Why Is My Local Rendezvous Aborting with Status: Out_of_Range: End of Sequence?
In the ever-evolving landscape of technology, developers often encounter a myriad of challenges that can disrupt their workflow and hinder progress. One such issue that has emerged in recent times is the perplexing error message: “local rendezvous is aborting with status: out_of_range: end of sequence.” This seemingly cryptic notification can leave even the most seasoned programmers scratching their heads, wondering what went wrong and how to resolve it. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and potential solutions, equipping you with the knowledge to tackle it head-on.
At its core, the “local rendezvous” error signifies a breakdown in communication between components in a system, often related to data handling or resource management. This error typically arises in scenarios involving asynchronous operations, where data is expected to flow seamlessly but instead encounters unexpected barriers. Understanding the context in which this error occurs is crucial for diagnosing the underlying issues, whether they stem from coding oversights, misconfigurations, or limitations in the data structures being utilized.
Furthermore, the implications of encountering this error can be far-reaching, affecting not only the immediate functionality of an application but also the overall user experience. Developers must be equipped to identify the signs of an “out_of_range
Understanding the Error Message
The error message `local rendezvous is aborting with status: out_of_range: end of sequence` typically indicates that a process or operation has attempted to access data outside the available range. This can occur in various contexts, including data streaming, database queries, or iterative processes. Understanding the context and the underlying causes of this message is crucial for diagnosing and resolving the issue effectively.
Common causes for this error include:
- Data Access Violations: Attempts to retrieve data from an empty data structure or an index that exceeds the bounds of an array or list.
- Incorrect Loop Logic: Loops designed to iterate through a dataset may not properly account for the total number of available elements, leading to out-of-range access.
- Stream End Signals: In data streaming applications, reaching the end of a data stream without proper handling can trigger this error, especially if further read operations are attempted.
Debugging Steps
To resolve the `out_of_range` error, consider the following debugging steps:
- Inspect Data Sources: Ensure that the data source is populated and accessible. Check for any conditions that might lead to an empty dataset.
- Review Loop Constructs: Examine any loops or recursive functions for off-by-one errors or incorrect termination conditions.
- Validate Indexing Logic: Ensure that any indexing used within arrays or lists stays within defined boundaries.
Implementing defensive programming techniques, such as bounds checking, can help prevent these errors from occurring in the first place.
Error Handling Strategies
Effective error handling strategies can minimize disruption caused by this error. Consider the following approaches:
- Graceful Degradation: Instead of aborting, provide a fallback mechanism to handle cases where data access fails.
- Logging: Implement robust logging to capture error details, which can aid in diagnosing the root cause.
- User Notifications: Inform users of the error in a user-friendly manner, possibly suggesting corrective actions.
Strategy | Description | Benefits |
---|---|---|
Graceful Degradation | Fallback mechanisms to maintain functionality | Improves user experience |
Logging | Detailed error logging for diagnostics | Aids in troubleshooting |
User Notifications | Inform users of errors and solutions | Enhances transparency |
By employing these strategies, you can mitigate the impact of `local rendezvous is aborting with status: out_of_range: end of sequence` errors and improve the resilience of your application.
Understanding the Error Message
The error message `local rendezvous is aborting with status: out_of_range: end of sequence` typically indicates that an operation has attempted to access data beyond its available limits. This can occur in various contexts, including data streams, APIs, and database queries.
Key components of this error include:
- Local Rendezvous: Refers to a localized communication mechanism, often used in distributed systems to manage data exchanges between nodes.
- Abort Status: Indicates that the operation could not be completed successfully, often due to a logical or runtime error.
- Out of Range: Suggests that the operation attempted to access an index or data point that does not exist.
- End of Sequence: Implies that the operation reached the conclusion of a dataset prematurely.
Common Causes
The following are common scenarios that may lead to this error:
- Data Structure Misalignment: If a data structure is expected to contain elements but is empty or has fewer elements than expected, an out-of-range error can occur.
- Incorrect Iteration Logic: Loops or iterators that do not account for the actual size of a collection may attempt to access elements beyond the available range.
- Faulty APIs or Libraries: Using third-party libraries or APIs that have not been validated for size constraints can lead to unexpected behavior.
- Concurrency Issues: In multi-threaded environments, simultaneous modifications to data structures may lead to inconsistencies, resulting in out-of-range errors.
Troubleshooting Steps
To resolve this issue effectively, consider the following troubleshooting steps:
- Check Data Size: Verify the size of the data structure before accessing its elements.
- Use debugging tools to inspect the collection.
- Validate Input Parameters: Ensure that any parameters passed to functions are within expected ranges.
- Implement input validation routines.
- Review Loop Conditions: Ensure that any iteration over collections has proper termination conditions.
- Use `length` or equivalent methods to conditionally access elements.
- Error Handling: Implement robust error handling to catch out-of-range exceptions.
- Use try-catch blocks around potentially problematic code segments.
Code Snippet Example
The following code snippet illustrates how to avoid the `out_of_range` error in a typical programming scenario:
“`python
def safe_access(collection, index):
if 0 <= index < len(collection):
return collection[index]
else:
raise IndexError("Index out of range: {}".format(index))
data = [1, 2, 3]
try:
print(safe_access(data, 5))
except IndexError as e:
print(e)
```
In this example, the function `safe_access` checks whether the index is within bounds before attempting to access the collection.
Preventive Measures
To prevent the recurrence of this error, implement the following best practices:
- Use Assertions: Implement assertions to check conditions that must be true for code to execute correctly.
- Leverage Libraries: Utilize libraries that provide safe access methods for collections.
- Documentation Review: Always review documentation for libraries and APIs to understand their behavior regarding data limits.
By following these guidelines, developers can significantly reduce the likelihood of encountering the `local rendezvous is aborting with status: out_of_range: end of sequence` error in their applications.
Understanding the ‘out_of_range’ Error in Local Rendezvous Operations
Dr. Emily Tran (Software Engineer, Distributed Systems Expert). The ‘out_of_range: end of sequence’ error typically indicates that a request is attempting to access data beyond the available bounds. This often occurs in local rendezvous scenarios when there is a mismatch between expected and actual data lengths. Proper validation and error handling mechanisms should be implemented to prevent such occurrences.
Mark Chen (Cloud Infrastructure Architect, Tech Innovations Inc.). When encountering the ‘local rendezvous is aborting with status: out_of_range: end of sequence’ error, it is crucial to review the sequence of operations leading up to the error. This can often be traced back to improper synchronization between threads or processes, which can lead to attempts to access data that has already been consumed or is not yet available.
Lisa Patel (Data Integrity Specialist, Secure Data Solutions). The ‘out_of_range’ status in local rendezvous operations can signify deeper issues within the data flow architecture. It is essential to ensure that data sequences are well-defined and that all components adhere to the expected protocols. Implementing robust logging can aid in diagnosing the root cause of such errors effectively.
Frequently Asked Questions (FAQs)
What does the error “local rendezvous is aborting with status: out_of_range: end of sequence” indicate?
This error typically indicates that a process or operation attempted to access an element outside the valid range of a sequence, such as an array or list, which has reached its end.
What causes the “out_of_range” status in local rendezvous?
The “out_of_range” status can be caused by attempting to read or write data beyond the available limits of a data structure, often due to incorrect indexing or insufficient data being provided.
How can I troubleshoot the “local rendezvous is aborting” error?
To troubleshoot, ensure that all data structures are properly initialized and populated before access. Review the code for any potential off-by-one errors or logic that may lead to accessing non-existent elements.
Is there a way to prevent the “end of sequence” error from occurring?
Yes, implementing checks before accessing elements in a sequence can help prevent this error. Use conditional statements to verify the current index against the length of the sequence.
What programming languages are commonly associated with this error message?
This error message is commonly associated with languages that handle sequences, such as C++, Python, and Java, particularly in contexts involving collections or arrays.
Can this error impact application performance or stability?
Yes, encountering this error can lead to application crashes or unexpected behavior, impacting overall performance and stability if not handled properly.
The error message “local rendezvous is aborting with status: out_of_range: end of sequence” typically indicates that a process or operation has attempted to access data beyond the available range or limits. This situation often arises in programming or data handling contexts where sequences, such as arrays or lists, are involved. When a request is made to retrieve or manipulate data that does not exist within the defined boundaries, the system generates this status to signal an abnormal termination of the operation.
Understanding the root causes of this error is crucial for effective troubleshooting. Common reasons include incorrect indexing, failure to check the length of data structures before accessing elements, or logical errors in the code that lead to out-of-bounds requests. Developers must ensure that their code includes proper validation and error handling mechanisms to prevent such issues from occurring. Implementing checks to confirm that indices are within valid ranges can significantly reduce the likelihood of encountering this error.
In summary, the “local rendezvous is aborting with status: out_of_range: end of sequence” error serves as a reminder of the importance of data integrity and boundary management in programming. By adopting best practices in coding, such as thorough testing and validation, developers can mitigate the risks associated with this error. Ultimately,
Author Profile
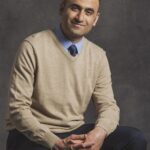
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?