efficient and reproducible analyses.
Methods to Load Parameters into the Environment in R Studio
Loading parameters into the R environment can significantly enhance the efficiency of your data analysis workflow. Here are several methods to achieve this:
Using R Scripts
You can define parameters directly in an R script file. This approach allows for easy modification and reusability.
- Create a new R script file (e.g., `params.R`).
- Define your parameters using assignment statements:
“`r
params.R
param1 <- 10
param2 <- "example"
param3 <- c(1, 2, 3, 4, 5)
```
- Source the file in your main R script to load the parameters:
“`r
source(“params.R”)
“`
This method ensures that all parameters are readily available in your R environment.
Using RMarkdown
RMarkdown allows you to define parameters that can be used throughout your document. This is particularly useful for reports and dynamic analysis.
- Define parameters in the YAML header of your RMarkdown file:
“`yaml
—
title: “My Analysis”
params:
param1: 10
param2: “example”
output: html_document
—
“`
- Access these parameters within your R code chunks using `params$param1`:
“`r
Example usage
print(params$param1)
“`
This method integrates parameter definitions directly into your documentation.
Loading from External Files
Parameters can also be loaded from external files, such as CSV or JSON, which is beneficial for larger datasets or configurations.
- CSV File: Create a `params.csv` file:
“`csv
param_name,param_value
param1,10
param2,example
“`
- Load the parameters into R:
“`r
params <- read.csv("params.csv")
param1 <- as.numeric(params[param_name == "param1", "param_value"])
param2 <- as.character(params[param_name == "param2", "param_value"])
```
- JSON File: Create a `params.json` file:
“`json
{
“param1”: 10,
“param2”: “example”
}
“`
- Load the parameters using the `jsonlite` package:
“`r
library(jsonlite)
params <- fromJSON("params.json")
param1 <- params$param1
param2 <- params$param2
```
Environment Variables
Using environment variables can help manage parameters across different R sessions without hardcoding values.
- Set an environment variable in your `.Renviron` file:
“`
PARAM1=10
PARAM2=example
“`
- Access the variables in your R script:
“`r
param1 <- as.numeric(Sys.getenv("PARAM1"))
param2 <- Sys.getenv("PARAM2")
```
This method helps maintain parameter values outside of your scripts, which can be beneficial for sensitive information.
Using R Packages
Some R packages, such as `config` and `dotenv`, offer structured ways to manage configurations.
- Using `config`:
- Create a `config.yml` file:
“`yaml
default:
param1: 10
param2: example
“`
- Load the configuration:
“`r
library(config)
config <- config::get()
param1 <- config$param1
param2 <- config$param2
```
- Using `dotenv`:
- Create a `.env` file:
“`
PARAM1=10
PARAM2=example
“`
- Load the environment variables:
“`r
library(dotenv)
dotenv::load_dotenv()
param1 <- as.numeric(Sys.getenv("PARAM1"))
param2 <- Sys.getenv("PARAM2")
```
These packages streamline the process of managing parameters and configurations within R.
Expert Insights on Loading Parameters into R Studio Environment
Dr. Emily Carter (Data Scientist, R Analytics Group). “Loading parameters into the environment in R Studio can significantly streamline your workflow. Utilizing the `load()` function allows you to import R data files directly into your session, ensuring that all your variables and data structures are readily available for analysis.”
James Liu (Senior R Programmer, Tech Solutions Inc.). “To effectively manage your workspace, it is crucial to understand the use of `.RData` files. By saving your workspace with the `save.image()` function, you can easily load all parameters back into the environment when you start a new session, thereby maintaining continuity in your projects.”
Linda Thompson (Statistical Consultant, Data Insights LLC). “For those looking to load parameters from external sources, consider using the `read.csv()` or `readRDS()` functions. These methods not only import data but also allow for more complex data structures, which can enhance your analytical capabilities in R Studio.”
Frequently Asked Questions (FAQs)
How can I load parameters into the environment in R Studio?
You can load parameters into the environment in R Studio by using the `load()` function, which retrieves objects stored in an R data file. Alternatively, you can assign values to parameters directly in the console or scripts.
What file formats can I use to load parameters into R Studio?
R Studio supports loading parameters from RData files using the `load()` function. You can also use CSV, TXT, or other text-based formats with functions like `read.csv()` or `read.table()` to import data.
Can I load parameters from an external script in R Studio?
Yes, you can source an external R script that contains parameter definitions using the `source()` function. This will execute the script and load all defined parameters into your current environment.
Is it possible to load parameters conditionally in R Studio?
Yes, you can load parameters conditionally by using control structures such as `if` statements within your scripts. This allows you to load specific parameters based on certain conditions.
How do I check which parameters are currently loaded in the R environment?
You can check the currently loaded parameters in the R environment by using the `ls()` function, which lists all objects in the environment, or `str()` to view the structure of specific objects.
What should I do if I encounter an error while loading parameters?
If you encounter an error while loading parameters, check the file path for accuracy, ensure the file format is compatible, and verify that the objects in the file match your expectations. Use the `traceback()` function for debugging.
In R Studio, loading parameters into the environment is a critical step for data analysis and modeling. This process typically involves defining variables, importing datasets, or configuring settings that will be utilized throughout the R session. Users can load parameters from various sources, including R scripts, external files, or directly from the console, ensuring that the necessary data and configurations are readily available for analysis.
One effective method for loading parameters is through the use of the `source()` function, which allows users to execute R scripts containing predefined variables and functions. Additionally, the `load()` function can be employed to import R data files, while the `read.csv()` function is useful for loading data from CSV files. These techniques facilitate a streamlined workflow, enabling analysts to set up their environment efficiently and focus on the analytical tasks at hand.
Moreover, leveraging R’s environment management capabilities, such as the `.Rprofile` file, can automate the loading of parameters each time R Studio is opened. This ensures consistency and saves time, particularly for repetitive tasks. Understanding these methods not only enhances productivity but also fosters a more organized approach to managing data and parameters within R Studio.
Author Profile
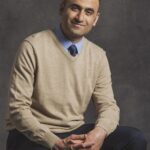
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?