Why Am I Getting an Error When I Try to Concatenate a Boolean Value in Lua?
In the world of programming, few languages are as versatile and approachable as Lua. Known for its lightweight design and ease of integration, Lua has become a favorite among game developers and embedded systems alike. However, like any programming language, it comes with its own set of quirks and challenges. One common pitfall that developers encounter is the infamous error message: “attempt to concatenate a boolean value.” This seemingly cryptic message can leave both novice and experienced programmers scratching their heads, wondering where they went wrong. In this article, we will unravel the mystery behind this error, explore its causes, and provide practical solutions to help you navigate the intricacies of Lua programming.
Understanding the “attempt to concatenate a boolean value” error is crucial for anyone working with Lua, as it often arises from a misunderstanding of data types and their operations. In Lua, concatenation is typically reserved for strings, and attempting to concatenate a boolean value—true or —can lead to unexpected behavior and runtime errors. This situation often stems from logical conditions or function returns that do not align with the expected string data type, creating a frustrating roadblock for developers.
As we delve deeper into this topic, we will examine common scenarios that lead to this error, the importance of type checking, and
Understanding the Error
The error message “attempt to concatenate a boolean value” in Lua typically arises when a script attempts to concatenate a boolean variable using the concatenation operator (`..`). In Lua, the `..` operator is used to join strings, and it is not designed to handle boolean values directly. When such an operation is attempted, Lua throws an error, indicating the type mismatch.
To resolve this error, it is essential to ensure that all operands involved in a concatenation operation are indeed strings. If a boolean value is being concatenated, it should be converted to a string first.
Common Causes
Several scenarios can lead to this error, including:
- Direct Concatenation: Attempting to concatenate a boolean variable without converting it.
- Function Returns: Functions that may return a boolean value instead of a string.
- Conditional Statements: Using boolean expressions directly in concatenation without proper handling.
Example Scenarios
Consider the following examples that illustrate how this error may occur:
“`lua
local isActive = true
local message = “The status is: ” .. isActive — This will cause an error
“`
To correct this, convert the boolean to a string:
“`lua
local isActive = true
local message = “The status is: ” .. tostring(isActive) — Correct usage
“`
Best Practices
To avoid encountering the “attempt to concatenate a boolean value” error, adhere to the following best practices:
- Always check the data types of variables before concatenation.
- Use `tostring()` to convert non-string values safely.
- Implement proper error handling to catch potential issues early.
Error Handling Techniques
Utilizing error handling can provide a robust defense against this issue. Below is a simple error handling structure:
“`lua
local function safeConcatenate(a, b)
if type(a) == “boolean” then a = tostring(a) end
if type(b) == “boolean” then b = tostring(b) end
return a .. b
end
local result = safeConcatenate(“Status: “, ) — Returns “Status: ”
“`
Summary Table of Data Types
The following table outlines the common data types in Lua and their appropriate handling when concatenating:
Data Type | Concatenation Method |
---|---|
String | No conversion needed |
Number | Use tostring(value) |
Boolean | Use tostring(value) |
Table | Convert to string representation if necessary |
By following these guidelines, developers can effectively manage data types in Lua and prevent common errors associated with improper concatenation.
Understanding the Error
In Lua, the error message “attempt to concatenate a boolean value” occurs when the code attempts to concatenate a boolean variable with a string. Lua does not support concatenation of boolean values (`true` or “) directly with strings. Instead, such an operation results in a runtime error.
Common Scenarios Leading to the Error
- **Direct Concatenation**:
- Attempting to concatenate a boolean directly with a string.
“`lua
local isActive = true
local message = “The status is: ” .. isActive — This will cause the error
“`
- **Function Return Values**:
- Functions returning boolean values that are directly concatenated.
“`lua
function checkCondition()
return true
end
local output = “Condition met: ” .. checkCondition() — This will cause the error
“`
- **Conditional Statements**:
- Using boolean expressions in string concatenation.
“`lua
local x = 10
local y = 20
local result = “Is x greater than y? ” .. (x > y) — This will cause the error
“`
How to Fix the Error
To resolve the “attempt to concatenate a boolean value” error, you can convert the boolean value to a string before attempting to concatenate it. This can be achieved using the `tostring()` function.
Example Fixes
- **Using `tostring()`**:
“`lua
local isActive = true
local message = “The status is: ” .. tostring(isActive) — Correctly concatenates
“`
- **Function Output Handling**:
“`lua
function checkCondition()
return true
end
local output = “Condition met: ” .. tostring(checkCondition()) — Correctly concatenates
“`
- **Conditional Statements**:
“`lua
local x = 10
local y = 20
local result = “Is x greater than y? ” .. tostring(x > y) — Correctly concatenates
“`
Best Practices to Avoid the Error
To minimize the occurrence of this error in your Lua code, consider the following practices:
- Explicit Conversion: Always convert boolean values to strings before concatenation using `tostring()`.
- Type Checking: Use type checking to ensure that variables are of the expected type before performing operations.
- Debugging Output: When debugging, ensure any output statements clearly indicate the types of variables being processed.
Practice | Description |
---|---|
Explicit Conversion | Use `tostring()` to convert booleans before concatenation. |
Type Checking | Verify the types of variables using `type()` function. |
Debugging Output | Print variable types and values for clarity during development. |
By adhering to these practices, you can effectively prevent the “attempt to concatenate a boolean value” error and enhance the robustness of your Lua scripts.
Understanding the Lua Error: Attempt to Concatenate a Boolean Value
Dr. Emily Carter (Senior Software Engineer, Lua Development Team). “The error ‘attempt to concatenate a boolean value’ in Lua typically arises when a programmer mistakenly tries to concatenate a boolean variable with a string. This is a common oversight, especially for those new to the language, as Lua does not automatically convert booleans to strings during concatenation.”
Mark Thompson (Programming Language Researcher, Tech Innovations Lab). “In Lua, the concatenation operator is represented by ‘..’. When you encounter this error, it is crucial to check the types of all variables involved in the concatenation. Ensuring that all components are strings will prevent this error and enhance code reliability.”
Lisa Chen (Lead Lua Instructor, Coding Academy). “Understanding type handling in Lua is essential for effective programming. The ‘attempt to concatenate a boolean value’ error serves as a reminder to always validate variable types before performing operations. Utilizing functions like tostring() can help convert booleans to strings when necessary.”
Frequently Asked Questions (FAQs)
What does the error “attempt to concatenate a boolean value” mean in Lua?
This error occurs when you try to use the concatenation operator (`..`) on a boolean value. Lua does not support concatenating booleans with strings or other types directly.
How can I fix the “attempt to concatenate a boolean value” error?
To resolve this error, ensure that all values being concatenated are strings. You can convert a boolean to a string using the `tostring()` function before concatenation.
What are common scenarios that lead to this error in Lua?
Common scenarios include mistakenly using a boolean result from a conditional statement or function call in a string concatenation operation without proper conversion.
Can I concatenate boolean values directly in Lua?
No, Lua does not allow direct concatenation of boolean values. You must convert them to strings first to avoid the error.
What is the proper way to check a boolean value before concatenation?
You can use an `if` statement to check the boolean value and then concatenate accordingly, or use the `tostring()` function to ensure the value is a string before concatenation.
Are there any best practices to avoid this error in Lua programming?
To avoid this error, always validate the types of variables before concatenation and utilize type conversion functions like `tostring()` when necessary. Additionally, consider using debugging tools to identify problematic concatenation attempts.
In Lua, the error message “attempt to concatenate a boolean value” typically arises when a programmer tries to concatenate a boolean value (true or ) with a string using the concatenation operator (`..`). This operator is designed to combine strings, and attempting to use it with a boolean type results in a runtime error. Understanding the types of values being manipulated is crucial to avoiding this common pitfall in Lua programming.
To resolve this issue, developers should ensure that all values being concatenated are strings. If a boolean value is involved, it should be converted to a string using the `tostring()` function before concatenation. This approach not only prevents errors but also enhances code readability and maintainability by making the data types explicit.
Additionally, this error serves as a reminder of the importance of type checking in programming. Lua is a dynamically typed language, which means that types are determined at runtime. As such, developers should be vigilant about the types of variables they are working with, especially when performing operations that expect specific types. Proper error handling and type validation can significantly reduce the occurrence of such errors in Lua applications.
Author Profile
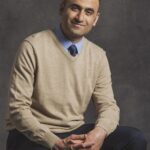
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?